PDF (portable document format) serves as a reliable and standardized platform for information dissemination.
In this blog, we’ll disclose the top 10 features of a C# PDF Library. These are the features that every PDF library should incorporate to ensure a seamless, efficient, and robust experience for developers.
The top 10 must-have features in a C# PDF Library are:
- PDF creation
- Text manipulation
- Page management
- Graphics and images
- Annotations and forms
- Security and encryption
- Digital signatures
- PDF data extraction
- Optical character recognition (OCR)
- Text-to-PDF and PDF-to-text conversion
Surprisingly, you can enjoy all these features in the Syncfusion C# PDF Library. Let’s explore them with code examples.

Transform your PDF files effortlessly in C# with just five lines of code using Syncfusion's comprehensive PDF Library!
PDF creation
Syncfusion’s .NET PDF Library provides a powerful solution to generate PDFs and seamlessly integrate text, images, and diverse elements. Whether your goal is crafting reports, producing invoices, or constructing customized PDFs, Syncfusion’s capabilities enable you to prepare professional, dynamic, and visually captivating PDFs within your apps.
The following code example shows how to generate a basic PDF document containing text, images, and shapes using C#.
//Create a new PDF document. PdfDocument document = new PdfDocument(); //Set the page size. document.PageSettings.Size = PdfPageSize.A4; //Add a page to the document. PdfPage page = document.Pages.Add(); //Create PDF graphics for the page. PdfGraphics graphics = page.Graphics; //Set the font. PdfFont font = new PdfStandardFont(PdfFontFamily.Helvetica, 20); //Draw the text. graphics.DrawString("Adventure Cycle Works", font, PdfBrushes.IndianRed, new Syncfusion.Drawing.PointF(150, 50)); //Load the image from the disk. FileStream imageStream = new FileStream("AdventureCycle.jpg", FileMode.Open, FileAccess.Read); PdfBitmap image = new PdfBitmap(imageStream); //Draw the image. graphics.DrawImage(image, new RectangleF(150,100,200,100)); //Initialize PdfPen to draw the polygon. PdfPen pen = new PdfPen(PdfBrushes.Brown, 10f); //Initialize PdfLinearGradientBrush for drawing the polygon PdfLinearGradientBrush brush = new PdfLinearGradientBrush(new PointF(10, 100), new PointF(100, 200), new PdfColor(Color.Red), new PdfColor(Color.Green)); //Create the polygon points. PointF p1 = new PointF(10, 100); PointF p2 = new PointF(10, 200); PointF p3 = new PointF(100, 100); PointF p4 = new PointF(100, 200); PointF p5 = new PointF(55, 150); PointF[] points = { p1, p2, p3, p4, p5 }; //Draw the polygon on PDF document. page.Graphics.DrawPolygon(pen, brush, points); //Create file stream. using (FileStream outputFileStream = new FileStream(Path.GetFullPath(@"Output.pdf"), FileMode.Create, FileAccess.ReadWrite)) { //Save the PDF document to file stream. document.Save(outputFileStream); } //Close the document. document.Close(true);
By executing this code example, you will get a PDF document like the following image.
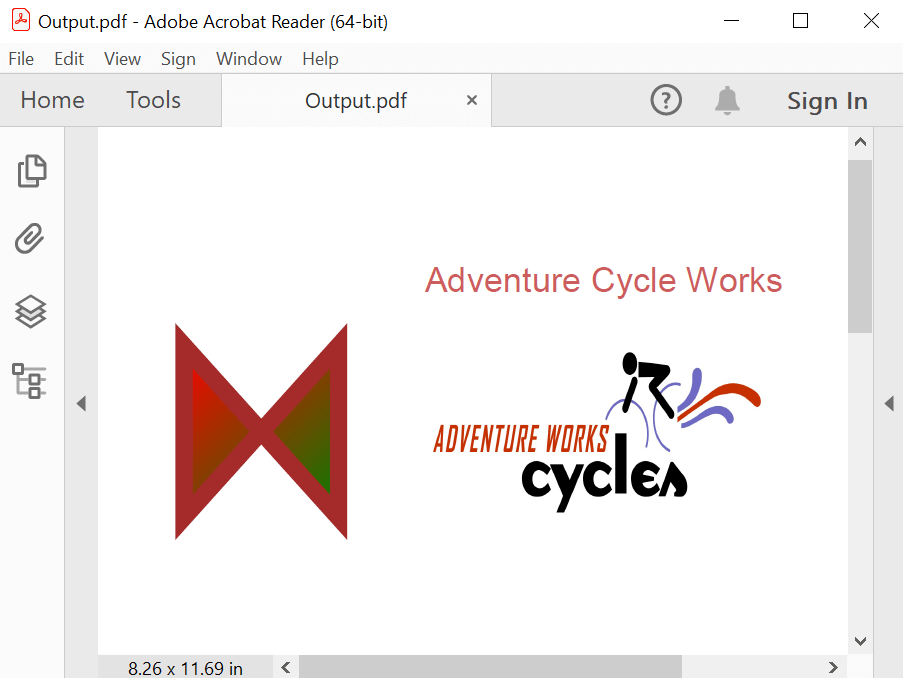
Note: Refer to the Create PDF file documentation for more information.
PDF data extraction
The Syncfusion C# PDF Library provides a comprehensive set of APIs for parsing PDF files. These APIs can extract text, images, and metadata or perform more advanced tasks. The library also provides APIs for manipulating PDF files, such as adding, removing, and editing content.
The following code example explains how to extract text, images, document information, and metadata from an existing PDF document using C#.
//Load the PDF document. FileStream docStream = new FileStream("Input.pdf", FileMode.Open, FileAccess.Read); //Load the PDF document. PdfLoadedDocument loadedDocument = new PdfLoadedDocument(docStream); //Load the first page. PdfPageBase firstPage = loadedDocument.Pages[0]; PdfPageBase secondPage = loadedDocument.Pages[1]; //Extract text from first page. string extractedText = firstPage.ExtractText(); File.WriteAllText("ExtractedText.txt", extractedText); //Extract images from first page. Stream[] extractedImages = secondPage.ExtractImages(); for (int i = 0; i < extractedImages.Length; i++) { FileStream imageStream = new FileStream("ExtractedImage" + i + ".png", FileMode.Create, FileAccess.Write); extractedImages[i].CopyTo(imageStream); imageStream.Dispose(); } //Extracting metadata and document information. PdfDocumentInformation documentInformation = loadedDocument.DocumentInformation; XmpMetadata metadata = loadedDocument.DocumentInformation.XmpMetadata; //Close the document. loadedDocument.Close(true);
Refer to the following output images.
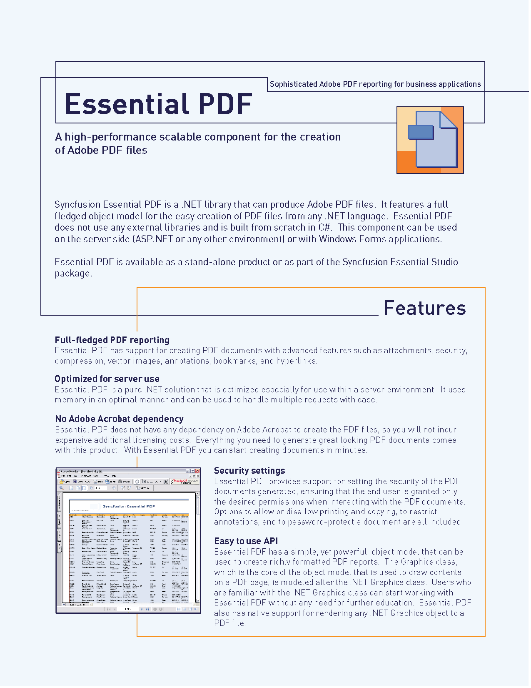
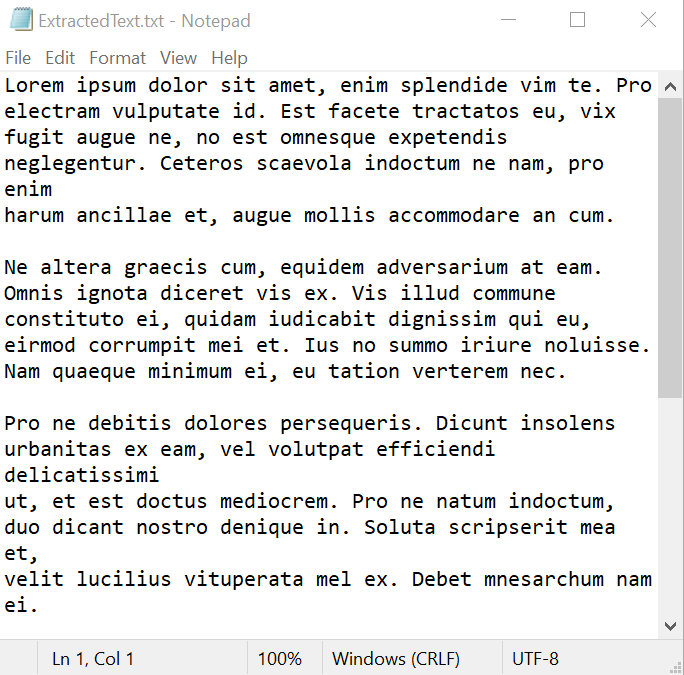
Note: For more details, refer to the extracting text , images, and XMP metadata documentation for PDF Library.

Say goodbye to tedious PDF tasks and hello to effortless document processing with Syncfusion's PDF Library.
Text manipulation
Text manipulation is a fundamental part of working with PDF documents, whether it’s adding text, finding existing content, extracting information, replacing fonts, and embedding fonts.
Syncfusion‘s .NET C# PDF Library simplifies text manipulation tasks, making it a powerful tool for users working with PDFs.
The following code example explains adding, finding, and extracting text in a PDF document.
//Load the PDF document. FileStream docStream = new FileStream("Input.pdf", FileMode.Open, FileAccess.Read); PdfLoadedDocument loadedDocument = new PdfLoadedDocument(docStream); //Get first page from document. PdfLoadedPage loadedPage = loadedDocument.Pages[0] as PdfLoadedPage; //Create PDF graphics for the page. PdfGraphics graphics = loadedPage.Graphics; //Set the standard font. PdfFont font = new PdfStandardFont(PdfFontFamily.Helvetica, 20); //Draw the text. graphics.DrawString("Hello World!!!", font, PdfBrushes.Black, new Syncfusion.Drawing.PointF(0, 0)); //Returns page number and rectangle positions of the text matches. Dictionary<int, List<Syncfusion.Drawing.RectangleF>> matchRects = new Dictionary<int, List<Syncfusion.Drawing.RectangleF>>(); loadedDocument.FindText("document", out matchRects); for (int i = 0; i < loadedDocument.Pages.Count; i++) { List<RectangleF> rectCoords = matchRects[i]; for (int j = 0; j < rectCoords.Count; j++) { RectangleF bounds = new RectangleF(rectCoords[j].X, rectCoords[j].Y, rectCoords[j].Width, rectCoords[j].Height); loadedDocument.Pages[i].Graphics.DrawRectangle(PdfPens.Red, bounds); } } //Extract text from first page. string extractedText = loadedPage.ExtractText(); //Create file stream. using (FileStream outputFileStream = new FileStream(Path.GetFullPath(@"Output.pdf"), FileMode.Create, FileAccess.ReadWrite)) { //Save the PDF document to file stream. loadedDocument.Save(outputFileStream); } //Close the document. loadedDocument.Close(true);
By executing this code example, you will get a PDF document like the following image.
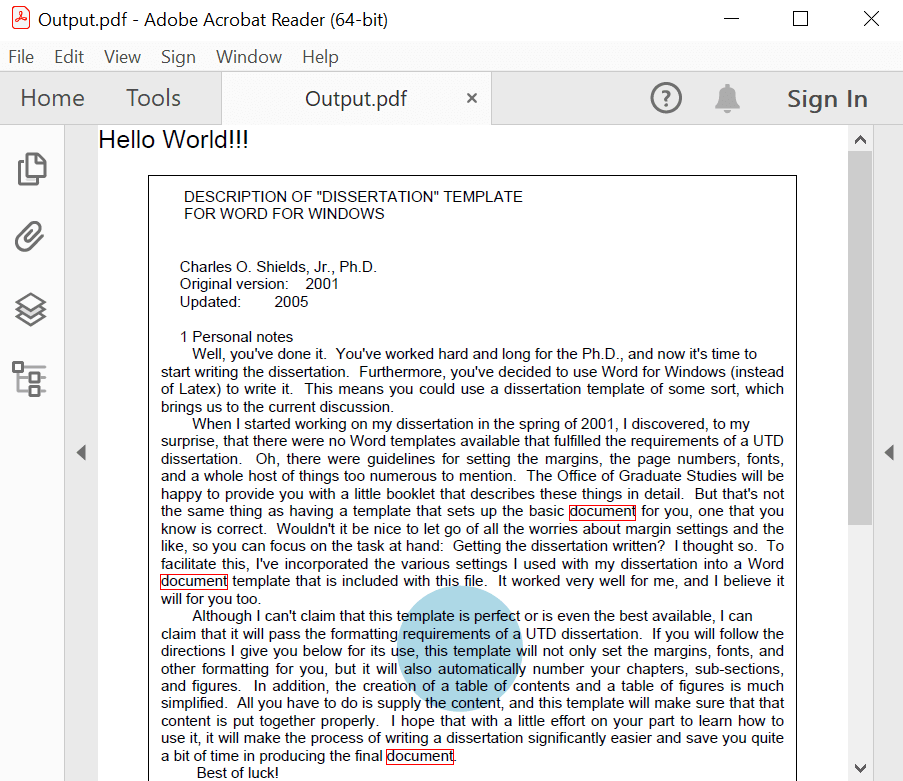
Note: Refer to the edit or manipulate PDF documentation for more details.
Page management
Syncfusion provides a comprehensive set of APIs for managing pages in PDF documents. These APIs can add, remove, rotate, and rearrange pages. They also perform page splitting, page scaling, page numbering, merging PDF documents, and splitting documents.
Key features of page management:
- Add, remove, insert, and rotate pages: You can easily add, remove, insert, crop and rotate pages in a PDF document using the corresponding APIs.
- Get and set page properties: You can get and set the properties of a page, such as its size, orientation, margin, and background color.
- Merge and split PDF documents: You can merge multiple PDF documents into one document or split a PDF document into multiple documents.
Note: Refer to the page manipulation in PDF documentation for more details.
Insert, remove, rotate, and rearrange the pages
The following code example explains how to insert, remove, and rearrange pages in a PDF document using C#.
//Load the PDF document. FileStream docStream = new FileStream("Input.pdf", FileMode.Open, FileAccess.Read); PdfLoadedDocument loadedDocument = new PdfLoadedDocument(docStream); //Rearrange the page by index. loadedDocument.Pages.ReArrange(new int[] { 2, 1, 0 }); //Insert a new page at the beginning of the document. loadedDocument.Pages.Insert(0); loadedDocument.Pages[0].Graphics.DrawString("Regular Page", new PdfStandardFont(PdfFontFamily.Helvetica, 20), PdfBrushes.Black, new PointF(0, 0)); //Remove the first page in the PDF document. loadedDocument.Pages.RemoveAt(3); //Create file stream. using (FileStream outputFileStream = new FileStream(Path.GetFullPath(@"Output.pdf"), FileMode.Create, FileAccess.ReadWrite)) { //Save the PDF document to file stream. loadedDocument.Save(outputFileStream); } //Close the document. loadedDocument.Close(true);
Merge and split PDF documents
Merge PDF documents
You can easily merge PDF files into a single, organized file using the Syncfusion .NET C# PDF Library. This only requires a few simple steps to accomplish.
Note: You can refer to the merge PDF files documentation for more information.
Here’s an example of how to merge PDF files using C# code.
// Create a PDF document. using (PdfDocument finalDocument = new PdfDocument()) { // Get the stream from an existing PDF documents. using (FileStream firstStream = new FileStream("File1.pdf", FileMode.Open, FileAccess.Read)) using (FileStream secondStream = new FileStream("File2.pdf", FileMode.Open, FileAccess.Read)) { // Create a PDF stream for merging. Stream[] streams = { firstStream, secondStream }; // Merge PDF documents. PdfDocumentBase.Merge(finalDocument, streams); // Save the document into a stream. using (MemoryStream outputStream = new MemoryStream()) { finalDocument.Save(outputStream); } } }
Split PDF document
Here’s an example of how to split a PDF file using C# code.
// Create the FileStream object. using (FileStream inputFileStream = new FileStream("Input.pdf", FileMode.Open, FileAccess.Read)) { // Create a PdfLoadedDocument object from the FileStream object. PdfLoadedDocument loadedDocument = new PdfLoadedDocument(inputFileStream); // Iterate over the pages in the PdfLoadedDocument object. for (int pageIndex = 0; pageIndex < loadedDocument.PageCount; pageIndex++) { // Create a new PdfDocument object. using (PdfDocument outputDocument = new PdfDocument()) { // Import the page from the PdfLoadedDocument object to the PdfDocument object. outputDocument.ImportPage(loadedDocument, pageIndex); //Save the document into a filestream object. using (FileStream outputFileStream = new FileStream("Output" + pageIndex + ".pdf", FileMode.Create, FileAccess.Write)) { outputDocument.Save(outputFileStream); } } } }
Note: For more details, refer to the split PDF documentation.

Boost your productivity with Syncfusion's PDF Library! Gain access to invaluable insights and tutorials in our extensive documentation.
Graphics and images
Syncfusion provides a wide range of robust tools and capabilities for unlocking the complete potential of graphics and images. Integrating these elements elevates the visual appeal and comprehensibility of your PDF documents.
Here’s how you can use Syncfusion to add graphics to your PDFs:
- Adding images: You can insert, resize, and crop images sourced from diverse locations. Additionally, you can perform tasks like image masking, image pagination, image replacement, conversion from multipage TIFF to PDF, and image removal.
- Drawing shapes: Create custom shapes (polygon, line, curve, path, rectangle, pie, arc, Bezier, and ellipse), diagrams, and annotations within your documents.
- Vector graphics: You can add vector graphics to your PDFs, ensuring they maintain quality and sharpness regardless of the display size.
- Interactive elements: Make your PDFs more engaging by adding interactive elements such as buttons and hyperlinks to graphics and images.
Refer to the following code example of converting a multiple-page TIFF to a PDF document.
//Create a PDF document. PdfDocument document = new PdfDocument(); //Setting the margin size of all the pages to zero. document.PageSettings.Margins.All = 0; //Load the multi-frame TIFF image from the disk. FileStream imageStream = new FileStream("image.tiff", FileMode.Open, FileAccess.Read); PdfTiffImage tiffImage = new PdfTiffImage(imageStream); //Get the frame count. int frameCount = tiffImage.FrameCount; //Access each frame and draw into the page. for (int i = 0; i < frameCount; i++) { //Add a section to the PDF document. PdfSection section = document.Sections.Add(); //Set page margins. section.PageSettings.Margins.All = 0; tiffImage.ActiveFrame = i; //Create a PDF unit converter instance. PdfUnitConvertor converter = new PdfUnitConvertor(); //Convert to point. Syncfusion.Drawing.SizeF size = converter.ConvertFromPixels(tiffImage.PhysicalDimension, PdfGraphicsUnit.Point); //Set page orientation. section.PageSettings.Orientation = (size.Width > size.Height) ? PdfPageOrientation.Landscape : PdfPageOrientation.Portrait; //Set page size. section.PageSettings.Size = size; //Add a page to the section. PdfPage page = section.Pages.Add(); //Draw TIFF image intoin the PDF page. page.Graphics.DrawImage(tiffImage, Syncfusion.Drawing.PointF.Empty, size); } //Create file stream. using (FileStream outputFileStream = new FileStream(Path.GetFullPath("Output.pdf"), FileMode.Create, FileAccess.ReadWrite)) { //Save the PDF document to file stream. document.Save(outputFileStream); } //Close the document. document.Close(true);
By executing this code example, you will get a PDF document like the following image.
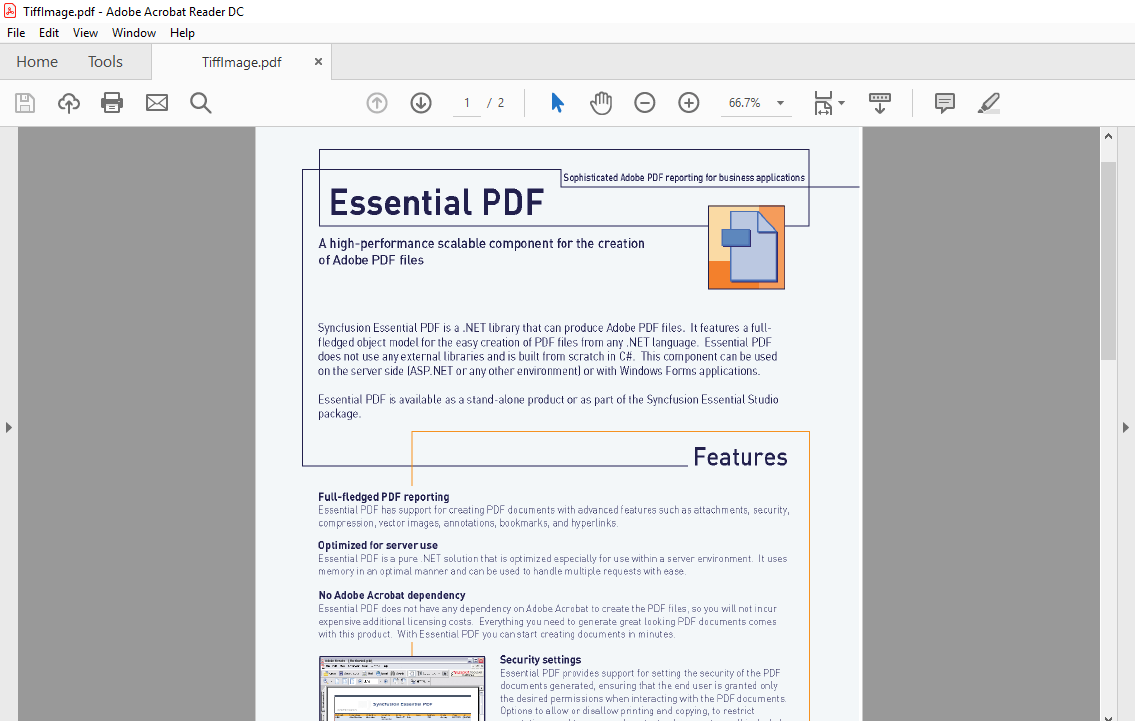
Annotations and forms
Syncfusion’s PDF Library provides comprehensive support for adding and managing annotations and forms in PDF documents.
Annotations
Annotations in a PDF document are additional content, notes, or markup that can be included without altering the original content. They provide feedback, comments, or explanations related to specific sections or elements within the document.
Syncfusion’s PDF Library supports a wide range of annotations: 3D art, file links, rich media, free text, lines, rubber stamps, ink, pop-ups, file attachments, sound, URIs, document links, and redactions.
Refer to the following code example to annotate a PDF document.
//Create a new PDF document. PdfDocument document = new PdfDocument(); //Create a new page. PdfPage page = document.Pages.Add(); FileStream fontStream = new FileStream("Arial.ttf", FileMode.Open, FileAccess.Read); PdfFont pdfFont = new PdfTrueTypeFont(fontStream, 14); //Create a new PDF brush. PdfBrush pdfBrush = new PdfSolidBrush(Color.Black); //Draw text in the new page. page.Graphics.DrawString("Text Markup Annotation Demo", pdfFont, pdfBrush, new PointF(150, 10)); string markupText = "Text Markup"; SizeF size = pdfFont.MeasureString(markupText); RectangleF rectangle = new RectangleF(200, 40, size.Width, size.Height); page.Graphics.DrawString(markupText, pdfFont, pdfBrush, rectangle); //Create a PDF text markup annotation. PdfTextMarkupAnnotation markupAnnotation = new PdfTextMarkupAnnotation("Markup annotation", "Markup annotation with highlight style", markupText, new PointF(200, 40), pdfFont); markupAnnotation.TextMarkupColor = new PdfColor(Color.BlueViolet); markupAnnotation.TextMarkupAnnotationType = PdfTextMarkupAnnotationType.Highlight; //Add this annotation to a new page. page.Annotations.Add(markupAnnotation); //Save the PDF document to memory stream. MemoryStream stream = new MemoryStream(); document.Save(stream); //Close the document. document.Close(true);
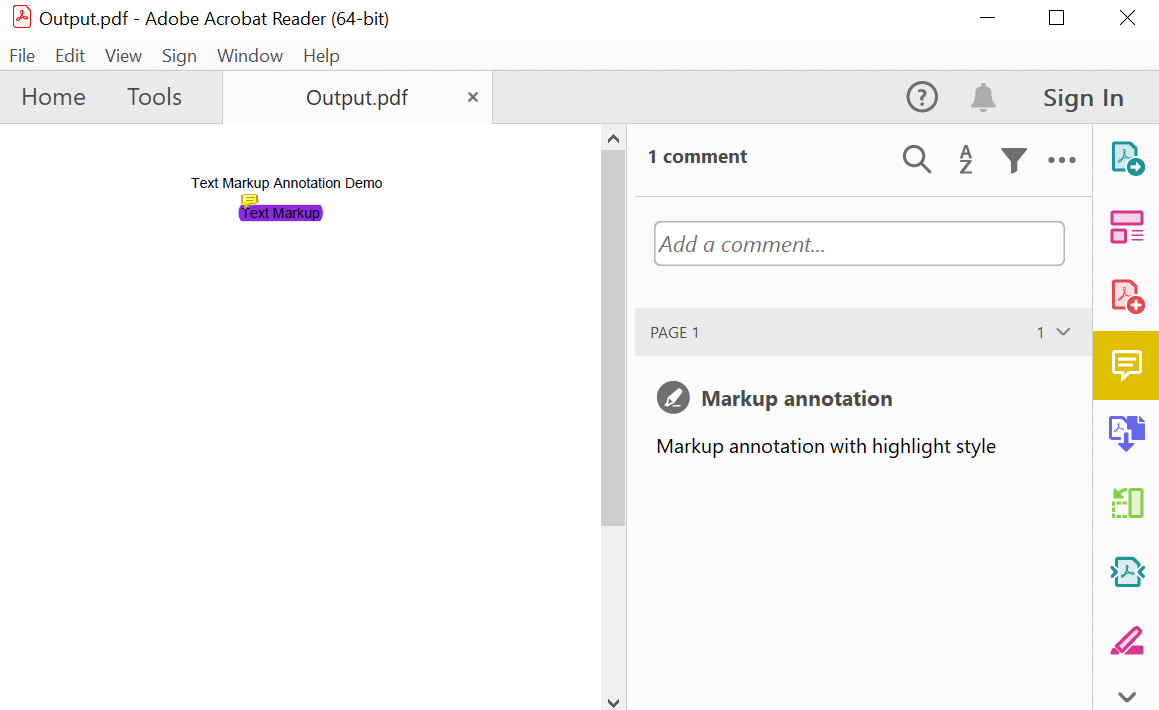
By executing this code example, you will get a PDF document like the following image.
Note: Refer to the working with annotations in PDF documentation for more details.
PDF forms
Interactive PDF forms are widely used for data collection and user input. Syncfusion’s C# PDF Library provides robust support for creating, filling, and processing interactive PDF forms.
Users can easily add form fields such as text boxes, checkboxes, radio buttons, combo boxes, list boxes, signatures, and buttons. Additionally, the library allows you to populate form fields and programmatically extract data submitted by users.
The following code example shows how to create a simple form in a PDF document using C#.
//Create a new PDF document. PdfDocument document = new PdfDocument(); //Add a new page to the PDF document. PdfPage page = document.Pages.Add(); //Set the standard font. PdfFont font = new PdfStandardFont(PdfFontFamily.Helvetica, 16); //Draw the string. page.Graphics.DrawString("Job Application", font, PdfBrushes.Black, new PointF(250, 0)); font = new PdfStandardFont(PdfFontFamily.Helvetica, 12); page.Graphics.DrawString("Name", font, PdfBrushes.Black, new PointF(10, 20)); //Create a text box field for the name. PdfTextBoxField textBoxField1 = new PdfTextBoxField(page, "Name"); textBoxField1.Bounds = new RectangleF(10, 40, 200, 20); textBoxField1.ToolTip = "Name"; document.Form.Fields.Add(textBoxField1); page.Graphics.DrawString("Email address", font, PdfBrushes.Black, new PointF(10, 80)); //Create a text box field for the email address. PdfTextBoxField textBoxField3 = new PdfTextBoxField(page, "Email address"); textBoxField3.Bounds = new RectangleF(10, 100, 200, 20); textBoxField3.ToolTip = "Email address"; document.Form.Fields.Add(textBoxField3); page.Graphics.DrawString("Phone", font, PdfBrushes.Black, new PointF(10, 140)); //Create a text box field for the phone number. PdfTextBoxField textBoxField4 = new PdfTextBoxField(page, "Phone"); textBoxField4.Bounds = new RectangleF(10, 160, 200, 20); textBoxField4.ToolTip = "Phone"; document.Form.Fields.Add(textBoxField4); page.Graphics.DrawString("Gender", font, PdfBrushes.Black, new PointF(10, 200)); //Create a radio button for gender. PdfRadioButtonListField employeesRadioList = new PdfRadioButtonListField(page, "Gender"); document.Form.Fields.Add(employeesRadioList); page.Graphics.DrawString("Male", font, PdfBrushes.Black, new PointF(40, 220)); PdfRadioButtonListItem radioButtonItem1 = new PdfRadioButtonListItem("Male"); radioButtonItem1.Bounds = new RectangleF(10, 220, 20, 20); page.Graphics.DrawString("Female", font, PdfBrushes.Black, new PointF(140, 220)); PdfRadioButtonListItem radioButtonItem2 = new PdfRadioButtonListItem("Female"); radioButtonItem2.Bounds = new RectangleF(110, 220, 20, 20); employeesRadioList.Items.Add(radioButtonItem1); employeesRadioList.Items.Add(radioButtonItem2); //Create file stream. using (FileStream outputFileStream = new FileStream("Output.pdf", FileMode.Create, FileAccess.ReadWrite)) { //Save the PDF document to file stream. document.Save(outputFileStream); } //Close the document. document.Close(true);
By executing this code example, you will get a PDF document like the following image.
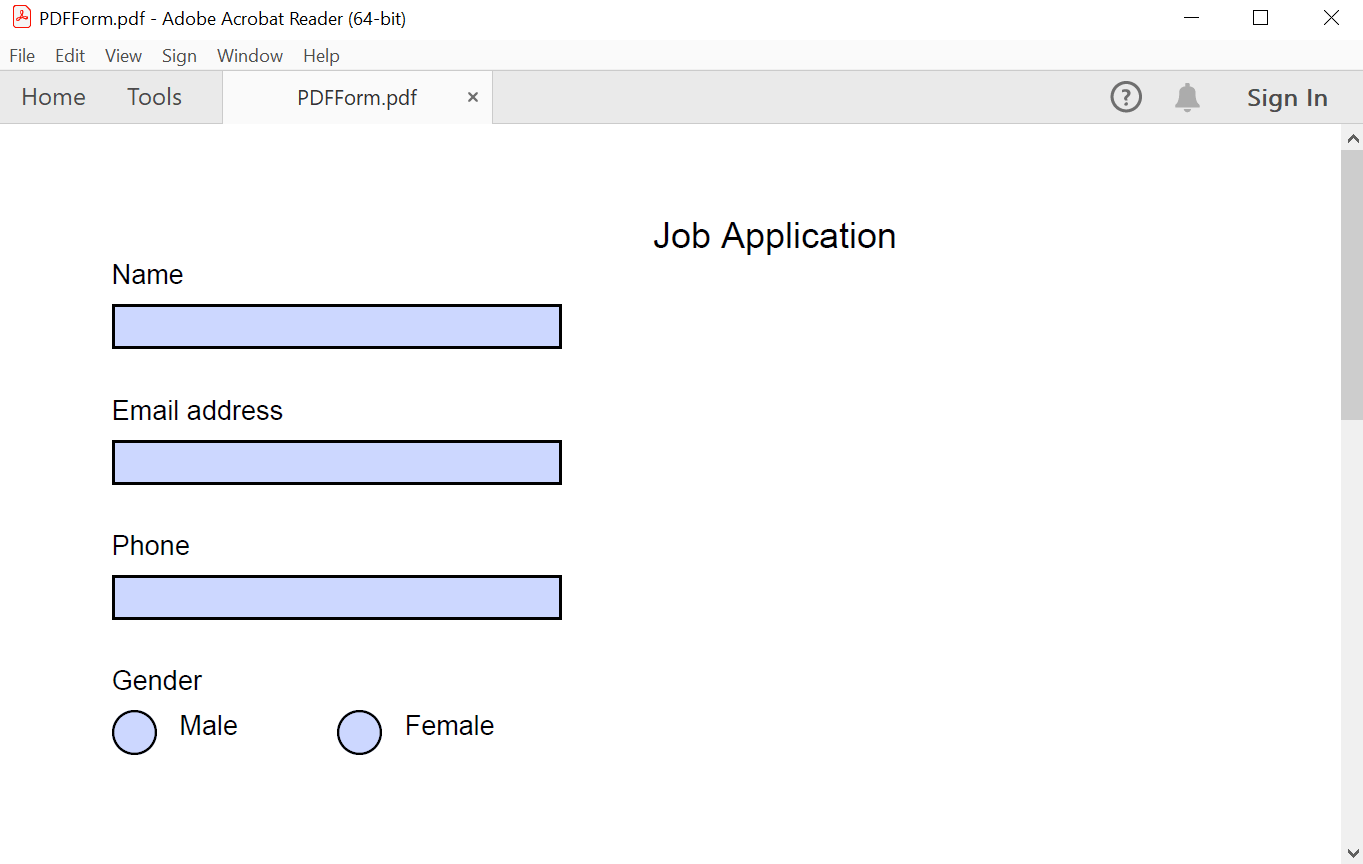
The following code example illustrates how to fill form fields in a PDF document using C#.
//Load the PDF document. PdfLoadedDocument loadedDocument = new PdfLoadedDocument(docStream); //Load the form from the loaded document. PdfLoadedForm form = loadedDocument.Form; //Load the form field collections from the form. PdfLoadedFormFieldCollection fieldCollection = form.Fields as PdfLoadedFormFieldCollection; PdfLoadedField loadedField = null; //Get and fill the field using the TryGetField Methodmethod. if (fieldCollection.TryGetField("Name", out loadedField)) { (loadedField as PdfLoadedTextBoxField).Text = "Simons"; } if (fieldCollection.TryGetField("Email address", out loadedField)) { (loadedField as PdfLoadedTextBoxField).Text = "simonsbistro@outlook.com"; } if (fieldCollection.TryGetField("Phone", out loadedField)) { (loadedField as PdfLoadedTextBoxField).Text = "31 12 34 56"; } if (fieldCollection.TryGetField("Gender", out loadedField)) { (loadedField as PdfLoadedRadioButtonListField).SelectedIndex = 0; } //Create file stream. using (FileStream outputFileStream = new FileStream("Output.pdf", FileMode.Create, FileAccess.ReadWrite)) { //Save the PDF document to file stream. loadedDocument.Save(outputFileStream); } //Close the document. loadedDocument.Close(true);
By executing the previous code example, you will get a filled PDF document with the values set for the form fields.
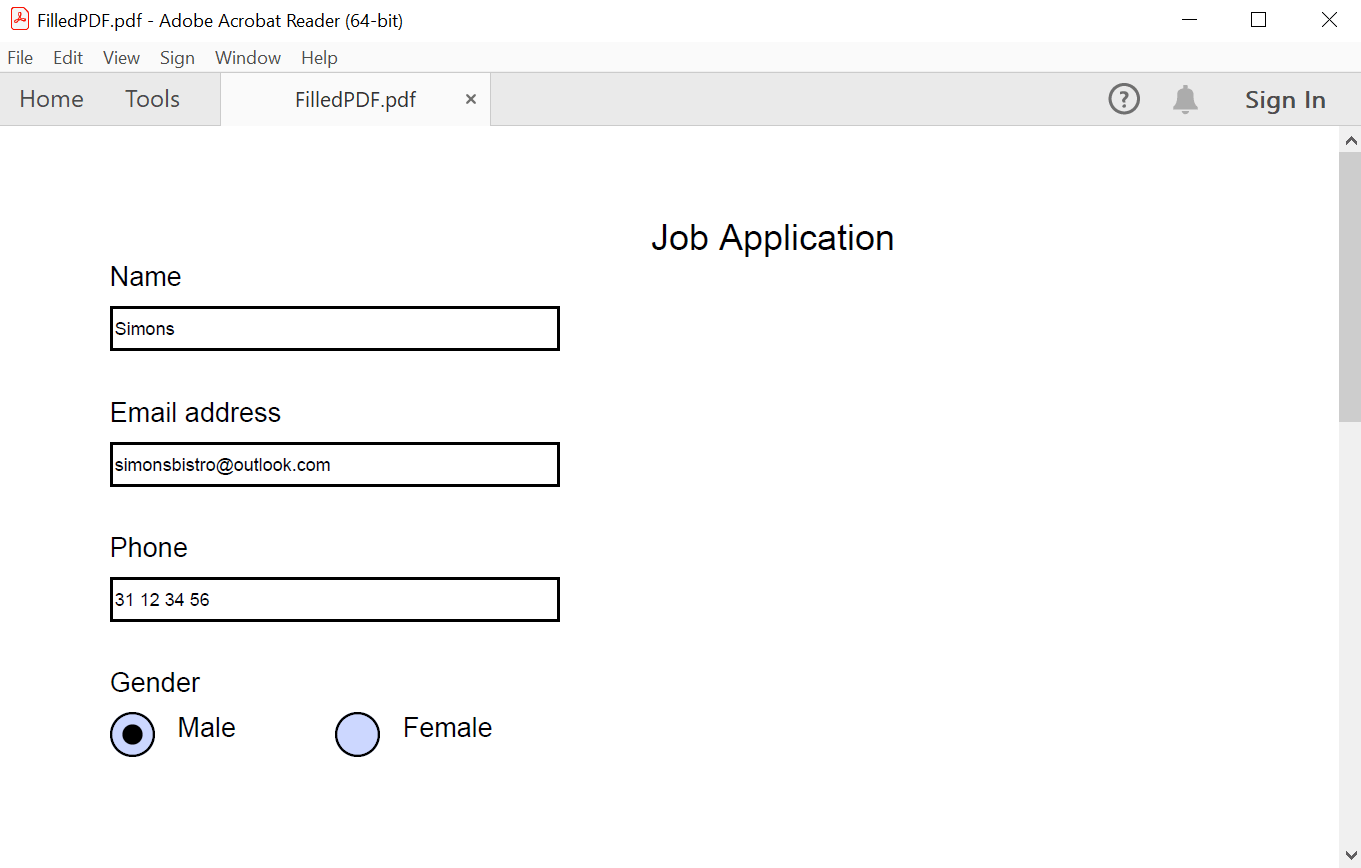
Note: Refer to the working with forms in PDF documentation for more details.

Embark on a virtual tour of Syncfusion's PDF Library through interactive demos.
Security and encryption
Encryption becomes crucial when dealing with PDF documents containing sensitive data to protect them from unauthorized access. Syncfusion offers robust encryption and decryption capabilities to safeguard your PDF documents.
Note: Refer to the working with security in PDF documentation for more details.
PDF encryption
PDF encryption involves applying algorithms to render the content of the PDF document unreadable without the correct decryption key.
Refer to the following code example to encrypt a PDF document.
//Create a new PDF document. PdfDocument document = new PdfDocument(); //Add a page to the document. PdfPage page = document.Pages.Add(); //Create PDF graphics for the page. PdfGraphics graphics = page.Graphics; //Create an instance of document security. PdfSecurity security = document.Security; //Specifies key size, encryption algorithm, and user password. security.KeySize = PdfEncryptionKeySize.Key128Bit; security.Algorithm = PdfEncryptionAlgorithm.RC4; security.UserPassword = "password"; //Draw the text. graphics.DrawString("Encrypt PDF with user password and RC4 128bit keysize", new PdfStandardFont(PdfFontFamily.TimesRoman, 20f, PdfFontStyle.Bold), PdfBrushes.Black, new PointF(0, 40)); //Save the document into stream. MemoryStream stream = new MemoryStream(); document.Save(stream); //Close the document. document.Close(true);
By executing this code example, you will get a PDF document like the following image.
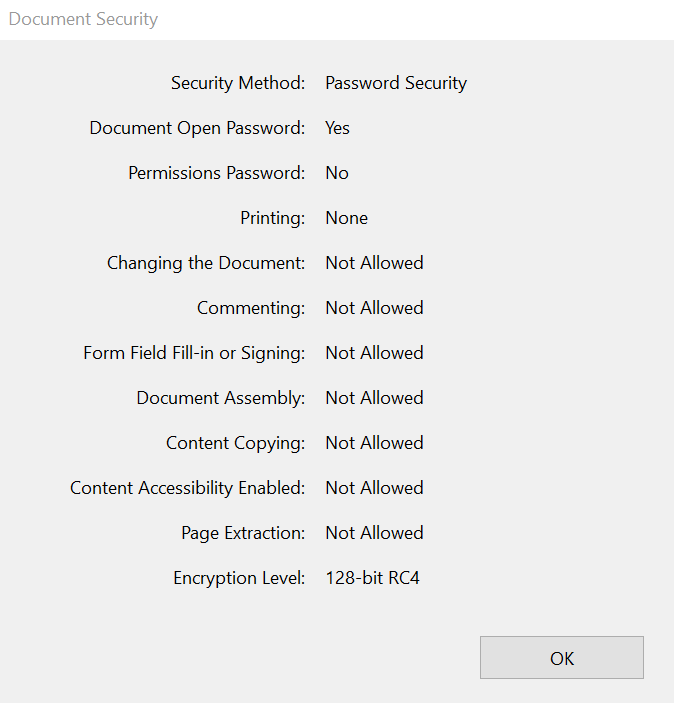
Decrypting PDF documents
Decrypting a PDF document using Syncfusion’s PDF Library is just as straightforward. The decryption process requires the correct password (user or owner) to access the content.
Refer to the following code example to decrypt a PDF document.
//Load the PDF document. FileStream docStream = new FileStream("Input.pdf", FileMode.Open, FileAccess.Read); PdfLoadedDocument loadedDocument = new PdfLoadedDocument(docStream, "password"); //Change the user password. loadedDocument.Security.UserPassword = string.Empty; //Save the document into stream. MemoryStream stream = new MemoryStream(); loadedDocument.Save(stream); //Close the document. loadedDocument.Close(true);
By executing this code example, you can remove the protection from a PDF document. The resulting PDF document will not have password protection and can be freely opened, edited, and printed.
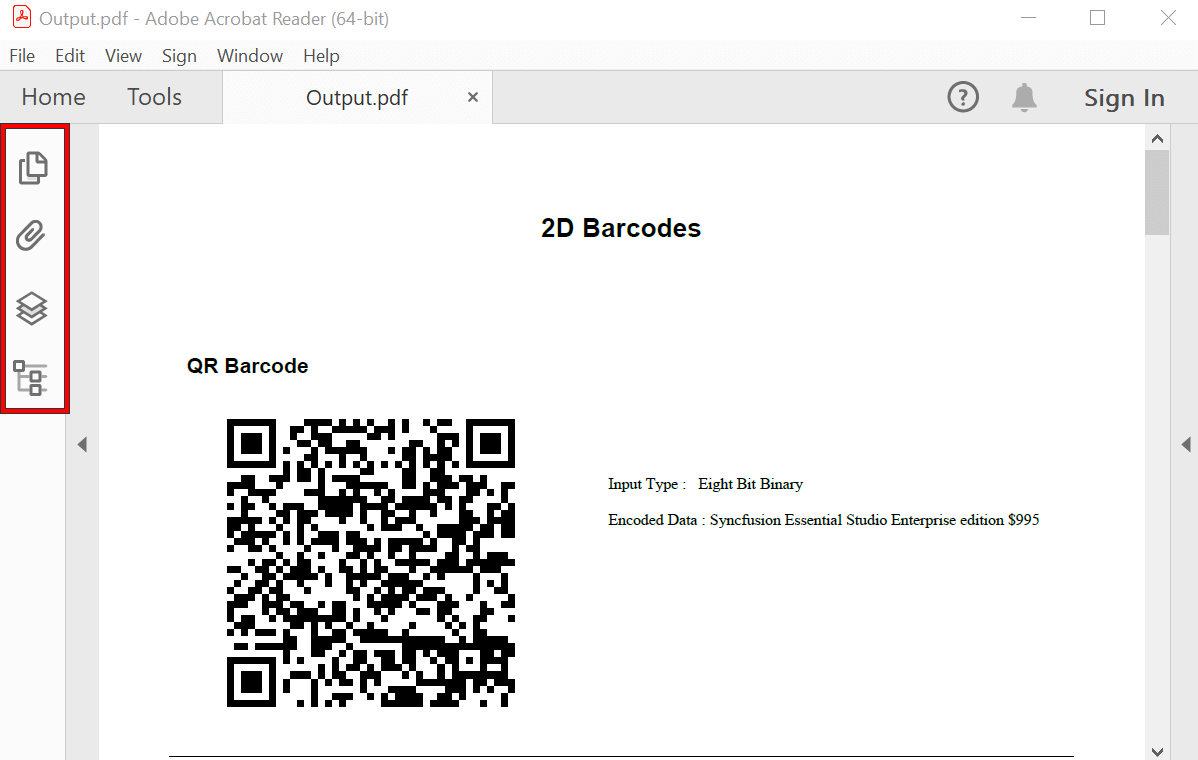
Digital signature
A digital signature verifies the authenticity and integrity of a PDF document, ensuring that the content has not been altered or tampered with since the time of signing. Using Syncfusion’s PDF Library, you can create a digital signature by associating it with a valid digital certificate obtained from a trusted certificate authority.
Here’s the code example of how to sign a PDF document.
//Creates a new PDF document. PdfDocument document = new PdfDocument(); //Adds a new page. PdfPageBase page = document.Pages.Add(); PdfGraphics graphics = page.Graphics; //Creates a certificate instance from a PFX file with private key. FileStream certificateStream = new FileStream(@"PDF.pfx", FileMode.Open, FileAccess.Read); PdfCertificate pdfCert = new PdfCertificate(certificateStream, "syncfusion"); //Creates a digital signature. PdfSignature signature = new PdfSignature(document, page, pdfCert, "Signature"); //Sets an image for the signature field. FileStream imageStream = new FileStream(@"logo.png", FileMode.Open, FileAccess.Read); PdfBitmap signatureImage = new PdfBitmap(imageStream); //Sets signature information. signature.Bounds = new RectangleF(0, 0, 200, 100); signature.ContactInfo = "johndoe@owned.us"; signature.LocationInfo = "Honolulu, Hawaii"; signature.Reason = "I am the author of this document."; //Draws the signature image. signature.Appearance.Normal.Graphics.DrawImage(signatureImage, signature.Bounds); //Creates file stream. using (FileStream outputFileStream = new FileStream("Output.pdf", FileMode.Create, FileAccess.ReadWrite)) { //Saves the PDtqF document to file stream. document.Save(outputFileStream); }
By executing this code example, you will get a PDF document like the following image.
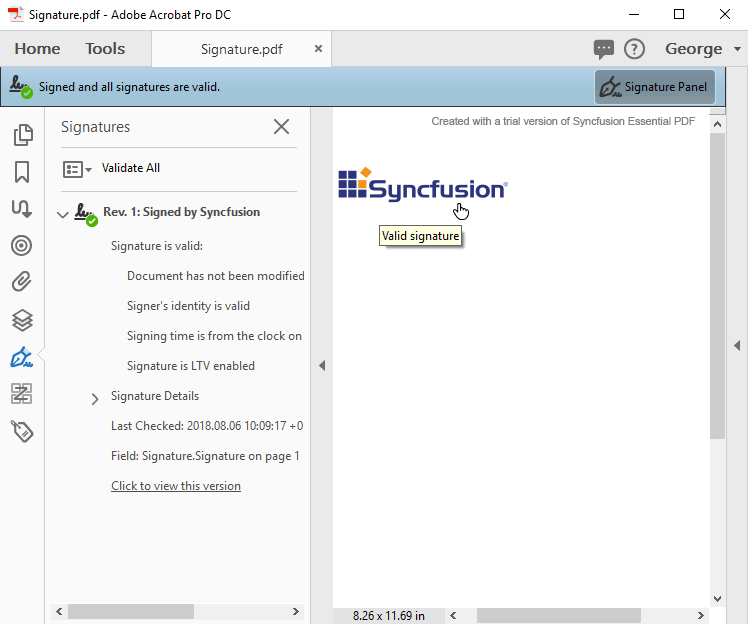
Note: Refer to the working with digital signature in PDF documentation to learn more.

Witness the advanced capabilities of Syncfusion's PDF Library with feature showcases.
Optical character recognition (OCR)
OCR, or optical character recognition, refers to the capability of recognizing text content from scanned images or raster images and convert it into machine-readable text.
The Syncfusion .NET optical character recognition (OCR) library allows users to extract textual information from images, PDF files, or other scanned documents, making the content searchable, editable, and accessible.
The following code example shows how to convert an entire scanned PDF into a searchable PDF document.
//Initialize the OCR processor. using (OCRProcessor processor = new OCRProcessor()) { //Load an existing PDF document. FileStream inputPDFstream = new FileStream("Input.pdf", FileMode.Open); PdfLoadedDocument document = new PdfLoadedDocument(inputPDFstream); //Set OCR language. processor.Settings.Language ="deu"; //Perform OCR with input document. processor.PerformOCR(document, "Tessdata/"); //Create file stream. using (FileStream outputFileStream = new FileStream("Output.pdf", FileMode.Create, FileAccess.ReadWrite)) { //Save the PDF document to file stream. document.Save(outputFileStream); } }
By executing this code example, you will get a PDF document like in the following image.
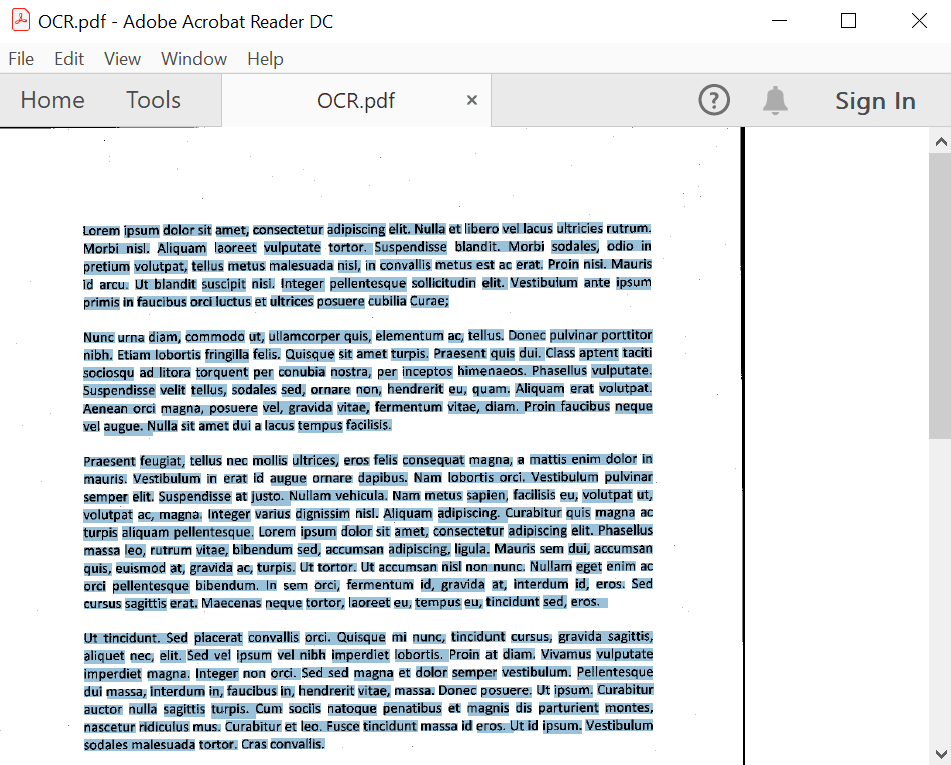
Text-to-PDF and PDF-to-text conversion
Here’s how you can leverage the PDF Library for text-to-PDF and PDF-to-text conversion tasks.
Text-to-PDF conversion
Refer to the following code example to convert text into a PDF document using C#.
//Create a new PDF document. PdfDocument document = new PdfDocument(); //Add a page to the document. PdfPage page = document.Pages.Add(); //Create PDF graphics for the page. PdfGraphics graphics = page.Graphics; //Set the standard font. PdfFont font = new PdfStandardFont(PdfFontFamily.Helvetica, 20); //Draw the text. graphics.DrawString("Hello World!!!", font, PdfBrushes.Black, new Syncfusion.Drawing.PointF(0, 0)); //Create file stream. using (FileStream outputFileStream = new FileStream(Path.GetFullPath(@"Output.pdf"), FileMode.Create, FileAccess.ReadWrite)) { //Save the PDF document to file stream. document.Save(outputFileStream); } //Close the document. document.Close(true);
By executing this code example, you will get the following PDF document.
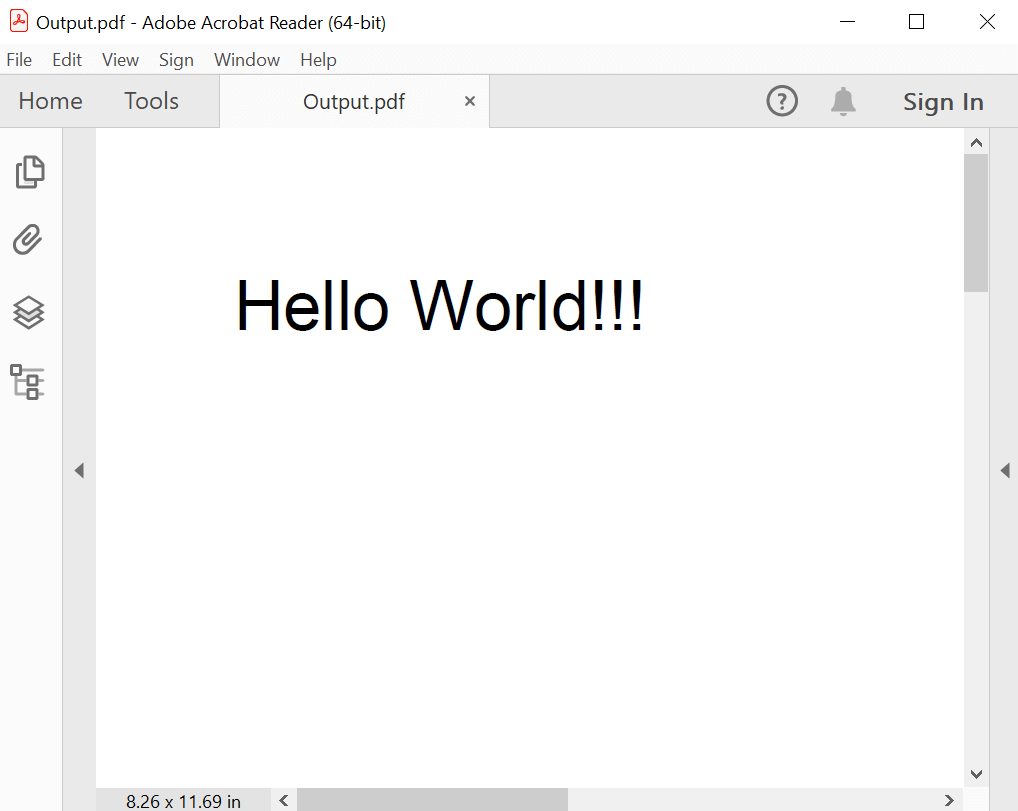
PDF-to-text conversion
You can also extract text from PDF documents. Refer to the following code example.
//Load the PDF document. FileStream docStream = new FileStream("Input.pdf", FileMode.Open, FileAccess.Read); //Load the PDF document. PdfLoadedDocument loadedDocument = new PdfLoadedDocument(docStream); //Load the first page. PdfPageBase page = loadedDocument.Pages[0]; //Extract text from first page. string extractedText = page.ExtractText(); File.WriteAllText("ExtractedText.txt", extractedText); //Close the document. loadedDocument.Close(true);
By executing this code example, you will get the following PDF document.
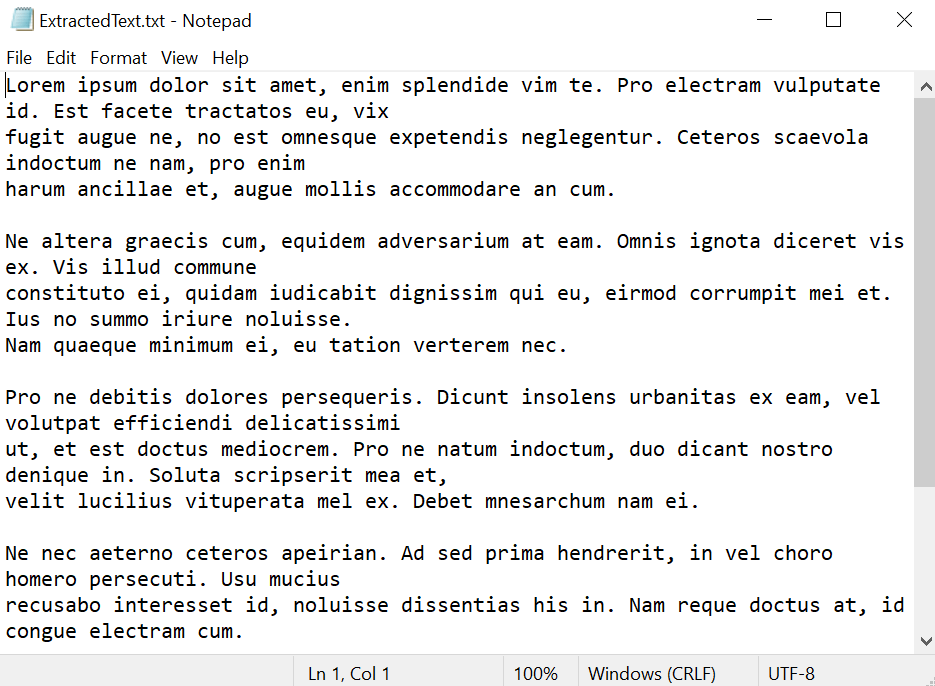
Additional features
These are some of the advanced features the PDF Library offers that can take your PDF documents to the next level:
- PDF/A compliance: PDF/A is an ISO-standardized PDF version designed for long-term electronic document preservation. The PDF Library makes it easy to create PDF/A-compliant documents, ensuring that your files will remain accessible and reliable for years.
- Watermarks: Watermarks are an excellent way to personalize or protect your documents from unauthorized use. PDF Library allows you to add text or image watermarks to your PDF documents.
- Bookmarks: Bookmarks provide an organized and efficient way for users to navigate lengthy PDF documents and quickly access specific sections or pages. PDF Library allows users to create a hierarchical list of entries in a separate pane or panel within the PDF document.
- Accessible PDFs: Adding tags to the elements of a PDF document makes it accessible. When a tagged PDF document has accessibility tags, it is useful for screen readers and other assistive technologies to read and navigate it. With PDF Library, you can easily create a tagged PDF document. Refer to the working with tagged PDFs documentation to learn more.
- Redaction: Redacting a PDF removes sensitive or confidential information from PDF documents. PDF Library provides an easy way to redact PDF documents. Refer to the working with PDF redaction documentation for more info.
- Compression: Large PDF files can be challenging to share and store. Syncfusion’s PDF Library includes compression options to reduce file size without compromising the quality. Refer to the working with compression in PDF documentation to learn more.
- Tables: With just a few lines of code, you can generate tables with the desired number of rows and columns, define cell properties, and populate data. This streamlined process ensures that you can quickly produce professional-looking tables in your PDF documents. Refer to the working with PDF tables documentation.
- Table of contents: A well-structured table of contents is essential for lengthy documents. PDF Library automates TOC generation based on document structure.
GitHub reference
Refer to the demo for the Syncfusion C# PDF Library on GitHub.

Join thousands of developers who rely on Syncfusion for their PDF needs. Experience the difference today!
Conclusion
We appreciate your time in reading this blog! We’ve explored the top 10 essential features that make the Syncfusion .NET PDF Library a must-have tool for users and developers. Give the steps in this blog post a try, and share your thoughts in the comments section below.
Take a moment to look at the PDF forms documentation, where you can find other options and features, all with accompanying code examples.
For questions, you can contact us through our support forum, support portal, or feedback portal. We are always happy to assist you!