TL;DR: Want to write cleaner, more maintainable JavaScript and TypeScript code? Explore 5 powerful linters in this guide! Discover features that streamline your development process, like automatic fixing and effortless setup.
Linters are essential tools for JavaScript and TypeScript developers. These tools help catch potential bugs, enforce code style conventions, and improve overall code quality, streamlining the development process and boosting efficiency.
This article explores five popular linters for JavaScript and TypeScript, highlighting their key features and installation steps.
1. ESLint
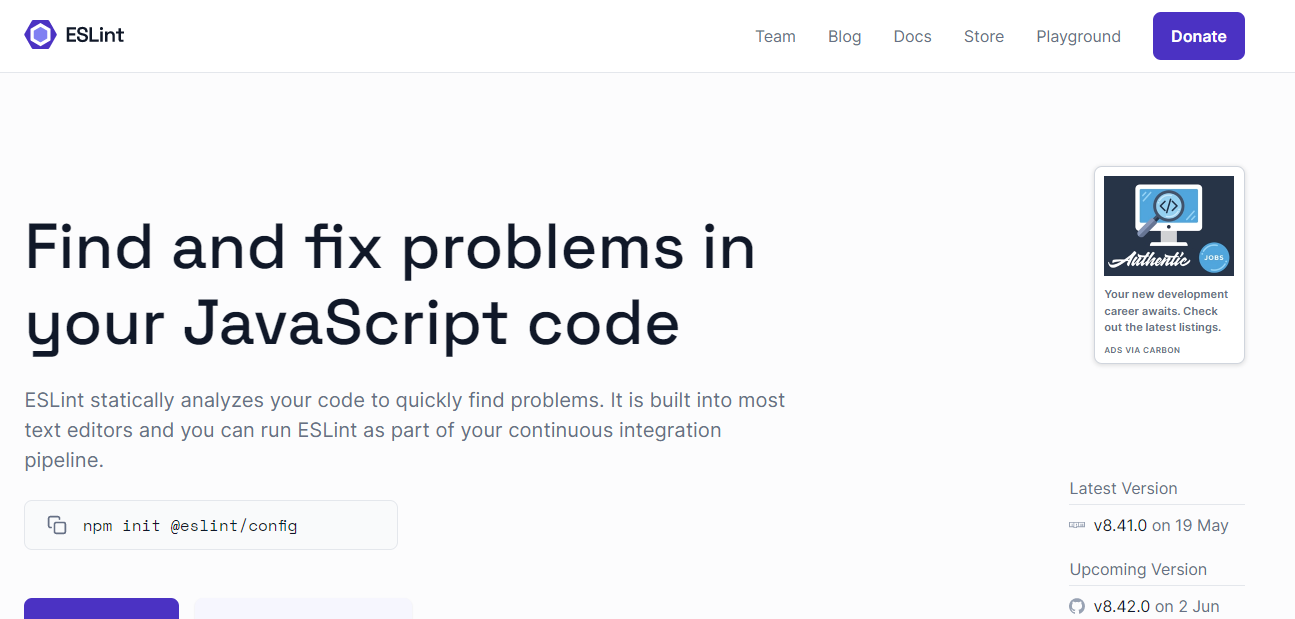
ESLint is a widely recognized and powerful linter catering to JavaScript and TypeScript developers. Offering extensive customization options, ESLint enables users to tailor rules, incorporate plugins, and seamlessly integrate them into their workflow across various editors and build systems. ESLint facilitates the creation of clean and error-free code.
When encountering invalid JavaScript code, ESLint promptly issues warning messages to pinpoint specific errors. For instance, consider the following code example illustrating a syntax error in JavaScript.
console.log("Hello, World!")
Error: Missing semicolon at the end of the statement.
Installation
To install ESLint, developers can utilize either npm or yarn package managers.
For npm installation, navigate to the project’s root directory via the command-line interface (CLI) or terminal and execute the following command.
npm install eslint --save-dev
This command installs ESLint as a development dependency, saving it in the project’s package.json file under the devDependencies section. Upon completion of the installation process, ESLint is available for configuration.
Users can create a .eslintrc file or use the eslint –init command to generate a configuration file.
Alternatively, for yarn installation, execute the following command.
yarn add eslint –dev
Yarn installs ESLint and adds it to your project’s package.json file under the devDependencies section.
Features
- Customizable rules: Allows you to define and customize rules based on your project’s requirements.
- Extensibility: Supports plugins, expanding its functionality to cover specific coding styles, frameworks, or libraries.
- Editor integration: Seamlessly integrates with popular code editors, providing real-time feedback and suggestions within the editor.
- Automatic fixing: Automatically resolves many common code issues, saving time by handling minor code style problems without manual intervention.
- TypeScript support: Now includes built-in support for TypeScript, allowing you to lint TypeScript code alongside JavaScript.
2. JSHint
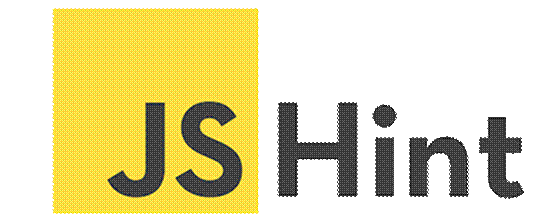
JSHint is a well-established JavaScript linter that enhances code quality by identifying errors and enforcing coding standards. Widely used within the JavaScript ecosystem, JSHint aids developers in maintaining clean, error-free code. It adopts a more opinionated approach, relying on default coding standards to highlight common errors, making it an ideal choice for simpler projects or those seeking a straightforward setup.
In addition to detecting syntax errors, JSHint ensures adherence to specific coding styles. For instance, consider the following code example that would trigger a JSHint error.
function calculateArea(radius) { var area; if(radius <= 0) { console.log("Invalid radius"); } else { area = Math.PI * r * r; } return area; }
In the previous code, a reference to r occurs inside the calculation of the area, yet the variable r is not defined within the function. The correct variable should be the radius. JSHint would identify this mistake and provide the following error message.
Note: Error: 'r' is not defined.
Installation
To install JSHint, you can utilize npm or yarn, which is similar to ESLint.
Here’s how you can install it using npm.
npm install jshint --save-dev
This command installs JSHint as a development dependency and saves it in your project’s package.json file under the devDependencies section.
If you prefer using yarn, you can use the following command instead.
yarn add jshint –dev
Yarn will install JSHint and add it to your project’s package.json file under the devDependencies section.
Features
- Default coding standards: Enforces a set of default coding standards, which are relatively strict, to help catch common coding errors.
- Configuration: Configurable to some extent, allowing you to adjust certain rules and options to fit your project’s needs.
- Simplicity: Easy to set up and use, making it a good choice for projects that need a simple, straightforward linter.
- No TypeScript support: Primarily for JavaScript and does not provide native TypeScript support. If you are working with TypeScript, ESLint is a more suitable choice.
- Limited extensibility: While allowing some configuration, it’s not as extensible as ESLint.
3. Prettier
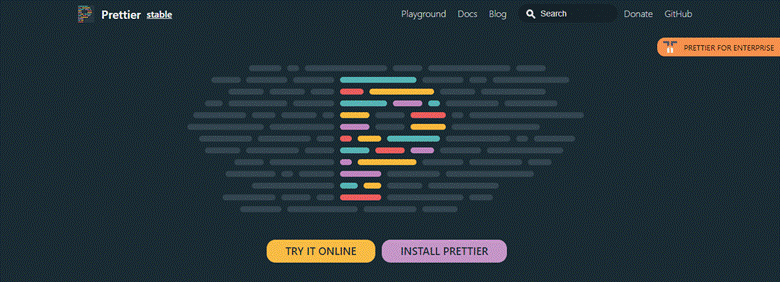
Prettier is a widely used code formatting tool known for maintaining consistent code style across projects. It automates code formatting by analyzing code and applying a standardized set of rules. Prettier supports multiple programming languages, including JavaScript, TypeScript, HTML, and CSS, making it highly versatile.
Prettier focuses solely on code formatting, avoiding stylistic debates. Prettier automatically formats code and highlights any deviations from the configured rules.
Refer to the following code example of a Prettier error.
function greet(name){ console.log("Hello, " + name + "!") }
If this code snippet contains only stylistic issues (such as missing spaces or inconsistent indentation), Prettier automatically formats it without any error messages. However, it’s different if there’s a syntax error, like a missing parenthesis.
function greet(name){ console.log("Hello, " + name + "!"; }
In such a scenario, Prettier will be unable to format the code and produce the following error message.
Note: SyntaxError: Unexpected token (2:40)
Installation
Before proceeding with the installation, ensure your system has Node.js and npm installed. Once confirmed, execute the following command in your terminal or command prompt to install Prettier globally.
npm install -g prettier
This enables execution from any project directory. Upon completion, navigate to your project directory in the terminal. To format a file using Prettier, execute the following command, replacing path/to/file with the actual file path.
prettier --write path/to/file
This command modifies the file, applying standardized code formatting rules.
Features
- Automatic code formatting: Automatically formats code based on a predefined set of rules, ensuring consistent and uniform code style across projects.
- Language support: Supports many programming languages, including JavaScript, TypeScript, HTML, CSS, and JSON, making it versatile for different development environments.
- Opinionated formatting: Follows a strict formatting style, removing the need for decisions or debates about coding style. It focuses on providing a consistent and readable output.
- Customizable configuration: While it has default formatting rules, you can customize its behavior by creating a configuration file in your project and changing specific options.
- Editor integrations: Integrates with popular code editors and IDEs, allowing you to format code directly within the editor.
4.StandardJS
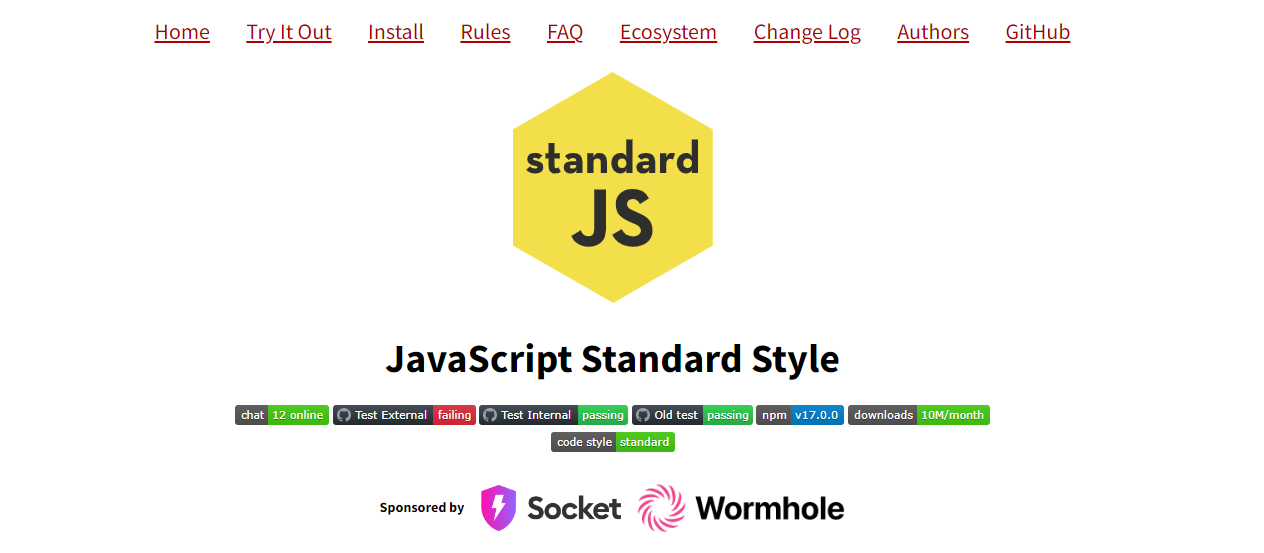
StandardJS is an opinionated JavaScript style guide and linter that aims to establish a consistent coding style across projects. It enforces a set of rules to promote the readability and maintainability of code. StandardJS embraces simplicity and avoids configuration options to minimize debates on coding style.
StandardJS follows the JS standard style guide. Refer to the following code example of a StandardJS error.
var age = 21; if (age >= 18) { console.log("You are eligible to vote."); }
Error: Expected let or const instead of var.
Installation
To use StandardJS, ensure you have Node.js and npm installed on your system. After that, open a terminal or command prompt and execute the following command to install StandardJS, enabling access from any project directory globally.
npm install -g standard
Upon completion of the installation process, navigate to your JavaScript project directory in the terminal and execute the following command to lint your JavaScript files using StandardJS.
standard
This command will analyze your code based on the StandardJS rules and identify any style violations or errors encountered.
Features
- Opinionated: Follows a strict set of predefined rules, reducing the need for configuration and style debates.
- Consistent coding style: Enforces a consistent coding style across projects, promoting readability and maintainability of code.
- Automatic formatting: StandardJS can automatically fix many style issues, making it easier to adhere to the defined coding style.
- Minimal configuration: Designed to be easy to set up and use with minimal configuration requirements.
- Widely adopted: Has widespread acceptance within the JavaScript community.
5. SonarTS
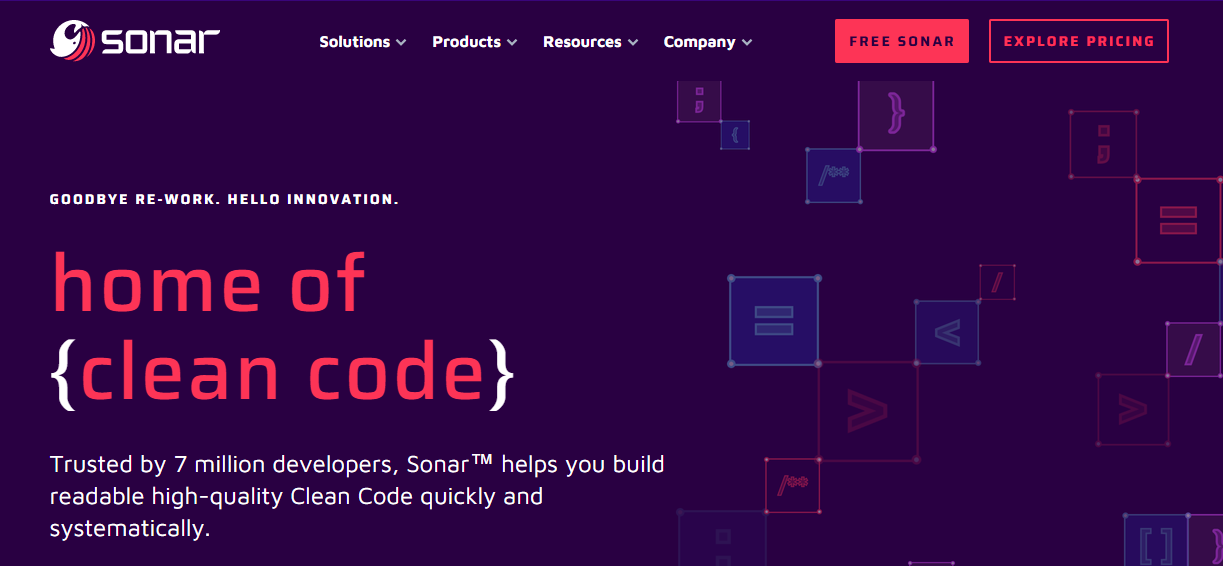
SonarTS is a static code analysis tool specifically designed for TypeScript projects. It helps identify bugs, code smells, security vulnerabilities, and other code quality issues. SonarTS provides a comprehensive set of rules and best practices for TypeScript development, enabling developers to write cleaner and more maintainable code. With its integration into popular development environments and support for continuous integration pipelines, SonarTS assists in improving code quality and ensuring adherence to coding standards in TypeScript projects.
SonarTS can be used to find bugs and vulnerabilities in TypeScript code. Refer to the following code example of a SonarTS error.
function addNumbers(a: number, b: number) { if (a = 5) { return a + b; } } const result = addNumbers(3, 2);
In this example, the issue lies in the conditional statement of the addNumbers function.
Note: Bug: Did you mean to use '==' or '===' instead of '='?
Installation
To install SonarTS and integrate it into your TypeScript project, ensure you have Node.js and npm installed on your system. Open a terminal or command prompt and run the following command to install SonarTS globally, allowing you to use it from any project directory.
npm install -g sonarts
Navigate to your TypeScript project directory in the terminal.
Next, create a SonarQube configuration file named sonar-project.properties in your project’s root directory.
Open the sonar-project.properties file and configure the required properties, such as project key, project name, and project version.
Finally, run the following command to analyze your TypeScript code using SonarTS.
sonarts
This command will analyze your TypeScript files based on the configured SonarQube properties and provide a detailed report of code quality issues, bugs, and vulnerabilities.
Features
- Static code analysis: Performs comprehensive static code analysis for TypeScript projects, identifying bugs, code smells, security vulnerabilities, and other code quality issues.
- TypeScript-specific rules: Provides a wide range of TypeScript-specific rules and best practices to ensure high-quality TypeScript code.
- Detailed code quality reports: Generates detailed reports highlighting code quality issues, allowing developers to identify and address potential problems easily.
- Integration with development environments: Integrates with popular development environments like Visual Studio Code, enabling real-time feedback and assistance during development.
- Continuous integration support: Integrates into continuous integration (CI) pipelines, allowing for automated code analysis and quality checks in the software development process.
Example: Code Quality Analysis
Consider the following code snippet, which has several issues.
// JavaScript code snippet with multiple issues function addNumbers(a, b) { if (a = 5) { console.log("Result is " + a + b); } return a + b; }
If you run this code through all five linters discussed, they will capture different errors:
- ESLint: Unexpected constant condition. (no-constant-condition).
- JSHint: Expected a conditional expression and instead saw an assignment, missing semicolons.
- Prettier: Code formatting issues (inconsistent spacing and indentation).
- StandardJS: Use var instead of let or const, and missing semicolons.
- SonarTS: TypeScript-specific issues are not applicable here as it’s not TypeScript code, but if this were TypeScript, potential type assertion issues or any misuse of TypeScript features could be flagged.
This example demonstrates how each tool improves different aspects of the code, from potential bugs and code quality to formatting and style consistency.
Conclusion
Thank you for reading! In this article, we’ve explored the top 5 linters along with their installation processes and features. The choice of linter depends on your project requirements, team preferences, and coding style guidelines, as each tool addresses different aspects of your code.
The Syncfusion JavaScript suite is a comprehensive solution for app development, offering high-performance, lightweight, modular, and responsive UI components. We encourage you to download the free trial and assess these controls.
If you have any questions or feedback, you can contact us through our support forums, support portal, or feedback portal. We’re always here to help you!