TLDR: Discover the seven must-known JavaScript unit testing frameworks. Each offers unique advantages, catering to diverse project needs. Choose wisely to ensure robust, bug-free applications.
JavaScript powers many modern websites’ dynamic and interactive elements. As the complexity of JavaScript apps increases, so does the need for robust testing frameworks to ensure their reliability and performance. This article will introduce you to 7 JavaScript unit test frameworks that every developer should know.
These tools are the secret weapons of many successful developers, providing the confidence to build scalable, maintainable, and bug-free apps. This guide will equip you with the knowledge to choose the proper testing framework for your needs.
Let’s dive in!
1. Jest: The queen of popularity
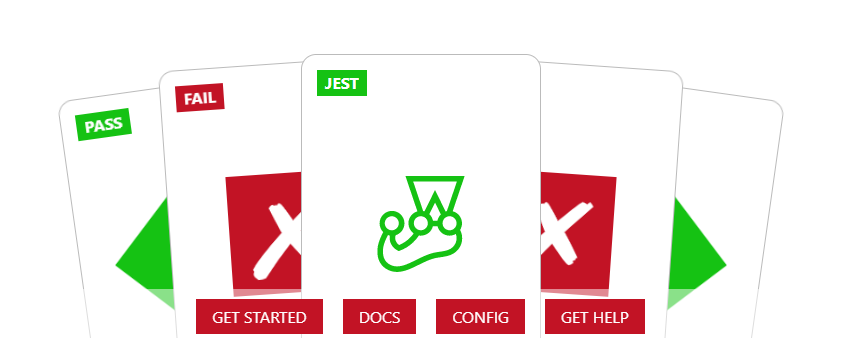
Imagine a fast framework that makes your tests zoom by like greased lightning. That’s Jest, the undisputed champion of speed and simplicity. Its intuitive API and zero-configuration setup make it ideal for beginners and veterans. It seamlessly integrates with popular frameworks like React and Node.js, and its auto-mocking feature saves you tons of boilerplate code.
Jest has over 40.9K GitHub stars and over 19,187K weekly NPM downloads.
Advantages of Jest
- High performance: Jest can run tests in parallel to speed up your testing process.
- Compatibility: Although developers primarily use Jest to test React apps, you can use it with other frameworks with simple integration. For example, it works with other Babel-based projects, such as Angular, Vue.js, and Node.js.
- Auto-mocking: Jest automatically mocks your libraries when you import them into your test files, reducing boilerplate.
- Extended API: Jest has a broad API compared to the other libraries. So you don’t need to add any extra libraries.
- Coverage reports: You can generate code coverage reports with Jest by adding the –coverage a flag.
Disadvantages of Jest
- Auto-mocking leads to a slow runtime: Although auto-mocking is considered an advantage, it may work against you since it automatically wraps all your libraries and slows down your tests.
- Limited mocking flexibility: Advanced mocking scenarios might require additional libraries.
Installation
You can easily install Jest using NPM or Yarn as follows.
// NPM npm install --save-dev jest // Yarn yarn add --dev jest
Usage
The following code shows a simple Jest unit test to verify the functionality of a multiply function.
test('multiplies 2 * 2 to equal 4', () => { expect(multiply(2, 2)).toBe(4); });
You can find a working example of this in Stackblitz. Ensure you run the npm install and npm test commands to execute the code.
2. Mocha: The lean, mean testing machine
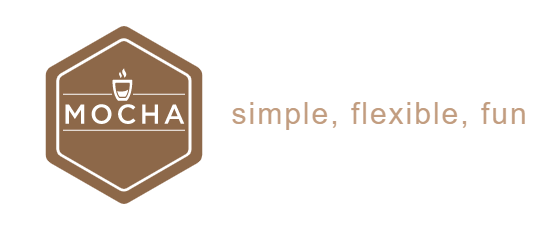
If you value stability and flexibility, Mocha is your trusty companion. This mature framework boasts a clear and concise API, making it a favorite among experienced developers. Its asynchronous testing capabilities easily handle even the trickiest code, and its modular design allows you to customize it to your heart’s content.
Mocha has over 21.8K GitHub stars and over 7 million weekly NPM downloads.
Advantages of Mocha
- Light and simple: Mocha is an easy solution for smaller projects that don’t require complex assertions or testing logic.
- Clear and straightforward API: The Mocha API is more user-friendly than other frameworks like Jasmine.
- ES module assistance: Mocha allows you to write your tests as ES modules in addition to CommonJS. (Using import and using require.)
Disadvantages of Mocha
- More work to set up: Assertions require additional libraries, making the setup more complex than others.
- More configurations: More configurations are required since it is not a fully independent framework.
- Difficulties in auto-mocking: Auto-mocking and snapshot testing are not easy.
Installation
You can easily install Mocha using NPM.
// NPM npm install --save-dev mocha
Usage
The following code shows a simple Mocha unit test to verify the functionality of adding two numbers.
const expect = require('chai').expect; describe('add', function () { it('should add two numbers', () => { expect(add(2, 3)).to. Equal(5); }); });
You can find a working example of this in Stackblitz. Ensure you run the npm install and npm test commands to execute the code.
3. Jasmine: The BDD bard
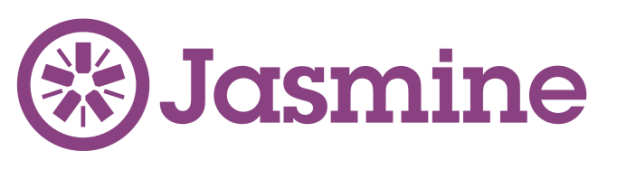
Jasmine is a popular JavaScript behavior-driven development (BDD) framework for unit-testing JavaScript apps. It offers tools for running automated synchronous and asynchronous code tests. Apart from JavaScript, Jasmine supports Python, Ruby, TypeScript, and CoffeeScript.
Jasmine has 15.5K+ GitHub stars and more than 1.5 million weekly NPM downloads.
Advantages of Jasmine
- Simple API: It offers a clear and simple API for writing unit tests with clean and understandable syntax.
- Batteries are also included: Jasmine includes built-in assertion methods and matches like toEqual, toBe, toBeTruthy, and toBeFalsy.
- Good documentation: Jasmine has strong community support and good documentation.
- No DOM required: Jasmine does not require any DOM support since it does not depend on any framework.
Disadvantages of Jasmine
- Configurations: You must select an assertion or mocking library before using Jasmine. Furthermore, integrating with third-party libraries like jasmine-snapshot requires additional configurations.
- Async testing can be tricky: Although it supports asynchronous testing, implementation can be challenging.
- Limited to smaller projects: Might not scale well for complex applications.
Installation
You can easily install Jasmine using NPM or Yarn as follows:
// NPM npm i jasmine // Yarn yarn add jasmine
Usage
The following code shows a simple Jasmine unit test to verify the functionality of adding two numbers.
describe('Arithmatic', function () { it('should add two numbers', function () { expect(sum(2, 2)).toBe(4); }); });
You can find a working example of the previous example in Stackblitz. Ensure to run the npm install and npm run test commands to execute the code.
4. AVA: The concurrency queen
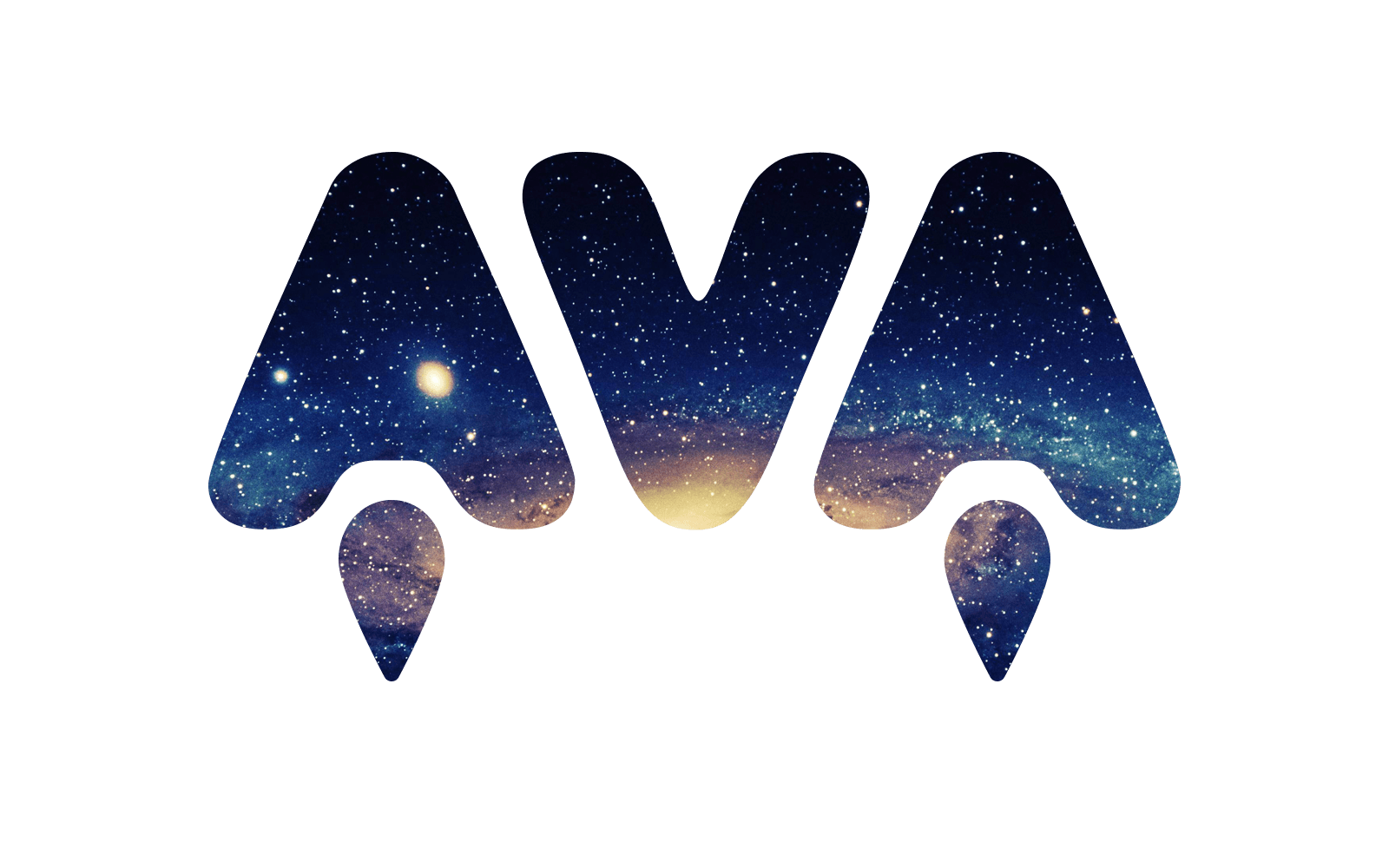
If you appreciate elegance and efficiency, AVA is your minimalist muse. This streamlined framework utilizes JavaScript’s async capabilities to run tests concurrently, boosting your development speed. Its straightforward API and built-in features, like snapshot testing and TAP reporting, make it a compelling choice for fans of clean code and modern practices.
AVA has more than 20.2K GitHub stars and 272K+ weekly NPM downloads.
Advantages of AVA
- Concurrently runs tests: It takes advantage of JavaScript’s async nature, reducing the gap between deployments.
- Straightforward API: It contains a basic API that only offers the needed features.
- Snapshot testing: Support jest-snapshot to run snapshot tests.
- Tap reporter: By default, AVA displays a report humans can read. However, you can also generate the report in TAP format.
Disadvantages of AVA
- No test grouping: AVA does not allow for grouping-related tests.
- No built-in mocking: Mocking is not included with AVA, but you can use third-party libraries.
- The learning curve for advanced features: May require a deeper understanding of async concepts.
Installation
You can easily install AVA using NPM or Yarn.
// NPM npm i ava // Yarn yarn add ava
Usage
The following code shows a simple AVA unit test to verify the functionality of multiplying two numbers.
var test = require('ava'); test('multipication of 2 numbers', (t) => { t.plan(2); t.pass('this assertion passed'); t.is(multiply(2, 2), 4); });
You can find a working example of the previous example in Stackblitz. Ensure you run the npm install and npm run test commands to execute the code.
5. QUnit: The DOM dominator
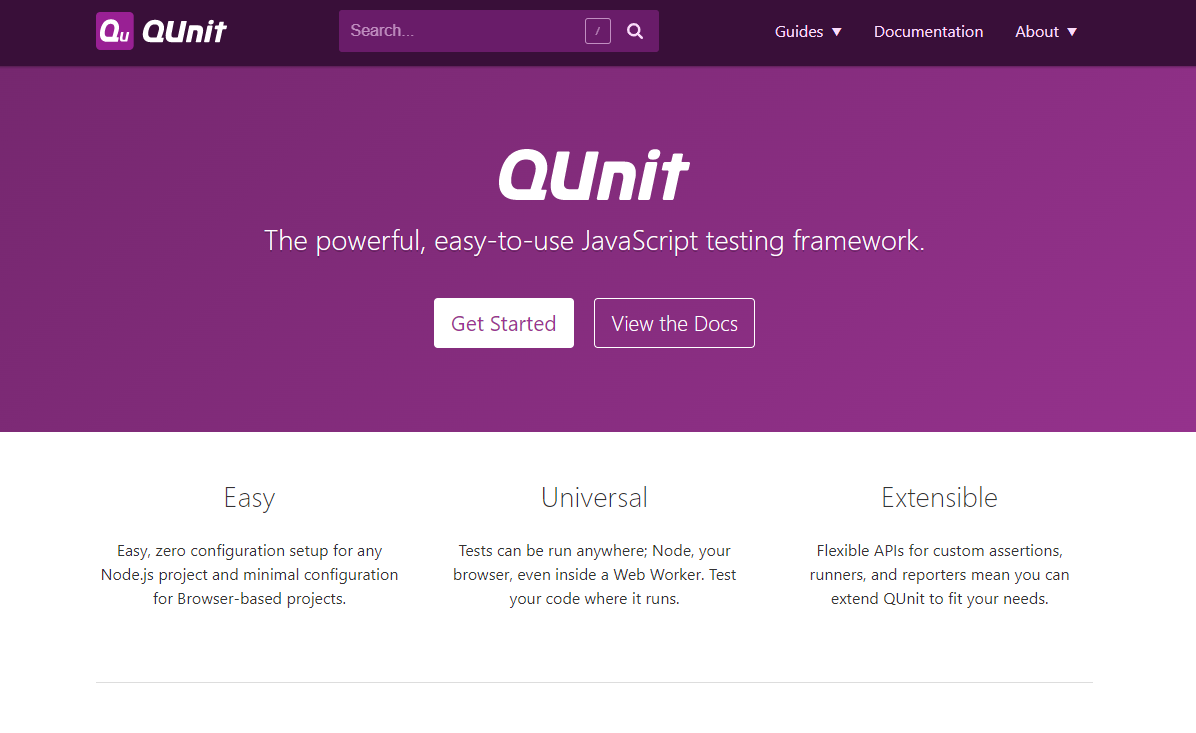
For those building web apps with intricate DOM interactions, QUnit is your knight in shining armor. Initially designed for jQuery testing, it manipulates and evaluates browser elements. Its easy setup and familiar syntax make it perfect for beginners who want to test the front end.
QUnit has more than 4K GitHub stars and 193K+ weekly NPM downloads.
Advantages of QUnit
- Easy to use: All you have to do is create a new test case, including the QUnit library from the CDN, and run it. Your outputs will be presented in your browser.
- It works well when DOM testing: QUnit makes testing document object model (DOM) elements effortless since it was built as a part of jQuery.
Disadvantages of QUnit
- Challenges in testing async operations: When using QUnit, you must call start() before the async function and stop() after completion. When you have no way of knowing when your function will begin or end, this can be a problem.
Installation
You can easily install QUnit using NPM or Yarn.
// NPM npm i qunit // Yarn yarn add qunit
Usage
The following code shows a simple QUnit unit test to verify the functionality of multiplying two numbers.
QUnit.module('multiply'); QUnit.test('multiply two numbers', (assert) => { assert.equal(multiply(2, 2), 4); });
You can also find a working example of the previous example in Stackblitz.
6. Playwright: The multi-browser maestro
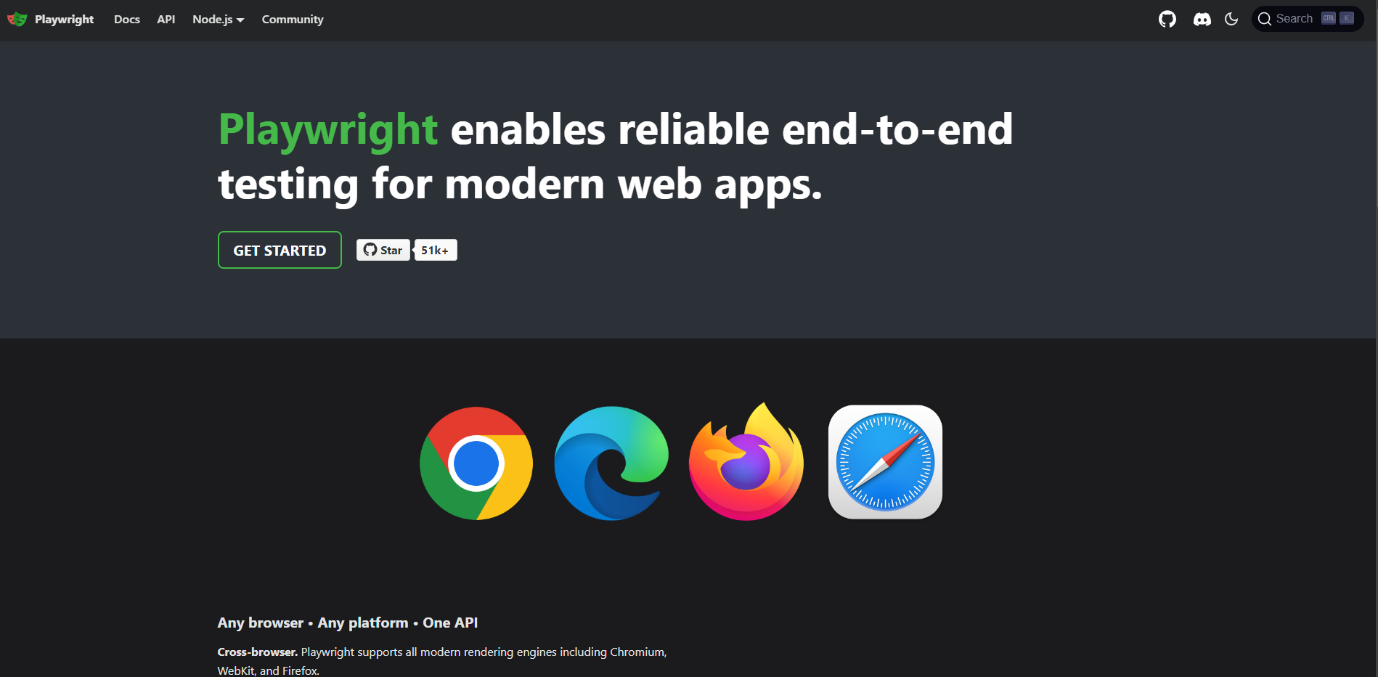
Playwright, developed by Microsoft, lets you test your code across all major browsers with ease. Its powerful API lets you control browsers like puppet strings, precisely automating interactions and verifying results. You can ensure flawless performance across platforms with built-in support for multiple browsers, including Chrome, Firefox, and Safari.
Advantages of Playwright
- Multi-browser support: Playwright offers excellent cross-browser testing capabilities, allowing you to run tests on different browsers efficiently.
- Fast and reliable: Playwright is known for its speed and reliability in executing tests, making it suitable for large-scale projects.
- Headless and browserless modes: Choose the right testing environment for your needs.
- Robust API: Fine-grained control over browser actions and interactions.
Disadvantages of Playwright
- Steep learning curve: The Playwright’s extensive feature set can be overwhelming for beginners.
- Limited community support: Has a smaller community than established frameworks like Jest or Mocha.
- Focus on web automation: Primarily for end-to-end testing, not solely unit testing.
Installation
You can easily install Playwright using NPM.
// NPM npm install --save-dev playwright
Usage
The following code shows a simple Playwright unit test to verify the functionality of adding two numbers.
import { expect, test } from '@playwright/test'; test.describe('describe title', () => { test('test title', ({}) => { expect(1 + 1).toEqual(2); }); });
7. Cypress: The real-time rockstar
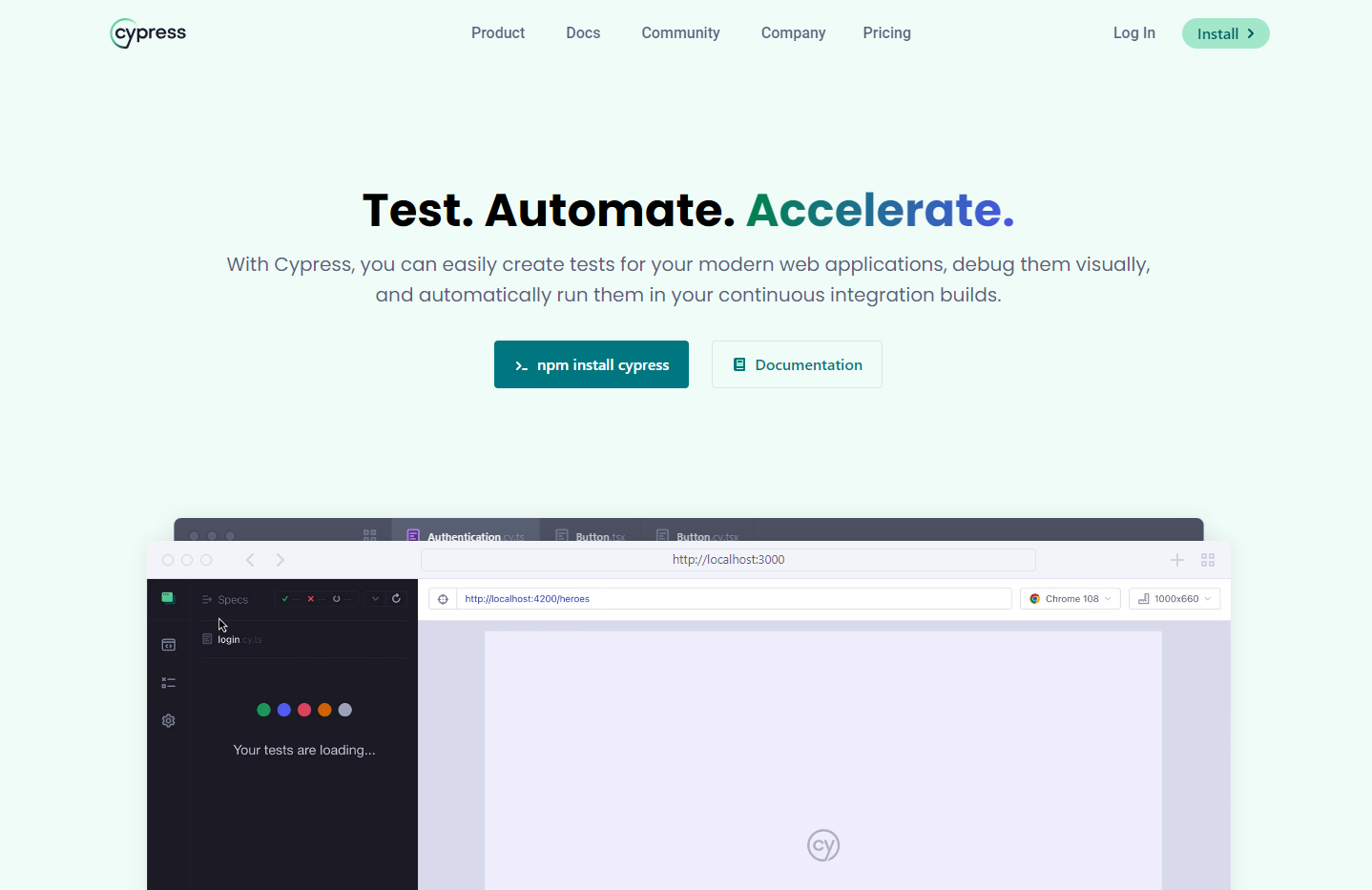
Cypress is your testing soulmate if you crave instant feedback and a developer-friendly experience. This framework operates directly in the browser, allowing you to see changes reflected in real time as you write tests. Its intuitive API and familiar JavaScript syntax make it a joy to use, even for testing veterans.
Advantages of Cypress
- Real-time reloading: Cypress provides real-time reloading during test development, allowing you to see changes instantly without requiring manual test reruns.
- Easy setup: Developers can get started quickly since it has a straightforward setup process.
- Developer-friendly API: Write tests in familiar JavaScript, no learning curve.
- Time travel debugging: Rewind and replay tests to pinpoint issues.
Disadvantages of Cypress
- Limited cross-browser support: Cypress currently supports only Chrome.
- No native mobile testing: Does not have built-in support for mobile testing. You must use additional tools or frameworks alongside Cypress to test your app on mobile devices.
Installation
You can easily install Cypress using NPM.
// NPM npm install --save-dev cypress
Usage
The following code shows a simple Cypress unit test to verify the functionality of multiplying two numbers.
describe('Multiplication Test', () => { it('should multiply two numbers correctly', () => { cy.get('#number1').type('2'); cy.get('#number2').type('3'); cy.get('#multiplyButton').click(); cy.get('#result').should('have.text', '6'); }); });

Easily build real-time apps with Syncfusion’s high-performance, lightweight, modular, and responsive JavaScript UI components.
Conclusion
Thanks for reading! Remember, there’s no one-size-fits-all solution in unit testing frameworks. Consider your project’s specific needs, your team’s experience, and your testing style when choosing the perfect match. Hopefully, this guide has equipped you with the knowledge to navigate the jungle and find your JavaScript unit test framework soulmate!
The Syncfusion JavaScript suite is a comprehensive solution for app development, offering high-performance, lightweight, modular, and responsive UI components. We encourage you to download the free trial and assess these controls.
If you have any questions or feedback, you can contact us through our support forums, support portal, or feedback portal. We’re always here to help you!