The Syncfusion .NET PDF Library offers a comprehensive solution for securing PDF documents and ensuring the confidentiality and integrity of sensitive information. Users can leverage the PDF Library to implement various security measures and safeguard their PDF files.
The framework also empowers users to apply permissions and restrictions to PDF documents, granting precise control over various actions, including printing, copying, modifying, and content extraction. This capability lets document owners control their PDF files, preventing unauthorized distribution or modification.
Users can encrypt their PDF documents using the following encryption algorithms:
- Rivest Cipher 4 (RC4)
- Advanced Encryption Standard (AES)
In this article, we will delve into the capabilities of the Syncfusion .NET PDF Library to secure PDF documents. We will cover the following topics:
- Encrypt PDF documents with a user password
- Encrypt PDF documents with an owner’s password
- Protect a PDF document
- Change the password of a PDF document
- Change the permissions of a PDF document
- Protect attachments in a PDF document
- Remove the password from a PDF document

Experience a leap in PDF technology with Syncfusion's PDF Library, shaping the future of digital document processing.
Getting started with app creation
- First, create a .NET console application using Visual Studio.
- Go to Tools -> NuGet package Manager -> Package Manager Console and open the Package Manager Console.
- Next, execute the following command to install the Syncfusion.Pdf.Net.Core NuGet package.
Install-Package Syncfusion.Pdf.Net.Core
Encrypt a PDF document with a user password
By setting a user password for a PDF document, you can prevent unauthorized individuals from opening or viewing it. When attempting to open the PDF document, Adobe Acrobat or Reader will prompt the user to enter the user password.
If the user password entered is incorrect, the document will remain inaccessible. This way, you can effectively secure your PDF documents.
Follow these steps to encrypt PDF documents with a user password:
- Create a new PDF document using the PdfDocument class.
- Then, add a new blank page using the PdfPage class.
- Create an instance of the PdfSecurity class.
- Specify the Algorithm property as RC4 through the PdfEncryptionAlgorithm enum and the KeySize property as 40bit or 128bit through the PdfEncryptionKeySize enum in the PdfSecurity class.
- Set the UserPassword property of the PdfSecurity class.
- Add some text using the DrawString method of the PdfGraphics class.
- Finally, save the PDF document to the memory stream.
Refer to the following code example to encrypt the PDF document with a UserPassword.
//Create a new PDF document. PdfDocument document = new PdfDocument(); //Add a page to the document. PdfPage page = document.Pages.Add(); //Create PDF graphics for the page. PdfGraphics graphics = page.Graphics; //Create instance of document security. PdfSecurity security = document.Security; //Specifies key size, encryption algorithm and user password. security.KeySize = PdfEncryptionKeySize.Key128Bit; security.Algorithm = PdfEncryptionAlgorithm.RC4; security.UserPassword = "password"; //Draw the text. graphics.DrawString("Encrypt PDF with user password and RC4 128bit keysize", new PdfStandardFont(PdfFontFamily.TimesRoman, 20f, PdfFontStyle.Bold), PdfBrushes.Black, new PointF(0, 40)); //Save the document into stream. MemoryStream stream = new MemoryStream(); document.Save(stream); //Close the document. document.Close(true);
By executing the previous code example, our PDF document will be protected with a user password, showing the security settings as follows.
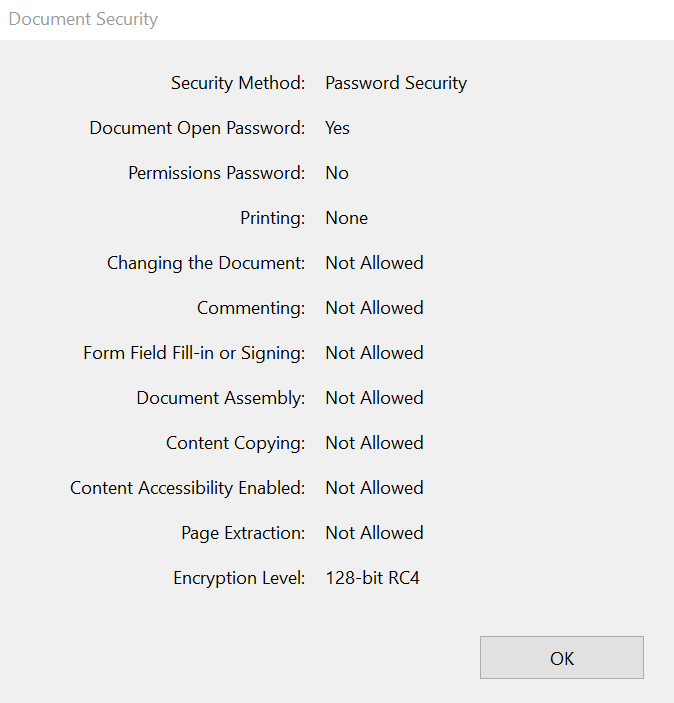

Explore the wide array of rich features in Syncfusion's PDF Library through step-by-step instructions and best practices.
Encrypt a PDF document with an owner password
By setting an owner password, you can establish various PDF document restrictions, such as printing, content copying, editing, page extracting, and commenting. Acrobat will then prompt for this password whenever any changes need to be made to the PDF. Applying a PDF owner password provides an additional layer of security, further protecting the integrity of the PDF document.
Follow these steps to encrypt a PDF document with an owner password:
- Initialize a new instance of the PdfDocument class.
- Add a new blank page using the PdfPage class.
- Create an instance of the PdfSecurity class.
- Specify the Algorithm property as AES through the PdfEncryptionAlgorithm enum and the KeySize property as 40bit, 128bit, or 256bit through the PdfEncryptionKeySize enum in the PdfSecurity class.
- Set the OwnerPassword property of the PdfSecurity class.
- Add some text using the DrawString method of the PdfGraphics class.
- Save the PDF document to the memory stream.
Refer to the following code example to encrypt the PDF document with an OwnerPassword.
//Create a new PDF document. PdfDocument document = new PdfDocument(); //Add a page to the document. PdfPage page = document.Pages.Add(); //Create PDF graphics for the page. PdfGraphics graphics = page.Graphics; //Create instance of document security. PdfSecurity security = document.Security; //Specifies key size, encryption algorithm and user password. security.KeySize = PdfEncryptionKeySize.Key256Bit; security.Algorithm = PdfEncryptionAlgorithm.AES; security.OwnerPassword = "Syncfusion"; //Draw the text. graphics.DrawString("This document protected with owner password", new PdfStandardFont(PdfFontFamily.TimesRoman, 20f, PdfFontStyle.Bold), PdfBrushes.Black, new PointF(0, 40)); //Save the document into stream. MemoryStream stream = new MemoryStream(); document.Save(stream); //Close the document. document.Close(true);
By executing the previous code example, our PDF document will be protected with an owner password, and it will show the security settings as follows.
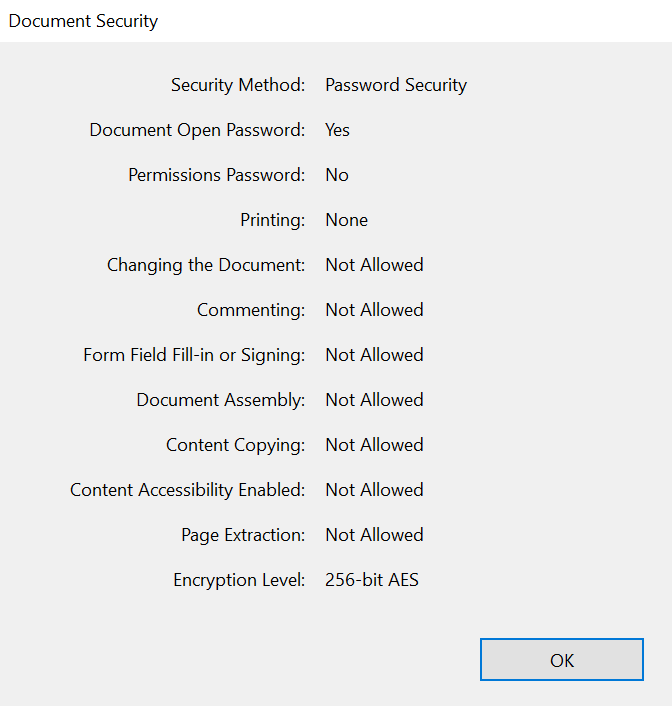
Protect a PDF document
With the help of our .NET PDF Library, you can easily protect a PDF document. To do so, please follow these steps:
- Use the PdfLoadedDocument class to load an existing PDF document.
- Create an instance of the PdfSecurity class.
- Specify the Algorithm property as AES through the PdfEncryptionAlgorithm enum and the KeySize property as 40bit, 128bit, or 256bit through the PdfEncryptionKeySize enum in the PdfSecurity class.
- Set the owner and user passwords using the UserPassword and OwnerPassword properties of the PdfSecurity class.
- Save the PDF document to the memory stream.
Refer to the following code example.
//Load the PDF document. FileStream docStream = new FileStream("Input.pdf", FileMode.Open, FileAccess.Read); PdfLoadedDocument document = new PdfLoadedDocument(docStream); //PDF document security. PdfSecurity security = document.Security; //Specifies encryption key size, algorithm, and permission. security.KeySize = PdfEncryptionKeySize.Key256Bit; security.Algorithm = PdfEncryptionAlgorithm.AES; //Provide owner and user password. security.OwnerPassword = "ownerPassword256"; security.UserPassword = "userPassword256"; //Save the document into stream. MemoryStream stream = new MemoryStream(); document.Save(stream); //Close the document. document.Close(true);
By executing this code example, you will get a PDF document like the following image.
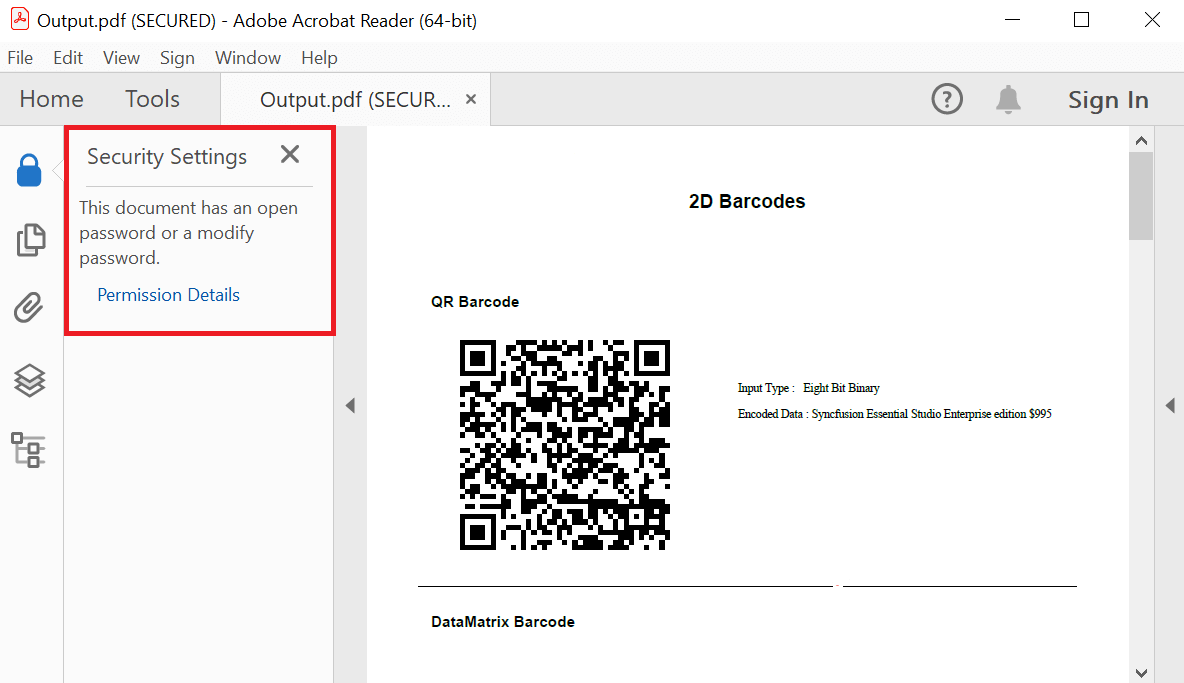

Witness the advanced capabilities of Syncfusion's PDF Library with feature showcases.
Change the password of a PDF document
The Syncfusion .NET PDF Library allows you to change both the user and owner passwords of a PDF document. This lets you enhance the existing password strength or grant access to authorized individuals.
Follow these steps to change the password of a PDF document:
- Use the PdfLoadedDocument class to load an existing PDF document.
- Change the password of the PDF document using the UserPassword and OwnerPassword properties of the PdfSecurity class.
- Save the PDF document into the memory stream.
Refer to the following code example.
//Load an existing PDF document. FileStream docStream = new FileStream("Input.pdf", FileMode.Open, FileAccess.Read); PdfLoadedDocument loadedDocument = new PdfLoadedDocument(docStream, "ownerPassword256"); //Change the user password. loadedDocument.Security.UserPassword = "NewUserPassword"; //Change the owner password. loadedDocument.Security.OwnerPassword = "NewOwnerPassword"; //Save the document into stream. MemoryStream stream = new MemoryStream(); loadedDocument.Save(stream); //Close the document. loadedDocument.Close(true);
Change the permissions of a PDF document
You can modify permissions for a PDF document using the .NET PDF Library. This will provide you complete control over access, editing, printing, and other operations performed on the file.
Follow these steps to change the permissions of a PDF document:
- Use the PdfLoadedDocument class to load an existing PDF document.
- Change the permission settings of the PDF document using the Permissions property of PdfSecurity class.
- Save the modified document into the memory stream.
Refer to the following code example.
//Load the PDF document. FileStream docStream = new FileStream("Input.pdf", FileMode.Open, FileAccess.Read); PdfLoadedDocument loadedDocument = new PdfLoadedDocument(docStream, "ownerPassword256"); //Change the permission. loadedDocument.Security.Permissions = PdfPermissionsFlags.CopyContent | PdfPermissionsFlags.AssembleDocument; //Save the document into stream. MemoryStream stream = new MemoryStream(); loadedDocument.Save(stream); //Close the PDF document. loadedDocument.Close(true);
Refer to the following images.
![]() | ![]() |
Changing the permissions of a PDF document
Protect attachments in a PDF document
We can also encrypt attachments in a PDF document with strong passwords. This adds authenticity to the PDF.
Follow these steps to protect attachments in a PDF document:
- Use the PdfLoadedDocument class to load a PDF document.
- Create an instance of the PdfSecurity class.
- Specify the Algorithm property as AES through the PdfEncryptionAlgorithm enum and the KeySize property as 40bit, 128bit, or 256bit through the PdfEncryptionKeySize enum in the PdfSecurity class.
- Set the user password using the UserPassword property of the PdfSecurity class.
- Specify the EncryptionOptions property as EncryptOnlyAttachments through the PdfEncryptionOptions enum in the PdfSecurity class. This will encrypt only the attachment files in a PDF document.
- Save the PDF document to the memory stream.
Refer to the following code example.
//Load the PDF document. FileStream docStream = new FileStream("Input.pdf", FileMode.Open, FileAccess.Read); PdfLoadedDocument document = new PdfLoadedDocument(docStream); //PDF document security. PdfSecurity security = document.Security; //Specifies encryption key size, algorithm and permission. security.KeySize = PdfEncryptionKeySize.Key256Bit; security.Algorithm = PdfEncryptionAlgorithm.AES; //Provide user password. security.UserPassword = "password"; //Specifies encryption option. security.EncryptionOptions = PdfEncryptionOptions.EncryptOnlyAttachments; //Save the document into stream. MemoryStream stream = new MemoryStream(); document.Save(stream); //Close the document. document.Close(true);

See a world of document processing possibilities in Syncfusion's PDF Library as we unveil its features in interactive demonstrations.
Remove a password from a PDF document
The .NET PDF Library also enables you to remove passwords from PDF documents. This lets you regain unrestricted access to the content, facilitating seamless editing, printing, and other operations without the need for password authentication.
Follow these steps to remove a password from a PDF document:
- Use the PdfLoadedDocument class to load an encrypted PDF document.
- Remove the UserPassword from the PDF document by setting it to an empty string.
- Save the modified document into the memory stream.
Refer to the following code example.
//Load the PDF document. FileStream docStream = new FileStream("Input.pdf", FileMode.Open, FileAccess.Read); PdfLoadedDocument loadedDocument = new PdfLoadedDocument(docStream, "password"); //Change the user password. loadedDocument.Security.UserPassword= string.Empty; //Save the document into stream. MemoryStream stream = new MemoryStream(); loadedDocument.Save(stream); //Close the document. loadedDocument.Close(true);
By executing this code example, you can remove the password protection from a PDF document. The resulting PDF document will not have password protection and can be freely opened, edited, and printed.
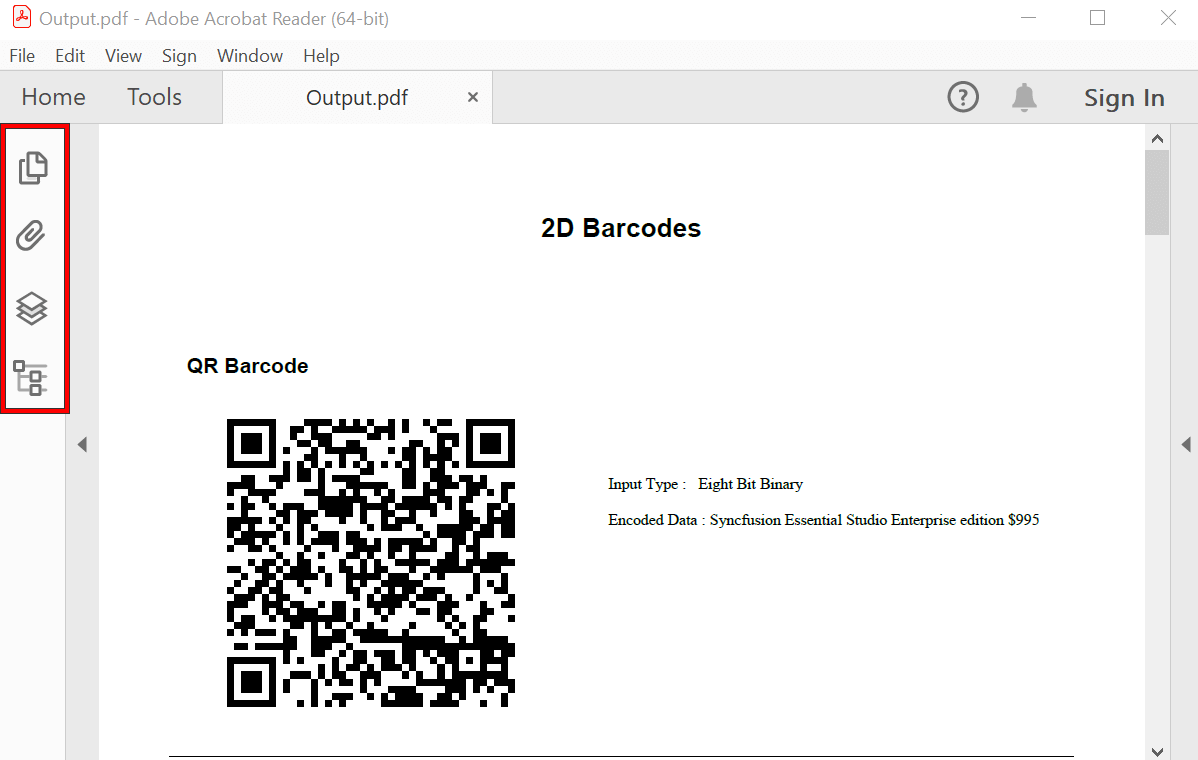
GitHub reference
To gain a better understanding, you can explore examples for Securing a PDF document using C# on our GitHub repository.

Syncfusion’s high-performance PDF Library allows you to create PDF documents from scratch without Adobe dependencies.
Conclusion
Thanks for reading! In this blog, we’ve seen the various security protection options available in our Syncfusion .NET PDF Library using C#. With these features, you can enhance the confidentiality, integrity, and authenticity of your PDF documents, ensuring they remain secure throughout their lifecycle.
Take a moment to look at the documentation, where you will find other options and features, all with accompanying code samples.
Please let us know in the comments below if you have any questions about these features. You can also contact us through our support forum, support portal, or feedback portal. We are always happy to assist you!
Comments (1)
I am using the following code, which create a document from a template merge. However when you open it, it says, “There is an error on this page. Acrobat may not display the page correctly Please contact…” Note: This does not happen when I do not apply the encryption, so the merge and everything else works perfectly. I also notice that if I accept the message, then click on the ‘lock’ icon, that it displays perfectly.
document.MailMerge.Execute(fieldNames, fieldValues);
DocIORenderer render = new DocIORenderer();
Syncfusion.Pdf.PdfDocument pdfDocument = render.ConvertToPDF(document);
pdfDocument.Compression = Syncfusion.Pdf.PdfCompressionLevel.Best;
PdfSecurity security = pdfDocument.Security;
security.KeySize = PdfEncryptionKeySize.Key256Bit;
security.Algorithm = PdfEncryptionAlgorithm.AES;
security.OwnerPassword = “user”;
//convert to stream and store in blob storage
render.Dispose();
document.Dispose();
MemoryStream stream = new();
stream.Position = 0;
pdfDocument.Save(stream);
pdfDocument.Close();
stream.Position = 0;
await _azureBlobStorage.StoreStreamAsync(stream, $”Certificate{captureId}.pdf”, “certificates”);
stream.Close();
Comments are closed.