If you are a mobile app developer and love to use the Flutter framework, you’ll be thrilled to know that the Syncfusion Flutter Charts package is available on pub.dev.
The Syncfusion Flutter Charts package is a data-visualization library written natively in Dart for creating beautiful and high-performance charts for high-quality mobile app user interfaces for iOS and Android using the Flutter framework. It allows users to plot data and render the customized chart types with seamless interactions and responsively smooth animations.
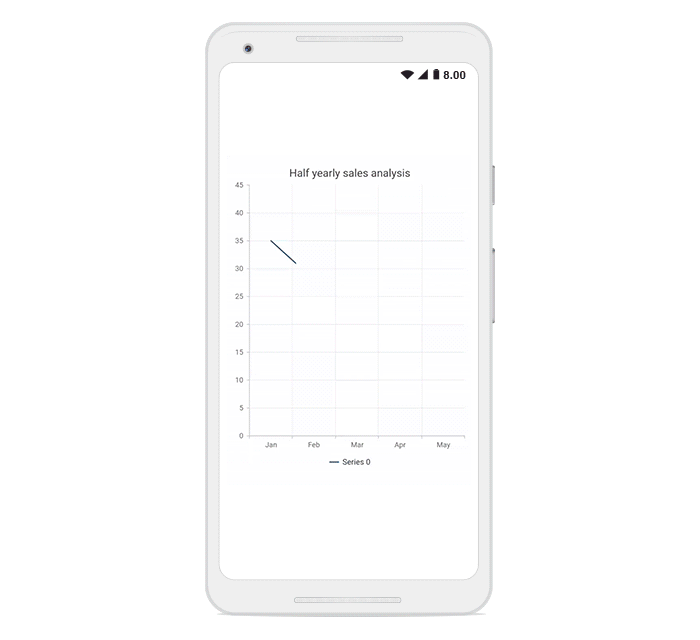
What is Flutter?
Flutter is a Google’s mobile app development SDK that has widgets and tools for creating natively compiled cross-platform mobile applications from a single code base. Flutter has the following advantages over other cross-platform mobile app development frameworks:
- Fast development cycle: With stateful hot reload, you can repaint the app live without building it again.
- Single code base: Use a single code base to build applications in iOS and Android.
- Flexible UIs and widgets: A wide range of widgets and tools are available for building flexible apps.
- Native performance: The Flutter framework provides complete native support, even for scrolling, navigation, icons, and fonts.
- Open source: Flutter is open-source and available to use or study at free of cost.
Flutter charts features
Syncfusion Flutter charts are rich in features, including functionality for rendering Cartesian charts, circular charts, pyramid and funnel charts. They are completely customizable and extendable, and their feature lists will expand aggressively in upcoming updates. Have a look at the following current features.
Chart types
The chart widget includes functionality for plotting 30+ series types:
- Line
- Spline
- Area
- Step line
- Fast line
- Column
- Bar
- Bubble
- Scatter
- Range column
- Stacked line
- Stacked area
- Stacked column
- Stacked bar
- Pie
- Doughnut
- Radial bar
- Pyramid
- Funnel
- Spline area
- Step area
- Range area
- Spline range area
- 100% stacked line
- 100% stacked area
- 100% stacked column
- 100% stacked bar
- Histogram
- Box and whisker
- Waterfall
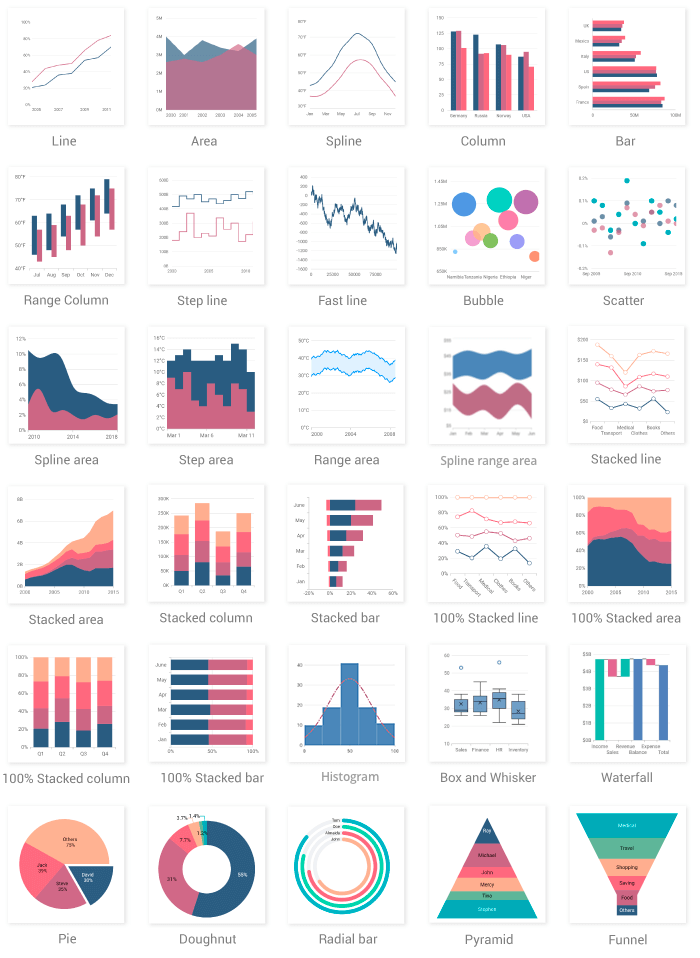
Each chart type is easily configured with built-in support for creating stunning visual effects. You can add any number of series to the chart. Features such as markers, data labels, data label builder, animation, gradient fill, dashes, sorting, and annotations are easy to incorporate and customize.
Axis types
Plot any type of data in a graph using these four axes types:
- Numeric
- Category
- Date-time
- Logarithmic
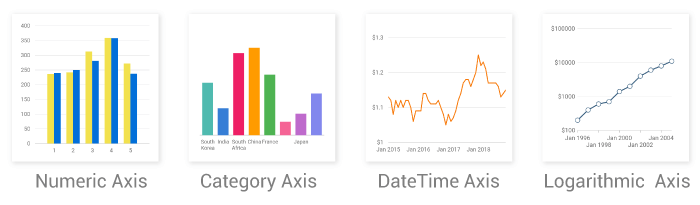
Axis features such as label intersecting, edge label placement, label rotation, axis opposition, inverse axis, and multiple axis allow users to customize axis elements further to make an axis more readable.
User interaction
User experience is greatly enhanced by the following interaction features:
- Zooming and panning
- Crosshairs
- Trackballs
- Drilling down
- Events
- Selection
- Tooltips
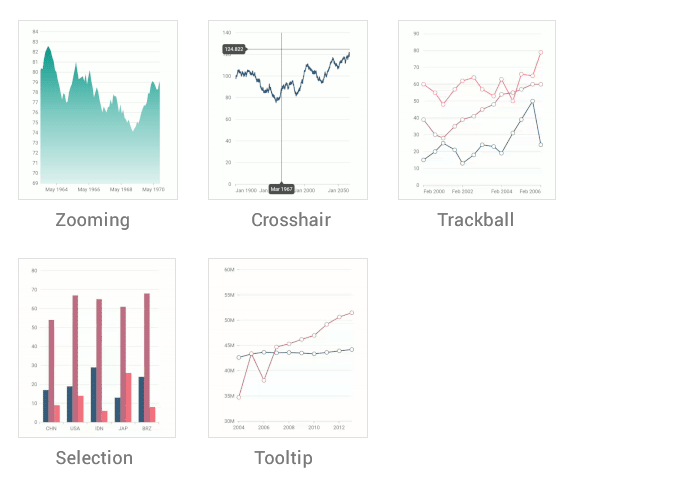
Legend
Display additional information about a chart series using legends. The chart legends can also be used to collapse the series. Legends can be wrapped or scrolled if items exceed the available bounds.
Dynamic updates
A chart can be updated dynamically with live data that changes in seconds, like stock prices, temperature, and speed (i.e., data points or series can be added or removed from the rendered chart dynamically). Also, the entire data source of the chart can be replaced completely.
We are planning to add more chart types, as well as richer charts, in future releases.
Add Flutter Charts to your app
This section explains how to create a simple chart and demonstrates its basic usage.
Add dependency
Add the Syncfusion Flutter Charts dependency to your the pubspec.yaml file.
dependencies: syncfusion_flutter_charts: ^17.3.26
Get packages
Run the following command to get the required packages.
$ flutter pub get
Import package
Import the following package in your Dart code.
import 'package:syncfusion_flutter_charts/charts.dart';
Add a chart to the widget tree
Add a chart widget as a child of any widget. Here, we are adding the chart widget as a child of the container widget.
@override Widget build(BuildContext context) { return Scaffold( body: Center( child: Container( child: SfCartesianChart( ) ) ) ); }
Bind data source
Based on your data, initialize the appropriate axis type and series type. In the series, you need to map the data source and the fields for x and y data points. Since we are going to render a line chart with a category axis, I have initialized them.
@override Widget build(BuildContext context) { return Scaffold( body: Center( child: Container( child: SfCartesianChart( primaryXAxis: CategoryAxis(), // Initialize category axis. series: <LineSeries<SalesData, String>>[ // Initialize line series. LineSeries<SalesData, String>( dataSource: [ SalesData('Jan', 35), SalesData('Feb', 28), SalesData('Mar', 34), SalesData('Apr', 32), SalesData('May', 40) ], xValueMapper: (SalesData sales, _) => sales.year, yValueMapper: (SalesData sales, _) => sales.sales ) ] ) ) ) ); } class SalesData { SalesData(this.year, this.sales); final String year; final double sales; }
Add chart elements
Finally, add Syncfusion Flutter Charts elements such as title, legend data labels, and tooltips to display additional information about the data plotted in chart.
@override Widget build(BuildContext context) { return Scaffold( body: Center( child: Container( child: SfCartesianChart( primaryXAxis: CategoryAxis(), title: ChartTitle(text: 'Half yearly sales analysis'), //Chart title. legend: Legend(isVisible: true), // Enables the legend. tooltipBehavior: TooltipBehavior(enable: true), // Enables the tooltip. series: <LineSeries<SalesData, String>>[ LineSeries<SalesData, String>( dataSource: [ SalesData('Jan', 35), SalesData('Feb', 28), SalesData('Mar', 34), SalesData('Apr', 32), SalesData('May', 40) ], xValueMapper: (SalesData sales, _) => sales.year, yValueMapper: (SalesData sales, _) => sales.sales, dataLabelSettings: DataLabelSettings(isVisible: true) // Enables the data label. ) ] ) ) ) ); }
The following screenshot illustrates the chart rendered using the above code snippet.
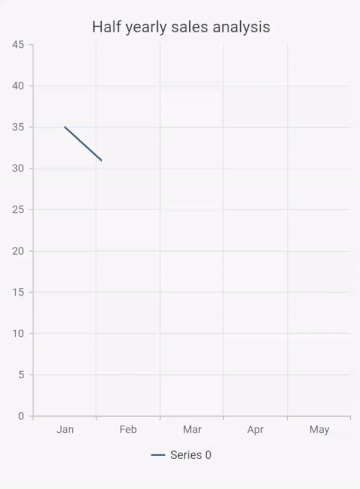
If you want an in-depth learning experience on creating a complete Flutter app, read Flutter Succinctly by Ed Freitas. It’s a part of Syncfusion’s library of free technical ebooks.
Upcoming development plans
Next, we are planning to implement Calendar and other data visualization widgets in Flutter and add chart types and usability features in the Charts widget.
We prioritize our development features and widgets based on developers’ needs. If you would like us to add a new widget, or if you have questions about our Flutter charts, please let us know in the comments section of this post. Unlock the power of Syncfusion’s highly customizable Flutter widgets.
Conclusion
In this blog post, we walked through our new Syncfusion Flutter Charts widget and discussed our future plans. We invite you to try out the widget and provide your feedback to make it more robust before its final release. As always, we are happy to assist you!
You can also contact us through our support forum, support portal, or feedback portal. This helps us prioritize the next set of controls and features to implement.
- You can see Syncfusion’s Flutter app with many examples in this GitHub.
- Additionally, take a look at our demo app in Google Play Store for Android devices and in App Store for iOS devices.
- Find all the available features in the chart from Syncfusion Flutter Charts product page.
- User guide documentation
- Video tutorials
Comments (21)
nice work! is it possible to make something that greatly resembles Apple Watch rings? with all the effects and gradients?
Hi MARCO POLO,
Thanks for trying Syncfusion Flutter widgets.
Yes. You can simulate the appearance of Apple watch rings with the existing chart features. Please find the output from the below link.
https://www.syncfusion.com/downloads/support/directtrac/general/output-2007561565
Here we have rendered the radial bar chart with 3 values (move, stand and exercise). Also, legend and tooltip have enabled in this example. We have attached the example project below for your reference.
https://www.syncfusion.com/downloads/support/directtrac/general/ze/APPLEW~11177283415
For any other queries, reach our Syncfusion support team (https://www.syncfusion.com/support/directtrac/incidents/newincident) or post the queries through our Community forums (https://www.syncfusion.com/forums/flutter).
Thanks,
Jayavigneshwaran
Hi,
I’m developing a diabetes tracker app and I need to display some graphs in it. At the moment I’m using the “official” charts_flutter package, which I had to edit to solve some issues.
I’m always looking for a maintained package to substitute it and I look pretty seriously at yours. The main features I need are: range overlay on graph based on axis position, multiple series on single graph and possibly annotation points below axis. Does your package provide this features?
This is an example of graph I would like to obtain: https://imgur.com/a/PSrspeA
Right now I’m stuck with a problem: I need to embed a graph in a pdf report, but I cannot add any widget in a pdf, just images. Does you package provide a method to save the chart as a image? I know that a Custompainter and Canvas can be saved as Image but I can’t figure out a solution for it.
Thanks in advance,
Luca
Hi Luca,
We have analyzed your queries. I hereby confirm that the Syncfusion Flutter widgets are highly maintainable. As stated in the blog, for any other queries/bugs/new feature requests, you can reach our Syncfusion support team (https://www.syncfusion.com/support/directtrac/incidents/newincident) or post the queries through our Community forums (https://www.syncfusion.com/forums/flutter). We will revert back to you within 24 hours.
You can render multiple series types in a chart and you can find the sample for this from the below location. Here we have rendered column and line series together.
https://github.com/syncfusion/flutter-examples/blob/master/lib/samples/chart/series_features/animation/series_animation.dart
‘Range overlay on graph based on axis position’ feature is currently in progress and it will be included in our next update. But as of now, there is no support to render annotations as you have mentioned in the screenshot and exporting support. We will consider these as new feature requests and implement it based on the priority of other features.
Please let us know if you have any concerns.
Thanks,
Jayavigneshwaran
Will this library support vertical bar charts? I see this chart type is currently available in your Xamarin library, but not in your Flutter library. I have a link to the page, as well as a direct link to the image on the page, down below.
https://www.syncfusion.com/xamarin-ui-controls/xamarin-charts
https://www.syncfusion.com/products/xamarin/control/images/chart/xamarin.forms-charts-legend-wrapping.png
Hi JEREMY,
Vertical bar chart(Column chart) is available in Flutter now. Please find the below screenshot created using Flutter Charts widget.
https://help.syncfusion.com/flutter/chart/images/cartesian-chart-types/column.jpg
Sample code can be found from the below link.
https://github.com/syncfusion/flutter-examples/blob/master/lib/samples/chart/cartesian_charts/column_series/default_column_chart.dart
But as of now, stacking vertical bar chart(Stacking column chart) is not available in Flutter charts. We are working on this and this chart type will be included in our next update.
https://www.syncfusion.com/products/xamarin/control/images/chart/xamarin.forms-charts-legend-wrapping.png
Thanks,
Jayavigneshwaran
Hello, does it possible to use Syncfusion Flutter Charts package for the web?
Hi PAUL,
As of now it is not possible to use the Syncfusion Flutter Charts in Web. As the Flutter itself rolled out preview for web and they may make breaking changes in future, so we didn’t provide support for this now. We will provide once the Flutter releases the stable web version.
Thanks,
Jayavigneshwaran
Hello Sir,
Can we take data from website in syncfusion charts …. If so how can it be done?
Hi SNEHA,
Can you please confirm that are you referring to render our chart with remote data? If so you can fetch the data from the external server and it should be converted to list and then you have to bind it to the chart, as our chart intakes list type data source.
Please let us know if you need any further assistance on this. Also, you can contact us by creating a support incident. (https://www.syncfusion.com/support/directtrac/incidents)
Thanks,
Jayavigneshwaran
Hi,
I have just bought the Flutter Charts package but I dont have the slighest idea how to get rid of the license popup when using the Charts.
Im developing on a MacBook Pro.
Any help is appreciated.
Regards Morten
Hi Morten,
Thanks for using Syncfusion Flutter widgets.
To hide the license information popup in your project, you need to specify the license key which you have bought. Please follow the below steps.
1. Generate the license key
Please follow the steps mentioned in the below link to generate a valid license key.
https://help.syncfusion.com/common/essential-studio/licensing/license-key#how-to-generate-syncfusion-license-key
2. Register that key in your app
Register the license key in the main method of your example and import the ‘syncfusion_flutter_core/core.dart’ library. Please refer the code snippet mentioned in the below page.
https://help.syncfusion.com/common/essential-studio/licensing/license-key#flutter
Now the license popup will not be displayed while running your app.
Please try the above steps and let us know if you face any concerns. Also, you can contact us by creating a support incident. (https://www.syncfusion.com/support/directtrac/incidents)
Thanks,
Jayavigneshwaran
Will this library support force directed graphs or other link node diagrams in the future?
Hi BOBBY,
As of now, we do not provide support force-directed graphs or link node diagrams. You can log this requirement as a new feature request in the below link.
https://www.syncfusion.com/feedback/flutter
So, we will consider this for implementation based on our priorities and complexities. Also while logging the feature request, please mention some related links or screenshots of your requirement, which will help us understand your requirement more clearly.
Thanks,
Jayavigneshwaran
Does the community version for personal development purpose supports all feature? I don’t have a plan to distribute the app yet.
Hi Asme,
The Syncfusion Community License is not just for personal development purpose, but you can distribute the application as well. All the feature available for our paid customers are available for the customers with the Community License as well. For more details and the terms & conditions, please visit the following page,
https://www.syncfusion.com/products/communitylicense
Thanks,
Suresh
Hi Suresh
You are saying that we can develop an app and Publish it in Play Store and App Store without any issues.
Hi Aaqib,
Yes, that is true.
the Unexpected:
I would like to have options to visualization flow/net diagrams as a Petri Net, BPMN, UML (activity), Graphs (nodes and edge).
Hi Levius Tecnologiaa,
The requested diagrams are not supported by Syncfusion. You can submit this requirement as a new feature request using the link provided below.
https://www.syncfusion.com/feedback/flutter
We will consider this requirement based on our priorities and complexities. Also while logging the feature request, please mention some related links or screenshots of your requirement, which will help us understand your requirement more clearly.
Comments are closed.