In real-life scenarios, you often need to compare two Word documents to identify the differences between them.
For example, if you have an original Word document and a revised version, finding the differences between them can be time-consuming. The solution to this challenge is to utilize the Syncfusion .NET Word Library (DocIO).
From the 2023 Volume 3 onwards, Syncfusion .NET Word Library supports programmatically comparing two Word documents with just a few lines of code in C#. This library does not need Microsoft Word or any other interop dependencies. With this functionality, you can easily identify the changes between two versions of a Word document.
Note: If you are new to our Word Library, following our Getting Started guide is highly recommended.

Mastering Word documents is a breeze with the Syncfusion Word Library, simplifying every aspect of document creation and management!
Getting started
Step 1: First, create a new C# .NET Core Console App in Visual Studio.
Step 2: Then, install the Syncfusion.DocIO.Net.Core NuGet package as a reference to the app from the NuGet Gallery.
Step 3: Include the following namespaces in the Program.cs file.
using Syncfusion.DocIO; using Syncfusion.DocIO.DLS;
Now, the project is ready!

Get insights into the Syncfusion’s Word Library and its stunning array of features with its extensive documentation.
Compare two Word documents
Imagine you’ve sent a Word document to someone, and you need to identify the changes they made for review purposes quickly. By comparing two versions of the Word document programmatically, you can quickly identify changes such as insertions, deletions, and format changes.
Refer to the following code example to compare two Word documents using C#.
//Open the file as Stream. using (FileStream originalDocumentFileStream = new FileStream(Path.GetFullPath(@"OriginalDocument.docx"), FileMode.Open)) { //Load the original Word document. using (WordDocument originalDocument = new WordDocument(originalDocumentFileStream, FormatType.Docx)) { //Open the file as Stream. using (FileStream revisedDocumentFileStream = new FileStream(Path.GetFullPath(@"RevisedDocument.docx"), FileMode.Open)) { //Load the revised Word document. using (WordDocument revisedDocument = new WordDocument(revisedDocumentFileStream, FormatType.Docx)) { //Compare the original document with revised document originalDocument.Compare(revisedDocument, "Nancy Davolio", DateTime.Now.AddDays(-1)); //Create the output file stream. using (FileStream fileStreamOutput = File.Create(Path.GetFullPath(@"Output.docx"))) { //Save the document. originalDocument.Save(fileStreamOutput, FormatType.Docx); } } } } }
After executing this code example, we’ll get output like in the following image.
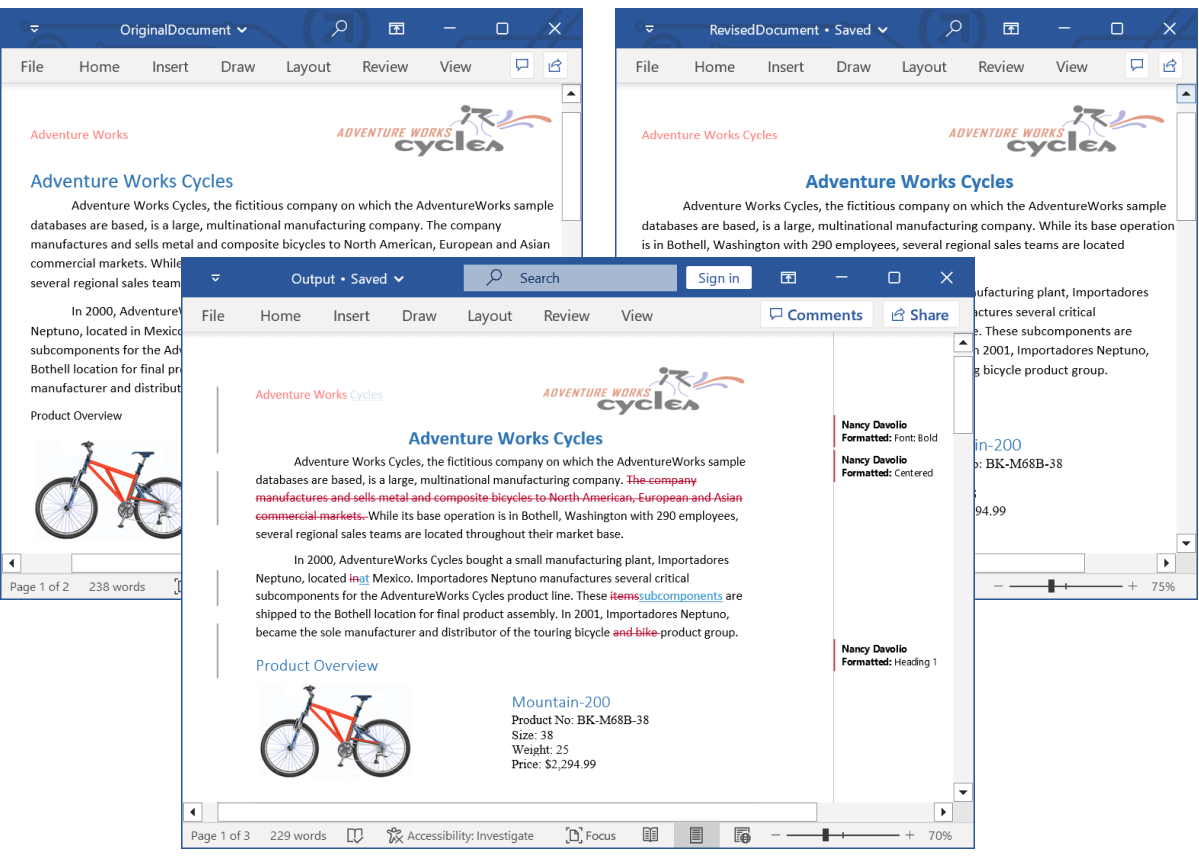

Unearth the endless potential firsthand through demos highlighting the features of the Syncfusion Word Library.
Ignore format changes
Consider a scenario where you’re reviewing the changes in a Word document. Some changes might involve formatting adjustments like font or style modifications, which aren’t substantial. By disabling the DetectFormatChanges flag in your code example, you can focus exclusively on the critical changes, such as content added or removed.
Refer to the following code example to compare two Word documents, but ignore the formatting changes.
// Open the file as Stream. using (FileStream originalDocumentFileStream = new FileStream(Path.GetFullPath(@"OriginalDocument.docx"), FileMode.Open)) { // Load the original Word document. using (WordDocument originalDocument = new WordDocument(originalDocumentFileStream, FormatType.Docx)) { // Open the file as Stream. using (FileStream revisedDocumentFileStream = new FileStream(Path.GetFullPath(@"RevisedDocument.docx"), FileMode.Open)) { // Load the revised Word document. using (WordDocument revisedDocument = new WordDocument(revisedDocumentFileStream, FormatType.Docx)) { // Disable the flag to ignore the formatting changes while comparing the documents. ComparisonOptions comparisonOptions = new ComparisonOptions(); comparisonOptions.DetectFormatChanges = false; // Compare the original document with revised document originalDocument.Compare(revisedDocument, "Nancy Davolio", DateTime.Now.AddDays(-1), comparisonOptions); // Create the output file stream. using (FileStream fileStreamOutput = File.Create(Path.GetFullPath(@"Output.docx"))) { // Save the document. originalDocument.Save(fileStreamOutput, FormatType.Docx); } } } } }
After executing this code example, we’ll get output like in the following image.
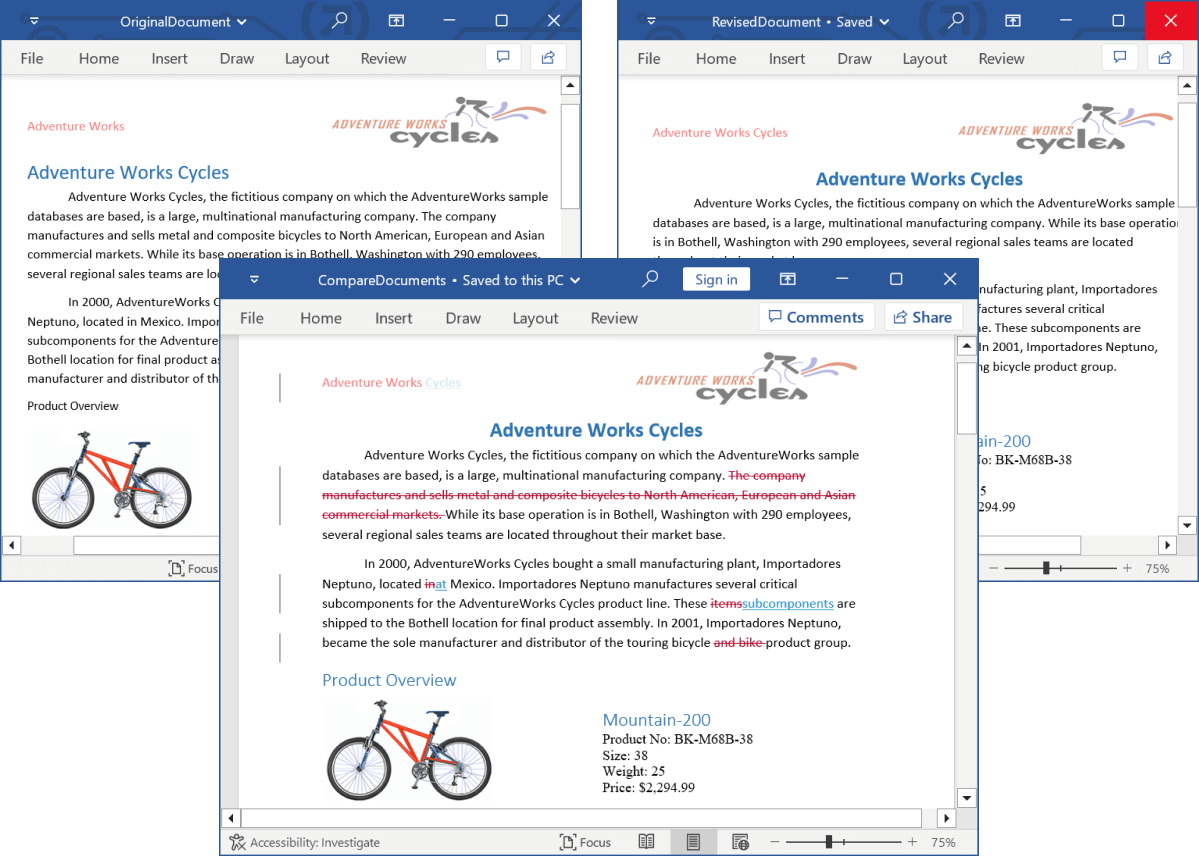
GitHub reference
You can find all the examples for comparing Word documents using C# in the GitHub repository.

The Syncfusion Word Library provides cross-platform compatibility and can be tailored to your unique needs.
Conclusion
Thanks for reading. In this blog, we’ve seen how to compare two Word documents and highlight the difference between them using the Syncfusion .NET Core Word Library (DocIO). Take a moment to peruse its documentation, where you’ll find other options and features, all with accompanying code examples.
Apart from this comparison functionality, our Syncfusion .NET Word Library has the following significant functionalities:
- Create, read, and edit Word documents programmatically.
- Create complex reports by merging data into a Word template from various data sources through mail merge.
- Merge, split, and organize Word documents.
- Convert Word documents into HTML, RTF, PDF, images, and other formats.
You can find more Word Library examples at this GitHub location.
Are you already a Syncfusion user? You can download the product setup here. If you’re not yet a Syncfusion user, you can download a 30-day free trial.
If you have questions, contact us through our support forum, support portal, or feedback portal. We are always happy to assist you!