Bookmarks in PDFs are links or pointers that function as a table of contents, providing an easy way to navigate to specific sections in a document.
In this article, we will use the Syncfusion .NET PDF Library to create, update, and remove bookmarks in PDF documents using C#. With the help of the .NET PDF Library, we can efficiently manage and manipulate PDF documents to enhance the reading experience of our audience.
Agenda:
- Add bookmarks to a PDF document.
- Customize the bookmark style.
- Insert bookmarks in an existing PDF document.
- Modify bookmarks.
- Remove bookmarks from a PDF document.
- Get bookmarks and their page indices from a PDF.

Say goodbye to tedious PDF tasks and hello to effortless document processing with Syncfusion's PDF Library.
Getting started with app creation
- First, create a .NET console application using Visual Studio.
- Then, navigate to Tools > NuGet Package Manager > Package Manager Console.
- Execute the following command to install the Syncfusion.Pdf.Net.Core NuGet package.
Install-Package Syncfusion.Pdf.Net.Core
Add bookmarks to the PDF document
With the help of our .NET PDF Library, you can easily add parent and child bookmarks to both new and existing PDF documents.
Follow these steps to add bookmarks to an existing PDF document:
- Use the PdfLoadedDocument class to load the PDF document.
- Create a bookmark using the PdfBookmark class.
- Set the bookmark destination using the PdfDestination class. This will represent a specific target location within a PDF file where we need to add the bookmark, such as a specific area on a page.
- Finally, save the PDF document to the disk using the Save method.
The following code example shows how to add bookmarks in a PDF document using C#.
//Load an existing PDF document. FileStream docStream = new FileStream("Input.pdf", FileMode.Open, FileAccess.Read); PdfLoadedDocument document = new PdfLoadedDocument(docStream); //Creates parent bookmark. PdfBookmark bookmark = document.Bookmarks.Add("Chapter 1"); //Sets the destination page. bookmark.Destination = new PdfDestination(document.Pages[1]); //Sets the text style and color for the parent bookmark. bookmark.TextStyle = PdfTextStyle.Bold; bookmark.Color = Color.Red; //Sets the destination location for the parent bookmark. bookmark.Destination.Location = new PointF(20, 20); //Adds the child bookmark. PdfBookmark childBookmark = bookmark.Insert(0, “Section 1”); //Sets the destination location for the child bookmark. childBookmark.Destination = new PdfDestination(document.Pages[1]); childBookmark.Destination.Location = new PointF(0, 200); //Save the document to the memory stream. MemoryStream stream = new MemoryStream(); document.Save(stream); //Close the document. document.Close(true);
By executing this code example, you will get a PDF like in the following screenshot.
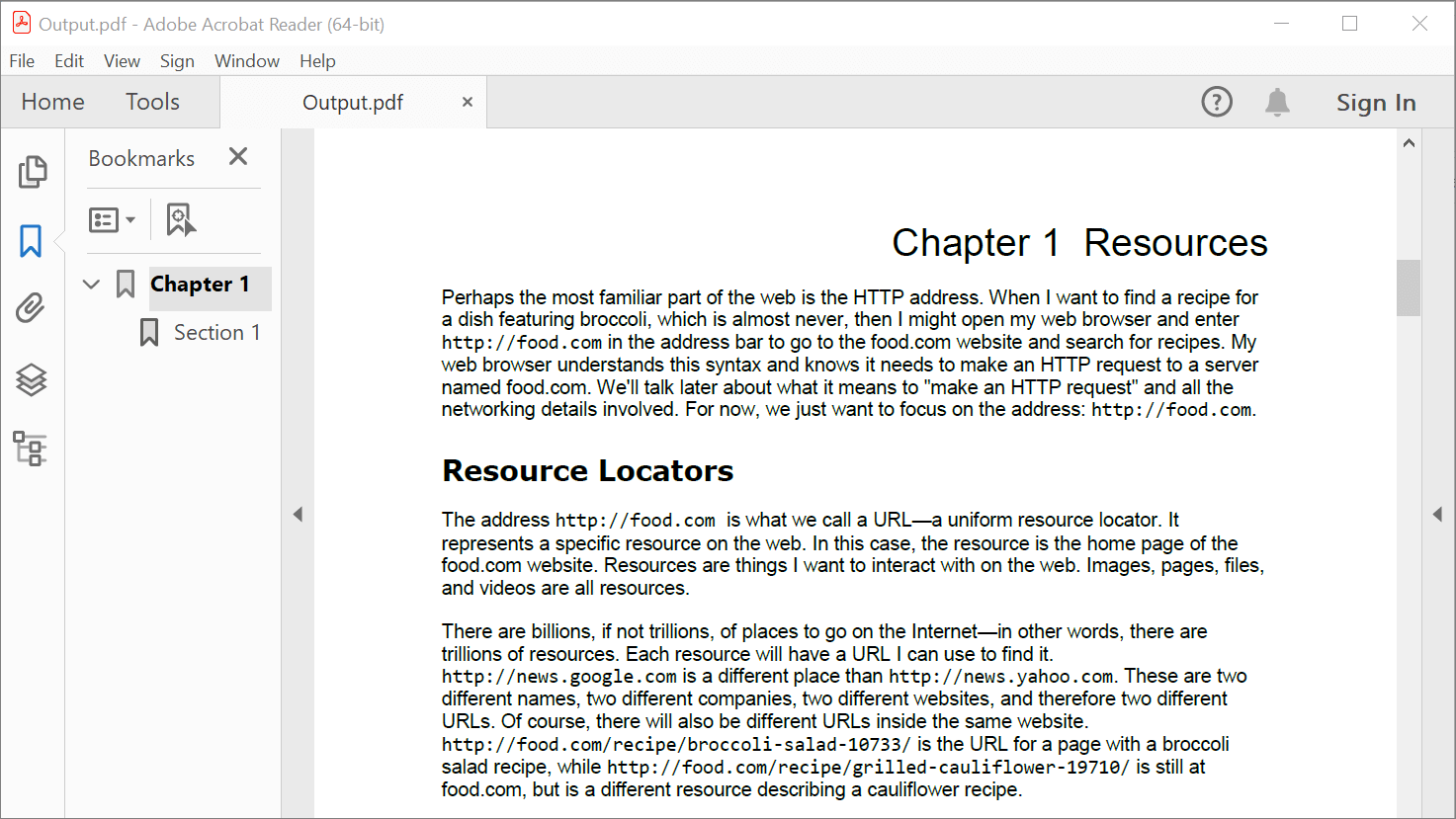

Boost your productivity with Syncfusion's PDF Library! Gain access to invaluable insights and tutorials in our extensive documentation.
Customize the bookmark style
Users can also create customized bookmarks for their PDF documents. Follow these steps:
- Use the PdfLoadedDocument class to load a PDF document.
- Create a bookmark using the PdfBookmark class.
- Then, set the bookmark destination using the PdfDestination class.
- Set the color and text style for the bookmark using the Color and TextStyle properties of PdfBookmark class.
- Finally, save the PDF document to disk using the Save method.
The following code example shows how to customize bookmarks in a PDF document using C#.
//Load an existing PDF document. FileStream docStream = new FileStream(“Input.pdf”, FileMode.Open, FileAccess.Read); PdfLoadedDocument document = new PdfLoadedDocument(docStream); //Creates parent bookmark. PdfBookmark bookmark = document.Bookmarks.Add("Chapter 1"); //Sets the destination page. bookmark.Destination = new PdfDestination(document.Pages[1]); //Sets the text style and color for the parent bookmark. bookmark.TextStyle = PdfTextStyle.Bold; bookmark.Color = Color.DarkBlue; //Sets the destination location for the parent bookmark. bookmark.Destination.Location = new PointF(20, 20); //Adds the child bookmark. PdfBookmark childBookmark = bookmark.Insert(0, "Section 1"); //Sets the text style and color for the child bookmark. childBookmark.TextStyle = PdfTextStyle.Italic; childBookmark.Color = Color.Orange; //Sets the destination location for the child bookmark. childBookmark.Destination = new PdfDestination(document.Pages[1]); childBookmark.Destination.Location = new PointF(0, 200); //Creates parent bookmark. PdfBookmark bookmark2 = document.Bookmarks.Add(“Chapter 2”); //Sets the destination page. bookmark2.Destination = new PdfDestination(document.Pages[1]); //Sets the text style and color for parent bookmark. bookmark2.TextStyle = PdfTextStyle.Bold; bookmark2.Color = Color.DarkBlue; //Sets the destination location for parent bookmark. bookmark2.Destination.Location = new PointF(0, 500); //Adds the child bookmark. PdfBookmark childBookmark2 = bookmark2.Insert(0, "Section 1"); //Sets the text style and color for the child bookmark. childBookmark2.TextStyle = PdfTextStyle.Italic; childBookmark2.Color = Color.Orange; //Sets the destination location for the child bookmark. childBookmark2.Destination = new PdfDestination(document.Pages[1]); childBookmark2.Destination.Location = new PointF(0, 500); //Save the document to memory stream. MemoryStream stream = new MemoryStream(); document.Save(stream); //Close the document. document.Close(true);
By executing the previous code example, you will get a PDF like in the following screenshot.
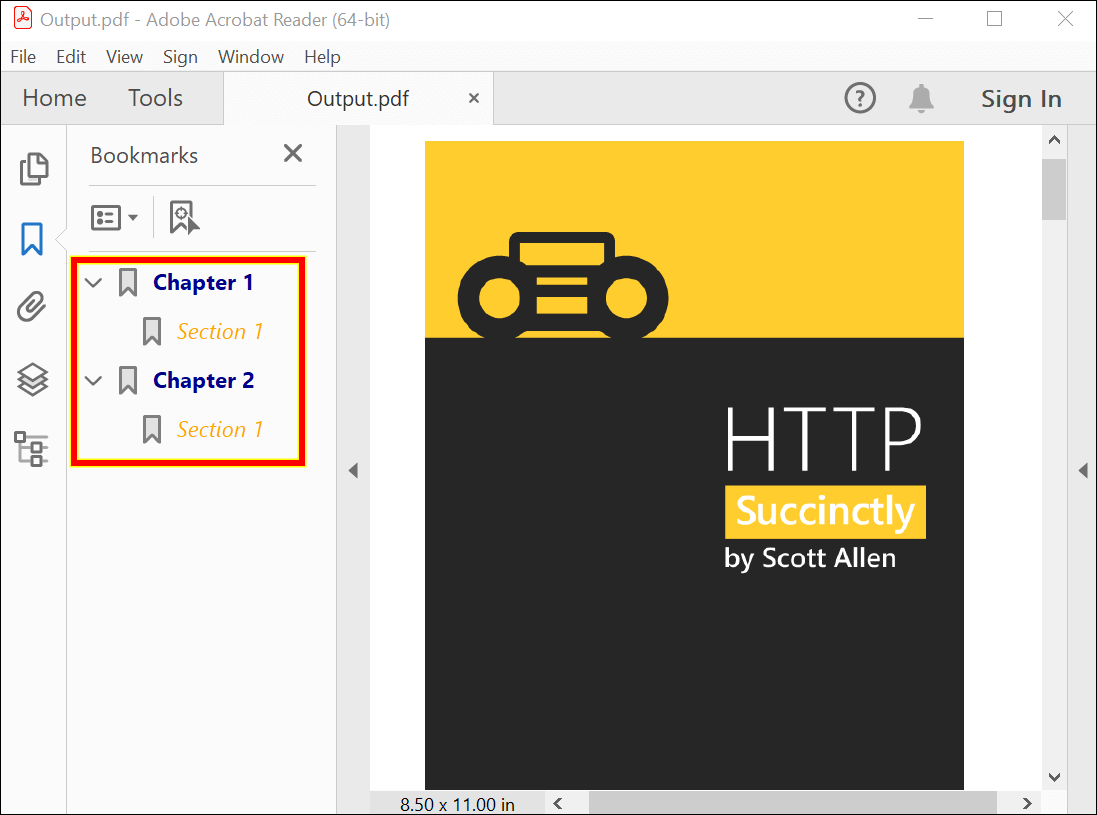
Inserting bookmarks into the existing bookmark collection
The .NET PDF Library enables us to insert bookmarks at any location based on the bookmark collection. Each loaded bookmark is represented by the PdfLoadedBookmark class.
Follow these simple steps to add bookmarks to a PDF document:
- Load the PDF document using the PdfLoadedDocument class.
- Insert a new bookmark in the existing bookmark collection using the Insert method of the PdfBookmarkBase class.
- Set the bookmark destination using the PdfDestination class.
- Finally, save the modified PDF document to the disk using the Save method.
The following code example shows inserting a bookmark into a PDF document using C#.
//Load the PDF document. FileStream docStream = new FileStream("Input.pdf", FileMode.Open, FileAccess.Read); PdfLoadedDocument document = new PdfLoadedDocument(docStream); //Inserts a new bookmark in the existing bookmark collection. PdfBookmark bookmark = document.Bookmarks.Insert(0, "Title Page"); //Sets the destination page and location. bookmark.Destination = new PdfDestination(document.Pages[0]); bookmark.Destination.Location = new PointF(0, 0); //Sets the text style and color. bookmark.TextStyle = PdfTextStyle.Bold; bookmark.Color = Color.Green; //Save the document to memory stream. MemoryStream stream = new MemoryStream(); document.Save(stream); //Closes the document. document.Close(true);
By executing this code example, you will get a PDF like in the following screenshot.
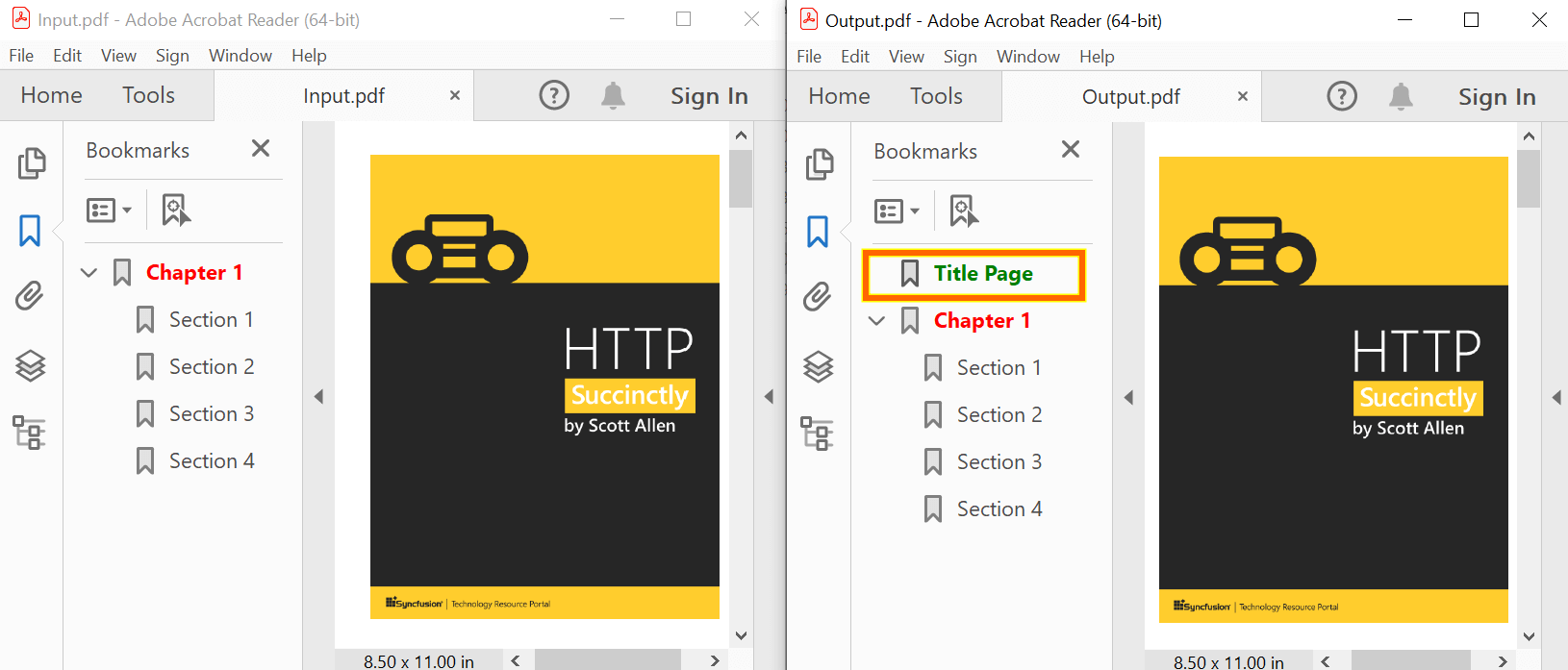

Witness the advanced capabilities of Syncfusion's PDF Library with feature showcases.
Modify bookmarks
Syncfusion’s .NET PDF Library supports modifying bookmarks in PDF documents. You can perform the following modifications to the bookmarks in a PDF document using our .NET PDF Library:
- Modify the bookmark style, color, title, and destination.
- Add new bookmarks to the existing bookmark collection.
- Add new bookmarks as children of another bookmark.
- Assign the destination of the added bookmarks to an existing page or a new document page.
The following code example shows how to modify bookmarks in a PDF document using C#.
//Load the PDF document. FileStream docStream = new FileStream("Input.pdf", FileMode.Open, FileAccess.Read); PdfLoadedDocument document = new PdfLoadedDocument(docStream); //Gets all the bookmarks. PdfBookmarkBase bookmarks = document.Bookmarks; //Gets the first bookmark and changes the properties of the bookmark. PdfLoadedBookmark bookmark = bookmarks[0] as PdfLoadedBookmark; bookmark.Destination = new PdfDestination(document.Pages[2]); bookmark.Color = Color.Green; bookmark.TextStyle = PdfTextStyle.Bold; bookmark.Title = "Chapter 2"; //Save the document to memory stream. MemoryStream stream = new MemoryStream(); document.Save(stream); //Close the document. document.Close(true);
By executing this code example, you will get a PDF like in the following screenshot.
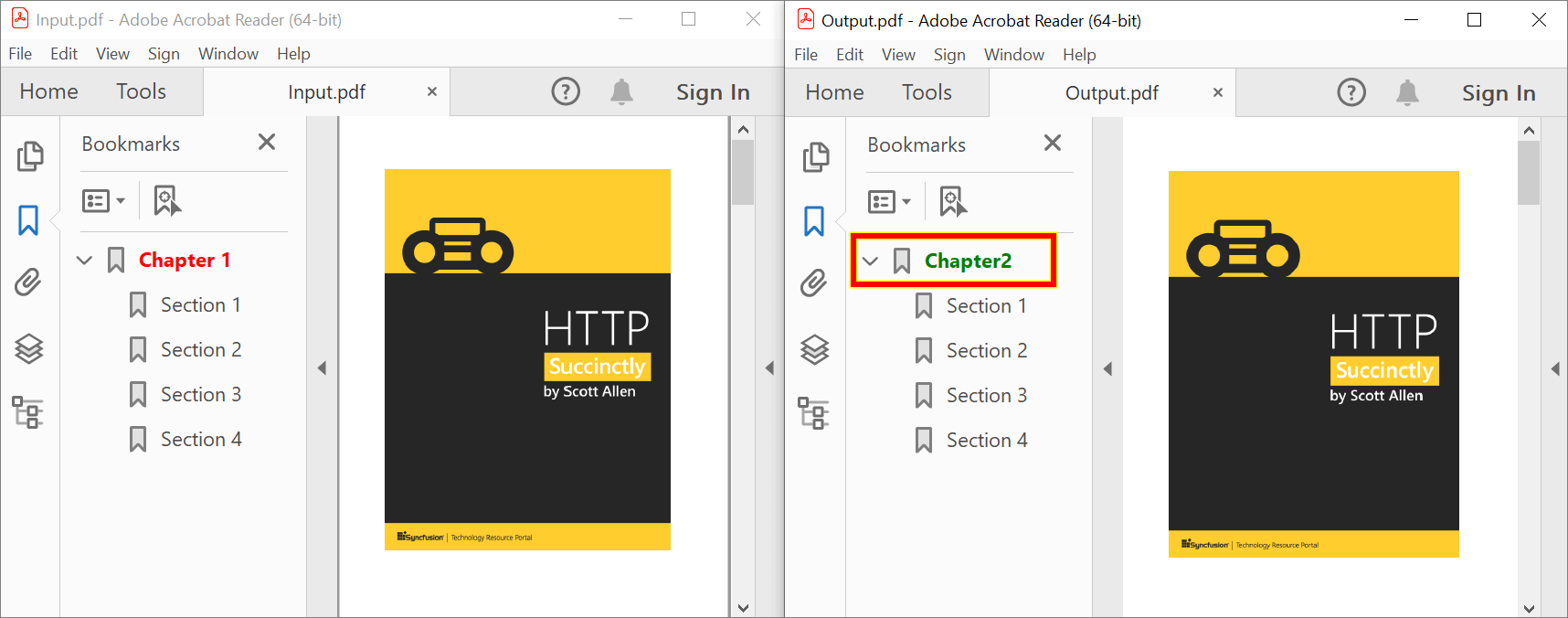
Removing bookmarks from a PDF document
To remove any parent or child bookmark from a PDF document, please follow these steps:
- Load the existing PDF document using the PdfLoadedDocument class.
- Obtain the bookmark collection from the PDF document using the PdfBookmarkBase class.
- Use the Remove (string bookmarkName) or RemoveAt (int bookmarkIndex) method to remove the bookmark.
- Finally, save the modified PDF document to the disk using the Save method.
Refer to the following code example to remove bookmarks from a PDF document using C#.
//Load the PDF document. FileStream docStream = new FileStream("Input.pdf", FileMode.Open, FileAccess.Read); PdfLoadedDocument document = new PdfLoadedDocument(docStream); //Gets all the bookmarks. PdfBookmarkBase bookmarks = document.Bookmarks; //Remove parent bookmark using index. bookmarks.RemoveAt(1); //Remove child bookmark with bookmark name. PdfLoadedBookmark parentBookmark = bookmarks[0] as PdfLoadedBookmark; parentBookmark.Remove("Section 4"); //Save the document to memory stream. MemoryStream stream = new MemoryStream(); document.Save(stream); //Close the document. document.Close(true);
By executing the previous code example, you will get a PDF like in the following screenshot.
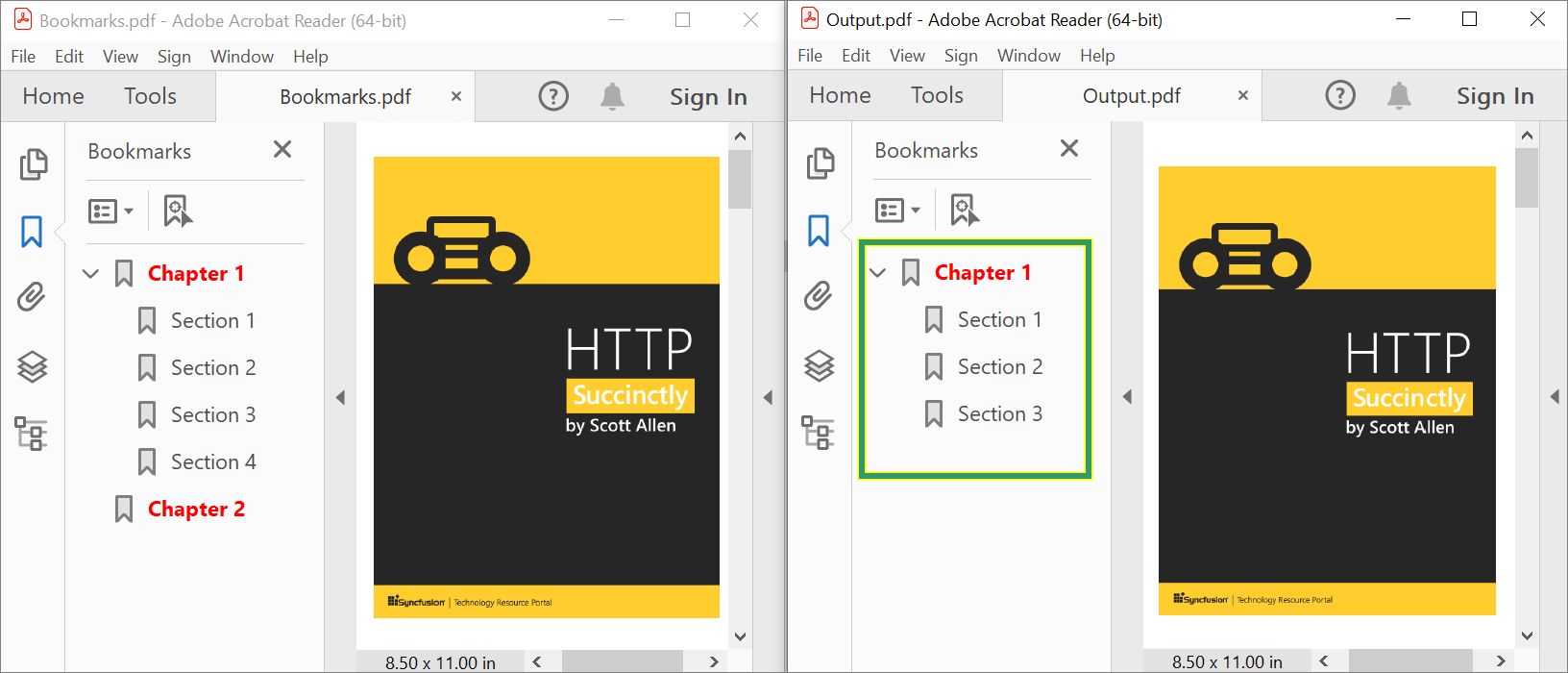
Get bookmarks and their page indices from a PDF document
You can also get the bookmarks and their page indices by following these steps:
- First, load the existing PDF document using the PdfLoadedDocument class.
- Now, retrieve the bookmark collection from the document using the PdfBookmarkBase class.
- Get the bookmark page index by accessing the PageIndex property of the PdfDestination class.
- Finally, save the modified PDF document using the Save method.
Refer to the following code example to get bookmarks and their page indices from a PDF document using C#.
//Load the PDF document. FileStream docStream = new FileStream("Input.pdf", FileMode.Open, FileAccess.Read); PdfLoadedDocument document = new PdfLoadedDocument(docStream); //Gets all the bookmarks. PdfBookmarkBase bookmark = document.Bookmarks; //Get the bookmark page index. int index = bookmark[0].Destination.PageIndex; //Close the document. document.Close(true);
By executing this code example, you will get output like in the following screenshot.
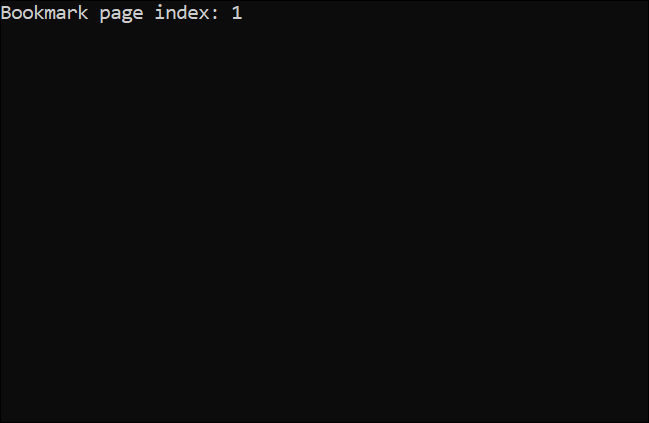
GitHub reference
For more details, refer to the examples in this GitHub repository.

Syncfusion’s high-performance PDF Library allows you to create PDF documents from scratch without Adobe dependencies.
Conclusion
Thanks for reading! In this blog, we’ve seen how to create, update, and remove bookmarks in PDF documents using Syncfusion’s .NET PDF Library. Please let us know in the comments below if you have any questions about these features.
Take a moment to look at the documentation, where you will find other options and features, all with accompanying code samples.
You can also contact us through our support forum, support portal, or feedback portal. We are always happy to assist you!