Syncfusion Blazor Scheduler lets you synchronize and manage calendars like Google calendar and Outlook calendar in one place. This will help you avoid conflicts among the multiple calendars (like personal and professional appointments) and manage time efficiently. In this article, we will walk you through the steps to implement two-way synchronization between Syncfusion Blazor Scheduler and Google Calendar. This means that if we make changes in either calendar, it will be reflected in both the places. Google Calendar provides flexible APIs for synchronization.
Let’s get started!
Project setup
Refer to the Getting Started with Blazor Scheduler Component UG documentation to create a simple Blazor Scheduler in a Blazor server-side application.
Configuring Google service
To add the required NuGet packages to the project, right-click on Dependencies and select Manage NuGet Packages.
In the Browse tab, find and install the Google.Apis.Calendar.v3 NuGet package.
Enable Google Calendar API
Refer to the Quick Start documentation to turn on the Google Calendar API, download the credentials.json, and place it in the application root directory.
Initialize service
Add the GoogleService class in the Data folder and add the following code to initialize Google Calendar service.
public class GoogleService { string[] Scopes = { CalendarService.Scope.Calendar }; string ApplicationName = "Google Calendar Sync with Blazor Scheduler"; CalendarService Service; public GoogleService() { UserCredential credential; using (FileStream stream = new FileStream("credentials.json", FileMode.Open, FileAccess.Read)) { credential = GoogleWebAuthorizationBroker.AuthorizeAsync(GoogleClientSecrets.Load(stream).Secrets, Scopes, "admin", CancellationToken.None, new FileDataStore("token.json", true)).Result; } Service = new CalendarService(new BaseClientService.Initializer() { HttpClientInitializer = credential, ApplicationName = ApplicationName }); } }
In the StartUp.cs file, register GoogleService as AddSingleton in the ConfigureServices method. Refer to the following code.
public void ConfigureServices(IServiceCollection services) { … services.AddSingleton<GoogleService>(); }
Blazor Scheduler fields
Create an Appointment class in the Data folder and add in the following code. This Appointment class will serve as the model class.
public class Appointment { public string Id { get; set; } public string Subject { get; set; } public string Location { get; set; } public string Description { get; set; } public DateTime StartTime { get; set; } public DateTime EndTime { get; set; } public string StartTimezone { get; set; } public string EndTimezone { get; set; } public bool IsAllDay { get; set; } public bool IsBlock { get; set; } public bool IsReadonly { get; set; } public string RecurrenceID { get; set; } public string FollowingID { get; set; } public string RecurrenceRule { get; set; } public string RecurrenceException { get; set; } }
Add Syncfusion Blazor Scheduler component
Now add the Blazor Scheduler component to the Index.razor page and set Tvalue to the Appointment model type.
<SfSchedule TValue="Appointment"> </SfSchedule>
Getting events from Google Calendar
In previous steps, we initialized the Google Calendar service and added an empty Scheduler without appointments. In the following method, we will make an API request to read Google Calendar events. Then, we will convert them to the Appointment model type for the Blazor Scheduler.
public List<Appointment> GetEvents(DateTime date) { try { EventsResource.ListRequest request = Service.Events.List("primary"); request.TimeMin = date; request.MaxResults = 2500; Events events = request.Execute(); List<Appointment> eventDatas = new List<Appointment>(); if (events.Items != null && events.Items.Count > 0) { foreach (Event eventItem in events.Items) { if (eventItem.Start == null && eventItem.Status == "cancelled") { continue; } DateTime start; DateTime end; if (string.IsNullOrEmpty(eventItem.Start.Date)) { start = (DateTime)eventItem.Start.DateTime; end = (DateTime)eventItem.End.DateTime; } else { start = Convert.ToDateTime(eventItem.Start.Date); end = Convert.ToDateTime(eventItem.End.Date); } Appointment eventData = new Appointment() { Id = eventItem.Id, Subject = eventItem.Summary, StartTime = start, EndTime = end, Location = eventItem.Location, Description = eventItem.Description, IsAllDay = !string.IsNullOrEmpty(eventItem.Start.Date) }; eventDatas.Add(eventData); } } return eventDatas; } catch (Exception ex) { Console.WriteLine(ex.Message); return new List<Appointment>(); } }
Refer to the Google Calendar event fields to learn more about it.
Refer to the Syncfusion UG documentation to learn more about the Blazor Scheduler fields.
Binding events to Blazor Scheduler
Inject the GoogleService in the Index.razor file to get Google Calendar events from that service. Assign the resultant value as the DataSource in the Syncfusion Blazor Scheduler.
Refer to the following code example.
@inject GoogleService GoogleService <SfSchedule TValue="Appointment" Width="100%" Height="650px" @bind-SelectedDate="SelectedDate"> <ScheduleEventSettings DataSource="@DataSource"></ScheduleEventSettings> </SfSchedule> @code{ private DateTime SelectedDate; private List<Appointment> DataSource; protected async override Task OnInitializedAsync() { await base.OnInitializedAsync(); SelectedDate = new DateTime(2020, 11, 1); DataSource = GoogleService.GetEvents(SelectedDate); } }
Perform CRUD actions in Blazor Scheduler
Use the ActionCompleted event to capture the insert, update, and delete actions in the Blazor Scheduler. This event will provide the complete details of the changes made in the Calendar during any operation. Using this information, we can perform CRUD actions in Google Calendar via GoogleService.
Refer to the following code.
@inject GoogleService GoogleService <SfSchedule TValue="Appointment" Width="100%" Height="650px" @bind-SelectedDate="SelectedDate"> <ScheduleEventSettings DataSource="@DataSource"></ScheduleEventSettings> <ScheduleEvents TValue="Appointment" ActionCompleted="OnActionCompleted"></ScheduleEvents> </SfSchedule> @code{ private DateTime SelectedDate; private List<Appointment> DataSource; protected async override Task OnInitializedAsync() { await base.OnInitializedAsync(); SelectedDate = new DateTime(2020, 11, 1); DataSource = GoogleService.GetEvents(SelectedDate); } private void OnActionCompleted(ActionEventArgs<Appointment> args) { if (args.ActionType == ActionType.EventCreate || args.ActionType == ActionType.EventChange || args.ActionType == ActionType.EventRemove) { if (args.AddedRecords != null && args.AddedRecords.Count > 0) { foreach (Appointment eventData in args.AddedRecords) { GoogleService.InsertEvent(eventData); } } if (args.ChangedRecords != null && args.ChangedRecords.Count > 0) { foreach (Appointment eventData in args.ChangedRecords) { GoogleService.UpdateEvent(eventData); } } if (args.DeletedRecords != null && args.DeletedRecords.Count > 0) { foreach (Appointment eventData in args.DeletedRecords) { GoogleService.RemoveEvent(eventData.Id); } } this.DataSource = GoogleService.GetEvents(SelectedDate); } } }
Inserting Blazor Scheduler events in Google Calendar
Add the following method in the GoogleService class to convert a Scheduler event to a Google Calendar event and insert it in the Google Calendar.
public void InsertEvent(Appointment eventData) { try { EventDateTime start; EventDateTime end; if (eventData.IsAllDay) { start = new EventDateTime() { Date = eventData.StartTime.ToString("yyyy-MM-dd") }; end = new EventDateTime() { Date = eventData.EndTime.ToString("yyyy-MM-dd") }; } else { start = new EventDateTime() { DateTime = eventData.StartTime, TimeZone = "UTC" }; end = new EventDateTime() { DateTime = eventData.EndTime, TimeZone = "UTC" }; } Event eventItem = new Event() { Summary = eventData.Subject, Start = start, End = end, Location = eventData.Location, Description = eventData.Description, Created = DateTime.Now.ToUniversalTime() }; EventsResource.InsertRequest addEvent = Service.Events.Insert(eventItem, "primary"); addEvent.Execute(); } catch (Exception ex) { Console.WriteLine(ex.Message); } }
Updating Blazor Scheduler events in Google Calendar
Refer to the following code to do updates in Google Calendar.
public void UpdateEvent(Appointment eventData) { try { var editedEvent = Service.Events.Get("primary", eventData.Id).Execute(); editedEvent.Summary = eventData.Subject; editedEvent.Location = eventData.Location; editedEvent.Description = eventData.Description; editedEvent.Updated = DateTime.Now.ToUniversalTime(); if (eventData.IsAllDay) { editedEvent.Start = new EventDateTime() { Date = eventData.StartTime.ToString("yyyy-MM-dd") }; editedEvent.End = new EventDateTime() { Date = eventData.EndTime.ToString("yyyy-MM-dd") }; } else { editedEvent.Start = new EventDateTime() { DateTime = eventData.StartTime }; editedEvent.End = new EventDateTime() { DateTime = eventData.EndTime }; } var updatedEvent = Service.Events.Update(editedEvent, "primary", editedEvent.Id); updatedEvent.Execute(); } catch (Exception ex) { Console.WriteLine(ex.Message); } }
Removing Blazor Scheduler events in Google Calendar
Refer to the following code to remove events in Google Calendar.
public void RemoveEvent(string id) { try { EventsResource.DeleteRequest deleteEvent = Service.Events.Delete("primary", id); deleteEvent.Execute(); } catch (Exception ex) { Console.WriteLine(ex.Message); } }
Output
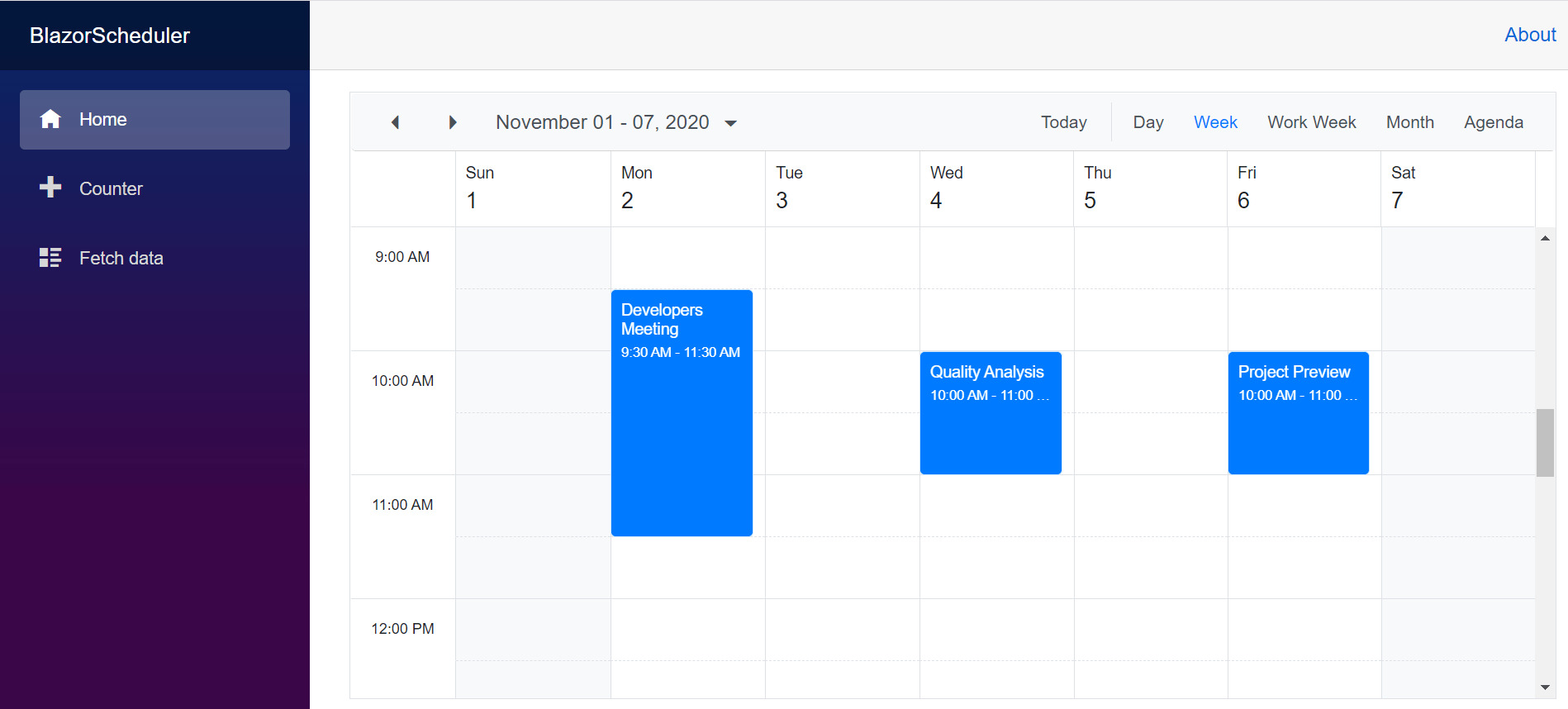
GitHub reference
For more information, you can check out Synchronize Google Calendar with Syncfusion Blazor Scheduler demo.
Summary
This blog explained in detail how to synchronize Google Calendar with Syncfusion Blazor Scheduler. Try this yourselves and leave your feedback in the comments section below.
Syncfusion Blazor offers 65+ high-performance, lightweight, and responsive UI components for the web, including file-format libraries, in a single package. Please take a look at our live demos in our sample browser for a hands-on experience.
For existing customers, the latest version is available for download from the License and Downloads page. If you are not yet a Syncfusion customer, you can try our 30-day free trial to check out the available features. You can try our samples from this GitHub location.
You can also contact us through our support forums, Direct-Trac, or feedback portal. We are always happy to assist you!
If you like this post, we think you will also like the following:
Comments (1)
I tried to run the GitHub solution (SyncfusionExamples/google-calendar-synchronization-with-blazor-scheduler) after updating to the latest NuGet packages. I have Enabled Google Calendar API and added https://127.0.0.1:5001/ and https://localhost:5001/ as redirect_uris. I keep getting the error………
Error 400: redirect_uri_mismatch
You can’t sign in to this app because it doesn’t comply with Google’s OAuth 2.0 policy.
If you’re the app developer, register the redirect URI in the Google Cloud Console.
Request details: redirect_uri=http://127.0.0.1:55669/authorize/
Do you have any ideas on how to make this work?
Comments are closed.