The Syncfusion .NET PDF Library provides support for rotating the elements in a PDF document by setting the rotation angle of the elements.
This article will cover the process of rotating a page, text, table, and an image in a PDF file using the Syncfusion .NET PDF Library.
Agenda:

Experience a leap in PDF technology with Syncfusion's PDF Library, shaping the future of digital document processing.
Getting started with app creation
- First, create a new C# .NET console application using Visual Studio.
- Then, install the Syncfusion.Pdf.Net.Core NuGet package as a reference in your .NET app from the NuGet Gallery.
Rotating a page in a PDF document
Rotating a PDF page means changing the orientation of the page to a specified degree.
For example, rotating a page by 90 degrees would turn it from portrait to landscape orientation or vice versa. This can be useful when a page has been scanned or saved in an inappropriate orientation or if you want to view a page from a different perspective. The rotation can be done either clockwise or counterclockwise.
The following code example shows how to rotate a page in a PDF document using C#.
//Load PDF document as a stream. FileStream inputStream = new FileStream("Input.pdf", FileMode.Open, FileAccess.Read, FileShare.ReadWrite); //Load the existing PDF document. PdfLoadedDocument loadedDocument = new PdfLoadedDocument(inputStream); for (int i = 0; i < loadedDocument.PageCount; i++) { //Gets the page. PdfPageBase loadedPage = loadedDocument.Pages[i] as PdfPageBase; //Set the rotation for the loaded page. loadedPage.Rotation = PdfPageRotateAngle.RotateAngle90; } //Create the file stream to save the PDF document. using(FileStream fileStream = new FileStream("Output.pdf", FileMode.CreateNew, FileAccess.ReadWrite)) { loadedDocument.Save(fileStream); } //Close the document. loadedDocument.Close(true);
By executing this code example, you will get a PDF document like in the following screenshot.
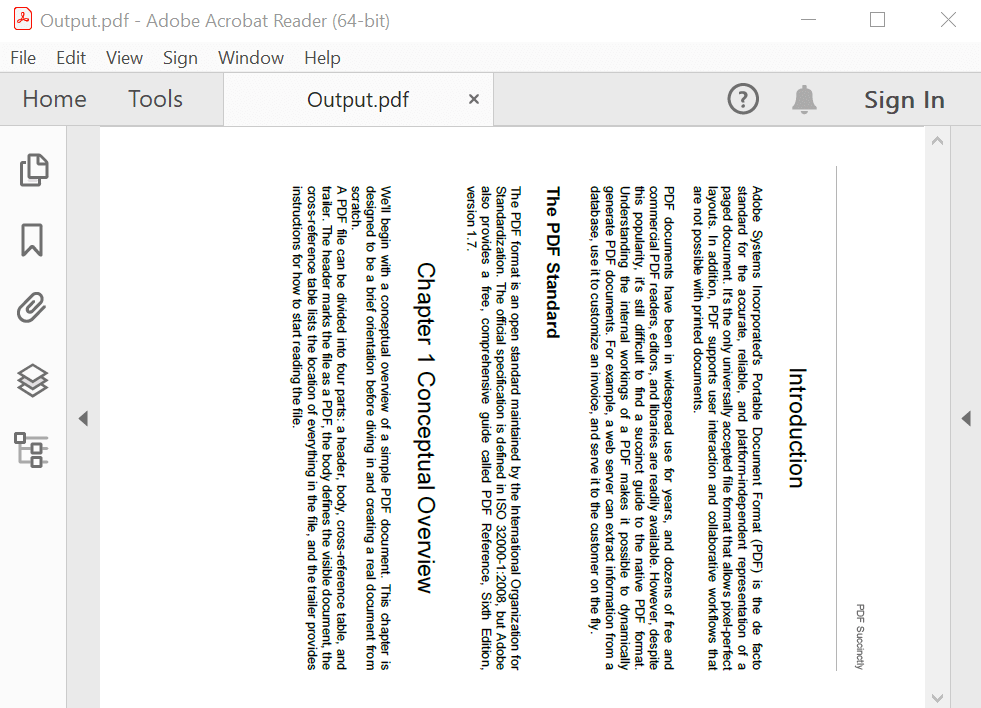

Unleash the full potential of Syncfusion's PDF Library! Explore our advanced resources and empower your apps with cutting-edge functionalities.
Rotating text in a PDF document
Rotating PDF text refers to changing the orientation of the text from its default horizontal alignment to a vertical or diagonal alignment. This can be useful in various scenarios, such as fitting more text on a page, improving the visual appeal of a document, or making the text easier to read in certain circumstances.
The following code example shows how to rotate text in a PDF document using C#.
//Create a new PDF document. PdfDocument pdfDocument = new PdfDocument(); //Add a page to the PDF document. PdfPage pdfPage = pdfDocument.Pages.Add(); //Create PDF graphics for the page. PdfGraphics graphics = pdfPage.Graphics; //Set the font. PdfFont font = new PdfStandardFont(PdfFontFamily.Helvetica, 20); //Add watermark text. PdfGraphicsState state = graphics.Save(); graphics.RotateTransform(-40); graphics.DrawString("Rotating a text in PDF document", font, PdfPens.Red, PdfBrushes.Red, new PointF(-150, 450)); //Create the file stream to save the PDF document. using (FileStream outputStream = new FileStream("Output.pdf", FileMode.CreateNew, FileAccess.ReadWrite)) { pdfDocument.Save(outputStream); } //Close the document. pdfDocument.Close(true);
By executing this code, you will get a PDF like in the following screenshot.
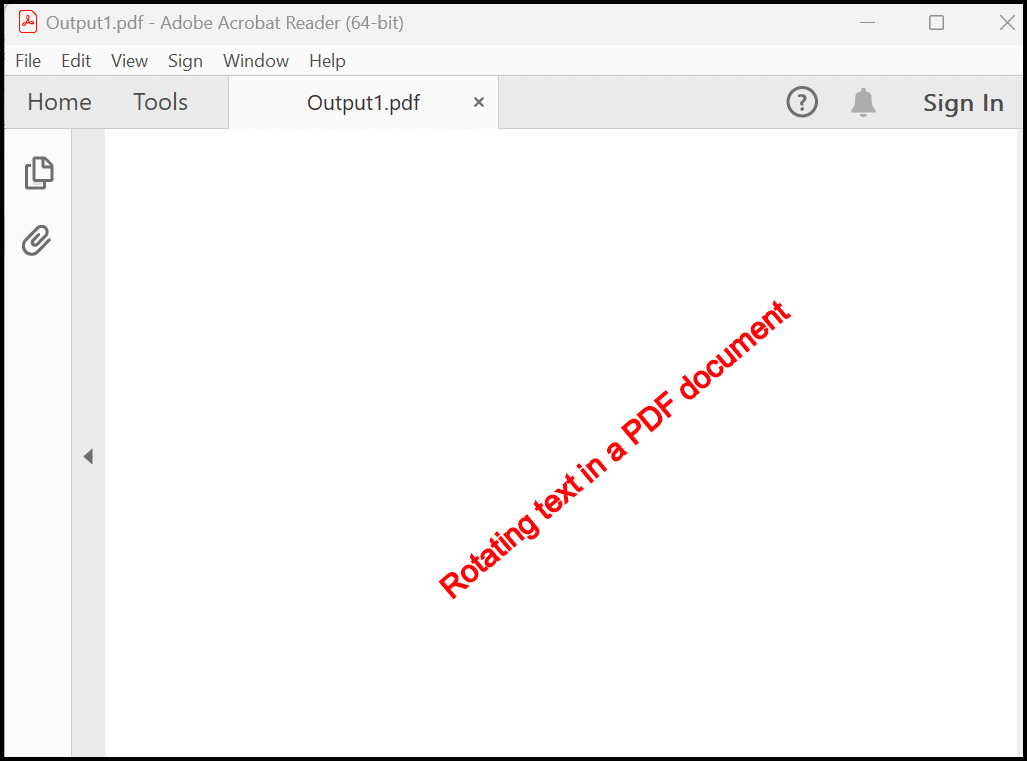
Rotating an image in a PDF document
We can also rotate an image in a PDF using the Syncfusion .NET PDF Library. This involves changing the orientation of the image embedded in the document to a specified degree.
For example, rotating an image by 90 degrees turns it from portrait to landscape orientation or vice versa. The rotation can be done either clockwise or counterclockwise.
The following code example shows how to rotate an image in a PDF document using C#.
//Created the PDF document. PdfDocument document = new PdfDocument(); //Add a new page. PdfPage page = document.Pages.Add(); FileStream imageStream = new FileStream("Input.png", FileMode.Open, FileAccess.Read, FileShare.ReadWrite); //Load a bitmap. PdfBitmap image = new PdfBitmap(imageStream); //Save the current graphics state. PdfGraphicsState state = page.Graphics.Save(); //Rotate the coordinate system. page.Graphics.RotateTransform(-40); //Draw image. image.Draw(page, -150, 450); //Restore the graphics state. page.Graphics.Restore(state); //Create the file stream to save the PDF document. using (FileStream fileStream = new FileStream("Output.pdf", FileMode.CreateNew, FileAccess.ReadWrite)) { document.Save(fileStream); } //Close the document. document.Close(true);
By executing this code example, we will get a PDF document like in the following screenshot.
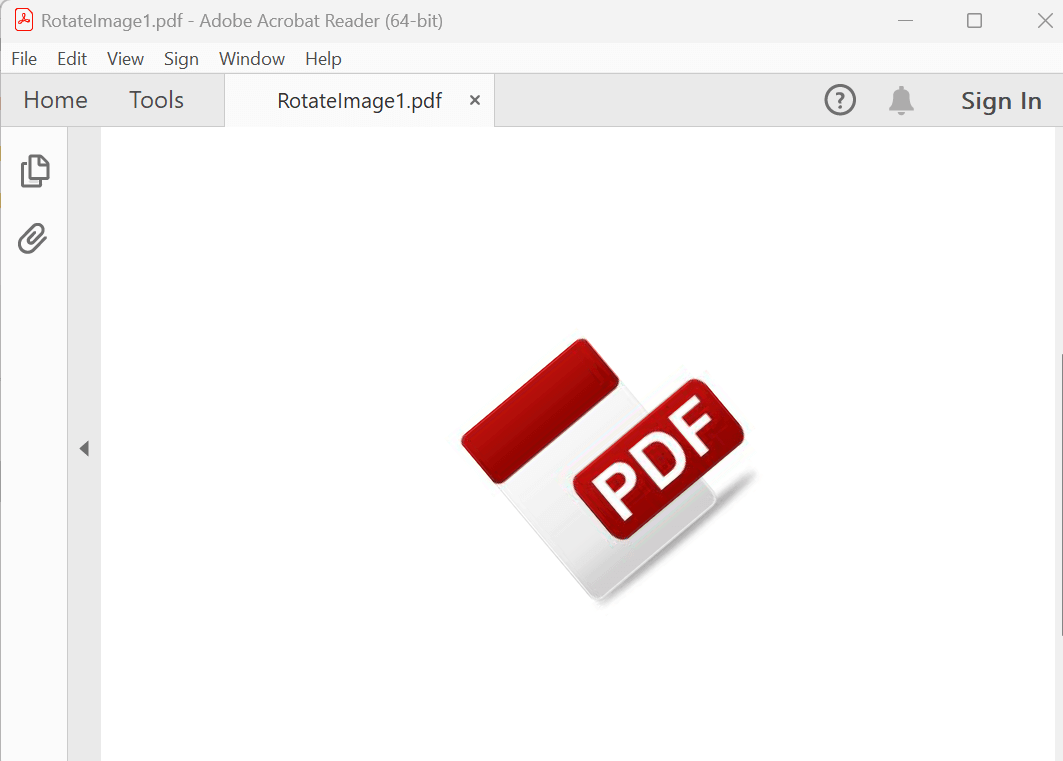

Embark on a virtual tour of Syncfusion's PDF Library through interactive demos.
Rotating a table in a PDF document
By rotating a table in a PDF, we can change the orientation from its original position to a different angle. This can be useful when the table’s original orientation is not convenient for viewing or when the table’s data needs to be presented in a different orientation.
The following code example shows how to rotate a table in a PDF document using C#.
//Create a new PDF document. PdfDocument document = new PdfDocument(); //Add a page. PdfPage page = document.Pages.Add(); //Create a PdfGrid. PdfGrid pdfGrid = new PdfGrid(); //Add a handler to rotate the table. pdfGrid.BeginPageLayout += PdfGrid_BeginPageLayout; //Create a DataTable. DataTable dataTable = new DataTable(); //Add columns to the DataTable. dataTable.Columns.Add("ID", typeof(int)); dataTable.Columns.Add("Name", typeof(string)); //Add rows to the DataTable. for (int i = 0; i < 10; i++) { dataTable.Rows.Add(57, "AAA"); dataTable.Rows.Add(130, "BBB"); } //Assign data source. pdfGrid.DataSource = dataTable; //Set a repeat header for the table. pdfGrid.RepeatHeader = true; //Draw a grid to the page of a PDF document. pdfGrid.Draw(page, new RectangleF(100, 20, 0, page.GetClientSize().Width)); //Create the file stream to save the PDF document. using (FileStream fileStream = new FileStream("Output.pdf", FileMode.CreateNew, FileAccess.ReadWrite)) { document.Save(fileStream); } //Close the document. document.Close(true); void PdfGrid_BeginPageLayout(object sender, Syncfusion.Pdf.Graphics.BeginPageLayoutEventArgs e) { PdfPage page = e.Page; PdfGraphics graphics = e.Page.Graphics; //Translate the coordinate system to the required position. graphics.TranslateTransform(page.GetClientSize().Width, 0); //Rotate the coordinate system. graphics.RotateTransform(90); }
By executing this code example, we will get a PDF document like in the following screenshot.
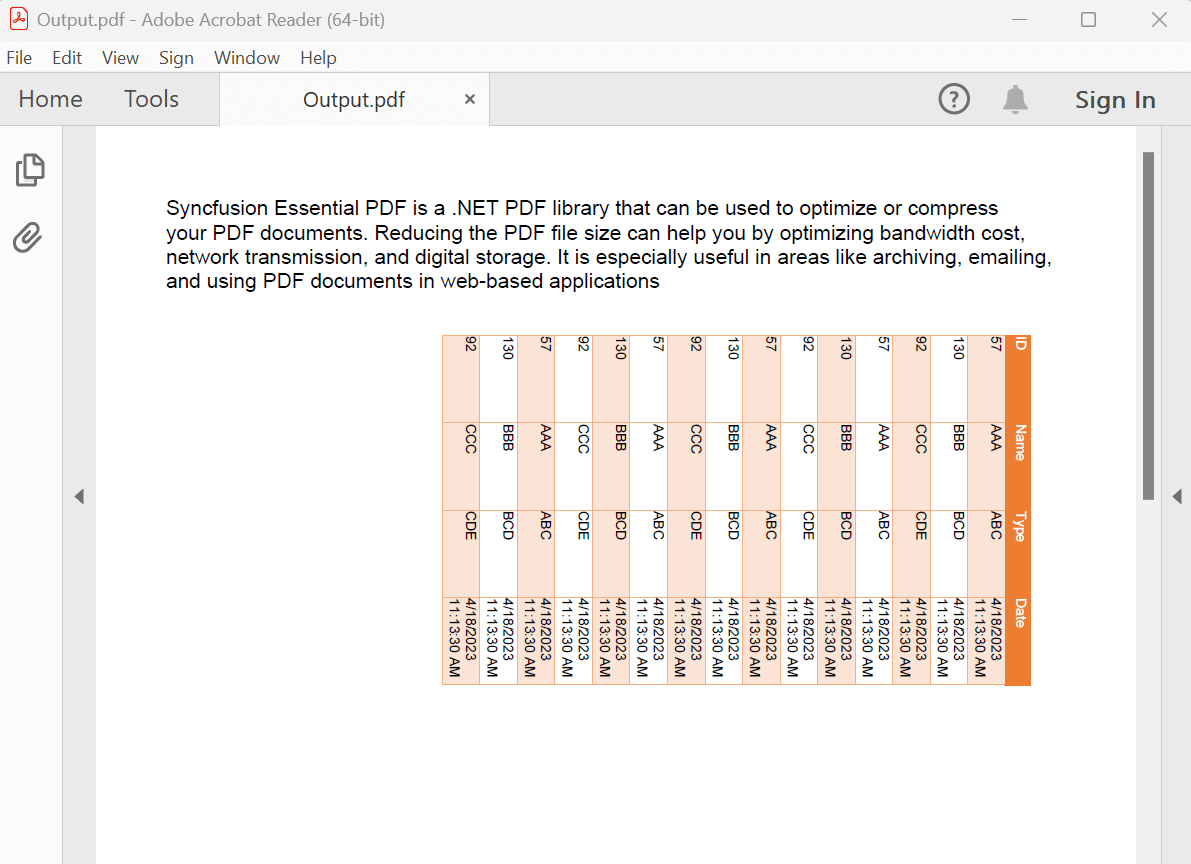
GitHub reference
For more details, check out the examples in the GitHub repository.

Syncfusion’s high-performance PDF Library allows you to create PDF documents from scratch without Adobe dependencies.
Conclusion
Thanks for reading! In this blog, we have learned how to rotate pages, text, tables, and images in a PDF using the Syncfusion .NET PDF Library. Try out these methods and leave your feedback in the comments section of this blog post!
Take a moment to look at the documentation, where you will find other options and features, all with accompanying code samples.
For existing customers, the new version of Essential Studio is available for download from the License and Downloads page. If you are not yet a Syncfusion customer, you can try our 30-day free trial to check out our available features.
For questions, you can contact us through our support forum, support portal, or feedback portal. We are delighted to assist you!