Modern JavaScript frameworks have entirely changed the way web development is done. They have provided a great abstraction by addressing common issues in browsers and enhancing performance using methodologies that were not possible through plain JavaScript.
React, one of the most popular JavaScript frameworks, is a great example. It uses something called a Virtual DOM under the hood to drastically increase performance. You have likely heard this term many times but may not truly understand how it works.
This article will discuss the virtual DOM and how it works. But to understand the virtual DOM, first, we must know how a page renders in a browser.

Syncfusion React UI components are the developers’ choice to build user-friendly web applications. You deserve them too.
How does a page render in a browser?
When a browser loads any webpage, the page is loaded in multiple parts, then it is constructed in the browser and rendered.
The start-to-end process of rendering a webpage looks like this:
- The HTTP protocol returns data in bytes.
- These bytes are converted into characters, then tokens, then nodes, and then, lastly, the object model.
- The DOM (Document Object Model) is a tree-like structure that represents HTML. It is generated from a webpage’s HTML markup. Similarly, the CSSOM (CSS Object Model) is generated from a webpage’s CSS markup. The DOM and CSSOM are loaded independently as trees, and then they are combined into a render tree to form a webpage.
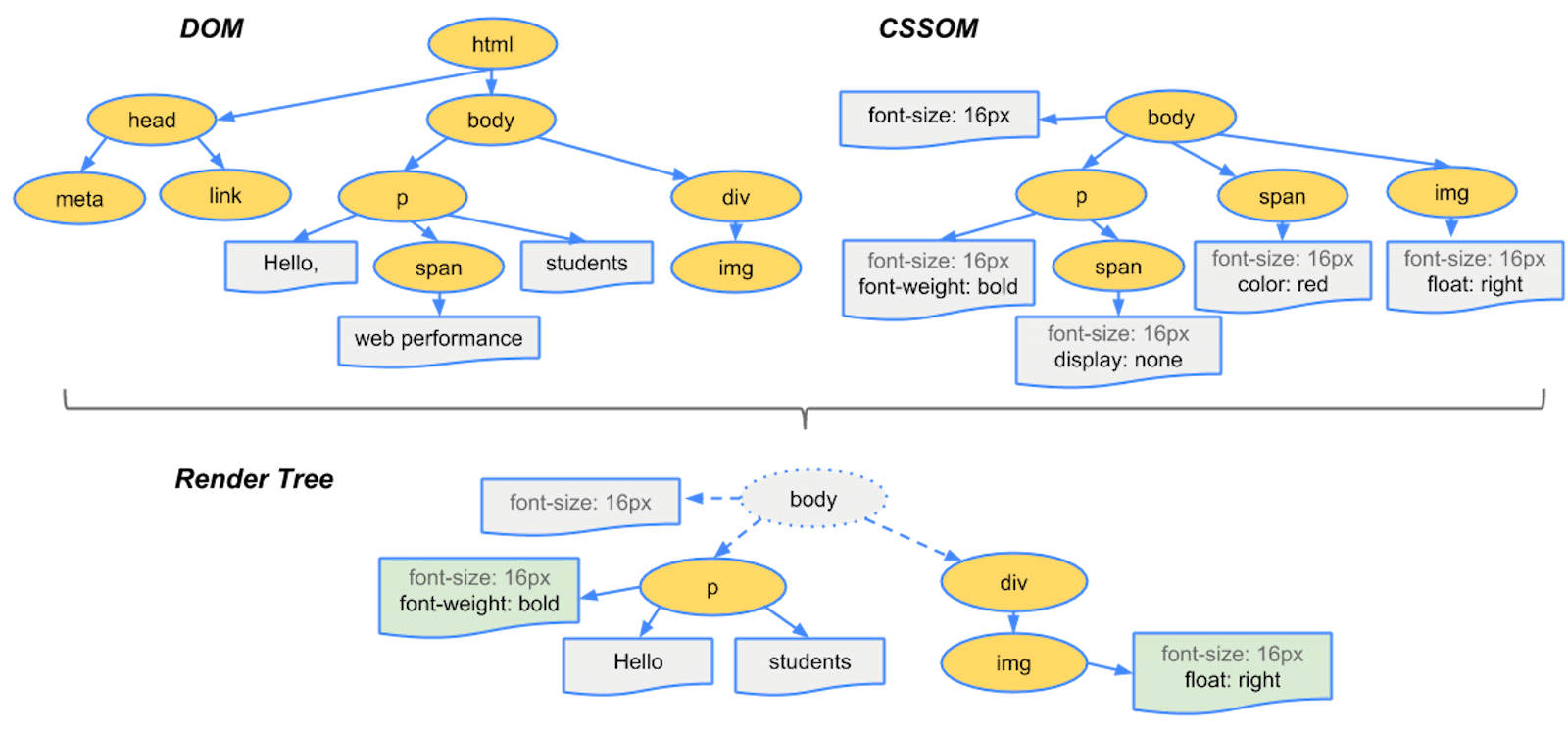
To construct the render tree, the browser processes each node in the DOM, looks for the node’s corresponding style in the CSSOM, and applies the style to the node. Once the final tree is formed, the page is laid out so that each node is placed in its calculated position on the screen.
Every time an HTML element is updated, a complete re-rendering process takes place.
The entire process of rendering a webpage is known as the critical rendering path. It is one of the most important considerations for SEO and web performance.
Now that we understand how a webpage is rendered in the browser, imagine a modern web application. Behind the scenes, fast DOM manipulation is happening, and the resulting frequent re-rendering heavily impacts performance. We need to find some way to address this. This is where the virtual DOM comes into play.

All Syncfusion’s 80+ React UI components are well-documented. Refer to them to get started quickly.
What is the virtual DOM, and how does it work?
One way to optimize webpage performance is by making the minimum changes possible to the DOM as infrequently as possible so that updates can be rendered quickly.
This is what the virtual DOM tries to do.
The virtual DOM is a representation of the original DOM through a JavaScript object. It is a concept that React has popularized and uses within its library to improve re-rendering performance whenever the DOM is updated. Whenever the DOM updates in React after a state change, the changes are first made to the virtual DOM.
The virtual DOM creates a snapshot (the existing state) of itself before the update. Then it compares the changes with the snapshot to determine what exactly has changed using a diffing algorithm called reconciliation.
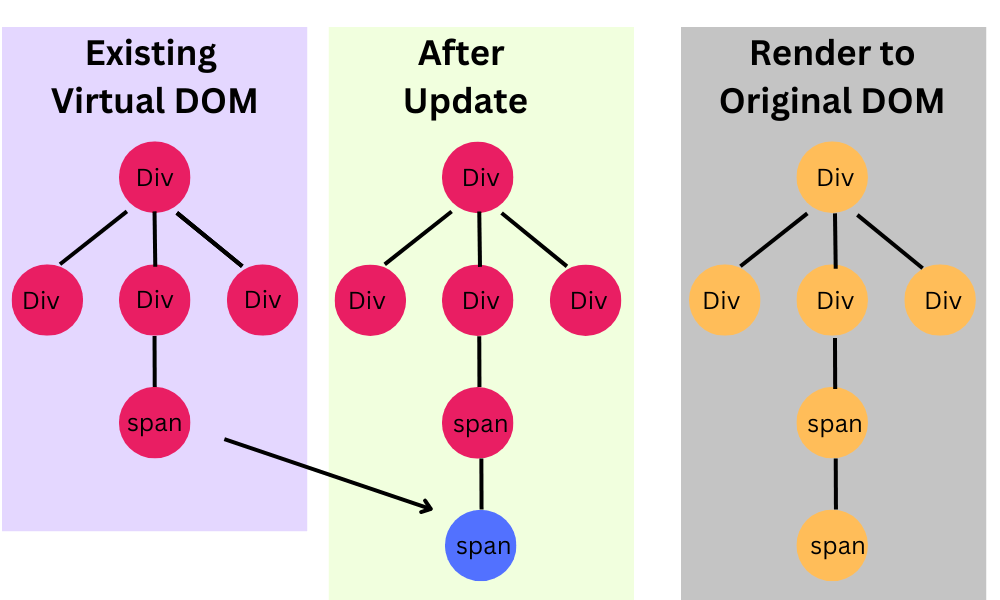
The reconciliation algorithm depends on an identifier to determine the uniqueness of each DOM element in the list. This identifier helps perform the comparison in O(n) time when it would otherwise take O(n3) time.
After reconciliation, with the help of the ReactDOM.render() method, React renders the DOM update to the original DOM. The virtual DOM helps eliminate unnecessary re-renders of DOM elements.
Each component in React can have its state, and state updates are batched in React. This helps group multiple updates in the virtual DOM so that the frequency of DOM updates can be reduced.
Let’s attain a better understanding of this with the help of the following example:
import React, { useState, useRef, useEffect } from 'https://esm.sh/react@18.2.0' import ReactDOM from 'https://esm.sh/react-dom@18.2.0' const App = () => { const [count, setCount] = useState(0); const timerIdRef = useRef(null); const onStart = () => { timerIdRef.current = setInterval(() => { setCount((prevCount) => prevCount + 1); }, 1000); }; const onStop = () => { clearInterval(timerIdRef.current); }; return( <div className="box"> <h1>Count: {count}</h1> <button onClick={onStart}>Start</button> <button onClick={onStop}>Stop</button> </div> ); } ReactDOM.render(<App />, document.getElementById("root"));
In the previous code, when the Start button is clicked, a timer starts that automatically updates the state at an interval of one second.
Consider the following DOM elements:
<div className="box"> <h1>Count: {count}</h1> <button onClick={onStart}>Start</button> <button onClick={onStop}>Stop</button> </div>
When the state changes for the count, the virtual DOM detects the difference, and only <h1>Count: {count}</h1> is re-rendered.
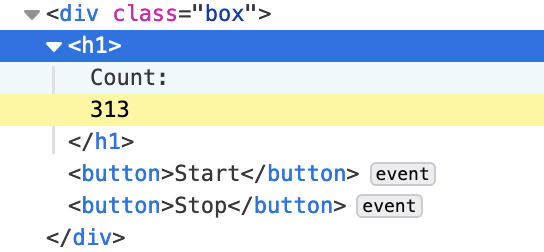

Be amazed exploring what kind of application you can develop using Syncfusion React components.
The virtual DOM is not a shadow DOM
Though both terms are used interchangeably often, they are completely different.
Shadow DOM is a concept that browsers use to abstract or hide the inner implementation of certain DOM elements.
For example, if you render <input type= “range”/> in the browser, you see a progress bar.
This progress bar is generated with a combination of HTML elements, but it is hidden from the end user by the browser. When you inspect the DOM, you will see <input type= “range”/> only.

However, if you enable the shadow DOM visibility in the browser, you will be able to see all the hidden elements.
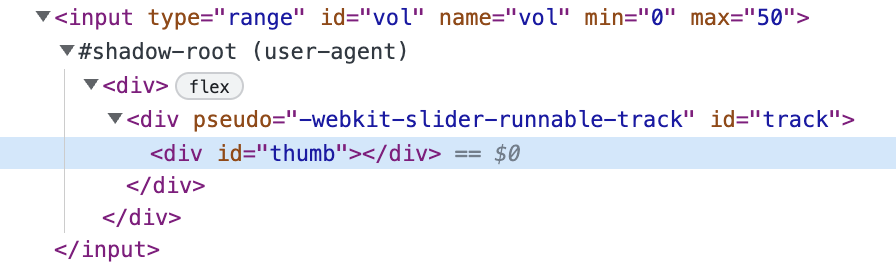
Conclusion
Virtual DOM is a powerful concept that React uses to overcome standard performance issues in the browser. It is not a perfect solution, and many other frameworks are working on improving it. In time, the original DOM in the browser is getting faster and will eliminate the need for virtual DOM altogether.
Thank you for reading!
Syncfusion’s Essential Studio for React offers over 80 high-performance, lightweight, modular, and responsive UI components in a single package. It’s the only suite to construct a complete web app.
If you have questions, contact us through our support forum, support portal, or feedback portal. We are always happy to assist you!
Comments (2)
Hello Prashant,
Thanks for explaining virtual-dom concept also for throwing light on its related concepts. I have a question for you. Although we know that virtual-dom executes the diffing and reconciliation process to update the real-dom, I want to know first of all how does the virtual-dom knows that something has been changed/updated inside a given component? Or who informs react.js that changes/updates have been triggered inside a component and virtual-dom needs to work on it? I am asking because this is a question that is often asked during interviews. I also couldn’t get a convincing answer on the web. If you can explain/add this process in the above article, it would be great! Thank you.
Hi Abhijit,
Component updates and re-rendering are two different things.
The React framework is designed to trigger a re-render in the component when its props or state changes, this is done by updating the component with the new props and state changes and then comparing it with the previous snapshot to decide what to re-render. This means the re-render is done after the changes are made.
The re-render takes place in the JSX part after comparison, but the component is updated before that.
They use a Graph data structure and check the updates with its snapshot to determine changes, similar to how GIT tracks what has changed in the files.
Comments are closed.