The Blazor AutoComplete is a text box component that provides a list of suggestions to select from as the user types. It has several out-of-the-box features, such as data binding, filtering, grouping, UI customization, and accessibility. You can customize the suggestion list, filter type, minimum length, autofill feature, and more. You can also define your own data source for the suggestion list.
In this blog, we’ll explore how to implement Google-like search suggestions in the Blazor AutoComplete component. This involves conducting a Google search for each keypress in the Blazor AutoComplete and displaying the relevant Google search results in the suggestion list.
Let’s get started!
Prerequisites
Procedure
Follow these steps to implement Google search suggestions in the Blazor AutoComplete control:
Step 1: Create a Blazor server app
Create a new Blazor Server app using Visual Studio, install the Syncfusion Blazor packages, and configure the style and script references using the getting started documentation.
Step 2: Add the AutoComplete component to the app
Add the Blazor AutoComplete control with the field settings configurations to the Index.razor file.
Refer to the following code example.
<SfAutoComplete TValue="string" Placeholder="Search something" Titem="GoogleSearch" Autofill=true> <AutoCompleteFieldSettings Value="SuggestionText" /> </SfAutoComplete> @code { class GoogleSearch { public string SuggestionText { get; set; } = String.Empty; } }
Step 3: Add the Filtering event in AutoComplete
Add the Filtering event to the AutoComplete component to handle custom search options within it.
<SfAutoComplete TValue="string" TItem="GoogleSearch"> <AutoCompleteFieldSettings Value="SuggestionText"/> <AutoCompleteEvents TItem="GoogleSearch" TValue="string" Filtering="@OnCustomFiltering" /> </SfAutoComplete> @code { class GoogleSearch { public string SuggestionText { get; set; } = String.Empty; } private async Task OnCustomFiltering(FilteringEventArgs args) { } }
Step 4: Get suggestions from the Google search API based on user input
When we use a search engine to look for something, the suggestions are displayed based on the characters we enter.
Let’s see how to retrieve suggestions from the Google search engine.
You can use the following URL to get the search result data based on user-typed text.
https://suggestqueries.google.com/complete/search?client=firefox&q={typed text}
Refer to the following code example to obtain suggestions from the Google search engine.
static readonly HttpClient client = new HttpClient(); private async Task OnCustomFiltering(FilteringEventArgs args) { var suggestions = new List<GoogleSearch>(); var url = "https://suggestqueries.google.com/complete/search?client=firefox&q=" + args.Text; var response = await client.GetAsync(url); response.EnsureSuccessStatusCode(); using (var stream = await response.Content.ReadAsStreamAsync()) }
Step 5: Parse the response content to a list of objects
The response content from the HttpClient request will be converted to JSON through stream conversion. The JSON data is then converted to a list of objects of the GoogleSearch class and loaded into the AutoComplete suggestion list using the FilterAsync public method.
Refer to the following code example.
@using Syncfusion.Blazor.Inputs @using Syncfusion.Blazor.DropDowns; @using System.Net; @using System.Text.Json; <SfAutoComplete @ref="AutoCompleteRef" Placeholder="Search something" TValue="string" TItem="GoogleSearch" Width="500px"> <AutoCompleteFieldSettings Value="SuggestionText" /> <AutoCompleteEvents TItem="GoogleSearch" TValue="string" Filtering="@OnCustomFiltering" /> </SfAutoComplete> @code { SfAutoComplete<string, GoogleSearch> AutoCompleteRef { get; set; } static readonly HttpClient client = new HttpClient(); class GoogleSearch { public string SuggestionText { get; set; } = String.Empty; } private async Task OnCustomFiltering(FilteringEventArgs args) { var suggestions = new List<GoogleSearch>(); var url = "https://suggestqueries.google.com/complete/search?client=firefox&q=" + args.Text; var response = await client.GetAsync(url); response.EnsureSuccessStatusCode(); using (var stream = await response.Content.ReadAsStreamAsync()) using (var reader = new StreamReader(stream)) { var result = reader.ReadToEnd(); var json = JsonDocument.Parse(result); var array = json.RootElement.EnumerateArray(); foreach (var item in array.Skip(1).Take(1).ToList()) { foreach (var suggestion in item.EnumerateArray()) { suggestions.Add(new GoogleSearch { SuggestionText = suggestion.GetString() }); } } } await AutoCompleteRef.FilterAsync(suggestions); } }
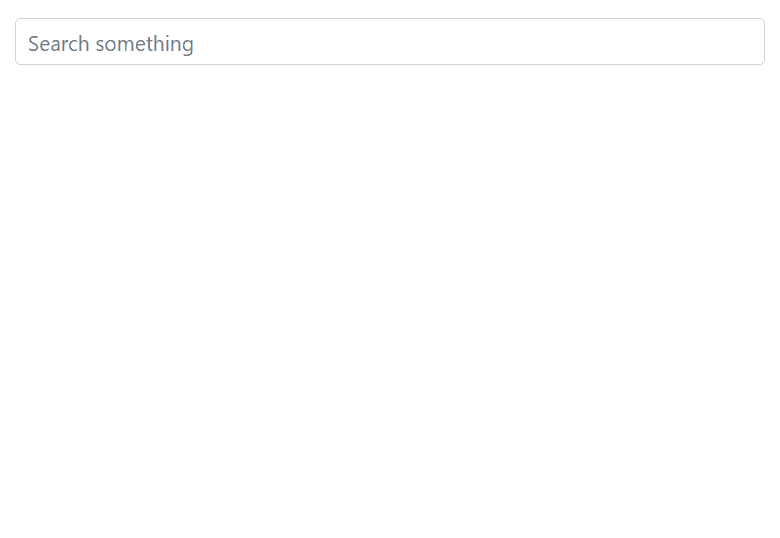
Reference
You can refer to the Google-like search suggestions in the Blazor Autocomplete demo on GitHub.
Conclusion
Thanks for reading! In this blog, we’ve seen how to implement Google-like autosuggestions in the Syncfusion Blazor AutoComplete component. Try out the steps and leave your feedback in the comments section below!
Try our Blazor components by downloading a free 30-day trial or by downloading our NuGet package. Feel free to have a look at our online examples and documentation to explore other available features.
If you want to contact us, you can reach us through our support forums, support portal, or feedback portal. We are always happy to assist you!