TLDR: Build a .NET Core console app with dependencies and convert Excel to PDF using Syncfusion’s Excel Library.
Microsoft Excel is a trusted tool for organizing, visualizing, and managing complex data. However, when you need to share data from an Excel workbook with other users, they will require Excel or an Excel file viewer to access it. To make sharing easier, you can convert Excel workbooks into the universal PDF format, allowing seamless access across various devices without the need for Excel.
The Syncfusion Excel Library, also known as Essential XlsIO, is a powerful tool for creating, reading, and editing Excel documents using C# without any Microsoft Office dependencies. Its extensive capabilities include support in creating Excel workbooks from scratch, modifying existing ones, importing and exporting data, handling formulas, applying conditional formatting, managing data validations, and much more.
Let’s see how to convert an Excel workbook to a PDF document with the help of the Syncfusion Excel Library using C#!

Enjoy a smooth experience with Syncfusion’s Excel Library! Get started with a few lines of code and without Microsoft or interop dependencies.
Excel-to-PDF conversion
Our Excel Library can convert an entire workbook or a single worksheet into a PDF document using the built-in Excel-to-PDF converter. You can customize the conversion process by embedding fonts, ignoring empty pages or worksheets, and managing the visibility of headers and footers. Conversion is fast, reliable, and compatible with hosting environments such as AWS, Google Cloud Platform, and Microsoft Azure Web Services. The Excel Library also supports converting workbooks with charts to PDF, substituting fonts during conversion, and many useful conversion options.
Steps to convert Excel to PDF using C#
Put simply, this is the procedure:
- Create a new .NET Core console application.
- Install and configure the Excel Library.
- Add namespaces.
- Convert Excel to PDF format.
- Get an Excel document stream.
Let’s dig into these steps in detail.
Step 1: Create a new .NET Core console application
First, create a new .NET Core console application in Visual Studio, as shown in the following figure:
Step 2: Install and configure the Excel Library
Add the Syncfusion.XlsIORenderer.Net.Core NuGet package to your application.

Handle Excel files like a pro with Syncfusion’s C# Excel Library, offering well-documented APIs for each functionality.
Step 3: Add namespaces
Next, add the following namespaces to the Program.cs file.
using Syncfusion.XlsIO; using Syncfusion.XlsIORenderer; using Syncfusion.Pdf;
Step 4: Convert the Excel workbook to PDF format
After that, implement the following code in the Program.cs file to convert an Excel document stream into a PDF stream of data.
static Stream ConvertExcelToPDF(Stream inputExcelData) { MemoryStream pdfStream = new MemoryStream(); //Instantiate the spreadsheet creation engine. using (ExcelEngine excelEngine = new ExcelEngine()) { //Instantiate the Excel application object. IApplication application = excelEngine.Excel; //Set the default application version. application.DefaultVersion = ExcelVersion.Xlsx; //Load the existing Excel file into IWorkbook. IWorkbook workbook = application.Workbooks.Open(inputExcelData); //Settings for Excel to PDF conversion. XlsIORendererSettings settings = new XlsIORendererSettings(); //Set the layout option to fit all columns on one page. settings.LayoutOptions = LayoutOptions.FitAllColumnsOnOnePage; //Initialize the XlsIORenderer. XlsIORenderer renderer = new XlsIORenderer(); //Initialize the PDF document. PdfDocument pdfDocument = new PdfDocument(); //Convert the Excel document to PDF. pdfDocument = renderer.ConvertToPDF(workbook, settings); //Save the PDF file. pdfDocument.Save(pdfStream); //Close the PDF document. pdfDocument.Close(); //Close the workbook. workbook.Close(); } return pdfStream; }

Witness the possibilities in demos showcasing the robust features of Syncfusion’s C# Excel Library.
Step 5: Get Excel document stream
Finally, add the following code to the Main method to get the Excel document streamed and pass it to the ConvertExcelToPDF method in the Program.cs file.
static void Main(string[] args) { string filePath = "../../../Data/Invoice.xlsx"; Stream inputExcelData = File.OpenRead(filePath); Stream outputPDFData = ConvertExcelToPDF(inputExcelData); File.WriteAllBytes("../../../Output/Invoice.pdf", ((MemoryStream)outputPDFData).ToArray()); }
The following image shows the input Excel document:
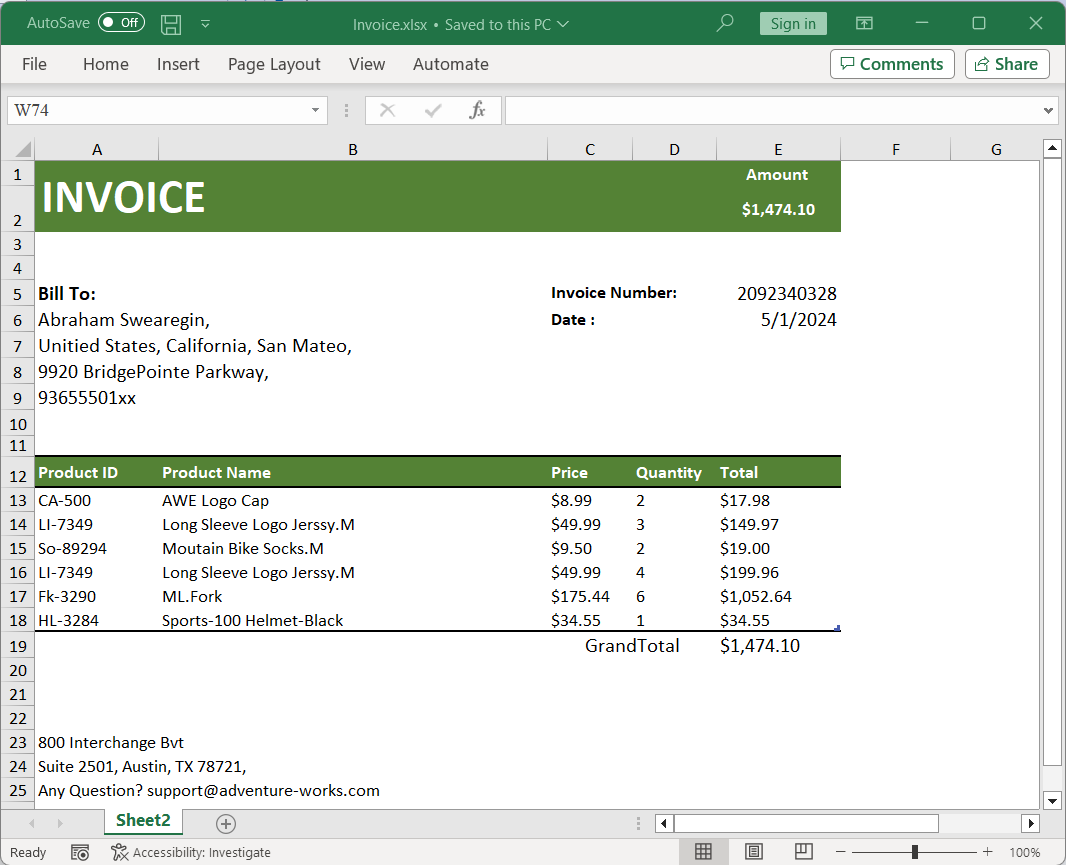
After executing the previous code example, the output PDF document will resemble the following image:
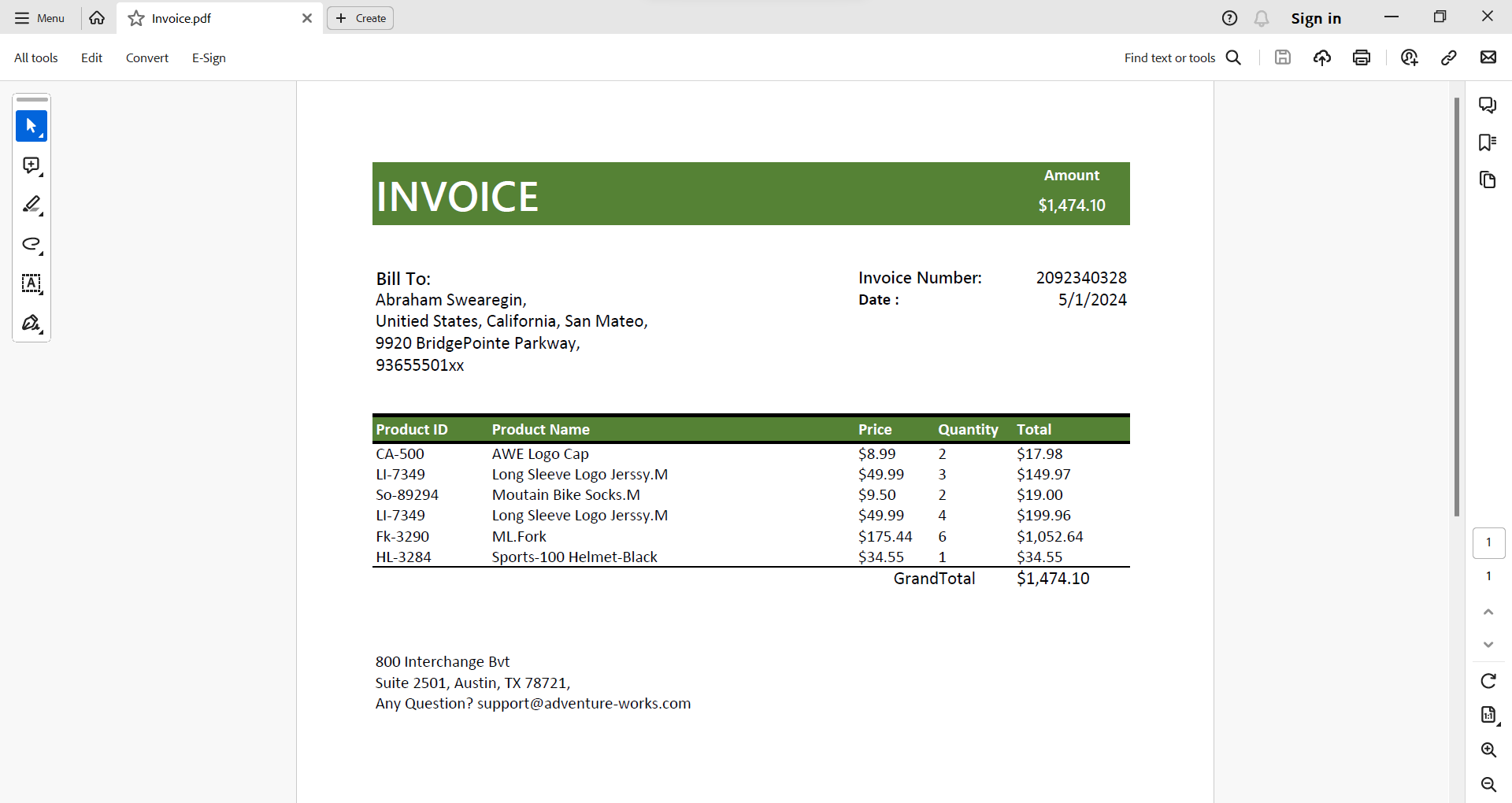
Note: For more details, explore the various Excel-to-PDF conversion settings.
Reference
For more details, refer to convert an Excel workbook to a PDF document using C# documentation and GitHub demos.

Trusted by industry giants worldwide, Syncfusion's Excel Framework has a proven track record of reliability and excellence.
Conclusion
Thanks for reading! In this blog, we explored how to convert Excel workbooks to PDF documents in C# with the Syncfusion Excel Library. With it, you can also export Excel data to images, data tables, CSV, TSV, collections of objects, ODS, JSON, and more file formats.
Take a moment to peruse the import data documentation, where you’ll discover how to import data tables, collection objects, grid view, data columns, and HTML into Excel workbooks, all accompanied by code samples.
Try out these conversions and share your feedback in the comment section of this blog post!
For current customers, the newest version of Essential Studio is available for download from the License and Downloads page. If you are not yet a Syncfusion customer, you can try our 30-day free trial to check out the enormous collection of powerful features.
If you have any questions, you can contact us through our support forum, support portal, or feedback portal. We are happy to assist you!
Related blogs
- Print Excel Documents in Just 4 Steps Using C#
- Converting XLS to XLSX Format in Just 3 Steps Using C#
- Merge Multiple Excel Files into One in Just 3 Steps Using C#
- 6 Easy Ways to Export Data to Excel in C#
- Seamlessly Import and Export CSV Data in Excel Using C#
- Easy Steps to Export HTML Tables to Excel in C#