The Syncfusion PDF Library is a .NET PDF library that allows the users to create and validate PDF digital signatures in C# and VB.NET.
A PDF digital signature is basically a secure way to ensure the following:
- Integrity of the document: Ensures that the document has not been altered somewhere in the workflow.
- Authenticity of the document: Assures the authenticity of the person who signed the electronic document.
- Nonrepudiation: The signatory cannot deny authorship.
The complete details to create and validate PDF digital signatures in C# are explained in the following topics in this post:
- Create PDF digital signatures
- Create PDF digital signatures with custom appearances
- Change the signature appearance based on the signature validation
- Create PDF digital signatures with CAdES and different hashing algorithms
- Add multiple PDF digital signatures in a single PDF document
- Digitally sign a PDF document using Windows certificate store
- Include an author or certifying signature in a PDF document
- Digitally sign a PDF document with an external signature
- Digitally sign the existing signature field in a PDF document
- Add a timestamp to a digital signature
- Digitally sign a PDF document with long-term validation (LTV)
- Digitally sign a PDF document with long-term archive timestamps (LTA)
- Create LTV in external signature
- Retrieve digital signature information from a PDF document
- Remove existing digital signatures from a PDF document
- Validate a PDF digital signature

Transform your PDF files effortlessly in C# with just five lines of code using Syncfusion's comprehensive PDF Library!
Create PDF digital signatures
To create a PDF digital signature, you need a digital ID. You can create a self-signed digital ID using Adobe Reader. The digital ID contains a private key and certificates with a public key.
You can follow these steps to sign an existing PDF document using Syncfusion PDF Library:
- Load the existing PDF document.
- Load the digital ID with a password.
- Create a signature with the loaded digital ID (this involves signing the PDF, as well).
- Save the PDF document.
The following code example shows how to create PDF digital signatures in C#.
using Syncfusion.Pdf.Parsing; using Syncfusion.Pdf.Security; class Program { static void Main(string[] args) { //Load existing PDF document. PdfLoadedDocument document = new PdfLoadedDocument("PDF_Succinctly.pdf"); //Load digital ID with password. PdfCertificate certificate = new PdfCertificate(@"DigitalSignatureTest.pfx", "DigitalPass123"); //Create a signature with loaded digital ID. PdfSignature signature = new PdfSignature(document, document.Pages[0], certificate, "DigitalSignature"); //Save the PDF document. document.Save("SignedDocument.pdf"); //Close the document. document.Close(true); } }
By executing this code example, you will get a PDF document similar to the following screenshot.
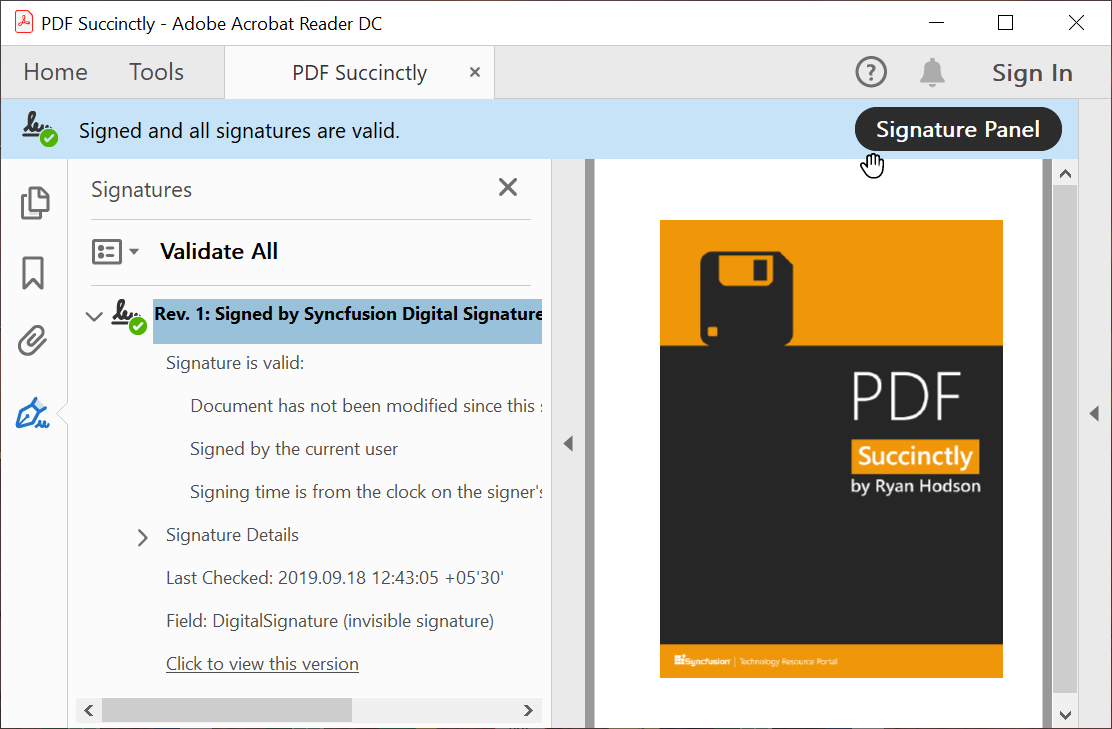
To get a valid green tick in your Adobe Acrobat Reader, as seen in the previous screenshot, you will have to register the self-signed digital ID in a trusted source.
Otherwise, to get a valid signature in any Adobe Acrobat Reader, your digital ID should be an AATL-enabled signing credential.
Create PDF digital signatures with custom appearances
PDF digital signatures with a custom appearance help users easily identify the digital signatures on a PDF page. You can create your own appearance, such as by drawing the signature, using signer information, etc.
To create a visible digital signature, you should set the bounds to the signature. You can customize its appearance using the Appearance property available in the class PdfSignature. You can draw any shape, text, or image for the signature appearance.
The following code example shows how to create PDF digital signatures in C# with a custom appearance.
using Syncfusion.Pdf.Graphics; using Syncfusion.Pdf.Parsing; using Syncfusion.Pdf.Security; class Program { static void Main(string[] args) { //Load existing PDF document. PdfLoadedDocument document = new PdfLoadedDocument("PDF_Succinctly.pdf"); //Load digital ID with password. PdfCertificate certificate = new PdfCertificate(@"DigitalSignatureTest.pfx", "DigitalPass123"); //Create a signature with loaded digital ID. PdfSignature signature = new PdfSignature(document, document.Pages[0], certificate, "DigitalSignature"); //Set bounds to the signature. signature.Bounds = new System.Drawing.RectangleF(40, 40, 350, 100); //Load image from file. PdfImage image = PdfImage.FromFile("signature.png"); //Create a font to draw text. PdfStandardFont font = new PdfStandardFont(PdfFontFamily.Helvetica, 15); //Drawing text, shape, and image into the signature appearance. signature.Appearance.Normal.Graphics.DrawRectangle(PdfPens.Black,PdfBrushes.White, new System.Drawing.RectangleF(50, 0, 300, 100)); signature.Appearance.Normal.Graphics.DrawImage(image, 0, 0,100,100); signature.Appearance.Normal.Graphics.DrawString("Digitally Signed by Syncfusion", font,PdfBrushes.Black, 120, 17); signature.Appearance.Normal.Graphics.DrawString("Reason: Testing signature", font, PdfBrushes.Black, 120, 39); signature.Appearance.Normal.Graphics.DrawString("Location: USA", font, PdfBrushes.Black, 120, 60); //Save the PDF document. document.Save("SignedAppearance.pdf"); //Close the document. document.Close(true); } }
By executing this code example, you will get a PDF document similar to the following screenshot.
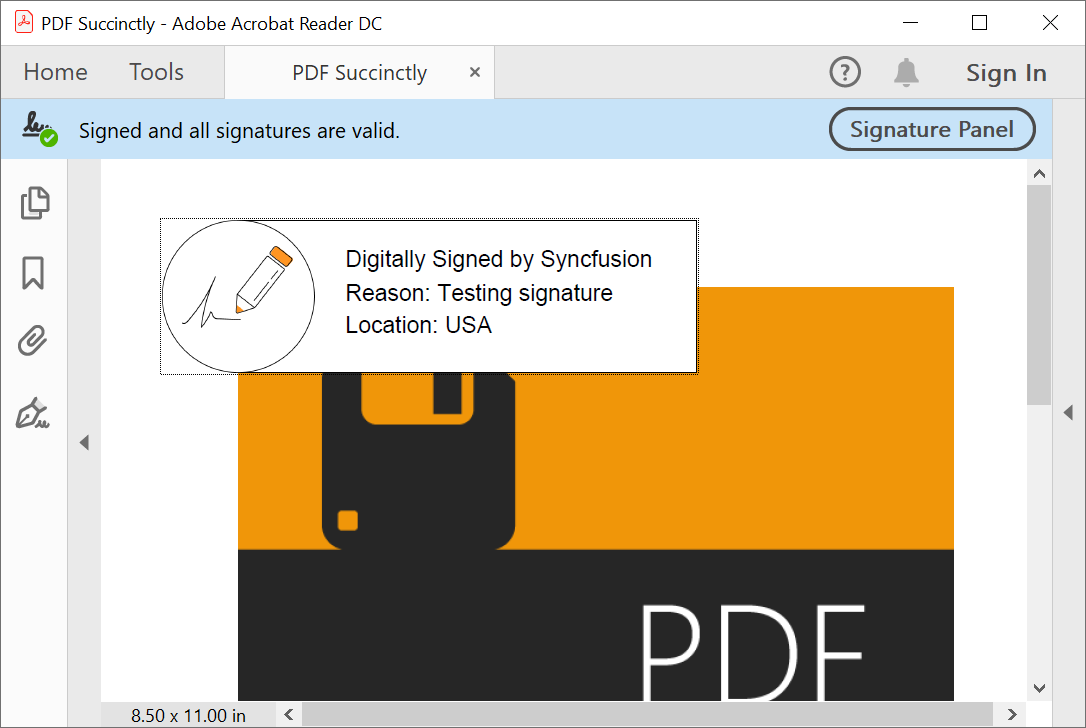

Experience a leap in PDF technology with Syncfusion's PDF Library, shaping the future of digital document processing.
Change the signature appearance based on the signature validation
You can add a dynamic signature-validation mark to the signature field by enabling the EnableValidationAppearance property available in the PdfSignature class. The appearance will change based on the PDF reader validation. So, it will give a visual indication of the signature validation after opening the PDF document.
Based on the PDF reader validation, the following three icons can be shown in the signature appearance:
- Green tick—Valid digital signature.
- Red X mark—Invalid signature.
- Yellow question mark—Signature is unknown or not validated.
The following code example shows how to sign a PDF document with signature validation.
//Load existing PDF document. PdfLoadedDocument document = new PdfLoadedDocument("PDF_Succinctly.pdf"); //Load digital ID with password. PdfCertificate certificate = new PdfCertificate(@"DigitalSignatureTest.pfx", "DigitalPass123"); //Create a signature with loaded digital ID. PdfSignature signature = new PdfSignature(document, document.Pages[0], certificate, "DigitalSignature"); signature.EnableValidationAppearance = true; //Set bounds to the signature. signature.Bounds = new System.Drawing.RectangleF(40, 30, 350, 100); //Load image from file. PdfImage image = PdfImage.FromFile("signature.png"); //Create a font to draw text. PdfStandardFont font = new PdfStandardFont(PdfFontFamily.Helvetica, 15); signature.Appearance.Normal.Graphics.DrawImage(image, 0, 0, 100, 100); signature.Appearance.Normal.Graphics.DrawString("Digitally Signed by Syncfusion", font, PdfBrushes.Black, 120, 17); signature.Appearance.Normal.Graphics.DrawString("Reason: Testing signature", font, PdfBrushes.Black, 120, 39); signature.Appearance.Normal.Graphics.DrawString("Location: USA", font, PdfBrushes.Black, 120, 60); //Save the PDF document. document.Save("SignedAppearance.pdf"); //Close the document. document.Close(true);
By executing this code example, you will get a PDF document similar to the following screenshot.
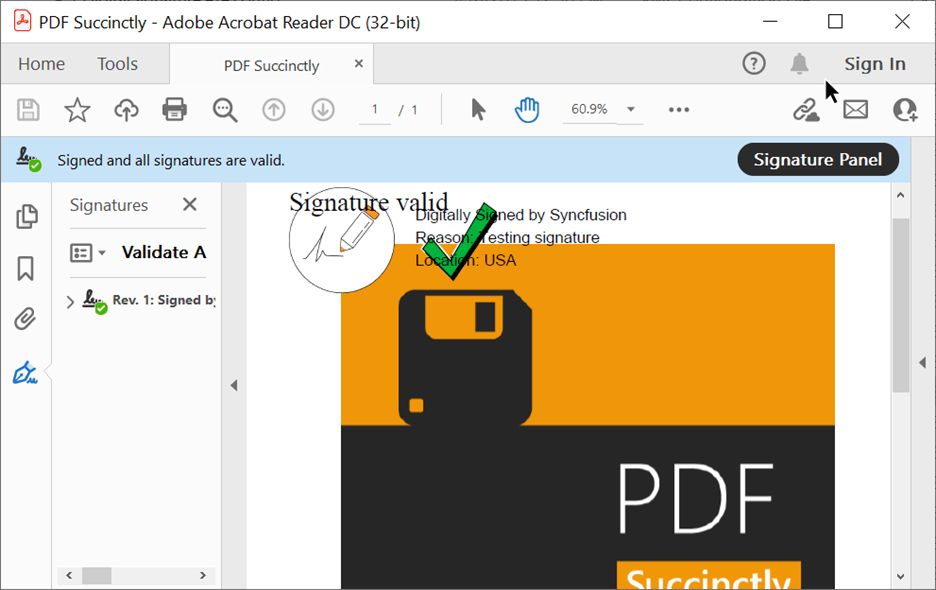
Create PDF digital signatures with CAdES and different hashing algorithms
CAdES (CMS advanced electronic signatures) is a standard developed by the European Telecommunications Standard Institute (ETSI) to facilitate secure, paperless transactions throughout the EU. You can find more details on the ETSI site.
By default, the Syncfusion PDF Library generates digital signatures with CMS (part 2 of the PAdES) standard and SHA 256 hashing algorithm.
You can change the digital signature standard to CAdES (part 3 of the PAdES) using the property CryptographicStandard available in the class PdfSignatureSettings. It also has the property DigestAlgorithm to change the hashing algorithm.
The following code example shows how to create a PDF digital signature in C# with CAdES standard and a different hashing algorithm.
using Syncfusion.Pdf.Parsing; using Syncfusion.Pdf.Security; class Program { static void Main(string[] args) { //Load existing PDF document. PdfLoadedDocument document = new PdfLoadedDocument("PDF_Succinctly.pdf"); //Load digital ID with password. PdfCertificate certificate = new PdfCertificate(@"DigitalSignatureTest.pfx", "DigitalPass123"); //Create a signature with loaded digital ID. PdfSignature signature = new PdfSignature(document, document.Pages[0], certificate, "DigitalSignature"); //Changing the digital signature standard and hashing algorithm. signature.Settings.CryptographicStandard = CryptographicStandard.CADES; signature.Settings.DigestAlgorithm = DigestAlgorithm.SHA512; //Save the PDF document. document.Save("SigneCAdES.pdf"); //Close the document. document.Close(true); } }
By executing this code example, you will get a PDF document with the following digital signature properties.
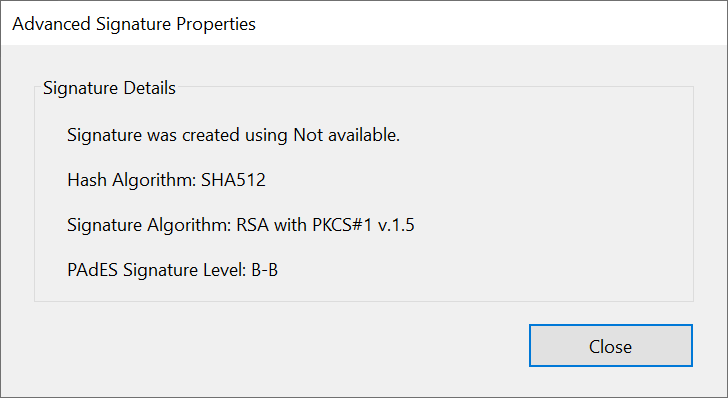

Unleash the full potential of Syncfusion's PDF Library! Explore our advanced resources and empower your apps with cutting-edge functionalities.
Add multiple digital signatures in a single PDF document
Imagine a book publisher creating a contract for a book. Such a contract could contain one certification signature from the publisher with terms and conditions and another approval signature from the author. So you need to add multiple digital signatures to a single PDF document.
You can add multiple digital signatures in a single PDF document by appending additional signatures to an already-signed PDF file.
In the following sample, we have added the first digital signature using the digital ID “TestAgreement.pfx”. This is called the first revision.
The second revision contains the digital signature with digital ID “DigitalSignatureTest.pfx”.
The following code example shows how to create multiple PDF digital signatures in C#.
using Syncfusion.Pdf.Parsing; using Syncfusion.Pdf.Security; class Program { static void Main(string[] args) { //Load existing PDF document. PdfLoadedDocument document = new PdfLoadedDocument("PDF_Succinctly.pdf"); //Load digital ID with password. PdfCertificate certificate = new PdfCertificate(@"TestAgreement.pfx", "Test123"); //Create a Revision 2 signature with loaded digital ID. PdfSignature signature = new PdfSignature(document, document.Pages[0], certificate, "DigitalSignature1"); //Changing the digital signature standard and hashing algorithm. signature.Settings.CryptographicStandard = CryptographicStandard.CADES; signature.Settings.DigestAlgorithm = DigestAlgorithm.SHA512; MemoryStream stream = new MemoryStream(); //Save the PDF document. document.Save(stream); //Close the document. document.Close(true); PdfLoadedDocument document2 = new PdfLoadedDocument(stream); //Load digital ID with password. PdfCertificate certificate2 = new PdfCertificate(@"DigitalSignatureTest.pfx", "DigitalPass123"); //Create a signature Revision 2 with loaded digital ID. PdfSignature signature2 = new PdfSignature(document2, document2.Pages[0], certificate2, "DigitalSignature2"); //Changing the digital signature standard and hashing algorithm. signature2.Settings.CryptographicStandard = CryptographicStandard.CADES; signature2.Settings.DigestAlgorithm = DigestAlgorithm.SHA512; //Save the PDF document. document2.Save("MultipleSignature.pdf"); //Close the document. document2.Close(true); } }
By executing this code example, you will get a PDF document with two digital signatures.
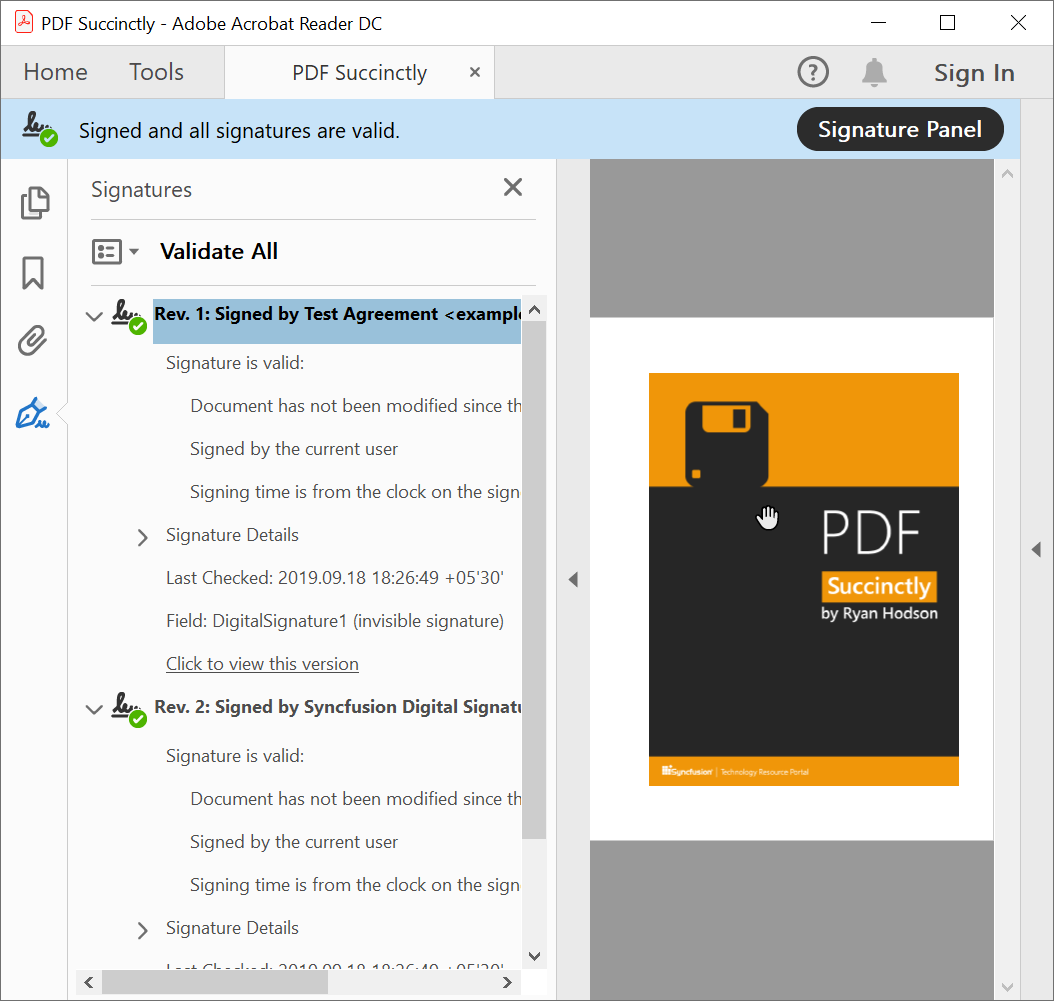
Digitally sign a PDF document using Windows certificate store
A secure way to store the digital ID is using a Windows certificate store. If a root certificate is added in the Windows certificate store, you don’t need to add and trust each of the certificates that are already present in the Windows certificate store manually.
You can retrieve the digital ID “X509Certificate2” from the Windows certificate store and use it to add a digital signature to a PDF document.
The following code example shows how to create a PDF digital signature in C# using the Windows certificate store.
using Syncfusion.Pdf.Parsing; using Syncfusion.Pdf.Security; using System.Security.Cryptography.X509Certificates; class Program { static void Main(string[] args) { //Initialize the Windows store. X509Store store = new X509Store("MY", StoreLocation.CurrentUser); store.Open(OpenFlags.ReadOnly | OpenFlags.OpenExistingOnly); X509Certificate2Collection collection = (X509Certificate2Collection)store.Certificates; //Find the certificate using thumb print. X509Certificate2Collection fcollection = (X509Certificate2Collection)collection.Find(X509FindType.FindByThumbprint, "F85E1C5D93115CA3F969DA3ABC8E0E9547FCCF5A", true); X509Certificate2 digitalID = collection[0]; //Load existing PDF document. PdfLoadedDocument document = new PdfLoadedDocument("PDF_Succinctly.pdf"); //Load X509Certificate2. PdfCertificate certificate = new PdfCertificate(digitalID); //Create a Revision 2 signature with loaded digital ID. PdfSignature signature = new PdfSignature(document, document.Pages[0], certificate, "DigitalSignature"); //Changing the digital signature standard and hashing algorithm. signature.Settings.CryptographicStandard = CryptographicStandard.CADES; signature.Settings.DigestAlgorithm = DigestAlgorithm.SHA512; //Save the PDF document. document.Save("WindowsStore.pdf"); //Close the document. document.Close(true); } }
By executing this code example, you will get a PDF document similar to the following screenshot.
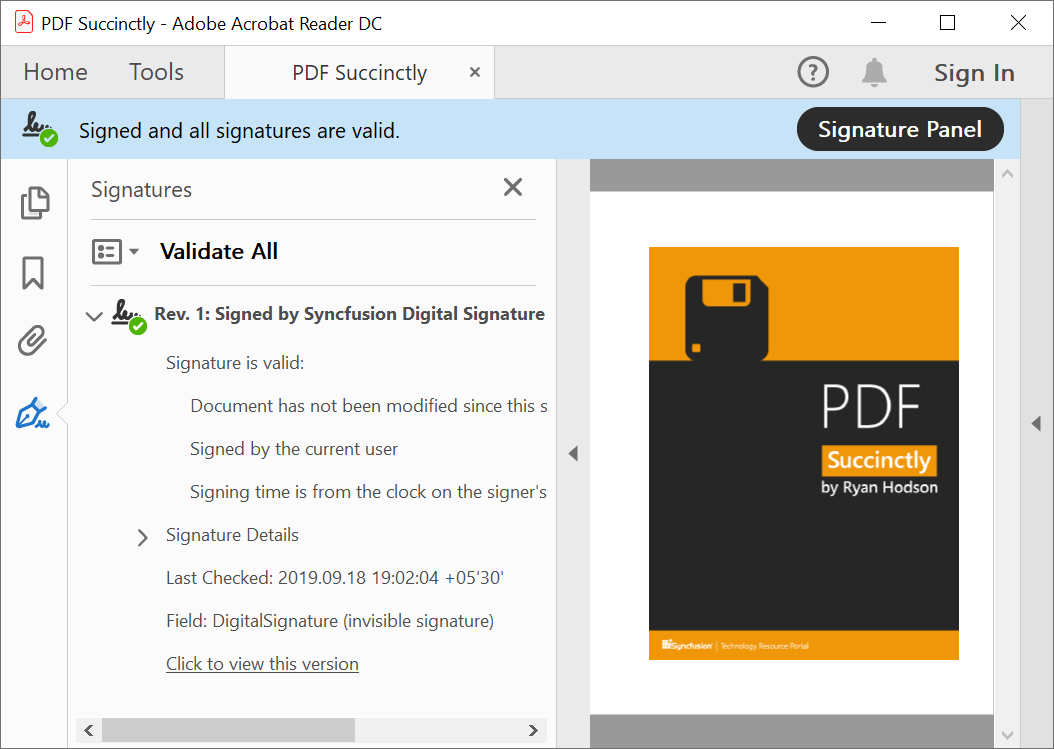

Embark on a virtual tour of Syncfusion's PDF Library through interactive demos.
Author or certify signature
An author signature, or certified signature, provides a higher level of document control than a normal signature over actions like form filling, comments, and digital signing.
For example, if an author publishes a book, he first signs the PDF document as an author as a way of certifying it. This way, he can control the document modification, such as annotation, form filling, and adding additional signatures. The publisher can then add a normal digital signature to the same document. If the publisher removes pages or adds comments, though, the document will not retain its certified status.
The certified signature can be applied only once to a PDF document and you cannot certify it if the document already has a digital signature. This means certifying is usually done by the author or creator of the document before it’s published or sent for additional signatures or form completion.
Certified documents display a blue ribbon across the top containing the signer’s name, company, and the certificate issuer—a clear, visual indicator of document authenticity and authorship.
The following code example shows a certified signature in a PDF document.
//Load existing PDF document. PdfLoadedDocument document = new PdfLoadedDocument("PDF_Succinctly.pdf"); //Load digital ID with password. PdfCertificate certificate = new PdfCertificate(@"DigitalSignatureTest.pfx", "DigitalPass123"); //Create a signature with loaded digital ID. PdfSignature signature = new PdfSignature(document, document.Pages[0], certificate, "DigitalSignature"); signature.Settings.CryptographicStandard = CryptographicStandard.CADES; signature.Settings.DigestAlgorithm = DigestAlgorithm.SHA256; //This property enables the author or certifying signature. signature.Certificated = true; //Allow the form fill and and comments. signature.DocumentPermissions = PdfCertificationFlags.AllowFormFill | PdfCertificationFlags.AllowComments; //Save the PDF document. document.Save("Certifying.pdf"); //Close the document. document.Close(true);
By executing this code example, you will get a PDF document similar to the following screenshot.
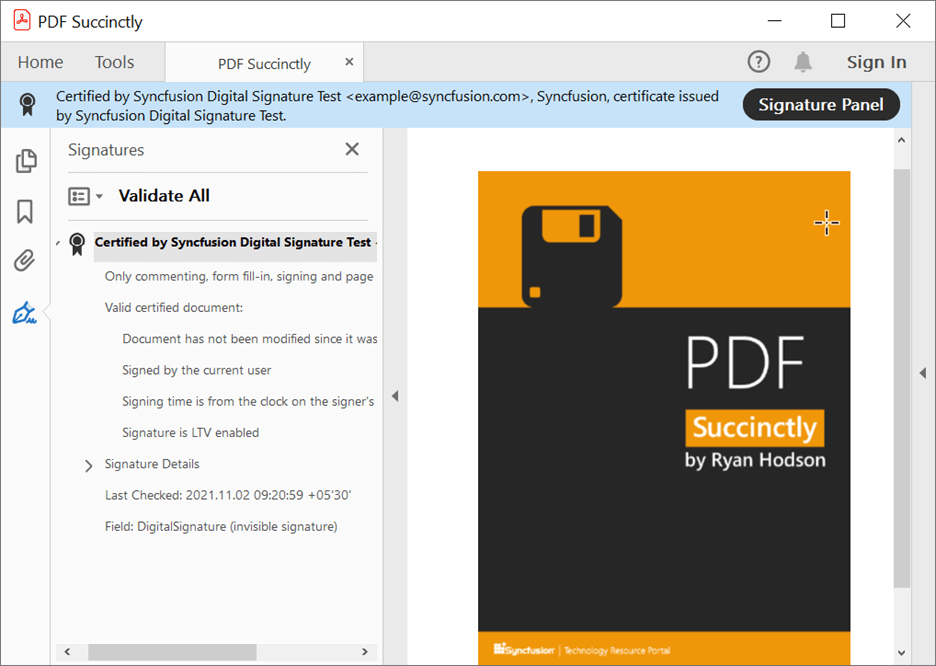
Digitally sign a PDF document with an external signature
Suppose that your company needs to create a huge number of signed PDF documents; you cannot do it manually, one by one. In this case, you need an automated solution such as signing documents in a server with its own HSM.
The PDF Library allows you to sign PDF documents with an external digital signature created from various sources such as an HSM, USB tokens, and smart cards, or other cloud services such as DigiSign.
The following code example shows how to create a PDF digital signature in C# using an external signature.
using Syncfusion.Pdf.Parsing; using Syncfusion.Pdf.Security; using System.Security.Cryptography; using System.Security.Cryptography.Pkcs; using System.Security.Cryptography.X509Certificates; class Program { static void Main(string[] args) { //Load existing PDF document. PdfLoadedDocument document = new PdfLoadedDocument("PDF_Succinctly.pdf"); //Create a Revision 2 signature with loaded digital ID. PdfSignature signature = new PdfSignature(document, document.Pages[0], null, "DigitalSignature"); signature.ComputeHash += Signature_ComputeHash; //Save the PDF document. document.Save("ExternalSignature.pdf"); //Close the document. document.Close(true); void Signature_ComputeHash(object sender, PdfSignatureEventArgs arguments) { //Get the document bytes. byte[] documentBytes = arguments.Data; SignedCms signedCms = new SignedCms(new ContentInfo(documentBytes), detached: true); //Compute the signature using the specified digital ID file and the password. X509Certificate2 certificate = new X509Certificate2("DigitalSignatureTest.pfx", "DigitalPass123"); var cmsSigner = new CmsSigner(certificate); //Set the digest algorithm SHA256. cmsSigner.DigestAlgorithm = new Oid("2.16.840.1.101.3.4.2.1"); signedCms.ComputeSignature(cmsSigner); //Embed the encoded digital signature to the PDF document. arguments.SignedData = signedCms.Encode(); } } }
By executing this code example, you will get a PDF document similar to the following screenshot.
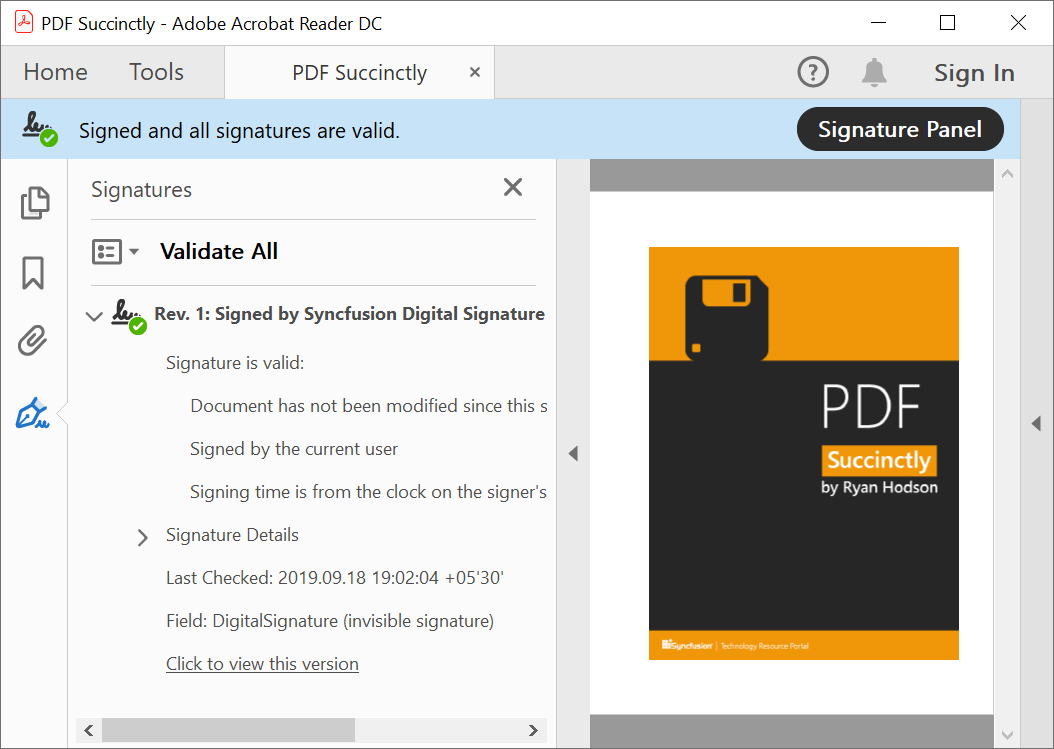

See a world of document processing possibilities in Syncfusion's PDF Library as we unveil its features in interactive demonstrations.
Digitally sign the existing signature field in a PDF document
You can load the signature field from an existing PDF document, and you can add the digital signature to it.
The following code example shows how to load an existing signature field and add a PDF digital signature in C#.
using Syncfusion.Pdf; using Syncfusion.Pdf.Graphics; using Syncfusion.Pdf.Parsing; using Syncfusion.Pdf.Security; class Program { static void Main(string[] args) { //Load existing PDF document. PdfLoadedDocument document = new PdfLoadedDocument("PDF_SignField.pdf"); //Get the first page of the document. PdfLoadedPage page = document.Pages[0] as PdfLoadedPage; //Gets the first signature field from the PDF document. PdfLoadedSignatureField field = document.Form.Fields[0] as PdfLoadedSignatureField; //Load digital ID with password. PdfCertificate certificate = new PdfCertificate("DigitalSignatureTest.pfx", "DigitalPass123"); field.Signature = new PdfSignature(document, page, certificate, "Signature", field); //Get graphics from form field. PdfGraphics graphics = field.Signature.Appearance.Normal.Graphics; //Load image from file. PdfImage image = PdfImage.FromFile("signature.png"); //Create a font to draw text. PdfStandardFont font = new PdfStandardFont(PdfFontFamily.Helvetica, 15); //Draw text, shape, and image into the signature appearance. graphics.DrawRectangle(PdfPens.Black, PdfBrushes.White, new System.Drawing.RectangleF(50, 0, field.Bounds.Width-50, field.Bounds.Height)); graphics.DrawImage(image, 0, 0, 100, field.Bounds.Height); graphics.DrawString("Digitally Signed by Syncfusion", font, PdfBrushes.Black, 120, 17); graphics.DrawString("Reason: Testing signature", font, PdfBrushes.Black, 120, 39); graphics.DrawString("Location: USA", font, PdfBrushes.Black, 120, 60); //Save the document. document.Save("SignedField.pdf"); //Close the document. document.Close(true); } }
To create a signature field, please refer to this UG documentation.
By executing this code example, you will get a PDF document similar to the following screenshot.
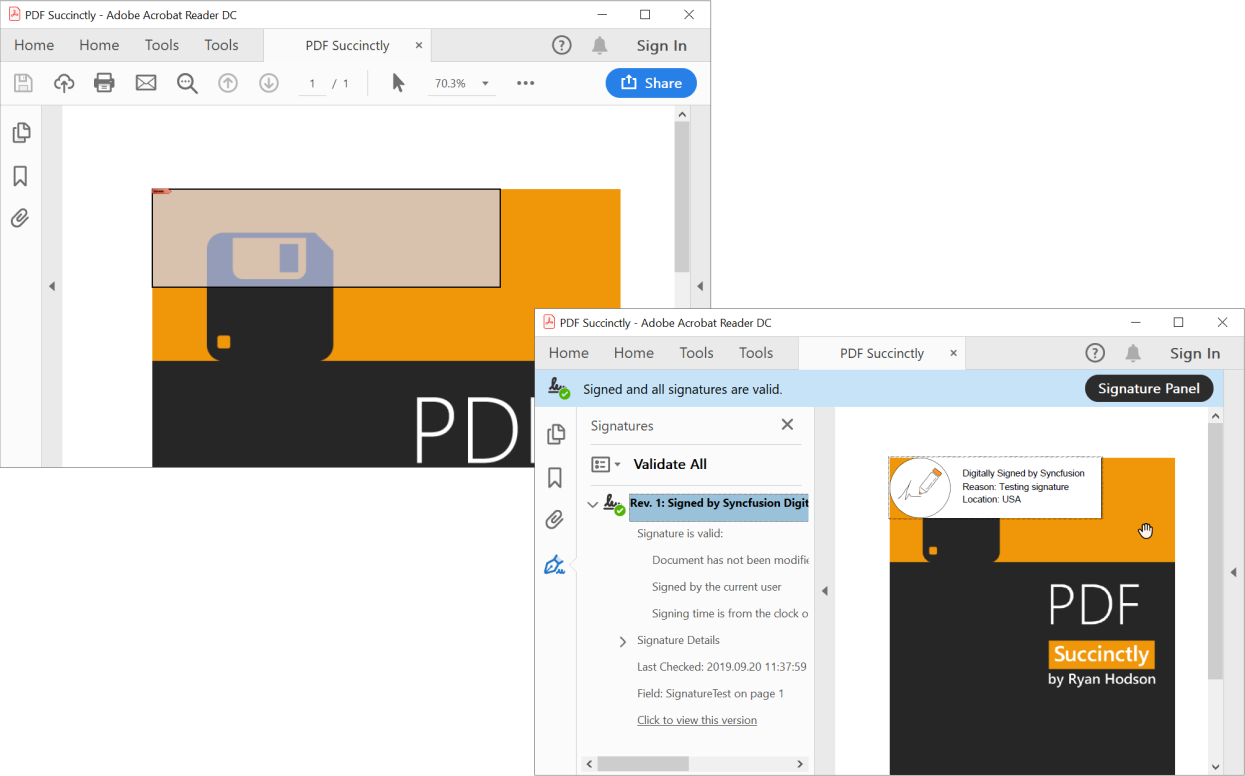
Add timestamp to the digital signature
A digital timestamp is used to create a PDF signature with a secure time and date as proof of integrity.
The following code example shows how to create a PDF digital signature in C# with a timestamp.
using Syncfusion.Pdf.Parsing; using Syncfusion.Pdf.Security; class Program { static void Main(string[] args) { //Load existing PDF document. PdfLoadedDocument document = new PdfLoadedDocument("PDF_Succinctly.pdf"); //Load digital ID with password. PdfCertificate certificate = new PdfCertificate(@"DigitalSignatureTest.pfx", "DigitalPass123"); //Create a signature with loaded digital ID. PdfSignature signature = new PdfSignature(document, document.Pages[0], certificate, "DigitalSignature"); //Change the digital signature standard and hashing algorithm. signature.Settings.CryptographicStandard = CryptographicStandard.CADES; signature.Settings.DigestAlgorithm = DigestAlgorithm.SHA512; //Add timestamp server link to the signature. signature.TimeStampServer = new TimeStampServer(new Uri("http://timestamp.digicert.com/")); //Save the PDF document. document.Save("SignedTimestamp.pdf"); //Close the document. document.Close(true); } }
By executing this code example, you will get a PDF document with the following information.
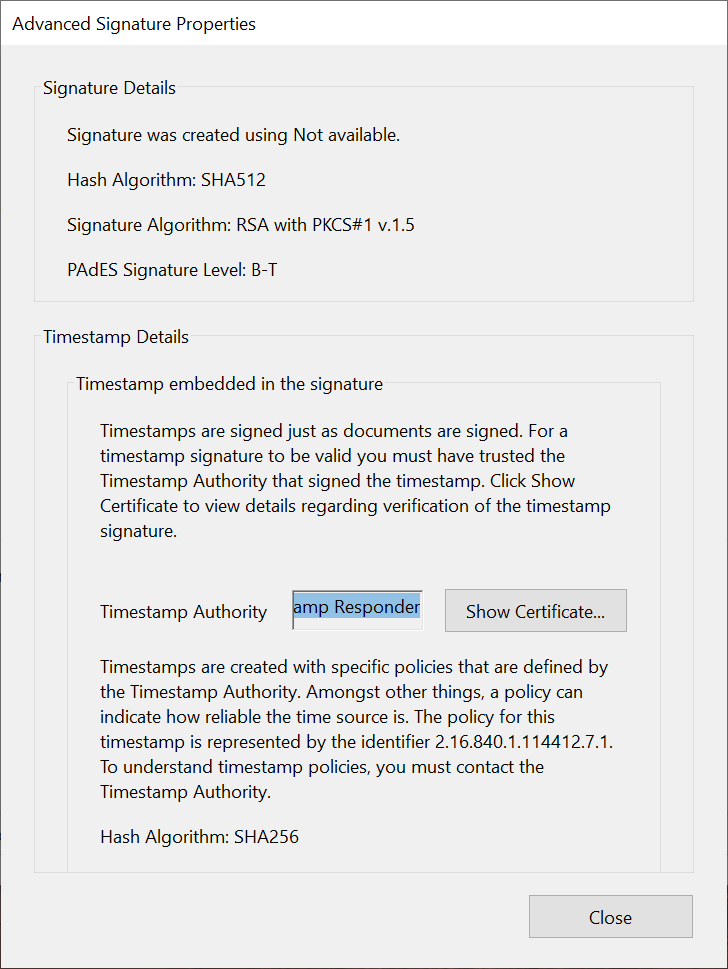
Digitally sign a PDF document with long-term validation (LTV)
A PDF document with an LTV signature allows you to create a signature for documents. Once a PDF document is signed with an LTV signature, it can be valid for the long term even if the root certificate (CA) is revoked.
The PDF LTV follows the standard PAdES B-LT, so at the time of signing the PDF document, all the signature information such as the OCSP, CRL, and CA certificate is captured and stored in the document security store (DSS). This stored information is used to validate the signature in the future without depending on any external services. This standard is recommended for advanced electronic signatures.
The following code example shows how to sign a PDF document with LTV.
using Syncfusion.Pdf.Parsing; using Syncfusion.Pdf.Security; namespace DigitalSignature { class Program { static void Main(string[] args) { //Load existing PDF document. PdfLoadedDocument document = new PdfLoadedDocument("PDF_Succinctly.pdf"); //Load digital ID with password. PdfCertificate certificate = new PdfCertificate(@"DigitalSignatureTest.pfx", "DigitalPass123"); //Create a signature with loaded digital ID. PdfSignature signature = new PdfSignature(document, document.Pages[0], certificate, "DigitalSignature"); signature.Settings.CryptographicStandard = CryptographicStandard.CADES; signature.Settings.DigestAlgorithm = DigestAlgorithm.SHA256; signature.TimeStampServer = new TimeStampServer(new Uri("http://timestamping.ensuredca.com")); //Enable LTV document. signature.EnableLtv = true; //Save the PDF document. document.Save("LTV_document.pdf"); //Close the document. document.Close(true); } } }
By executing this code example, you will get a PDF document similar to the following screenshot.
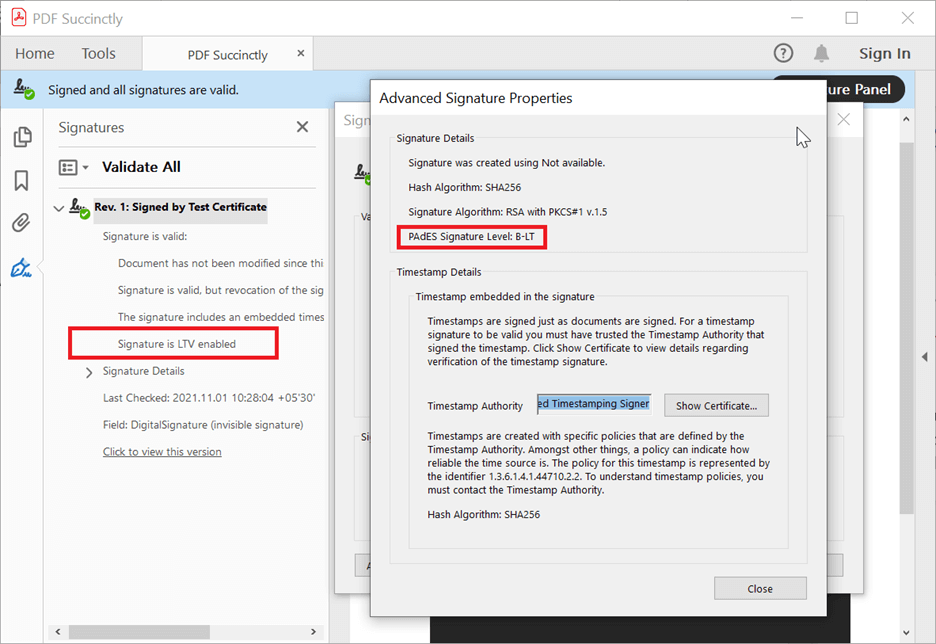

Witness the advanced capabilities of Syncfusion's PDF Library with feature showcases.
Digitally sign a PDF document with long-term archive timestamps (LTA)
The PDF LTA signature is the next level of the LTV signature. It follows the standard PAdES B-LTA. As per the standard, the validation-related information of the timestamp is added to the DSS along with other signature information mentioned in the LTV signature.
The document timestamp is also applied to the PDF document, so it provides more viability to the signature. This level is recommended for qualified electronic signatures.
The following code example shows how to sign a PDF document with LTA.
//Load existing PDF document. PdfLoadedDocument document = new PdfLoadedDocument("PDF_Succinctly.pdf"); //Load digital ID with password. PdfCertificate certificate = new PdfCertificate(@"DigitalSignatureTest.pfx", "DigitalPass123"); //Create a signature with loaded digital ID. PdfSignature signature = new PdfSignature(document, document.Pages[0], certificate, "DigitalSignature"); signature.Settings.CryptographicStandard = CryptographicStandard.CADES; signature.Settings.DigestAlgorithm = DigestAlgorithm.SHA256; signature.TimeStampServer = new TimeStampServer(new Uri("http://timestamping.ensuredca.com")); //Enable LTV document. signature.EnableLtv = true; //Save the PDF document. document.Save("LTV_document.pdf"); //Close the document. document.Close(true); PdfLoadedDocument ltDocument = new PdfLoadedDocument("LTV_document.pdf"); //Load the existing PDF page. PdfLoadedPage lpage = ltDocument.Pages[0] as PdfLoadedPage; //Create PDF signature with empty certificate. PdfSignature timeStamp = new PdfSignature(lpage, "timestamp"); timeStamp.TimeStampServer = new TimeStampServer(new Uri("http://timestamping.ensuredca.com")); ltDocument.Save("PAdES B-LTA.pdf"); ltDocument.Close(true);
By executing this code example, you will get a PDF document similar to the following screenshot.
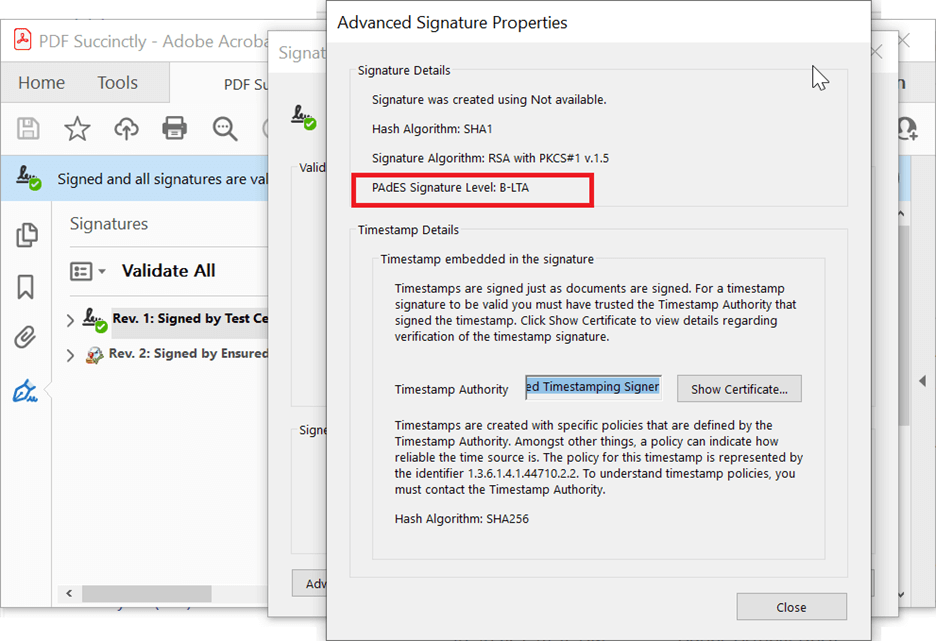
Create LTV in an external signature
An external signature is usually used to sign a document hash separately and later embed it into the PDF document. So, using an external signature, you can sign a PDF document from different sources such as HSM, USB tokens, and smart cards, or other cloud services such as DigiSign.
The following code example creates an LTV document when using an external signature.
using Syncfusion.Pdf.Parsing; using Syncfusion.Pdf.Security; using System; using System.Security.Cryptography; using System.Security.Cryptography.X509Certificates; namespace DigitalSignature { class Program { static void Main(string[] args) { //Load existing PDF document. PdfLoadedDocument document = new PdfLoadedDocument("PDF_Succinctly.pdf"); //Create certificate chain list. System.Collections.Generic.List<X509Certificate2> certificates = new System.Collections.Generic.List<X509Certificate2>(); X509Certificate2 digitalId = new X509Certificate2(@"certchain.pfx", "password", X509KeyStorageFlags.Exportable); X509Chain chain = new X509Chain(); chain.Build(digitalId); for (int i = 0; i < chain.ChainElements.Count; i++) { certificates.Add(chain.ChainElements[i].Certificate); } //Create a revision 2 signature with loaded digital ID. PdfSignature signature = new PdfSignature(document, document.Pages[0], null, "DigitalSignature"); //Set the cryptographic standard. signature.Settings.CryptographicStandard = CryptographicStandard.CADES; signature.Settings.DigestAlgorithm = DigestAlgorithm.SHA1; //Create an external signature. IPdfExternalSigner externalSignature = new ExternalSigner("SHA1"); //Add external signer to the signature. signature.AddExternalSigner(externalSignature, certificates, null); //Create long term validity signature.CreateLongTermValidity(certificates); //Set timestamp server. signature.TimeStampServer = new TimeStampServer(new Uri("http://timestamping.ensuredca.com")); //Save the PDF document. document.Save("ExternalSignature.pdf"); //Close the document. document.Close(true); } //Create an external signature to sign the document hash. class ExternalSigner : IPdfExternalSigner { private string _hashAlgorithm; public string HashAlgorithm { get { return _hashAlgorithm; } } public ExternalSigner(string hashAlgorithm) { _hashAlgorithm = hashAlgorithm; } //Sing the PDF hash. public byte[] Sign(byte[] message, out byte[] timeStampResponse) { timeStampResponse = null; return SignDocumentHash(message); } private byte[] SignDocumentHash(byte[] documentHash) { X509Certificate2 digitalID = new X509Certificate2(@"certchain.pfx", "password", X509KeyStorageFlags.Exportable); if (digitalID.PrivateKey is RSACryptoServiceProvider) { System.Security.Cryptography.RSACryptoServiceProvider rsa = (System.Security.Cryptography.RSACryptoServiceProvider)digitalID.PrivateKey; return rsa.SignData(documentHash, HashAlgorithm); } else if (digitalID.PrivateKey is RSACng) { RSACng rsa = (RSACng)digitalID.PrivateKey; return rsa.SignData(documentHash, System.Security.Cryptography.HashAlgorithmName.SHA1, RSASignaturePadding.Pkcs1); } else { return null; } } } } }
By executing this code example, you will get a PDF document similar to the following screenshot.
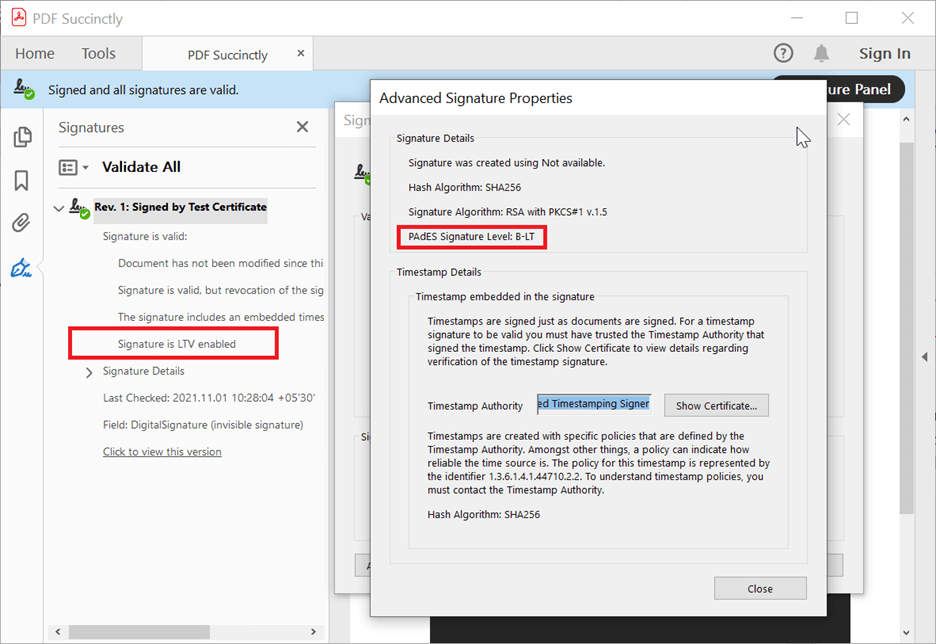
Retrieve digital signature information from an existing PDF document
Using Syncfusion PDF Library, you can retrieve useful digital signature information, such as issuer name, validity, and digest algorithm, from an existing PDF document and display this information in your application.
The following code example shows how to retrieve digital signature information from an existing PDF document.
using Syncfusion.Pdf.Parsing; using Syncfusion.Pdf.Security; class Program { static void Main(string[] args) { //Load an existing PDF document. PdfLoadedDocument document = new PdfLoadedDocument("SignedAppearance.pdf"); //Get the signature field from PdfLoadedDocument form field collection. PdfLoadedSignatureField signatureField = document.Form.Fields[0] as PdfLoadedSignatureField; PdfSignature signature = signatureField.Signature; //Extract the signature information. Console.WriteLine("Digitally Signed by: " + signature.Certificate.IssuerName); Console.WriteLine("Valid From: " + signature.Certificate.ValidFrom); Console.WriteLine("Valid To: " + signature.Certificate.ValidTo); Console.WriteLine("Hash Algorithm : " + signature.Settings.DigestAlgorithm); Console.WriteLine("Cryptographics Standard : " + signature.Settings.CryptographicStandard); //Close the document. document.Close(true); } }
Remove existing digital signatures from a PDF document
If you want to remove the existing contract and going to create a new one, you can remove the digital signatures from the existing PDF document.
You can remove a digital signature from a PDF document using the following code example.
using Syncfusion.Pdf.Parsing; class Program { static void Main(string[] args) { //Load an existing PDF document. PdfLoadedDocument document = new PdfLoadedDocument("SignedAppearance.pdf"); //Get the signature field from PdfloadedDocument form field collection. PdfLoadedSignatureField signatureField = document.Form.Fields[0] as PdfLoadedSignatureField; //Remove signature field from PdfLoadedDocument form field collection. document.Form.Fields.Remove(signatureField); //Save the PDF document. document.Save("RemoveDigital.pdf"); document.Close(true); } }
By executing this code example, you will get a PDF document like the following.
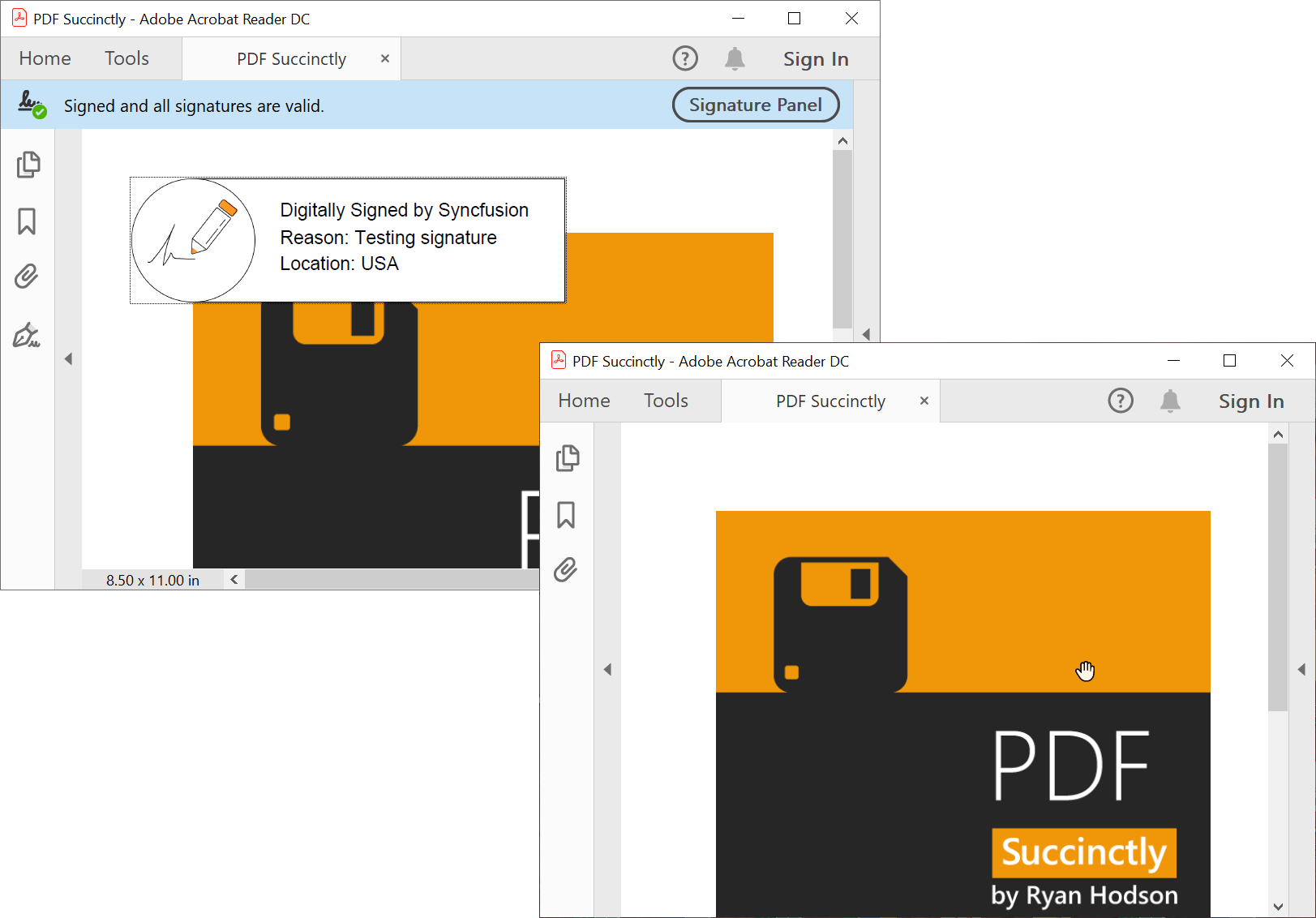
Validate PDF digital signature
To ensure the authenticity and integrity of a PDF document, you must validate the digital signature present in it. If you have a huge number of PDF documents, you cannot validate each document manually, so you need an automated solution.
The PDF Library has an API to validate digital signatures. You can validate the digital signatures in any number of PDF documents without human interaction.
Digital signature validation does the following steps to ensure validity:
- Validate the document modification.
- Validate the certificate chain.
- Ensure the signature with timestamp time.
- Check the revocation status of the certificate with OCSP and CRL.
- Ensure multiple digital signatures.
The following code example shows how to validate all the digital signatures present in a PDF document.
using Syncfusion.Pdf.Parsing; using Syncfusion.Pdf.Security; class Program { static void Main(string[] args) { //Load an existing PDF document. PdfLoadedDocument document = new PdfLoadedDocument("MultipleSignature.pdf"); //Load PDF form. PdfLoadedForm form = document.Form; List<PdfSignatureValidationResult> results; if (form != null) { //Validate all the digital signatures present in the PDF document. bool isvalid = form.Fields.ValidateSignatures(out results); //Show the result based on the result. if (isvalid) Console.WriteLine("All signatures are valid"); else Console.WriteLine("At least one signature is invalid"); } //Close the document. document.Close(true); } }
The previous code example will iterate and validate all the digital signatures present in the PDF document. If any one of the digital signatures is invalid, then the result will be false. You can also get the validation results of the individual signatures.
The PdfSignatureValidationResult contains information about each digital signature and its status. We will see more details in the upcoming topics.
Validate individual digital signatures in an existing PDF document
Syncfusion PDF Library allows you to iterate and validate individual digital signatures in an existing PDF document. The following code shows how to validate individual digital signatures.
using Syncfusion.Pdf.Parsing; using Syncfusion.Pdf.Security; class Program { static void Main(string[] args) { //Load an existing PDF document. PdfLoadedDocument document = new PdfLoadedDocument("MultipleSignature.pdf"); //Load PDF form. PdfLoadedForm form = document.Form; if (form != null) { foreach (PdfLoadedField field in form.Fields) { if (field is PdfLoadedSignatureField) { PdfLoadedSignatureField signatureField = field as PdfLoadedSignatureField; //Check whether the signature is signed. if (signatureField.IsSigned) { //Validate the digital signature. PdfSignatureValidationResult result = signatureField.ValidateSignature(); if (result.IsSignatureValid) Console.WriteLine("Signature is valid"); else Console.WriteLine("Signature is invalid"); //Retrieve the signature information. Console.WriteLine("<<<<Validation summary>>>>>>"); Console.WriteLine("Digitally Signed by: " + signatureField.Signature.Certificate.IssuerName); Console.WriteLine("Valid From: " + signatureField.Signature.Certificate.ValidFrom); Console.WriteLine("Valid To: " + signatureField.Signature.Certificate.ValidTo); Console.WriteLine("Signature Algorithm : " + result.SignatureAlgorithm); Console.WriteLine("Hash Algorithm : " + result.DigestAlgorithm); Console.WriteLine("Cryptographics Standard : " + result.CryptographicStandard); Console.Read(); } } } } } }
By executing this code example, you will get a PDF document with information similar to the following screenshot.
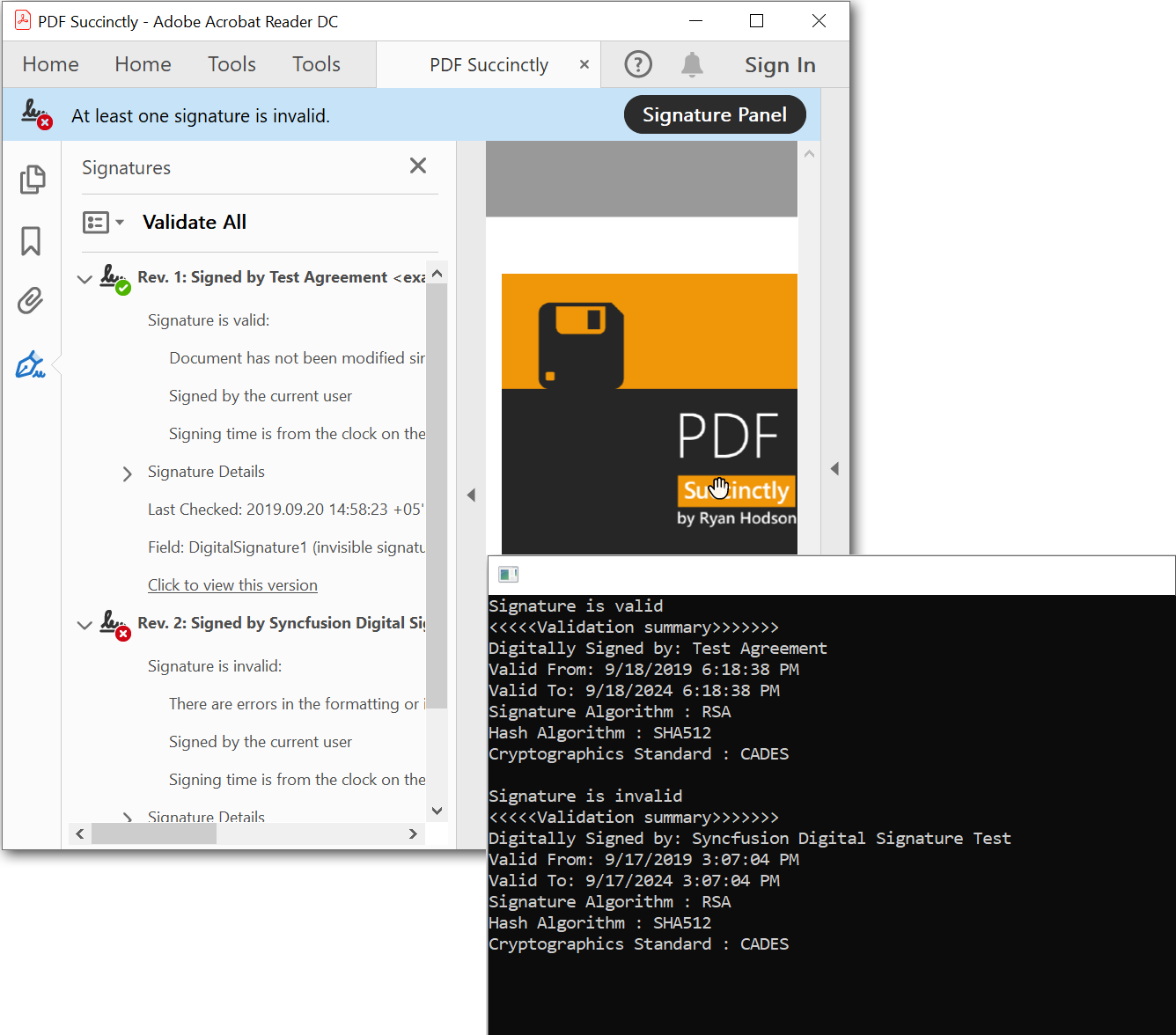
Validating signatures against a trusted list
You can create and pass your own trusted list of certificates to validate a digital signature in a PDF document.
The following example shows how to load a local Windows certificate store and validate the digital signature against the Window certificate store.
using Syncfusion.Pdf.Parsing; using Syncfusion.Pdf.Security; using System.Security.Cryptography.X509Certificates; class Program { static void Main(string[] args) { //Load an existing PDF document. PdfLoadedDocument document = new PdfLoadedDocument("MultipleSignature.pdf"); //Load PDF form. PdfLoadedForm form = document.Form; //Load Windows certificate store. X509Store store = new X509Store("MY", StoreLocation.CurrentUser); store.Open(OpenFlags.ReadOnly | OpenFlags.OpenExistingOnly); X509Certificate2Collection collection = (X509Certificate2Collection)store.Certificates; if (form != null) { foreach (PdfLoadedField field in form.Fields) { if (field is PdfLoadedSignatureField) { PdfLoadedSignatureField signatureField = field as PdfLoadedSignatureField; //Validate the digital signature against Windows certificate store. PdfSignatureValidationResult result = signatureField.ValidateSignature(collection); if (result.IsSignatureValid) Console.WriteLine("Signature is valid"); else Console.WriteLine("Signature is invalid"); //Update the signatures status based on the certificate validation against certificate store. Console.WriteLine("Signature status: " + result.SignatureStatus); } } } } }
GitHub reference
You can find all the examples of create and validate PDF digital signatures in the GitHub repository.

Syncfusion’s high-performance PDF Library allows you to create PDF documents from scratch without Adobe dependencies.
Conclusion
In this blog post, we have walked through how to create and validate PDF digital signatures in C# using Syncfusion PDF Library.
Now you can easily include PDF digital signatures and validate them in your development process with Syncfusion PDF Library.
Take a moment to peruse our documentation, where you’ll find other options and features, all with accompanying code examples.
If you have any questions about these features, please let us know in the comments below. You can also contact us through our support forum, support portal, or feedback portal. We are happy to assist you!
If you liked this article, we think you would also like the following articles about PDF Library:
Comments (17)
Nice article you have made.your information is useful for me thank you for sharing a needful knowledge.
thank you for sharing this valuable information
nice work ,
Hi Mr PRAVEENKUMAR,
I would like to know if Syncfusion have function to embed the adobe into web, so we can directly sign in adobe inside that web. Any suggestion will be appreciate. Thank You
Hi Shella Lim,
Yes, you can embed Syncfusion EJ2 PDF Viewer into web application to display the PDF document in the browser. Please refer the online demo sample from the following link,
Demo link: https://ej2.syncfusion.com/demos/#/material/pdfviewer/default
Documentation link: https://ej2.syncfusion.com/documentation/pdfviewer/getting-started
As of now, we don’t have support for adding a digital signature in our PDF Viewer. However, you can add a signature to the PDF document using Handwritten signature support provided in our PDF Viewer as using below steps
STEP 1: Click the Edit icon in the toolbar
STEP 2: Click the signature icon in the secondary toolbar
STEP 3: Add your sign in the popup dialogue and click the create button
STEP 4: Now, click anywhere in the PDF document to add your signature in that position.
Please let us know if you have any queries about this.
Regards,
Praveen
Very nice post. I certainly love this site.
Stick with it!
Hello Praveen,
Thanks for sharing this informative article .
Do Signatures created using these steps be considered as Class 2 Digital Signature as required by many agencies in India ? If possible could you please share some insights on how Class 2 or Class 3 Digital Signatures can be embedded to PDF reports generated using SSRS .
Hi Rohit,
We support both the self-signed and class 2 & 3 certificate using the same code stated in the article. We can use the below code for both the class 2 and class 3 digital certificates to sign the PDF document.
//Load existing PDF document.
PdfLoadedDocument document = new PdfLoadedDocument(“PDF_Succinctly.pdf”);
//Load digital ID with password.
PdfCertificate certificate = new PdfCertificate(@”DigitalSignatureTest.pfx”, “DigitalPass123”);
//Create a signature with loaded digital ID.
PdfSignature signature = new PdfSignature(document, document.Pages[0], certificate, “DigitalSignature”);
//Save the PDF document.
document.Save(“SignedDocument.pdf”);
//Close the document.
document.Close(true);
Also if you need to identify the certificate classes, then we can find it by using the certificate policy id like below.
Certificate Class OID
Class 1 2.16.356.100.2.1
Class 2 2.16.356.100.2.2
Class 3 2.16.356.100.2.3
Refer the following code to identify the certificate class.
//Load the certificate
X509Certificate2 certificate = new X509Certificate2(certificateData, password);
//Check whether the certificate is self-signed or not.
if (certificate.SubjectName.RawData.SequenceEqual(certificate.IssuerName.RawData))
{
Console.WriteLine(“Self-signed certificate.”);
}
else
{
String policyId = null;
//If the certificate is not self-signed, then get the policy id.
foreach (X509Extension extension in certificate.Extensions)
{
if (extension.Oid.FriendlyName.Equals(“Certificate Policies”))
{
policyId = GetPolicyID(extension.Format(false));
break;
}
}
if (policyId != null)
{
if (policyId == “2.16.356.100.2.1”)
{
Console.WriteLine(“Class 1 Certificate.”);
}
else if (policyId == “2.16.356.100.2.2”)
{
Console.WriteLine(“Class 2 Certificate.”);
}
else if (policyId == “2.16.356.100.2.3 “)
{
Console.WriteLine(“Class 3 Certificate.”);
}
}
else
{
Console.WriteLine(“Could not found policy identifier”);
}
}
///
/// Get the policy id
///
private string GetPolicyID(string policy)
{
string policyId;
string keyWord = “Policy Identifier=”;
int index = policy.IndexOf(keyWord) + keyWord.Length;
int nextIndex = policy.IndexOf(“,”, index);
if (nextIndex > 0)
{
policyId = policy.Substring(index, nextIndex – index);
}
else
{
policyId = policy.Substring(index, policy.Length – index);
}
return policyId;
}
Please let us know if you have any queries.
Regards,
Praveen
Nice Article
Dear Praveenkumar:
Is there any code sample, where user can draw rectangle (for positioning and sizing signature) using ej2 pdfviewer and then our razor page or cntroller gets the coordinates of the rectangle?
Please point me to right sample.
Hi Bharat,
We can draw the rectangle in the PDF document from our PDF Viewer, Please refer the following documentation for more information,
https://blazor.syncfusion.com/documentation/pdfviewer/annotation/shape-annotation/
Instead of selecting rectangle annotation mode from the toolbar, we can set the annotation mode by using the following code snippet then can draw the rectangle annotation in the PDF document directly,
Select Rectangle Annotation Mode
function selectAnnotationMode() {
var pdfViewer = document.getElementById(‘pdfViewer’).ej2_instances[0];
pdfViewer.annotation.setAnnotationMode(‘Rectangle’);
}
Please refer the following code snippet to get Rectangle bounds using annotationAdd event in Client,
function annotationAdded(args) {
var annotId = args.annotationId;
var annotBound = args.annotationBound;
}
Please refer the following code snippet to get Rectangle bounds using exportAnnotationsAsObject method in controller,
Client code
ExportObject
function exportObject() {
var pdfViewer = document.getElementById(‘pdfViewer’).ej2_instances[0];
pdfViewer.exportAnnotationsAsObject().then(function (values) {
var annotations = JSON.parse(values);
//Here also we can get the bounds
var bounds = annotations.pdfAnnotation[0].shapeAnnotation[0].Bounds;
});
}
Server code
[AcceptVerbs(“Post”)]
[HttpPost]
public IActionResult ExportAnnotations([FromBody] Dictionary jsonObject)
{
PdfRenderer pdfviewer = new PdfRenderer(_cache);
var annotations = jsonObject[“pdfAnnotation”];
var annotation = JsonConvert.DeserializeObject<Dictionary>(annotations);
for (int j = 0; j < annotation.Count; j++)
{
var annotationcollection= annotation[j];
var exportedAnnotations = JsonConvert.DeserializeObject<Dictionary>(annotationcollection.ToString());
var shapeAnnotationCollection = JsonConvert.DeserializeObject<List<Dictionary>>(exportedAnnotations[“shapeAnnotation”].ToString());
for (var s = 0; s < shapeAnnotationCollection.Count; s++)
{
Dictionary shapeAnnotation = shapeAnnotationCollection[s];
// Here we can get the bounds for rectangle annotation
Dictionary bounds = JsonConvert.DeserializeObject<Dictionary>(shapeAnnotation[“bounds”].ToString());
}
}
string jsonResult = pdfviewer.GetAnnotations(jsonObject);
return Content(jsonResult);
}
We have prepared the simple sample to add Rectangle in PDF page and get the bounds for that rectangle and it can be downloaded from the following location,
Sample Location: https://www.syncfusion.com/downloads/support/directtrac/general/7z/PdfViewerSample-13757716631463818922.7z
If you still need further assistance please create a support ticket with more details about your requirement, it will be helpful for us to investigate further and assist you better.
Regards,
Praveen
Hello Praveen, how can I loop through annotations on a pdf and replace the annotation with a signature popup control?
Hi Scott,
We can able to loop through the annotation in the PDF document and replace it with a signature field. Please refer the below code snippet for more details,
//Load PDF document
PdfLoadedDocument loadedDocument = new PdfLoadedDocument(“../../Data/Annotation.pdf”);
for (int i = 0; i =0; j–)
{
//Get bounds from annotation
RectangleF bounds = annotationCollection[j].Bounds;
//Remove the annotation
annotationCollection.RemoveAt(j);
//Create PDF Signature field
PdfSignatureField signatureField = new PdfSignatureField(loadedPage, “Signature”);
//Set properties to the signature field
signatureField.Bounds = bounds;
signatureField.ToolTip = “Signature”;
//Add the form field to the document.
loadedDocument.Form.Fields.Add(signatureField);
}
}
//Save the PDF document
loadedDocument.Save(“Sample.pdf”);
loadedDocument.Close(true);
Please try the above solution in your end and let us know if you have any concerns on this.
Regards,
Sowmiya Loganathan
Thank you Sowmiya! This works great!
One thing I noticed. After the signature control is added, when the user applies his signature and the control loses focus, the user cannot go back to the control and change his signature. Is that default behavior or is there a way to ensure the user can change the signature if they do not like it?
Hi Scott,
Once the signature is added to the signature field, we cannot change it (or go back to the signature field) and this is the behavior. If your requirement is to add the image (i.e., JPEG) to the signature field, we can achieve your requirement by using PdfRubberStampAnnotation (add an image in the appearance of the annotation). If we use RubberStampAnnotation to add the image, we can easily edit it. Please refer to the below sample for more details,
Sample: https://www.syncfusion.com/downloads/support/directtrac/general/ze/AnnotationSample-765982694
Output document: https://www.syncfusion.com/downloads/support/directtrac/general/pd/RubberStamp-24308161
Please try the above sample on your end and let us know if it satisfies your requirement.
Regards,
Gowthamraj K
Hi PRAVEENKUMAR,
I would like to use syncfusion to check if the pdf has digital signature or not. Do I need a license to use syncfusion for this purpose.
Thanks,
NP
Hi Nisha
Yes, you will need the license to consume the Syncfusion .NET PDF library. Otherwise, a watermark will be added to the generated PDF document.
The whole suite of controls is available for free (commercial applications also) through the community license ( https://www.syncfusion.com/products/communitylicense) program if you qualify. The community license is the full product with no limitations or watermarks.
Regards,
Praveen
TY for the useful information! I wouldnt have gotten this myself!
Comments are closed.