TL;DR: Syncfusion’s Excel Library provides a full-fledged object model that facilitates accessing and manipulating Excel documents without Microsoft Office or interop dependencies. Let’s learn to create Excel tables with this comprehensive tool in just three simple steps.
The Syncfusion Excel Library, also known as Essential XlsIO, is a robust tool that facilitates the smooth creation, reading, and editing of Excel documents using C#. It supports the creation of Excel documents from scratch, modification of existing Excel documents, data import and export, Excel formulas, conditional formats, data validations, charts, sparklines, tables, pivot tables, pivot charts, template markers, and much more.

Enjoy a smooth experience with Syncfusion’s Excel Library! Get started with a few lines of code and without Microsoft or interop dependencies.
This blog will explore the steps to create an Excel table using the Syncfusion Excel Library and C#.
Note: Please refer to the .NET Excel Library’s getting started documentation before proceeding.
Steps to create a table in Excel
Follow these steps to create a table in an Excel document using C#:
- First, create a .NET Core Console application in Visual Studio, as shown in the following image.
- Then, install the latest Syncfusion.XlsIO.NET.Core NuGet package to your app.
- Now, add the following code to create a table in the existing Excel document.
using Syncfusion.XlsIO; namespace ExcelTable { class Program { public static void Main() { Program program = new Program(); program.CreateTable(); } /// <summary> /// Creates a table in the existing Excel document. /// </summary> public void CreateTable() { using (ExcelEngine excelEngine = new ExcelEngine()) { IApplication application = excelEngine.Excel; application.DefaultVersion = ExcelVersion.Xlsx; FileStream fileStream = new FileStream("../../../Data/SalesReport.xlsx", FileMode.Open, FileAccess.Read); IWorkbook workbook = application.Workbooks.Open(fileStream, ExcelOpenType.Automatic); IWorksheet worksheet = workbook.Worksheets[0]; AddExcelTable("Table1", worksheet.UsedRange); string fileName = "../../../Output/Table.xlsx"; //Saving the workbook as stream. FileStream stream = new FileStream(fileName, FileMode.Create, FileAccess.ReadWrite); workbook.SaveAs(stream); stream.Dispose(); } } /// <summary> /// Adds a table to the worksheet with the given name and range. /// </summary> /// <param name="tableName">Table name</param> /// <param name="tableRange">Table range</param> public void AddExcelTable(string tableName, IRange tableRange) { IWorksheet worksheet = tableRange.Worksheet; //Create a table with the data in a given range. IListObject table = worksheet.ListObjects.Create(tableName, tableRange); //Set the table style. table.BuiltInTableStyle = TableBuiltInStyles.TableStyleMedium14; } } }

Witness the possibilities in demos showcasing the robust features of Syncfusion’s C# Excel Library.
Refer to the following images.
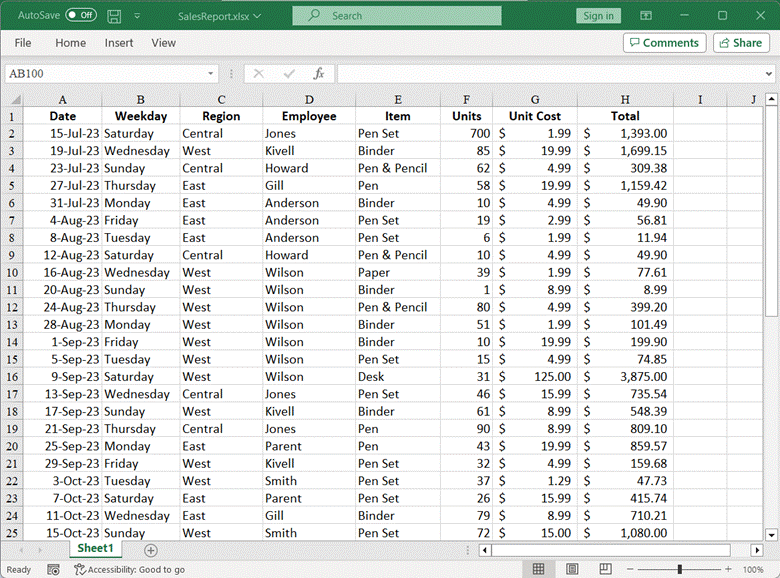
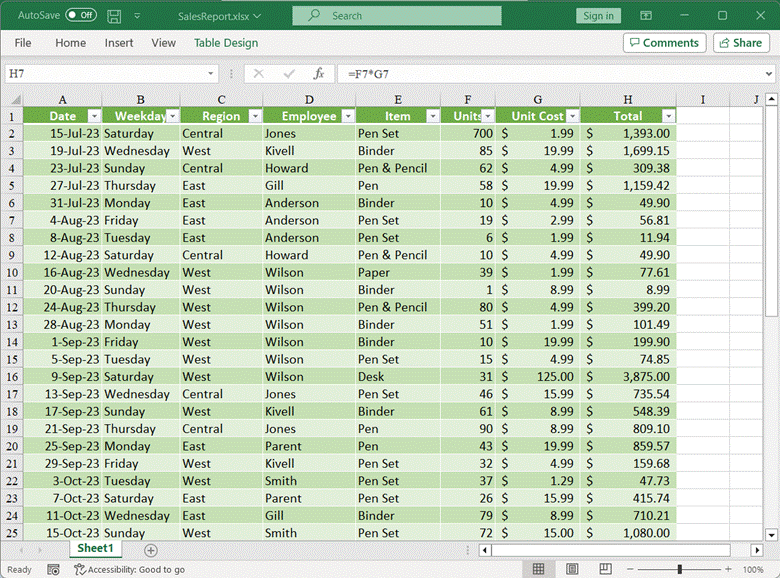
Note: For more details, refer to working with Excel table in C# documentation.
GitHub reference
You can also download the example for creating a table in Excel using C# on GitHub.

Don't settle for ordinary spreadsheet solutions. Switch to Syncfusion and upgrade the way you handle Excel files in your apps!
Conclusion
Thanks for reading! In this blog, we explored how to create tables in Excel documents using C# and Syncfusion Excel Library (XlsIO). The Excel Library also allows you to export Excel data to images, data tables, CSV, TSV, HTML, collections of objects, ODS, JSON, and other file formats.
Take a moment to peruse the import data documentation, where you’ll discover additional importing options and features such as data tables, collection objects, grid view, data columns, and HTML, all accompanied by code samples.
Feel free to try out this versatile .NET Excel Library and share your feedback in the comments section of this blog post!
For existing customers, the new version of Essential Studio is available for download from the License and Downloads page. If you are not yet a Syncfusion customer, you can try our 30-day free trial to check out our available features.
For questions, you can contact us through our support forum, support portal, or feedback portal. We are always happy to assist you!
Related blogs
- Print Excel Documents in Just 4 Steps Using C#
- Converting XLS to XLSX Format in Just 3 Steps Using C#
- Merge Multiple Excel Files into One in Just 3 Steps Using C#
- 6 Easy Ways to Export Data to Excel in C#
- Seamlessly Import and Export CSV Data in Excel Using C#
- Easy Steps to Export HTML Tables to Excel in C#