Splitting a PDF document is a common use case in PDF document manipulation. It helps reduce the PDF file size by breaking down large documents into smaller pieces to send them via email or any other form of digital distribution.
With the help of the Syncfusion PDF Library, you can easily split large PDF documents, and you can also automate the split function for batch processing.
In this blog, we are going to explore the following six ways in which a PDF document can be split:
- Split a PDF document into multiple files.
- Split specific pages into a separate PDF document.
- Split a range of pages into a separate PDF document.
- Split a PDF document based on PDF bookmarks.
- Find specific text in a page and split it into a separate PDF.
- Split a PDF document into multiple files with a specific number of pages.
Let’s get started!

Say goodbye to tedious PDF tasks and hello to effortless document processing with Syncfusion's PDF Library.
#1: Split a PDF document into multiple files
The primary need of splitting a PDF document is to reduce the file size of the document. For example, if an organization generates reports containing the paychecks for all employees, then they need to split the entire PDF file into individual files and email them separately to the respective employees.
We can easily split each page in a PDF document into individual PDF documents with the following two steps:
- Load the PDF document.
- Call the Split function with an output file pattern.
The following code example shows how to split an existing PDF document.
//Load PDF document PdfLoadedDocument document = new PdfLoadedDocument("PDF_Succinctly.pdf"); //Split PDF document with pattern document.Split("Document-{0}.pdf"); //Close the document document.Close(true);
By executing this code example, you will get individual PDF documents as shown in the following image.
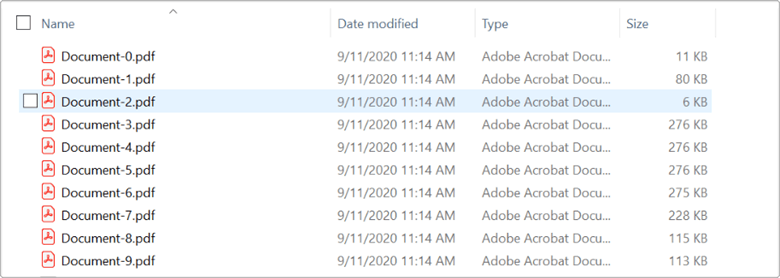
#2: Split specific pages into a separate PDF document
With a large PDF document, there may be occasions where only a single page needs to be reviewed by someone. In that case, it would be very effective to send that particular page instead of the complete document.
The following code example shows how to split a particular page from an existing PDF document.
//Load the PDF document PdfLoadedDocument loadedDocument = new PdfLoadedDocument("PDF_Succinctly.pdf"); //Create new PDF document PdfDocument document = new PdfDocument(); //Import the particular page from the existing PDF document.ImportPage(loadedDocument, 8); //Save the new PDF document document.Save("PDF_Succinctly8.pdf"); //Close the PDF document document.Close(true); loadedDocument.Close(true);
By executing this code, you will get a PDF document like the one in the following screenshot.
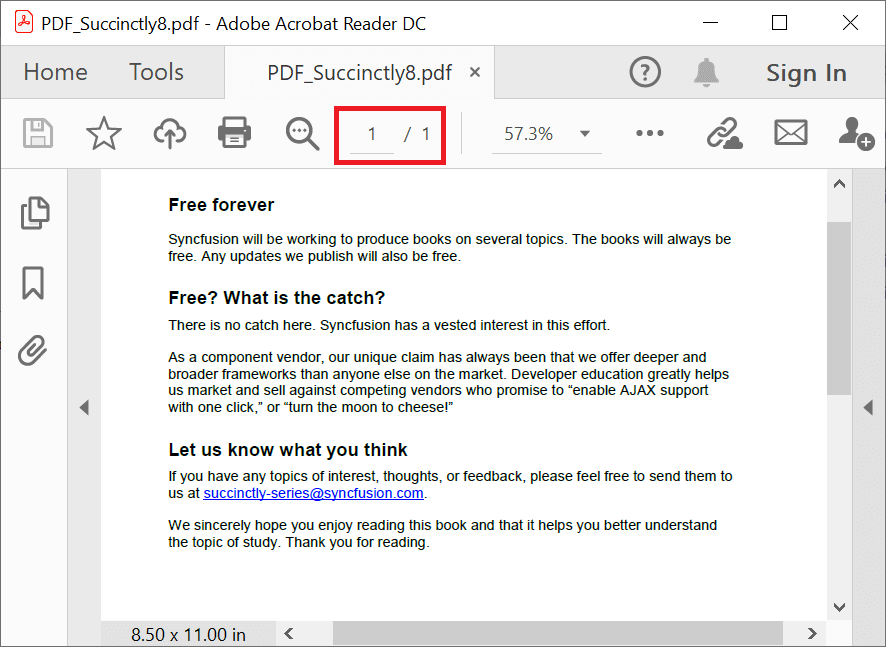

Explore the wide array of rich features in Syncfusion's PDF Library through step-by-step instructions and best practices.
#3: Split a range of pages into a separate PDF document
With the help of the Syncfusion PDF Library, you can split a range of pages from a large PDF document into an individual PDF. This will help the user send only the pages that need further processing, instead of sending the whole PDF document.
The following code example shows how to split a range of pages from an existing PDF document.
//Load the PDF document PdfLoadedDocument loadedDocument = new PdfLoadedDocument("PDF_Succinctly.pdf"); //Create new PDF document PdfDocument document = new PdfDocument(); //Import the range of pages from the existing PDF document.ImportPageRange(loadedDocument, 2,8); //Save the new PDF document document.Save("PDF_Succinctly.pdf"); //Close the PDF document document.Close(true); loadedDocument.Close(true);
By executing this code, you will get a PDF document with multiple pages as shown in the following screenshot.
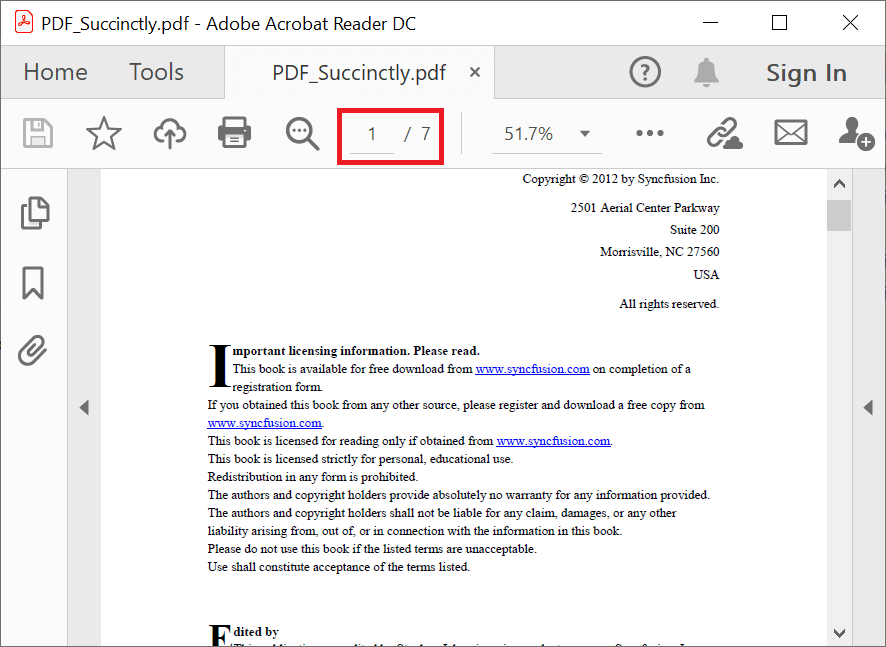
#4: Split a PDF document based on PDF bookmarks
A PDF document may contain bookmarks that indicate different sections. You can split a PDF document by sections using these bookmarks in cases where different sections of a PDF document should be reviewed by different people.
The following code example shows how to split a PDF document using a bookmark.
//Load the PDF document PdfLoadedDocument loadedDocument = new PdfLoadedDocument("PDF_Succinctly.pdf"); PdfBookmarkBase bookmarks = loadedDocument.Bookmarks; Dictionary<PdfPageBase, int> pages = new Dictionary<PdfPageBase, int>(); Dictionary<string, List<int>> splitRange = new Dictionary<string, List<int>>(); //Iterate all the pages and their indexes for (int i = 0; i < loadedDocument.Pages.Count; i++) { PdfPageBase page = loadedDocument.Pages[i] as PdfPageBase; pages.Add(page, i); } //Iterate all the bookmarks and their page ranges for (int i = 0; i < bookmarks.Count; i++) { if (bookmarks[i].Destination != null) { PdfPageBase page = bookmarks[i].Destination.Page; if (pages.ContainsKey(page)) { //Bookmark doesn't have any child bookmark if (bookmarks[i].Count == 0) { int startIndex = pages[page]; List<int> range = new List<int>(); range.Add(startIndex); range.Add(startIndex); splitRange.Add(bookmarks[i].Title, range); } //Bookmark has child bookmark else { int startIndex = pages[page]; int endIndex = startIndex; List<int> range = new List<int>(); foreach (PdfLoadedBookmark bookmark in bookmarks[i]) { PdfPageBase innerPage = bookmark.Destination.Page; if (pages.ContainsKey(innerPage)) { endIndex = pages[innerPage]; } } range.Add(startIndex); range.Add(endIndex); splitRange.Add(bookmarks[i].Title, range); } } } } //Split the PDF document based on the bookmark page range. foreach (string title in splitRange.Keys) { int startIndex = splitRange[title][0]; int endIndex = splitRange[title][1]; //Create a new PDF document. PdfDocument document = new PdfDocument(); //Import the pages to the new PDF document. document.ImportPageRange(loadedDocument, startIndex, endIndex); //Save the document in a specified folder. document.Save(title + ".pdf"); //Close the document. document.Close(true); } loadedDocument.Close(true);
On executing the code in this example, you will get individual PDF documents that each contain a different section of the original document, as shown in the following screenshot.
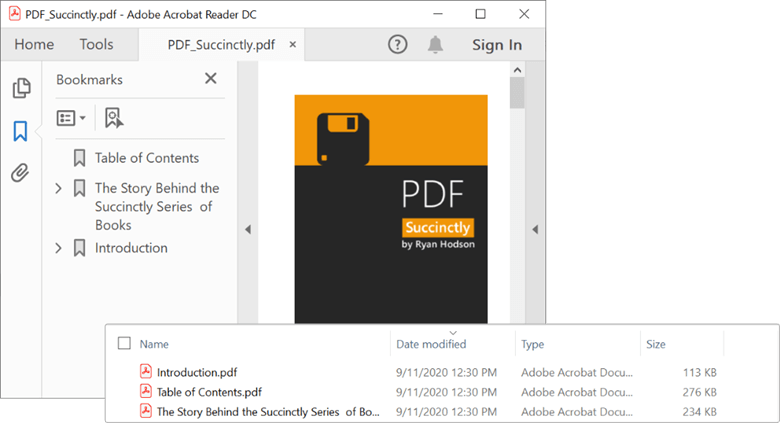

Witness the advanced capabilities of Syncfusion's PDF Library with feature showcases.
#5: Find a page with specific text and split it into a separate PDF
With the find text feature, you can split a particular page from a PDF based on the keyword present in the page. This will help you create a new document with the content you want.
The following code example shows how to split a PDF document using the find text feature.
//Load the PDF document PdfLoadedDocument loadedDocument = new PdfLoadedDocument("PDF_Succinctly.pdf"); Dictionary<int, List<RectangleF>> textFound = new Dictionary<int, List<RectangleF>>(); //Find the text in the PDF document loadedDocument.FindText("portable", out textFound); PdfDocument document = new PdfDocument(); //Import page based on the find text index foreach (int index in textFound.Keys) { if (textFound[index].Count > 0) { document.ImportPage(loadedDocument, index); } } //Save the PDF document document.Save("portable.pdf"); //Close the document document.Close(true); loadedDocument.Close(true);
In the above code example, we have searched for the text “portable” in the PDF. The text was found only in one page. That page has been split as a separate PDF file as shown in the following screenshot.
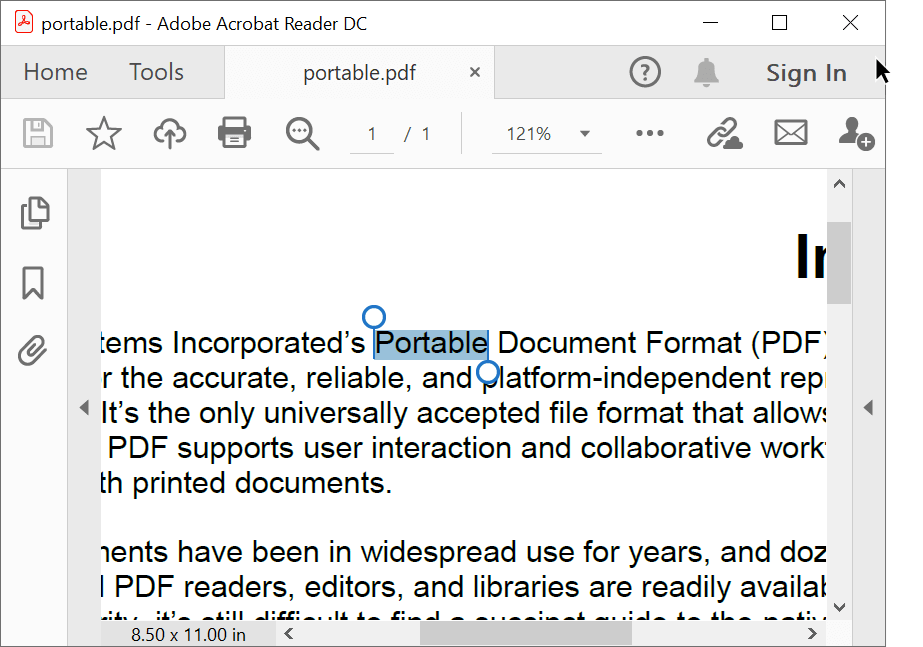
#6: Split a PDF into multiple documents with a specific number of pages:
You can split a large PDF document into multiple PDF files with any number of pages.
The following code sample shows how to split an existing PDF into multiple files with two pages each.
//Load the PDF document PdfLoadedDocument loadedDocument = new PdfLoadedDocument("PDF_Succinctly.pdf"); //Split the PDF documents so that each contains only 2 pages for (int i = 0; i < loadedDocument.Pages.Count; i = i + 2) { //Create new PDF document PdfDocument document = new PdfDocument(); //Import the particular page from the existing PDF document.ImportPageRange(loadedDocument, i,i+1); //Save the new PDF document document.Save("PDF_Succinctly-"+i+".pdf"); //Close the PDF document document.Close(true); } loadedDocument.Close(true);
By executing the example, you will get a list of PDF documents with two pages each.
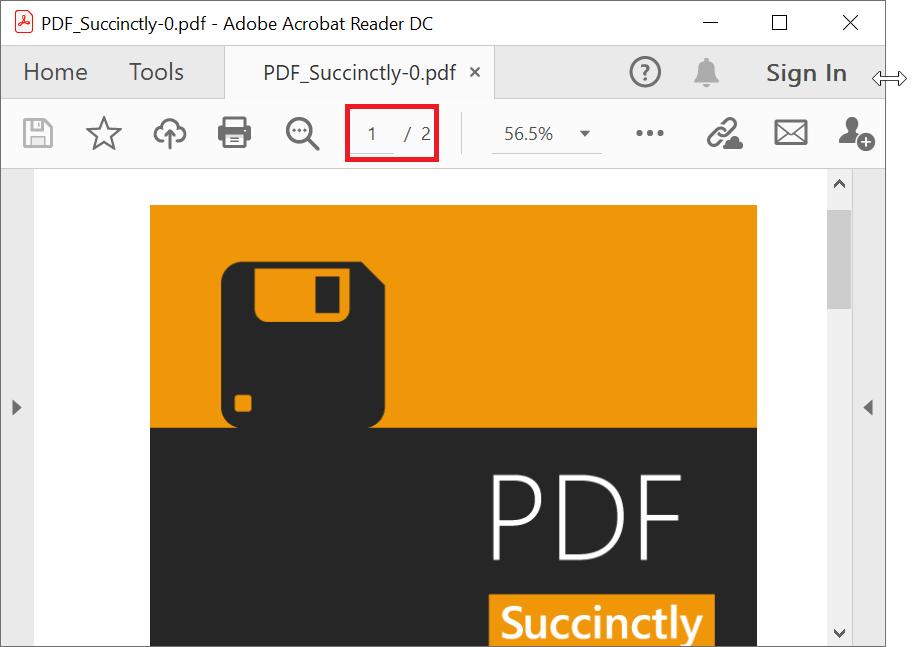
GitHub Sample
You can download all of these samples of splitting PDF documents on GitHub.

Syncfusion’s high-performance PDF Library allows you to create PDF documents from scratch without Adobe dependencies.
Conclusion
In this blog post, we learned six easy ways to split a PDF document using C#. I hope this blog post has been helpful to you. Splitting a PDF helps you reduce document file sizes and separate out your desired content. It also reduces the time spent on file transactions.
If you have any questions about these features, please let us know in the comments section below. You can also contact us through our support forums, Direct-Trac, or feedback portal. We are always happy to assist you!
If you like this article, we think you will also like the following articles about the Syncfusion PDF Library:
Comments (3)
Praveenkumar, the best!
Thanks Praveenkumar !
can we split one A4 into 2 pages A5 ?
Hi RICARDO,
At present, we do not have direct support for splitting the PDF document page into 2 pages in our PDF library. However, we can achieve your requirement by using PdfTemplate, we have created a sample to split the A4 document page into two A5 pages in a PDF document. We have attached the sample and output document for your reference, try the sample on your end and let us know whether it is suitable for you.
Sample: https://www.syncfusion.com/downloads/support/directtrac/general/ze/PdfSample-475580774
Document: https://www.syncfusion.com/downloads/support/directtrac/general/pd/TemplateOutput1-1397890039
You can refer to the below KB documentation link for more details,
KB: https://www.syncfusion.com/kb/9955/how-to-split-pdf-page-into-half-using-c-and-vb-net
Regards,
Chinnu M
Comments are closed.