In the following example, the cookie consent banner temple will display to notify you to accept cookies. Follow the below steps to create a consent cookie in Blazor.
1. Configure the HttpContextAccessor and CookiePolicyOptions to the Program.cs file to create a consent cookie.
[Program.cs]
builder.Services.Configure<CookiePolicyOptions>(options =>
{
options.CheckConsentNeeded = context => true;
options.MinimumSameSitePolicy = SameSiteMode.None;
});
builder.Services.AddHttpContextAccessor();
var app = builder.Build();
app.UseCookiePolicy();
2. Now, add the Cookie consent banner template as a Razor component under the Shared folder.
[ConsentCookie.razor]
@using Microsoft.AspNetCore.Http.Features
@using Microsoft.AspNetCore.Http
@inject IHttpContextAccessor Http
@inject IJSRuntime JSRuntime
@if (showBanner)
{
<div id="cookieConsent" class="alert alert-info alert-dismissible fade show" role="alert">
Consent to set cookies.
<button type="button" class="accept-policy close" data-dismiss="alert" aria-label="Close" data-cookie-string="@cookieString" @onclick="AcceptMessage">
Accept Cookie
</button>
</div>
}
@code {
ITrackingConsentFeature consentFeature;
bool showBanner;
string cookieString;
protected override void OnInitialized()
{
consentFeature = Http.HttpContext.Features.Get<ITrackingConsentFeature>();
showBanner = !consentFeature?.CanTrack ?? false;
cookieString = consentFeature?.CreateConsentCookie();
}
private void AcceptMessage()
{
// JsInterop call to store the consent cookies.
JSRuntime.InvokeVoidAsync("CookieFunction.acceptMessage", cookieString);
}
}
3. Add the JavaScript function in the _Layout.cshtml/_Host.cshtml/index.cshtml file to store the cookie.
[_Layout.cshtml]/_Host.cshtml/index.cshtml
<body>
. . .
. . .
<script>
window.CookieFunction = {
acceptMessage: function (cookieString) {
document.cookie = cookieString;
}
};
</script>
</body>
4. Refer to the cookie consent banner template Razor component in the MainLayout.razor file.
[MainLayout.razor]
<main>
<div class="top-row px-4">
<ConsentCookie />
</div>
<article class="content px-4">
@Body
</article>
</main>
5. Run the application, and you will find the consent cookie banner.
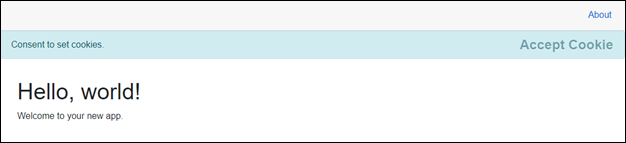
6. Now, click the Accept cookie button to store the cookie in the browser.

Share with