The Blazor File Manager is a graphical user interface component for managing the file system that allows users to perform the most common file operations like viewing, editing, and sorting files or folders. This component also provides easy navigation for browsing folders to select a file or folder from various file system providers like Amazon, Microsoft Azure, and more.
Previewing PDF and Word files using Blazor File Manager is impossible by default. However, this functionality can be added by integrating the Syncfusion Blazor PDF Viewer and Word Processor components.
Let’s get started!
Creating a Blazor Server app
Create a Blazor Server app by utilizing the Syncfusion Blazor Template Studio extension, and include the Blazor File Manager. You can refer to this documentation to begin working with the Blazor File Manager.
Note: You must include a valid license key (either paid or trial) within your applications. Refer to this help topic for more information.
Add the namespace @using Syncfusion.Blazor.FileManager in the _Imports.razor file and replace the following code in the Index.razor file:
@page "/" <PageTitle>Preview PDF and Word files using Blazor PDF Viewer and Word Processor in File Manager</PageTitle> <div class="control-region"> <SfFileManager TValue="FileManagerDirectoryContent" ShowThumbnail="true" AllowMultiSelection="false"> <FileManagerAjaxSettings Url="/api/FileManager/FileOperations" UploadUrl="/api/FileManager/Upload" DownloadUrl="/api/FileManager/Download" GetImageUrl="/api/FileManager/GetImage"> </FileManagerAjaxSettings> </SfFileManager> </div>
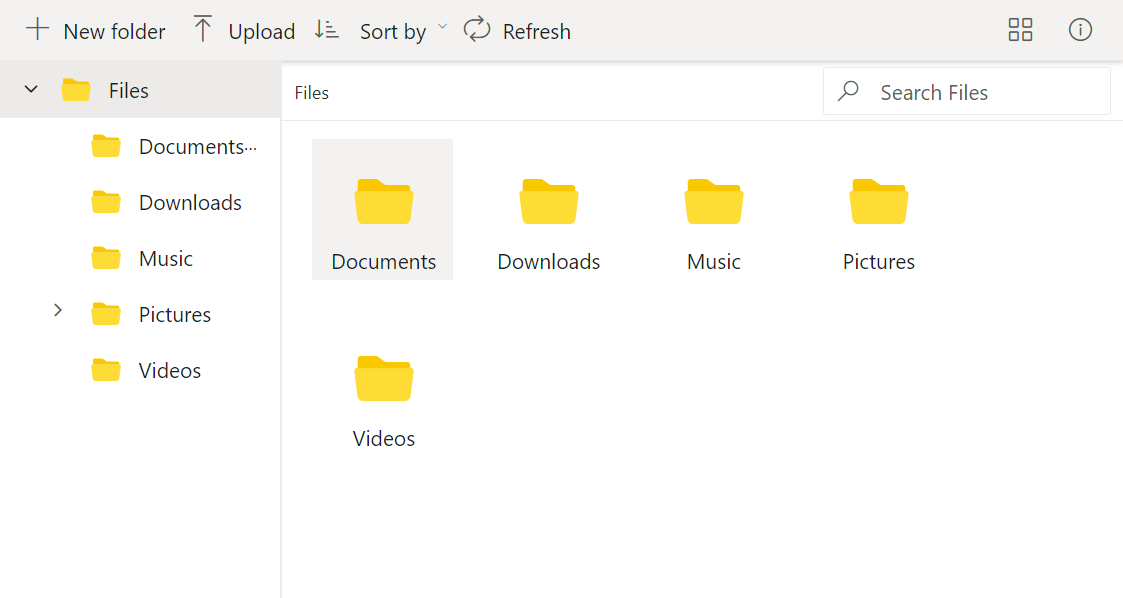
Implementing pop-up previews with Blazor Dialog
The Blazor Dialog component displays essential information, notifications, and prompts to users to confirm decisions and gather input. It can also be used to preview Word and PDF files in a pop-up window. To use this component, refer to its getting started documentation.
To open a modal dialog on opening a file, bind the OnFileOpen event in the FileManagerEvents component in Blazor File Manager.
<SfFileManager TValue="FileManagerDirectoryContent" ShowThumbnail="true" AllowMultiSelection="false"> <FileManagerAjaxSettings Url="/api/FileManager/FileOperations" UploadUrl="/api/FileManager/Upload" DownloadUrl="/api/FileManager/Download" GetImageUrl="/api/FileManager/GetImage"> </FileManagerAjaxSettings> <FileManagerEvents TValue="FileManagerDirectoryContent" OnFileOpen="OpenFilePreview"></FileManagerEvents> </SfFileManager> @code { private void OpenFilePreview(FileOpenEventArgs<FileManagerDirectoryContent> args) { } }
Render the dialog component by enabling the AllowDragging, EnableResize, and Visible properties. To prevent re-rendering the dialog’s content when closing, enable the AllowPrerender property. Additionally, include @using Syncfusion.Blazor.Popups in the Imports.razor component.
@page "/" <PageTitle>Preview PDF and Word files using Blazor PDF Viewer and Word Processor from File Manager</PageTitle> <div class="control-region"> @* File Manager component rendering section*@ <SfDialog Width="100%" Height="100%" EnableResize="true" AllowDragging="true" ShowCloseIcon="true" AllowPrerender="true" Visible="@IsDialogVisible"> <DialogTemplates> <Header> @DialogTitle </Header> <Content> @DialogContent </Content> </DialogTemplates> </SfDialog> </div> @code { private bool IsDialogVisible { get; set; } = true; private string DialogTitle { get; set; } = "Preview a File"; private string DialogContent { get; set; } = string.Empty; }
To render a dialog with 100% width and height, set the width and height of the dialog element and the body element of the HTML document to 100% using CSS. This can be done by adding the following CSS styles to your Index.razor component:
<style> html, body { width: 100%; height: 100%; } </style>
Integrating the Blazor PDF Viewer for PDF previews
The Blazor PDF Viewer is a UI component that allows you to display PDFs from byte arrays, streams, or file paths. To use the PDF Viewer, refer to this documentation. To specify the location of the PDF document, set the DocumentPath property in the PDF Viewer component. Additionally, create a PdfVisible variable to control the visibility of the PDF Viewer. Remember to include @using Syncfusion.Blazor.PdfViewerServer in the Imports.razor component to use the PDF Viewer component.
Note: Include the script file syncfusion-blazor-pdfviewer.min.js in the _Layout.cshtml page.
Use args.FileDetails.Type to obtain the PDF extension and dynamically change the document path of the PDF Viewer component. Set IsDialogVisible as false and PdfVisible and DocVisible as none to hide the Dialog, PDF Viewer, and Word Processor on initial loading.
@page "/" <PageTitle>Preview PDF and Word files using Blazor PDF Viewer and Word Processor in File Manager</PageTitle> <div class="control-region"> <SfFileManager TValue="FileManagerDirectoryContent" ShowThumbnail="true" AllowMultiSelection="false"> <FileManagerAjaxSettings Url="/api/FileManager/FileOperations" UploadUrl="/api/FileManager/Upload" DownloadUrl="/api/FileManager/Download" GetImageUrl="/api/FileManager/GetImage"> </FileManagerAjaxSettings> <FileManagerEvents TValue="FileManagerDirectoryContent" OnFileOpen="OpenFilePreview"></FileManagerEvents> </SfFileManager> <SfDialog Width="100%" Height="100%" EnableResize="true" AllowDragging="true" ShowCloseIcon="true" AllowPrerender="true" Visible="@IsDialogVisible"> <DialogTemplates> <Header> @DialogTitle </Header> <Content> <div style="display:@PdfVisible"> <SfPdfViewerServer DocumentPath="@DocumentPath" Height="500px" Width="1000px"></SfPdfViewerServer> </div> @DialogContent </Content> </DialogTemplates> </SfDialog> </div> @code { private bool IsDialogVisible { get; set; } = true; private string DialogTitle { get; set; } = "Preview a File"; private string DialogContent { get; set; } = string.Empty; private string DocumentPath { get; set; } = "filename.pdf"; private string PdfVisible { get; set; } = "block"; private void OpenFilePreview(FileOpenEventArgs<FileManagerDirectoryContent> args) { if (args.FileDetails.Type == ".pdf") { DialogTitle = "Preview PDF file: " + args.FileDetails.Name; PdfVisible = "block"; DocVisible = "none"; DialogContent = string.Empty; DocumentPath = "wwwroot\\Files" + args.FileDetails.FilterPath + args.FileDetails.Name; } } }
Integrating Blazor Word Processor to preview Word files
The Blazor Word Processor component is a tool that allows you to create, edit, view, and print Word documents. To use the Word Processor component, follow this documentation.
To open a Word document, define a @ref attribute to access the Word Processor instance. Additionally, create a DocVisible variable to control the visibility of the Word Processor. Remember to include @using Syncfusion.Blazor.DocumentEditor and @using System.Text.Json in the Imports.razor component to use the Word Processor component in your Blazor application.
Note: Be sure to include the syncfusion-blazor-documenteditor.min.js script file in the _Layout.cshtml page.
You can obtain a Word extension in args.FileDetails.Type of the OnFileOpen event. The code reads the Word file as a FileStream and converts it to a Word document. Then, it opens the document using the OpenAsync method of the Word Processor component.
@page "/" <PageTitle>Preview PDF and Word files using Blazor PDF Viewer and Word Processor in File Manager</PageTitle> <div class="control-region"> @* File Manager component rendering section*@ <SfDialog Width="100%" Height="100%" EnableResize="true" AllowDragging="true" ShowCloseIcon="true" AllowPrerender="true" Visible="@IsDialogVisible"> <DialogTemplates> <Header> @DialogTitle </Header> <Content> @* PDF Viewer component rendering section*@ <div style="display:@DocVisible"> <SfDocumentEditorContainer @ref="documentEditorContainer" Height="500px" Width="1000px" EnableToolbar="true"> </SfDocumentEditorContainer> </div> @DialogContent </Content> </DialogTemplates> </SfDialog> </div> @code { private bool IsDialogVisible { get; set; } = true; private string DialogTitle { get; set; } = "Preview a File"; private string DialogContent { get; set; } = string.Empty; private string DocumentPath { get; set; } = "filename.pdf"; private string PdfVisible { get; set; } = "block"; private string DocVisible { get; set; } = "block"; SfDocumentEditorContainer documentEditorContainer; private void OpenFilePreview(FileOpenEventArgs<FileManagerDirectoryContent> args) { if (args.FileDetails.Type == ".docx") { DialogTitle = "Preview Word file: " + args.FileDetails.Name; DocVisible = "block"; PdfVisible = "none"; DialogContent = string.Empty; this.OpenDocumentEditor("wwwroot\\Files" + args.FileDetails.FilterPath +
args.FileDetails.Name); } } public void OpenDocumentEditor(string filePath) { using (FileStream fileStream = new FileStream(filePath, System.IO.FileMode.Open, System.IO.FileAccess.Read)) { WordDocument document = WordDocument.Load(fileStream, ImportFormatType.Docx); string json = JsonSerializer.Serialize(document); document.Dispose(); document = null; documentEditorContainer.DocumentEditor.OpenAsync(json); json = null; } } }
Code sample and GitHub resources for implementation
The code sample for this functionality follows. For more information, refer to the complete code on GitHub.
@page "/" <PageTitle>Preview PDF and Word files using Blazor PDF Viewer and Word Processor in File Manager</PageTitle> <div class="control-region"> <SfFileManager TValue="FileManagerDirectoryContent" ShowThumbnail="true" AllowMultiSelection="false"> <FileManagerAjaxSettings Url="/api/FileManager/FileOperations" UploadUrl="/api/FileManager/Upload" DownloadUrl="/api/FileManager/Download" GetImageUrl="/api/FileManager/GetImage"> </FileManagerAjaxSettings> <FileManagerEvents TValue="FileManagerDirectoryContent" OnFileOpen="OpenFilePreview"></FileManagerEvents> </SfFileManager> <SfDialog Width="100%" Height="100%" EnableResize="true" AllowDragging="true" ShowCloseIcon="true" AllowPrerender="true" Visible="@IsDialogVisible"> <DialogTemplates> <Header> @DialogTitle </Header> <Content> <div style="display:@PdfVisible"> <SfPdfViewerServer DocumentPath="@DocumentPath" Height="500px" Width="1060px"></SfPdfViewerServer> </div> <div style="display:@DocVisible"> <SfDocumentEditorContainer @ref="documentEditorContainer" EnableToolbar="true"> </SfDocumentEditorContainer> </div> @DialogContent </Content> </DialogTemplates> </SfDialog> </div> @code { private string DocumentPath { get; set; } = string.Empty; private bool IsDialogVisible { get; set; } = false; private string PdfVisible { get; set; } = "none"; private string DocVisible { get; set; } = "none"; private string DialogTitle { get; set; } = "Preview a File"; private string DialogContent { get; set; } = string.Empty; SfDocumentEditorContainer documentEditorContainer; private void OpenFilePreview(FileOpenEventArgs<FileManagerDirectoryContent> args) { if (!string.IsNullOrEmpty(args.FileDetails.Type)) this.IsDialogVisible = true; else this.IsDialogVisible = false; if (args.FileDetails.Type == ".pdf") { DialogTitle = "Preview PDF file: " + args.FileDetails.Name; PdfVisible = "block"; DocVisible = "none"; DialogContent = string.Empty; DocumentPath = "wwwroot\\Files" + args.FileDetails.FilterPath + args.FileDetails.Name; } else if (args.FileDetails.Type == ".docx") { DialogTitle = "Preview Word file: " + args.FileDetails.Name; DocVisible = "block"; PdfVisible = "none"; DialogContent = string.Empty; this.OpenDocumentEditor("wwwroot\\Files" + args.FileDetails.FilterPath + args.FileDetails.Name); } else { DocumentPath = string.Empty; PdfVisible = "none"; DocVisible = "none"; DialogTitle = "Preview is unavailable"; DialogContent = "Preview is unavailable or not handled for this file type (" + args.FileDetails.Type + ")"; } } public void OpenDocumentEditor(string filePath) { using (FileStream fileStream = new FileStream(filePath, System.IO.FileMode.Open, System.IO.FileAccess.Read)) { WordDocument document = WordDocument.Load(fileStream, ImportFormatType.Docx); string json = JsonSerializer.Serialize(document); document.Dispose(); //To observe the memory, go down and null out the reference of the document variable. document = null; //editor = documentEditorContainer.DocumentEditor; documentEditorContainer.DocumentEditor.OpenAsync(json); //To observe the memory, go down and null out the reference of json variable. json = null; } } }
Conclusion
In this blog post, we discussed how to preview PDF and Word files in the Blazor File Manager using Blazor PDF Viewer and Word Processor components.
With Essential Studio for Blazor, you can access over 80 UI components and file-format libraries to build top-notch applications.
If you’re not yet a customer, you can download a free trial to test our Blazor components.
If you have any questions or concerns, please don’t hesitate to contact us through our support forums, support portal, or feedback portal. We are always available to assist you. Thank you for your time.