TLDR: Learn to export your grid data to an Excel document using the Syncfusion .NET MAUI DataGrid control with custom export options like excluding columns, exporting selected rows, and applying styles.
Syncfusion .NET MAUI DataGrid control is used to display and manipulate data in a tabular view. Its rich feature set includes different column types, sorting, autofit columns and rows, and styling all elements.
In this blog, we’ll guide you through the steps to export the .NET MAUI DataGrid to an Excel file with custom options!
Note: Please refer to the .NET MAUI DataGrid getting started documentation before proceeding.
Export DataGrid to Excel
The Syncfusion.Maui.DataGridExport NuGet package provides the required Excel exporting functionalities. Here, we will use our Syncfusion.XlsIO.NET packages to export the DataGrid to Excel.
To do so, include the Syncfusion.Maui.DataGridExport package in your application.
Then, add the .NET MAUI DataGrid control and an export button to your XAML page. Refer to the following code example.
<StackLayout> <HorizontalStackLayout Spacing="10"> <Button Text="Export To Excel" Margin="10,10,10,5" Clicked="ExportToExcel_Clicked" /> </HorizontalStackLayout> <syncfusion:SfDataGrid x:Name="dataGrid" Margin="20" VerticalOptions="FillAndExpand" ItemsSource="{Binding OrderInfoCollection}" GridLinesVisibility="Both" HeaderGridLinesVisibility="Both" AutoGenerateColumnsMode="None" ColumnWidthMode="Auto"> <syncfusion:SfDataGrid.Columns> <syncfusion:DataGridNumericColumn Format="D" HeaderText="Order ID" MappingName="OrderID"> </syncfusion:DataGridNumericColumn> <syncfusion:DataGridTextColumn HeaderText="Customer ID" MappingName="CustomerID"> </syncfusion:DataGridTextColumn> <syncfusion:DataGridTextColumn MappingName="Customer" HeaderText="Customer"> </syncfusion:DataGridTextColumn> <syncfusion:DataGridTextColumn HeaderText="Ship City" MappingName="ShipCity"> </syncfusion:DataGridTextColumn> <syncfusion:DataGridTextColumn HeaderText="Ship Country" MappingName="ShipCountry"> </syncfusion:DataGridTextColumn> </syncfusion:SfDataGrid.Columns> </syncfusion:SfDataGrid> </StackLayout>
All the Excel exporting methods are available in the DataGridExcelExportingController. Using the ExportToExcel method, you can export the DataGrid content to an Excel file and save it as a workbook.
Refer to the following code example.
private void ExportToExcel_Clicked(object sender, EventArgs e) { DataGridExcelExportingController excelExport = new DataGridExcelExportingController(); var excelEngine = excelExport.ExportToExcel(this.dataGrid); var workbook = excelEngine.Excel.Workbooks[0]; MemoryStream stream = new MemoryStream(); workbook.SaveAs(stream); workbook.Close(); excelEngine.Dispose(); string OutputFilename = "DefaultDataGrid.xlsx"; SaveService saveService = new(); saveService.SaveAndView(OutputFilename, "application/vnd.openxmlformats-officedocument.spreadsheetml.sheet", stream); }
To save the worksheet and view it at a specific location on your machine, you can use the SaveService class.
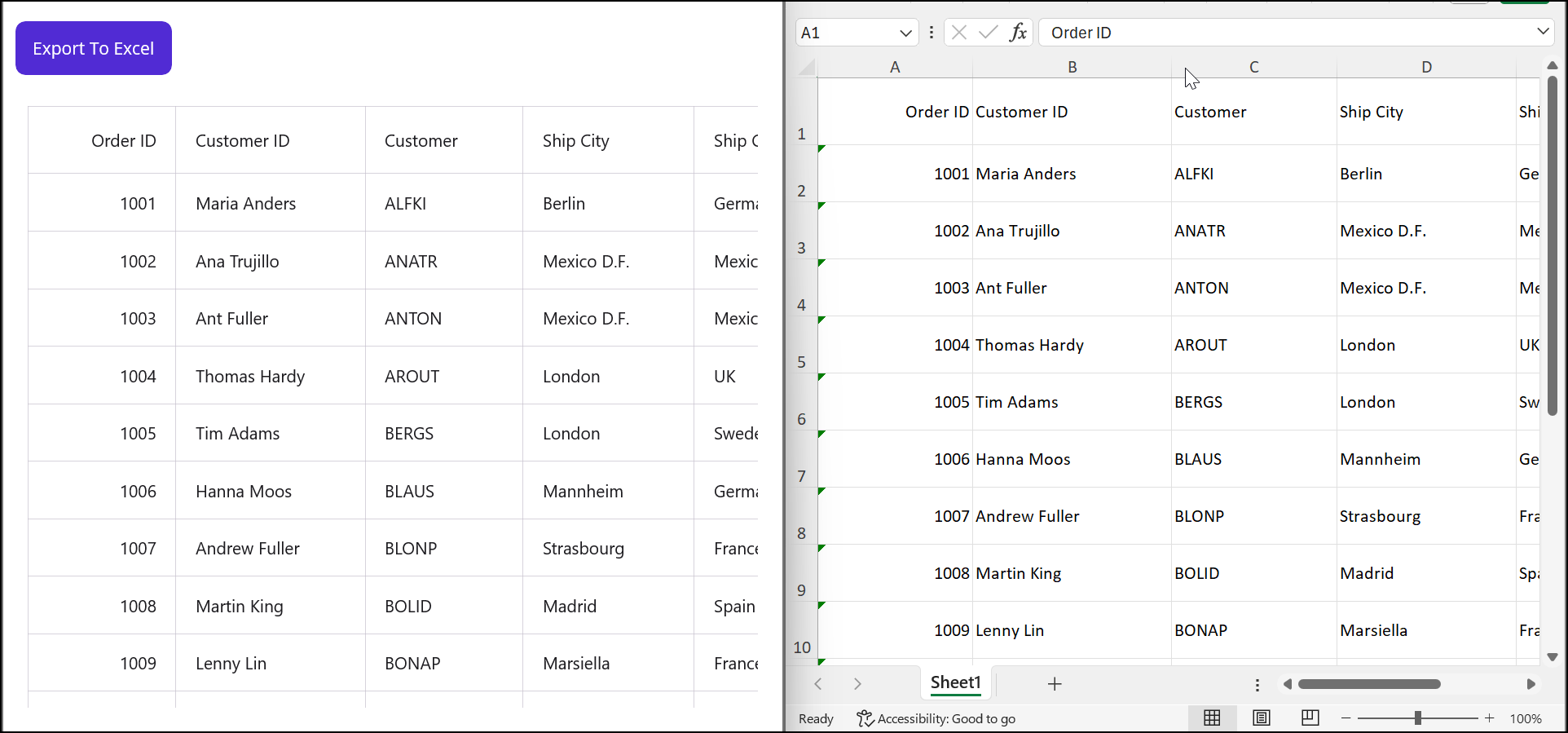
Excel exporting with customization
You can also customize the exported Excel sheet using the DataGridExcelExportingOption.
Export without header
The .NET MAUI DataGrid provides options to export grid data to Excel with or without a header. By default, the header will be included in the exported sheet.
Refer to the following code example.
private void ExportToExcel_Clicked(object sender, EventArgs e) { DataGridExcelExportingController excelExport = new DataGridExcelExportingController(); DataGridExcelExportingOption options = new DataGridExcelExportingOption(); options.CanExportHeader = false; var excelEngine = excelExport.ExportToExcel(this.dataGrid, options); var workbook = excelEngine.Excel.Workbooks[0]; MemoryStream stream = new MemoryStream(); workbook.SaveAs(stream); workbook.Close(); excelEngine.Dispose(); string OutputFilename = "DefaultDataGrid.xlsx"; SaveService saveService = new(); saveService.SaveAndView(OutputFilename, "application/vnd.openxmlformats-officedocument.spreadsheetml.sheet", stream); }
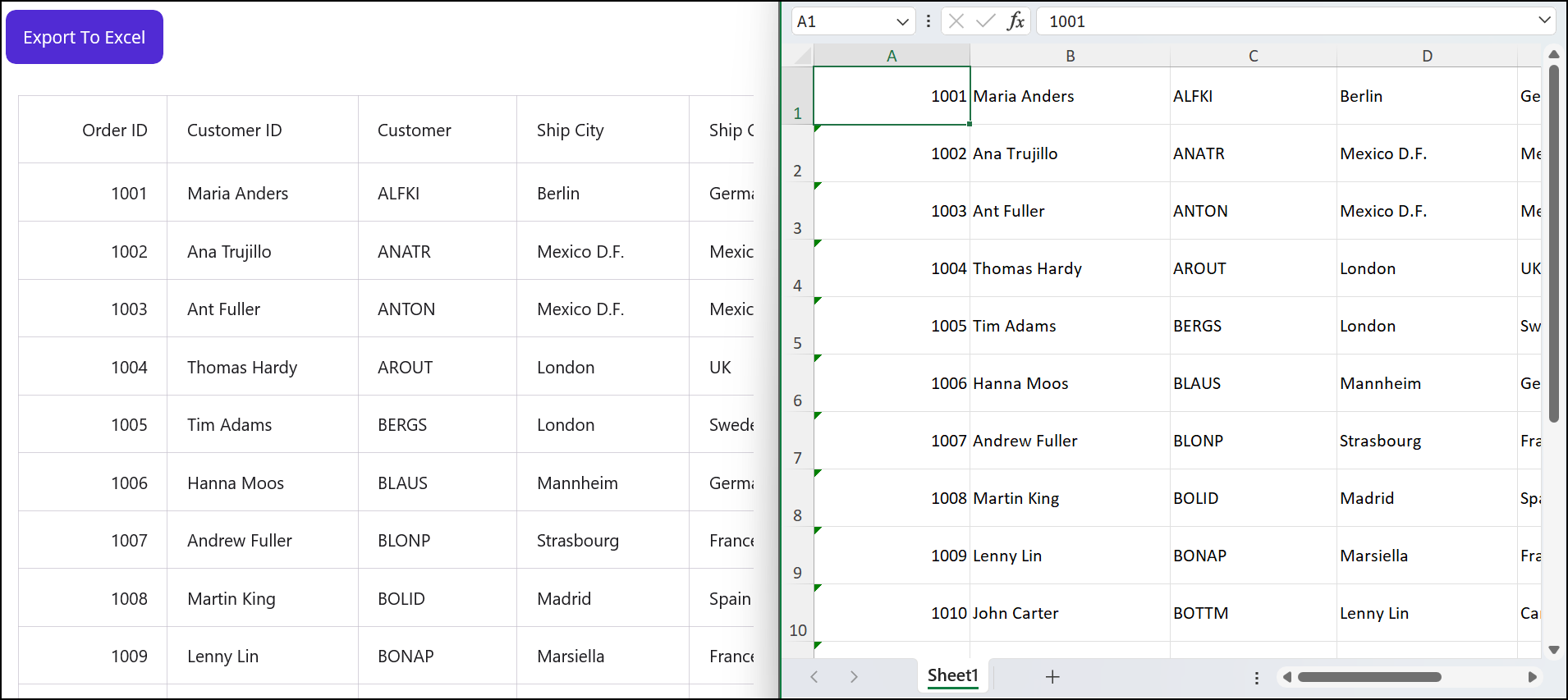
Exclude the columns in the exported Excel
You can exclude specific data columns in a grid from being exported to an Excel sheet. By default, all columns, including hidden ones, are exported.
Refer to the following code example.
private void ExportToExcel_Clicked(object sender, EventArgs e) { DataGridExcelExportingController excelExport = new DataGridExcelExportingController(); DataGridExcelExportingOption options = new DataGridExcelExportingOption(); var list = new List<string>(); list.Add("OrderID"); list.Add("CustomerID"); options.ExcludedColumns = list; var excelEngine = excelExport.ExportToExcel(this.dataGrid, options); var workbook = excelEngine.Excel.Workbooks[0]; MemoryStream stream = new MemoryStream(); workbook.SaveAs(stream); workbook.Close(); excelEngine.Dispose(); string OutputFilename = "DefaultDataGrid.xlsx"; SaveService saveService = new(); saveService.SaveAndView(OutputFilename, "application/vnd.openxmlformats-officedocument.spreadsheetml.sheet", stream); }
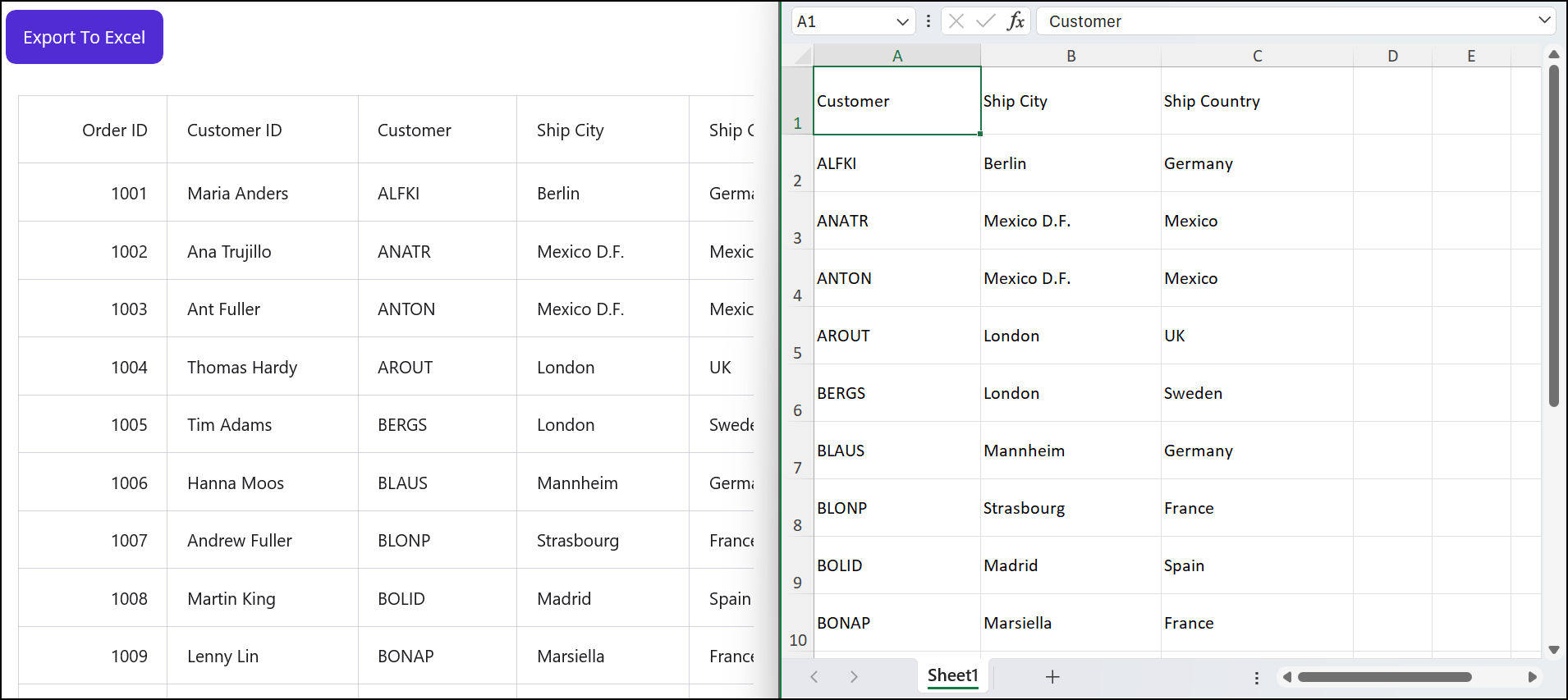
Export the selected rows
Let’s see how to export only the selected rows in a DataGrid by utilizing the ExportToExcel method and passing instances of the SelectedRows collection.
Refer to the following code example.
private void ExportToExcel_Clicked(object sender, EventArgs e) { DataGridExcelExportingController excelExport = new DataGridExcelExportingController(); ObservableCollection<object> selectedItems = dataGrid.SelectedRows; var excelEngine = excelExport.ExportToExcel(this.dataGrid, selectedItems); var workbook = excelEngine.Excel.Workbooks[0]; MemoryStream stream = new MemoryStream(); workbook.SaveAs(stream); workbook.Close(); excelEngine.Dispose(); string OutputFilename = "DefaultDataGrid.xlsx"; SaveService saveService = new(); saveService.SaveAndView(OutputFilename, "application/vnd.openxmlformats-officedocument.spreadsheetml.sheet", stream); }
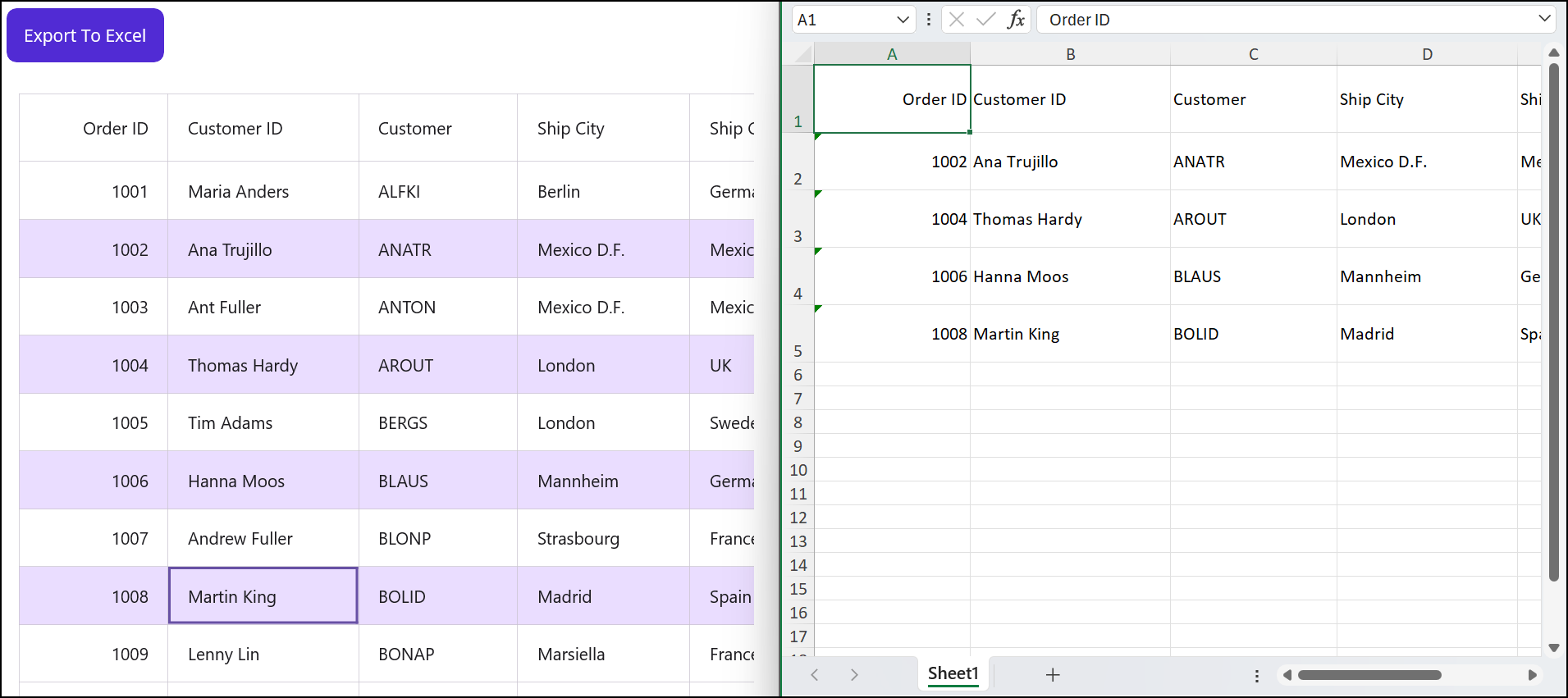
Customize the starting row and column in an Excel sheet
In the exporting option, you can specify the start column using the DataGridExcelExportingOption.StartColumnIndex and designate the start row using the DataGridExcelExportingOption.StartRowIndex.
Refer to the following code example.
private void ExportToExcel_Clicked(object sender, EventArgs e) { DataGridExcelExportingController excelExport = new DataGridExcelExportingController(); DataGridExcelExportingOption options = new DataGridExcelExportingOption(); options.StartRowIndex = 4; options.StartColumnIndex = 2; var excelEngine = excelExport.ExportToExcel(this.dataGrid, options); var workbook = excelEngine.Excel.Workbooks[0]; MemoryStream stream = new MemoryStream(); workbook.SaveAs(stream); workbook.Close(); excelEngine.Dispose(); string OutputFilename = "DefaultDataGrid.xlsx"; SaveService saveService = new(); saveService.SaveAndView(OutputFilename, "application/vnd.openxmlformats-officedocument.spreadsheetml.sheet", stream); }
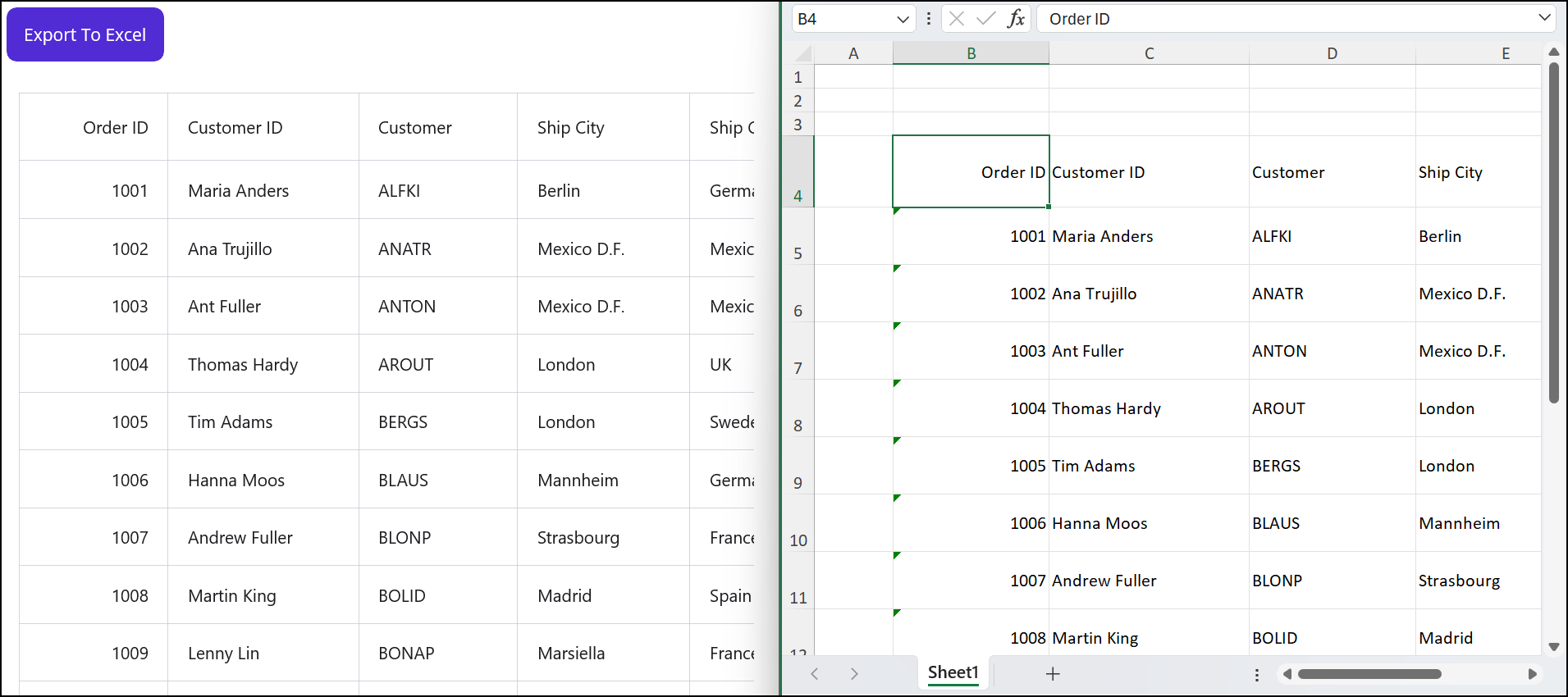
Apply styles for the exported sheet
You can also apply styles for the Excel sheet by utilizing the RowExporting and CellExporting events within the DataGridExcelExportingController.
Refer to the following code example.
private void ExportToExcel_Clicked(object sender, EventArgs e) { DataGridExcelExportingController excelExport = new DataGridExcelExportingController(); DataGridExcelExportingOption options = new DataGridExcelExportingOption(); excelExport.RowExporting += ExcelExport_RowExporting; var excelEngine = excelExport.ExportToExcel(this.dataGrid, options); var workbook = excelEngine.Excel.Workbooks[0]; MemoryStream stream = new MemoryStream(); workbook.SaveAs(stream); workbook.Close(); excelEngine.Dispose(); string OutputFilename = "DefaultDataGrid.xlsx"; SaveService saveService = new(); saveService.SaveAndView(OutputFilename, "application/vnd.openxmlformats-officedocument.spreadsheetml.sheet", stream); } private void ExcelExport_RowExporting(object? sender, DataGridRowExcelExportingEventArgs e) { if (!(e.Record.Data is OrderInfo)) return; if (e.RowType == ExportRowType.RecordRow) e.Range.CellStyle.ColorIndex = Syncfusion.XlsIO.ExcelKnownColors.Aqua; }
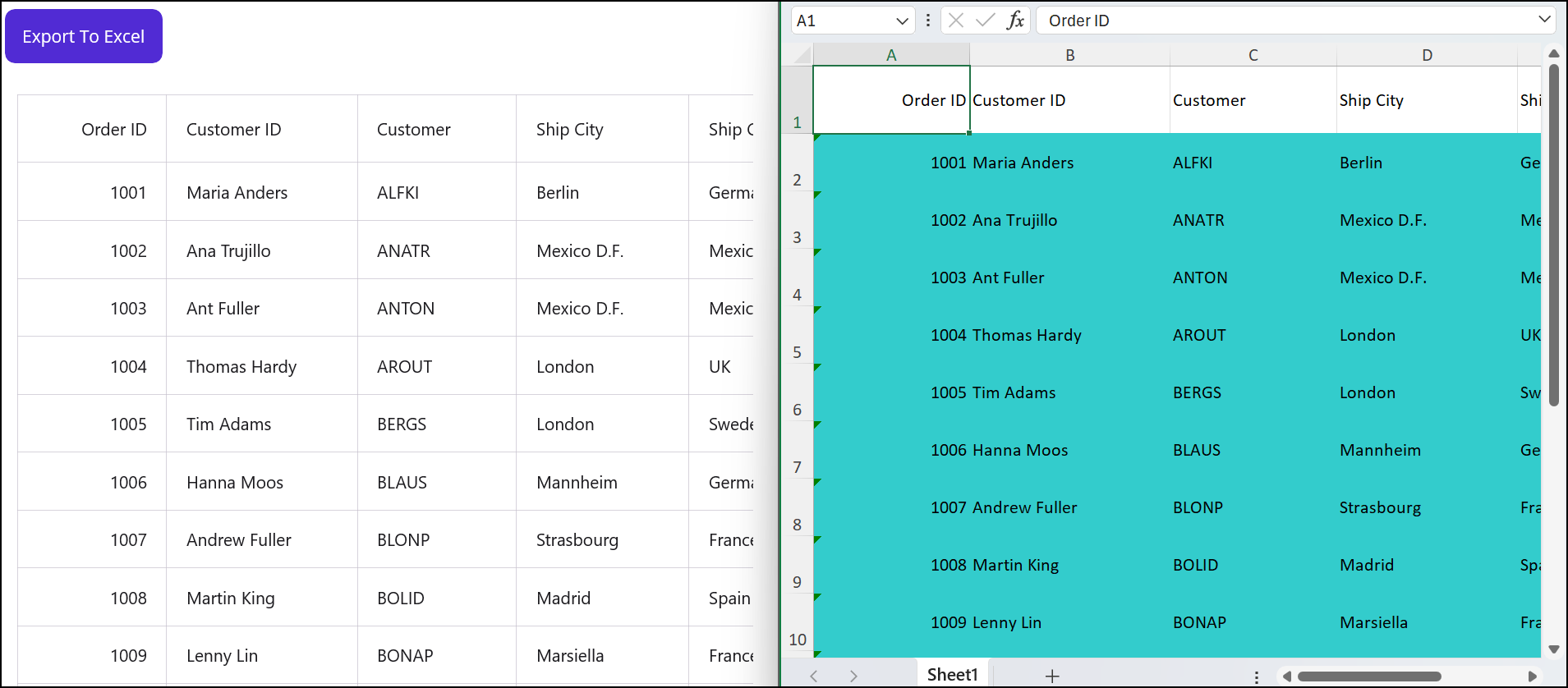
Reference
For more details, refer to Export .NET MAUI DataGrid to Excel documentation and GitHub demo.

Supercharge your cross-platform apps with Syncfusion's robust .NET MAUI controls.
Conclusion
Thanks for reading! This blog offers an extensive guide on efficiently exporting DataGrid to Excel in .NET MAUI, equipping developers with essential tools for a smooth process. We believe that the steps outlined here have been valuable and enlightening.
If you’re interested in delving deeper into .NET MAUI, you can easily access and evaluate it by downloading Essential Studio for .NET MAUI for free. Our esteemed customers can acquire the latest version of Essential Studio from the License and Downloads page.
Should you need any assistance or have further inquiries, contact us via our support forum, support portal, or feedback portal. We are committed to providing help and support in every possible way.