PDF forms allow users to input and submit data in a structured format. These forms are popular because they are easy to use and can be filled out electronically, reducing the need for paper and manual data entry. They can also be saved and shared electronically, making them convenient for individuals and organizations.
The Syncfusion .NET PDF Library provides comprehensive support for creating, customizing, and managing form fields in PDF documents.
This article will explore several use cases of PDF forms and how they can be implemented using the Syncfusion .NET PDF Library.
Agenda
- Create a new, fillable PDF form
- Fill form fields in a PDF
- Modify form fields in a PDF
- Remove form fields from a PDF
- Create a signature field and sign a PDF
- Restrict editing capability of form fields
- Import and export PDF forms data
- Retain extended rights of PDF forms
- Utilize JavaScript actions in PDF forms

Transform your PDF files effortlessly in C# with just five lines of code using Syncfusion's comprehensive PDF Library!
Create a new, fillable PDF form
Syncfusion’s .NET PDF Library allows users to easily create forms (Acroforms) in PDF documents with various form fields. You can include text boxes, combo boxes, radio buttons, list boxes, checkboxes, signature fields, buttons, and more in the PDF form.
Let’s create a simple form with a text box and radio button fields in a PDF document:
- First, create a new PDF document using the PdfDocument class.
- Add a new blank page using the PdfPage class.
- Create text box fields to collect the name, email address, and phone number using the PdfTextBoxField class.
- Then, create the radio button fields for gender selection using the PdfRadioButtonListField class and add the list items using the PdfRadioButtonListItem class.
- Finally, save the PDF document using the Save method.
The following code example shows how to create a simple form in a PDF document using C#.
//Create a new PDF document. PdfDocument document = new PdfDocument(); //Add a new page to the PDF document. PdfPage page = document.Pages.Add(); //Set the standard font. PdfFont font = new PdfStandardFont(PdfFontFamily.Helvetica, 16); //Draw the string. page.Graphics.DrawString("Job Application", font, PdfBrushes.Black, new PointF(250, 0)); font = new PdfStandardFont(PdfFontFamily.Helvetica, 12); page.Graphics.DrawString("Name", font, PdfBrushes.Black, new PointF(10, 20)); //Create a text box field for the name. PdfTextBoxField textBoxField1 = new PdfTextBoxField(page, "Name"); textBoxField1.Bounds = new RectangleF(10, 40, 200, 20); textBoxField1.ToolTip = "Name"; document.Form.Fields.Add(textBoxField1); page.Graphics.DrawString("Email address", font, PdfBrushes.Black, new PointF(10, 80)); //Create a text box field for the email address. PdfTextBoxField textBoxField3 = new PdfTextBoxField(page, "Email address"); textBoxField3.Bounds = new RectangleF(10, 100, 200, 20); textBoxField3.ToolTip = "Email address"; document.Form.Fields.Add(textBoxField3); page.Graphics.DrawString("Phone", font, PdfBrushes.Black, new PointF(10, 140)); //Create a text box field for the phone number. PdfTextBoxField textBoxField4 = new PdfTextBoxField(page, "Phone"); textBoxField4.Bounds = new RectangleF(10, 160, 200, 20); textBoxField4.ToolTip = "Phone"; document.Form.Fields.Add(textBoxField4); page.Graphics.DrawString("Gender", font, PdfBrushes.Black, new PointF(10, 200)); //Create a radio button for gender. PdfRadioButtonListField employeesRadioList = new PdfRadioButtonListField(page, "Gender"); document.Form.Fields.Add(employeesRadioList); page.Graphics.DrawString("Male", font, PdfBrushes.Black, new PointF(40, 220)); PdfRadioButtonListItem radioButtonItem1 = new PdfRadioButtonListItem("Male"); radioButtonItem1.Bounds = new RectangleF(10, 220, 20, 20); page.Graphics.DrawString("Female", font, PdfBrushes.Black, new PointF(140, 220)); PdfRadioButtonListItem radioButtonItem2 = new PdfRadioButtonListItem("Female"); radioButtonItem2.Bounds = new RectangleF(110, 220, 20, 20); employeesRadioList.Items.Add(radioButtonItem1); employeesRadioList.Items.Add(radioButtonItem2); //Create file stream. using (FileStream outputFileStream = new FileStream("Output.pdf", FileMode.Create, FileAccess.ReadWrite)) { //Save the PDF document to file stream. document.Save(outputFileStream); } //Close the document. document.Close(true);
Running this code example will generate a PDF that closely resembles the image displayed below.
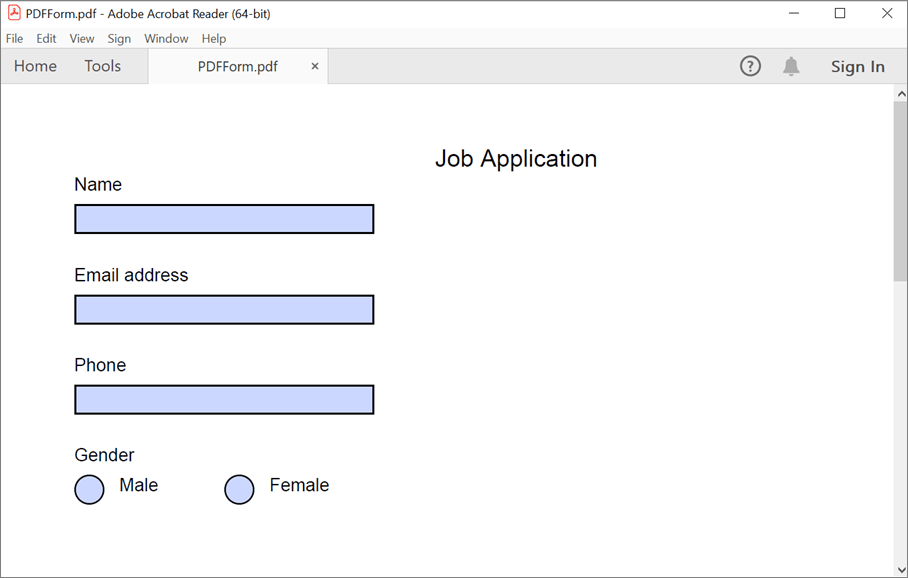

Say goodbye to tedious PDF tasks and hello to effortless document processing with Syncfusion's PDF Library.
Fill form fields in a PDF
We can use the TryGetField method to get a form field from an existing document using the field name. This method helps us determine the field’s availability within the form by returning a Boolean value.
Follow these steps to fill in the form fields in a PDF document:
- Use the PdfLoadedDocument class to load the created PDF document.
- Get the form from the PDF using the PdfLoadedForm class.
- Now, get the form field collections using the PdfLoadedFormFieldCollection class.
- Use the TryGetField method to obtain the form fields, such as text boxes and checkboxes, and then fill them with the necessary information.
- Finally, save the filled PDF document using the Save method.
The following code example shows how to fill form fields in a PDF document.
//Load the PDF document. FileStream docStream = new FileStream("Input.pdf", FileMode.Open, FileAccess.Read); PdfLoadedDocument loadedDocument = new PdfLoadedDocument(docStream); //Load the form from the loaded PDF document. PdfLoadedForm form = loadedDocument.Form; //Load the form field collections from the form. PdfLoadedFormFieldCollection fieldCollection = form.Fields as PdfLoadedFormFieldCollection; PdfLoadedField loadedField = null; //Get and fill the field using TryGetField Methodmethod. if (fieldCollection.TryGetField("Name", out loadedField)) { (loadedField as PdfLoadedTextBoxField).Text = "Simons"; } if (fieldCollection.TryGetField("Email address", out loadedField)) { (loadedField as PdfLoadedTextBoxField).Text = "simonsbistro@outlook.com"; } if (fieldCollection.TryGetField("Phone", out loadedField)) { (loadedField as PdfLoadedTextBoxField).Text = "31 12 34 56"; } if (fieldCollection.TryGetField("Gender", out loadedField)) { (loadedField as PdfLoadedRadioButtonListField).SelectedIndex = 0; } //Create file stream. using (FileStream outputFileStream = new FileStream("Output.pdf", FileMode.Create, FileAccess.ReadWrite)) { //Save the PDF document to file stream. loadedDocument.Save(outputFileStream); } //Close the document. loadedDocument.Close(true);
This code generates a pre-filled PDF with your designated form field values.
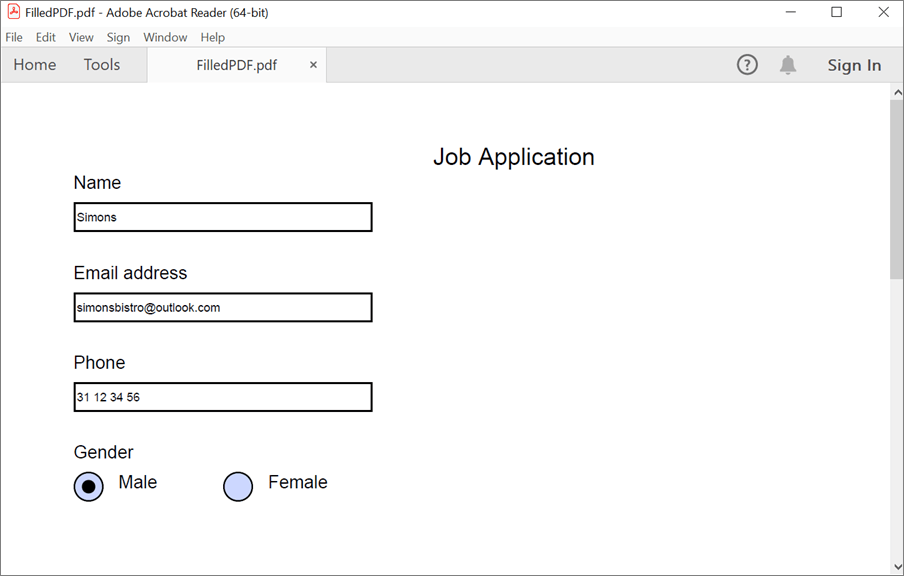
Note: The specific layout and design of the filled PDF document may vary based on the original PDF document and the values set for the form fields.
Modifying a form field in a PDF
You can also modify an existing form field and its properties like bounds, text, color, and border. To do so, please follow these steps:
- Use the PdfLoadedDocument class to load an existing PDF document.
- Get the existing form in a PDF using the PdfLoadedForm class.
- Get the required form fields from the PdfFormFieldCollection.
- Modify the text box form field properties.
- Finally, save the PDF document using the Save method.
The following code example illustrates how to modify an existing form field in a PDF document.
//Load the PDF document. FileStream docStream = new FileStream("Input.pdf", FileMode.Open, FileAccess.Read); PdfLoadedDocument loadedDocument = new PdfLoadedDocument(docStream); //Get the loaded form. PdfLoadedForm loadedForm = loadedDocument.Form; //Get the loaded form field and modify the properties. PdfLoadedTextBoxField loadedTextBoxField = loadedForm.Fields[0] as PdfLoadedTextBoxField; RectangleF newBounds = new RectangleF(200, 60, 300, 30); loadedTextBoxField.Bounds = newBounds; loadedTextBoxField.Text = "John"; loadedTextBoxField.BackColor = Color.LightYellow; loadedTextBoxField.BorderColor = Color.Red; //Create file stream. using (FileStream outputFileStream = new FileStream("Output.pdf", FileMode.Create, FileAccess.ReadWrite)) { //Save the PDF document to file stream. loadedDocument.Save(outputFileStream); } //Close the document. loadedDocument.Close(true);
By executing this code example, we will get a PDF like the following image.
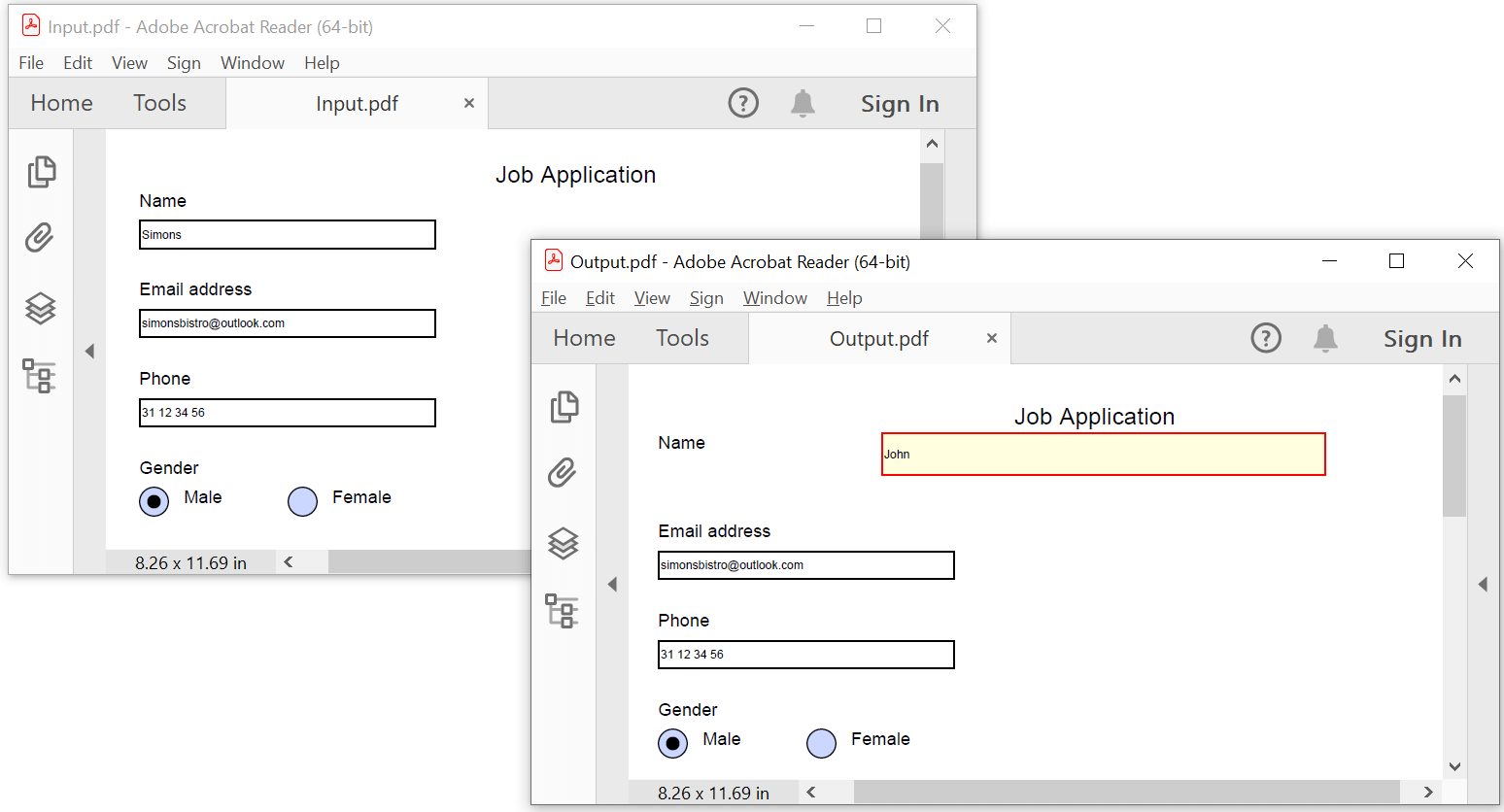
Removing form fields from a PDF
You can easily remove form fields from a PDF document by following these steps:
- Use the PdfLoadedDocument class to load a PDF document.
- Get the form using the PdfLoadedForm class.
- Now, remove the required form field from the index using the RemoveAt method or specify the field name in the Remove method of the PdfFieldCollection class.
- Then, save the PDF document using the Save method.
The following code illustrates how to remove form fields from a PDF document.
//Load the PDF document. FileStream docStream = new FileStream("Input.pdf", FileMode.Open, FileAccess.Read); PdfLoadedDocument loadedDocument = new PdfLoadedDocument(docStream); //Get the loaded form. PdfLoadedForm loadedForm = loadedDocument.Form; //Load the text box field. PdfLoadedTextBoxField loadedTextBoxField = loadedForm.Fields[2] as PdfLoadedTextBoxField; //Remove the field. loadedForm.Fields.Remove(loadedTextBoxField); //Remove the field at index 1. loadedForm.Fields.RemoveAt(1); //Create file stream. using (FileStream outputFileStream = new FileStream("Output.pdf", FileMode.Create, FileAccess.ReadWrite)) { //Save the PDF document to file stream. loadedDocument.Save(outputFileStream); } //Close the document. loadedDocument.Close(true);
By executing this code example, we will get the PDF in the following image.
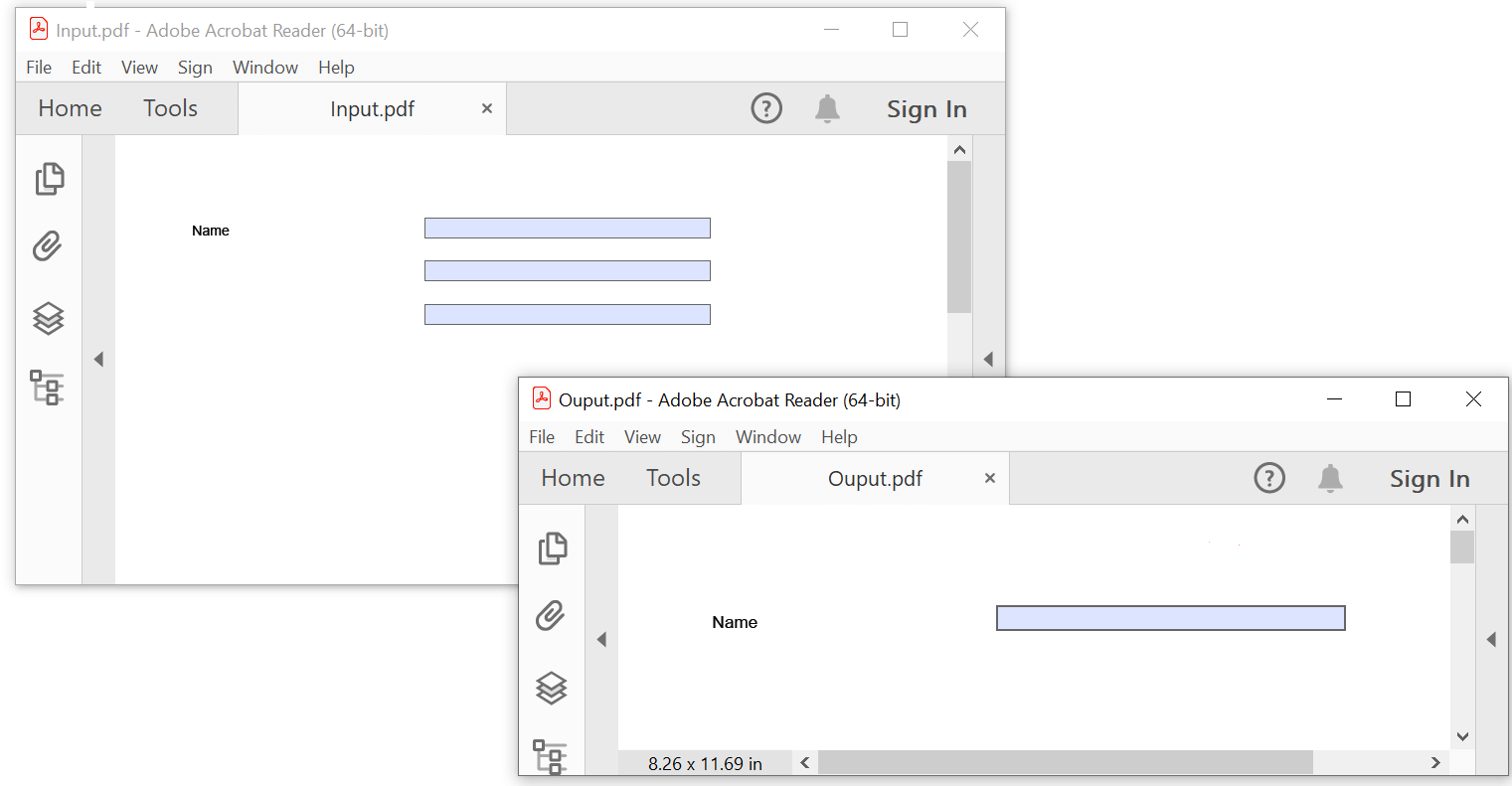

Explore the wide array of rich features in Syncfusion's PDF Library through step-by-step instructions and best practices.
Create a signature field and sign the PDF document
The Syncfusion .NET PDF Library helps create signature fields for signing PDF documents with ease.
Adding a signature field to a PDF
Follow these steps to create a signature field in a PDF document using Syncfusion’s .NET PDF Library:
- Create a new PDF document using the PdfDocument class.
- Add a new blank page using the PdfPage class.
- Create a new signature field using the PdfSignatureField class.
- Save the PDF document using the Save method.
The following code example illustrates creating a signature field in a new PDF document.
//Create a new PDF document. PdfDocument document = new PdfDocument(); //Add a new page to the PDF document. PdfPage page = document.Pages.Add(); //Create a PDF sSignature field. PdfSignatureField signatureField = new PdfSignatureField(page, "Signature"); //Set properties to the signature field. signatureField.Bounds = new RectangleF(0, 100, 90, 20); signatureField.ToolTip = "Signature"; //Add the form field to the document. document.Form.Fields.Add(signatureField); //Create file stream. using (FileStream outputFileStream = new FileStream("Output.pdf", FileMode.Create, FileAccess.ReadWrite)) { //Save the PDF document to file stream. document.Save(outputFileStream); } //Close the document. document.Close(true);
By executing this code example, you will get a PDF like in the following image.
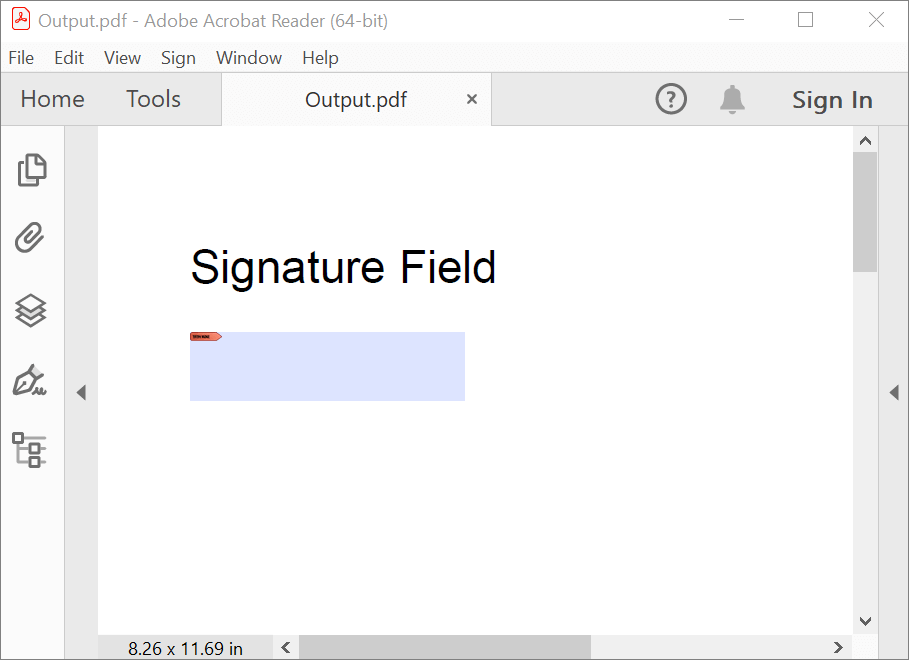
Digitally sign the PDF document
For how to digitally sign a PDF and verify signatures, refer to this blog.
Restrict editing capabilities of form fields
PDF forms are an excellent way to gather information from users. However, you may want to restrict the editing capability of all or certain form fields to prevent unintended modifications. This can be done by flattening the PDF document or marking the form or field as read-only.
Flattening form fields
The Syncfusion .NET PDF Library supports flattening a specific form field or entire form by removing the existing form field and replacing it with graphical objects that resemble the form field but cannot be edited.
Here are the steps to flatten the form fields in a PDF document:
- Use the PdfLoadedDocument class to load a PDF document.
- Get the form using the PdfLoadedForm class.
- Get the form field collections using the PdfLoadedFormFieldCollection class.
- Now, flatten the whole form using the Flatten property.
- Finally, save the PDF document using the Save method.
The following code example illustrates how to flatten the form fields in a PDF document.
//Load the PDF document. FileStream docStream = new FileStream("Input.pdf", FileMode.Open, FileAccess.Read); PdfLoadedDocument loadedDocument = new PdfLoadedDocument(docStream); //Get the loaded form. PdfLoadedForm loadedForm = loadedDocument.Form; PdfLoadedFormFieldCollection fields = loadedForm.Fields; //Flatten the whole form. loadedForm.Flatten = true; //Create file stream. using (FileStream outputFileStream = new FileStream("Output.pdf", FileMode.Create, FileAccess.ReadWrite)) { //Save the PDF document to file stream. loadedDocument.Save(outputFileStream); } //Close the document. loadedDocument.Close(true);
Marking the PDF form read-only
We can restrict the editing of a PDF form by marking it as read-only:
- Use the PdfLoadedDocument class to load a PDF document.
- Get the form using the PdfLoadedForm class.
- Set the form to read-only using the ReadOnly property.
- Then, save the PDF document using the Save method.
Refer to the following code example.
//Load the PDF document. FileStream docStream = new FileStream("Input.pdf", FileMode.Open, FileAccess.Read); PdfLoadedDocument loadedDocument = new PdfLoadedDocument(docStream); //Get the loaded form. PdfLoadedForm loadedForm = loadedDocument.Form; //Set the form as read-only. loadedForm.ReadOnly = true; //Create file stream. using (FileStream outputFileStream = new FileStream("Output.pdf", FileMode.Create, FileAccess.ReadWrite)) { //Save the PDF document to file stream. loadedDocument.Save(outputFileStream); } //Close the document. loadedDocument.Close(true);
By executing this code example, you will get a PDF like in the following image.
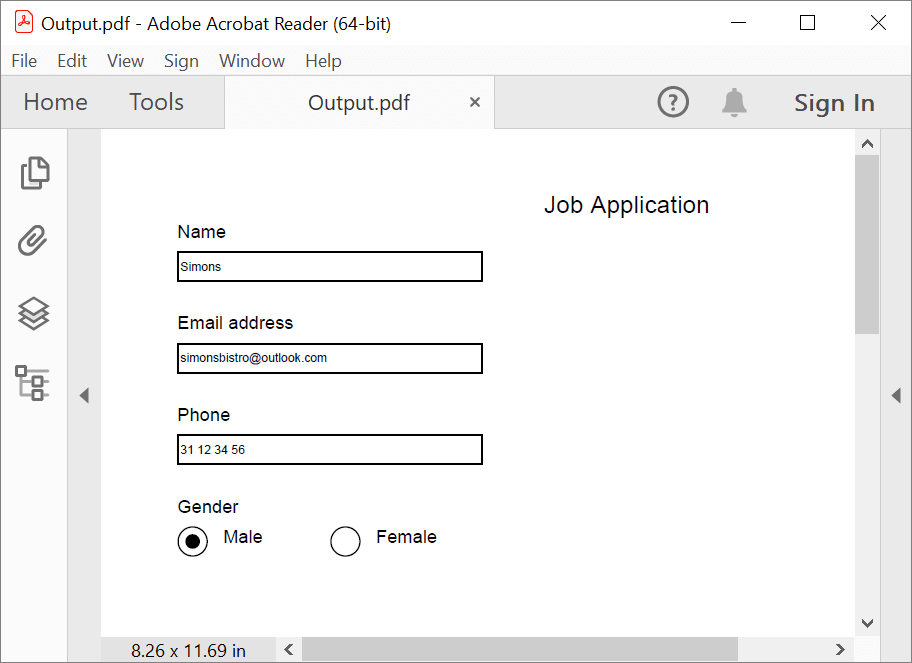

Witness the advanced capabilities of Syncfusion's PDF Library with feature showcases.
Import and export PDF form data
PDF forms are commonly used to collect data from users. Once the data is collected, it may need to be exported or imported for further analysis or processing. The Syncfusion .NET PDF Library supports importing and exporting PDF form data in various formats such as FDF, XFDF, JSON, and XML. This makes it easy to integrate form data with other apps and systems.
Import form field data to PDF
The following steps illustrate how to import form field data to a PDF document:
- Use the PdfLoadedDocument class to load a PDF document.
- Get the form in the PDF using the PdfLoadedForm class
- Import the form field data from an FDF file to the PDF using the ImportDataFDF method.
- Finally, save the modified PDF document using the Save method.
Refer to the following code example.
//Load the PDF document. FileStream docStream = new FileStream("Input.pdf", FileMode.Open, FileAccess.Read); PdfLoadedDocument loadedDocument = new PdfLoadedDocument(docStream); //Load the existing form. PdfLoadedForm loadedForm = loadedDocument.Form; //Load the FDF file. FileStream fileStream = new FileStream("Input.fdf", FileMode.Open, FileAccess.Read); //Import the FDF stream. loadedForm.ImportDataFDF(fileStream, true); //Create file stream. using (FileStream outputFileStream = new FileStream("Output.pdf", FileMode.Create, FileAccess.ReadWrite)) { //Save the PDF document to file stream. loadedDocument.Save(outputFileStream); } //Close the document. loadedDocument.Close(true);
By executing this code example, you will get a PDF like in the following image.
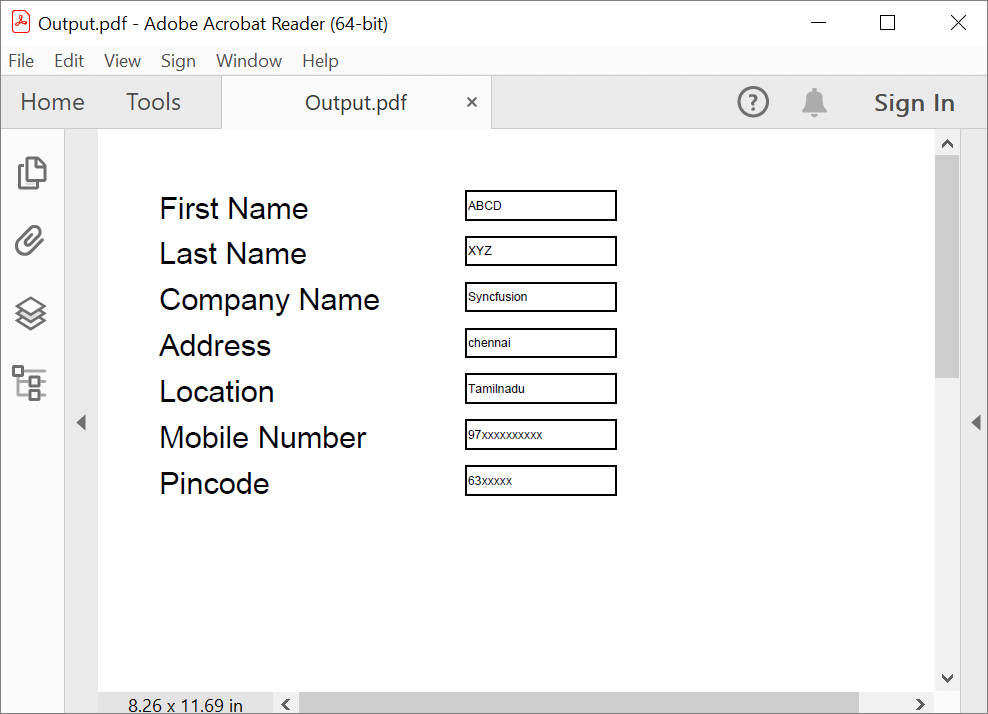
Export form field data from PDF
The following steps illustrate how to export PDF form field data to an XML file:
- Use the PdfLoadedDocument class to load a PDF document.
- Get the form from the PDF using the PdfLoadedForm class.
- Export the PDF form field data to an XML file using the ExportData You can specify the format of the exported data using the DataFormat enum.
- Save the modified PDF document using the Save method.
Refer to the following code example.
//Load the PDF document. PdfLoadedDocument loadedDocument = new PdfLoadedDocument(docStream); //Load an existing form. PdfLoadedForm loadedForm = loadedDocument.Form; //Create memory stream. MemoryStream ms = new MemoryStream(); //Create the XML file. FileStream stream = new FileStream("Export.xml", FileMode.Create, FileAccess.ReadWrite); //Export the existing PDF form fields data to the XML file. loadedForm.ExportData(stream, DataFormat.Xml, "AcroForm1"); //Close the document. loadedDocument.Close(true);
By executing this code example, you will get XML like in the following image.
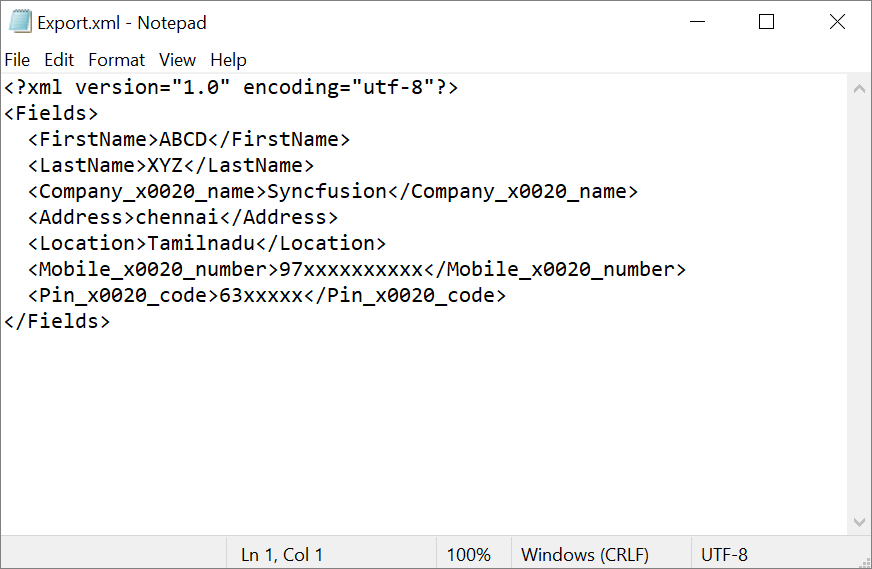
Retain extended rights of PDF forms
Extended features in a PDF form refer to additional capabilities that are not part of the standard PDF specification. These features allow users to do more with the form than just filling out fields and submitting data.
The Syncfusion .NET PDF Library supports preserving extended rights in PDF forms when performing actions like filling the form fields.
You can fill the reader-extended PDF document by following these steps:
- Use the PdfLoadedDocument class to load the extended featured PDF document.
- Get the form from the PDF using the PdfLoadedForm class.
- Get the form field collections using the PdfLoadedFormFieldCollection class.
- Use the TryGetField method to obtain the First Name, Last Name, and Email address form fields and then fill them with the necessary information.
- Finally, save the PDF document (Extended rights preserved) using the Save method.
Refer to the following code example.
//Load a PDF. PdfLoadedDocument loadedDocument = new PdfLoadedDocument(docStream); //Load the form from the loaded document. PdfLoadedForm loadedForm = loadedDocument.Form; loadedForm.SetDefaultAppearance(false); //Load the form field collections from the form. PdfLoadedFormFieldCollection fieldCollection = loadedForm.Fields as PdfLoadedFormFieldCollection; PdfLoadedField loadedField = null; //Get the field using TryGetField method. if (fieldCollection.TryGetField("First Name", out loadedField)) { (loadedField as PdfLoadedTextBoxField).Text = "Simons"; } if (fieldCollection.TryGetField("Last Name", out loadedField)) { (loadedField as PdfLoadedTextBoxField).Text = "Bistro"; } if (fieldCollection.TryGetField("Email Address", out loadedField)) { (loadedField as PdfLoadedTextBoxField).Text = "simonsbistro@outlook.com"; } //Create file stream. using (FileStream outputFileStream = new FileStream("Output.pdf", FileMode.Create, FileAccess.ReadWrite)) { //Save the PDF document to file stream. loadedDocument.Save(outputFileStream); } //Close the document. loadedDocument.Close(true);
By executing this code example, you will get a PDF like in the following image.
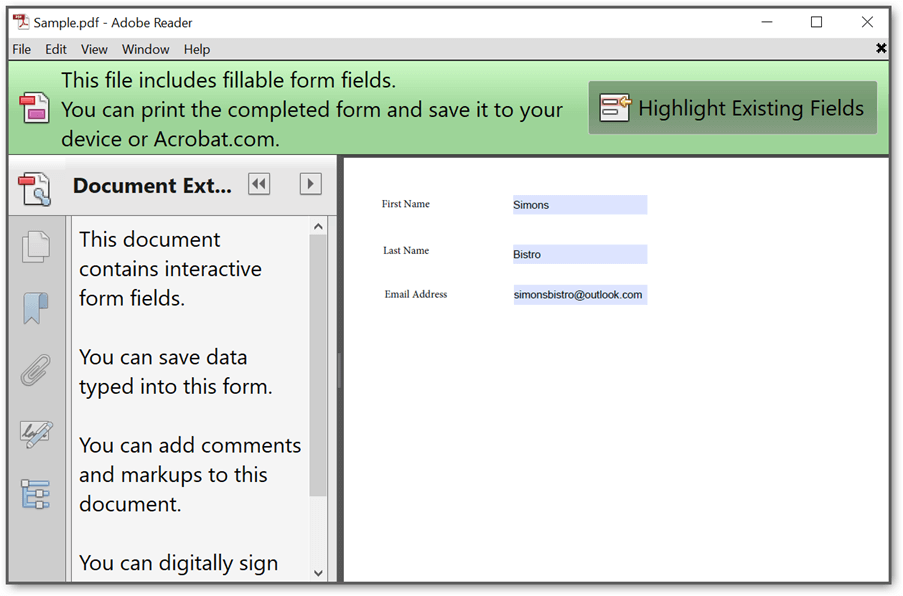

See a world of document processing possibilities in Syncfusion's PDF Library as we unveil its features in interactive demonstrations.
Utilizing JavaScript actions in PDF forms
The Syncfusion .NET PDF Library also supports adding JavaScript actions to the form fields using the PdfJavaScriptAction class:
- Create a new PDF document using the PdfDocument class.
- Then, add a new blank page in it using the PdfPage class.
- Use the PdfButtonField class to create a new button field.
- Set the JavaScript action for the submit button using the PdfJavaScriptAction class.
- Finally, save the PDF document using the Save method.
Refer to the following code example.
//Create a new PDF document. PdfDocument document = new PdfDocument(); //Create a new page. PdfPage page = document.Pages.Add(); //Create a new PdfButtonField. PdfButtonField submitButton = new PdfButtonField(page, "submitButton"); submitButton.Bounds = new RectangleF(25, 160, 100, 20); submitButton.Text = "Apply"; submitButton.BackColor = new PdfColor(181, 191, 203); //Create a new PdfJavaScriptAction. PdfJavaScriptAction scriptAction = new PdfJavaScriptAction("app.alert(\"You are looking at form fields action of PDF document\")"); //Set the scriptAction to submitButton. submitButton.Actions.MouseDown = scriptAction; //Add the submit button to the new document. document.Form.Fields.Add(submitButton); //Create file stream. using (FileStream outputFileStream = new FileStream("Output.pdf", FileMode.Create, FileAccess.ReadWrite)) { //Save the PDF document to file stream. document.Save(outputFileStream); } //Close the document. document.Close(true);
By executing this code example, you will get a PDF like in the following screenshot.
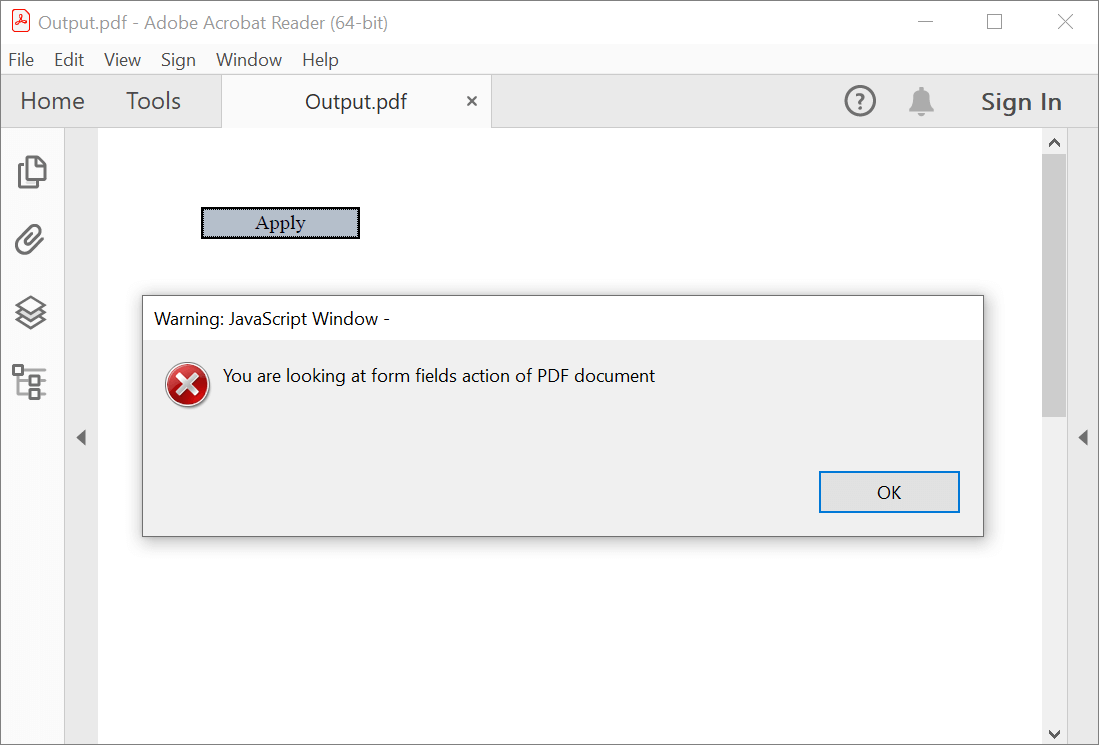
GitHub reference
For more details, refer to the create, fill, and edit fillable PDF forms using the C# demo on GitHub.

Join thousands of developers who rely on Syncfusion for their PDF needs. Experience the difference today!
Conclusion
Thanks for reading! In this blog, we’ve seen how to create, fill, and edit forms in PDF documents using the Syncfusion .NET PDF Library. Try out the steps in this blog post and leave your feedback in the comments section below.
Take a moment to look at the PDF forms documentation, where you can find other options and features, all with accompanying code examples.
For questions, you can contact us through our support forum, support portal, or feedback portal. We are always happy to assist you!