Data grid is a component that is used to display data in a tabular format. A conventional data grid component should include functionalities like data binding, sorting, grouping, editing, filtering, etc.
Syncfusion data grid has all the above and some additional functionalities like swiping, dragging, resizing, summaries, styling, paging, loading more items, pull-to-refresh, and exporting to Excel and PDF file formats. The best thing is that data grid is completely MVVM compatible and offers smooth and responsive touch scrolling in all directions upon binding with the commonly preferred data sources.
This blog explores the Syncfusion data grid component in Xamarin.Forms, which enables you to create a real-world data grid that renders the data collection from your view model into a table format. The below image represents the outcome of a simple data grid that has been built following the steps in this blog post.
Note: The data grid creation and configuration in the blog post is entirely explained with XAML logics. However, you can also do this with the code behind.
Configuring data grid
Data grid can be completely referenced using the NuGet package by following these steps in Visual Studio:
- Right-click on the solution and choose Manage NuGet Packages for Solution and select nuget.org from the package source.
- The NuGet packages listed (under the Browse tab) are available in the package source location. Search for data grid in the search box. Select “Syncfusion.Xamarin.SfDataGrid” from the search results.
- Select the project to which to install the package from the projects list shown and choose the appropriate version of the data grid to be installed from the drop-down.
- Install the package to your application by clicking Install.
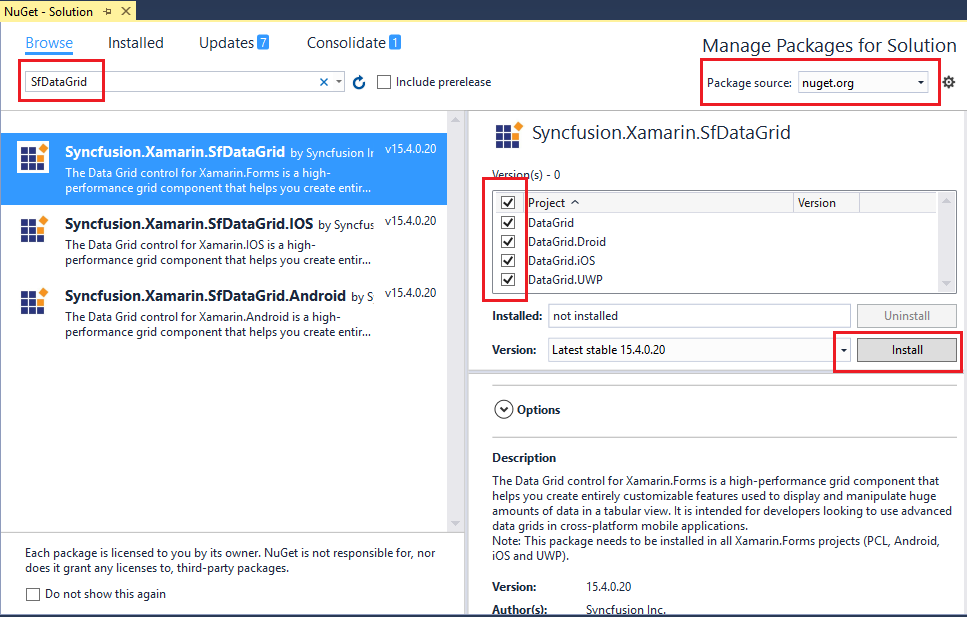
Note: In order to use the export to Excel and export to PDF functionalities of the data grid, install the NuGet Syncfusion.Xamarin.SfGridConverter from the nuget.org package source.
Creating data grid
Data grid is entirely configurable in both XAML and code behind. Follow the below steps to create a new data grid:
- Import the following data grid control namespace in the XAML page:
box-sizing: border-box; margin-top: 0px; margin-bottom: 10px;">
- Configure the data grid control either as content to the ContentPage or as a nested child.
<syncfusion:sfdatagrid x_name="dataGrid"/>
Launching grid on each platform
Androids launch the data grid without any initialization. However, an additional step is required for launching data grid (or any custom component) in iOS and UWP.
iOS project
Call the SfDataGridRenderer.Init() method in the FinishedLaunching overridden method of the AppDelegate class after the Xamarin.Forms Framework initialization and before the LoadApplication is called, as demonstrated in the following code example:
public override bool FinishedLaunching(UIApplication app, NSDictionary options) { Xamarin.Forms.Forms.Init(); ... Syncfusion.SfDataGrid.XForms.iOS.SfDataGridRenderer.Init(); ... LoadApplication(new App()); return base.FinishedLaunching(app, options); }
UWP project
Call the SfDataGridRenderer.Init() in the MainPage constructor before the LoadApplication is called, as demonstrated in the following code example:
public MainPage() { ... Syncfusion.SfDataGrid.XForms.UWP.SfDataGridRenderer.Init(); ... LoadApplication(new App()); }
For UWP alone, one more step is required if the project is built in release mode with .NET Native tool chain enabled, which is explained in the KB article here.
Configuring data grid with data
Data grid is a data-bound control. Hence, you must create a data model to bind it to the control.
Create a simple data source and bind it to data grid. In this case, a simple “order details to be shipped” has been modeled that generates random order details containing customer data, order information, and order values. In a real-world scenario, you can also bind the data from the services and database to render data grid.
Model class with properties
public class OrderInfo : INotifyPropertyChanged { public OrderInfo() { } #region private variables private ImageSource customerImage; private int orderID; ... private string shipCountry; private string freight; #endregion #region Public Properties public ImageSource CustomerImage { get { return this.customerImage; } set { this.customerImage = value; RaisePropertyChanged("CustomerImage"); } } public int OrderID { get { return this.orderID; } set { this.orderID = value; RaisePropertyChanged("OrderID"); } } ... public int ShipCountry { get { return this.shipCountry; } set { this.shipCountry = value; RaisePropertyChanged("ShipCountry"); } } public int Freight { get { return this.freight; } set { this.freight = value; RaisePropertyChanged("Freight"); } } #endregion #region INotifyPropertyChanged implementation public event PropertyChangedEventHandler PropertyChanged; private void RaisePropertyChanged(String Name) { if (PropertyChanged != null) this.PropertyChanged(this, new PropertyChangedEventArgs(Name)); } #endregion }
Populating the model collection
public class OrderInfoRepository { public OrderInfoRepository() { } private Random random = new Random(); public ObservableCollection<orderinfo> GetOrderDetails(int count) { ObservableCollection<orderinfo> orderDetails = new ObservableCollection<orderinfo>(); for (int i = 10001; i <= count + 10000; i++) { var ord = new OrderInfo() { CustomerImage = ImageSource.FromResource("DataGrid.Images.Image" + (i % 29) + ".png"), OrderID = i, ... ShipCountry = shipCountries[random.Next(5)], Freight = (Math.Round(random.Next(1000) + random.NextDouble(), 2)).ToString(), }; orderDetails.Add(ord); } return orderDetails; } private string[] shipCountries = new string[] { "Argentina", "Austria", … "Switzerland", "UK", "USA", "Venezuela" }; //Other data to be followed }
Configure the populated data or the data from the services or databases to a collection in the ViewModel. The collection can be any commonly preferred data sources. I would prefer ObservableCollection overall, as it has an internal INotifyCollectionChanged implementation, and as we generally expect the data grid to be responsive. If collection changes are not a concern, then a simple List can be used to hold the data, which is faster than the ObservableCollection .
public class ViewModel { public ViewModel() { SetRowstoGenerate(100); } private OrderInfoRepository order; private ObservableCollection<orderinfo> ordersInfo; public ObservableCollection<orderinfo> OrdersInfo { get { return ordersInfo; } set { this.ordersInfo = value; } } public void SetRowstoGenerate(int count) { order = new OrderInfoRepository(); ordersInfo = order.GetOrderDetails(count); } }
Binding data to data grid
In order to bind the data source to the data grid, simply bind the collection containing the data in your view model to the SfDataGrid.ItemsSource property as shown in the following.
Congratulations on configuring a simple data grid in your application. Just running the sample with above steps will render a beautiful data grid in your view.
The above screenshot shows the outcome of the grid without any additional settings. However, as an end user, you might want to exclude some columns from rendering, reorder the generation of the columns or generate columns of your choosing when using a data grid; those customization options when using data grid are explained below.
Defining columns
Data grid allows you to create and customize columns in two ways:
- Auto-generating columns
- Manually defining columns
Auto-generating columns
By default, the data grid automatically creates columns for all the properties in the data source. However, this can also be controlled by using the SfDataGrid.AutoGenerateColumns property. The type of column autogenerated depends on the type of data in the column.
When the columns are auto-generated, you can handle the SfDataGrid.AutoGeneratingColumn event to customize or remove the columns before they are rendered in data grid. You can subscribe the event either in the XAML or in code behind. You can also use Behaviors or EventToCommandBehavior to use this event if you are following MVVM.
private void DataGrid_AutoGeneratingColumn(object sender, AutoGeneratingColumnEventArgs e) { if (e.Column.MappingName == "CustomerImage" || e.Column.MappingName == "OrderID" || e.Column.MappingName == "ShipCountry" || e.Column.MappingName == "Freight") { e.Column.HeaderFontAttribute = FontAttributes.Bold; e.Column.HeaderTextAlignment = TextAlignment.Start; e.Column.LineBreakMode = LineBreakMode.TailTruncation; e.Column.Padding = new Thickness(5, 0, 5, 0); } else e.Cancel = true; }
Manually generating columns
You can also define the columns manually by setting the SfDataGrid.AutoGenerateColumns property to false and adding the GridColumn objects to the SfDataGrid.Columns collection. Below are the types of columns available in data grid:
- Text column
- Switch column
- Image column
- Numeric column
- Date-time column
- Picker column
- Template column
- Unbound column
- Row header column
The following code example illustrates creating columns manually in data grid.
<syncfusion:gridimagecolumn mappingname="CustomerImage" headertext="Customer" headerfontattribute="Bold"/> <syncfusion:gridtextcolumn mappingname="OrderID" headertext="Order ID" headerfontattribute="Bold"/> <syncfusion:gridtextcolumn mappingname="ShipCountry" headertext="Ship Country" headerfontattribute="Bold"/> <syncfusion:gridtextcolumn mappingname="Freight" headerfontattribute="Bold"/>
Summary
In this blog post, we looked at creating and configuring a Data Grid with data by automatically and manually creating columns. We only just touched the component; the best thing is, there are lots of other cool things you can do with data grid, including styling, data shaping and manipulation, swiping, and other interactive and advanced features that can be configured. Be sure to download the DataGrid application to deploy a readily runnable sample and see just how easy it is to create and configure beautiful data grids using Syncfusion!
Read Part 2 here: Overview of Data Grid in Xamarin.Forms Part 2
If you like this post, we think you’ll also enjoy: